add "LE Device Address" 0x1B to advertising data types
Fork of BLE_API by
GattService.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef __GATT_SERVICE_H__ 00018 #define __GATT_SERVICE_H__ 00019 00020 #include "UUID.h" 00021 #include "GattCharacteristic.h" 00022 00023 class GattService { 00024 public: 00025 enum { 00026 UUID_ALERT_NOTIFICATION_SERVICE = 0x1811, 00027 UUID_BATTERY_SERVICE = 0x180F, 00028 UUID_BLOOD_PRESSURE_SERVICE = 0x1810, 00029 UUID_CURRENT_TIME_SERVICE = 0x1805, 00030 UUID_CYCLING_SPEED_AND_CADENCE = 0x1816, 00031 UUID_DEVICE_INFORMATION_SERVICE = 0x180A, 00032 UUID_ENVIRONMENTAL_SERVICE = 0x181A, 00033 UUID_GLUCOSE_SERVICE = 0x1808, 00034 UUID_HEALTH_THERMOMETER_SERVICE = 0x1809, 00035 UUID_HEART_RATE_SERVICE = 0x180D, 00036 UUID_HUMAN_INTERFACE_DEVICE_SERVICE = 0x1812, 00037 UUID_IMMEDIATE_ALERT_SERVICE = 0x1802, 00038 UUID_LINK_LOSS_SERVICE = 0x1803, 00039 UUID_NEXT_DST_CHANGE_SERVICE = 0x1807, 00040 UUID_PHONE_ALERT_STATUS_SERVICE = 0x180E, 00041 UUID_REFERENCE_TIME_UPDATE_SERVICE = 0x1806, 00042 UUID_RUNNING_SPEED_AND_CADENCE = 0x1814, 00043 UUID_SCAN_PARAMETERS_SERVICE = 0x1813, 00044 UUID_TX_POWER_SERVICE = 0x1804 00045 }; 00046 00047 public: 00048 /** 00049 * @brief Creates a new GattService using the specified 16-bit 00050 * UUID, value length, and properties. 00051 * 00052 * @note The UUID value must be unique and is normally >1. 00053 * 00054 * @param[in] uuid 00055 * The UUID to use for this service. 00056 * @param[in] characteristics 00057 * A pointer to an array of characteristics to be included within this service. 00058 * @param[in] numCharacteristics 00059 * The number of characteristics. 00060 */ 00061 GattService(const UUID &uuid, GattCharacteristic *characteristics[], unsigned numCharacteristics) : 00062 _primaryServiceID(uuid), _characteristicCount(numCharacteristics), _characteristics(characteristics), _handle(0) { 00063 /* empty */ 00064 } 00065 00066 const UUID &getUUID(void) const {return _primaryServiceID; } 00067 uint16_t getHandle(void) const {return _handle; } 00068 uint8_t getCharacteristicCount(void) const {return _characteristicCount;} 00069 void setHandle(uint16_t handle) {_handle = handle;} 00070 00071 GattCharacteristic *getCharacteristic(uint8_t index) { 00072 if (index >= _characteristicCount) { 00073 return NULL; 00074 } 00075 00076 return _characteristics[index]; 00077 } 00078 00079 private: 00080 UUID _primaryServiceID; 00081 uint8_t _characteristicCount; 00082 GattCharacteristic **_characteristics; 00083 uint16_t _handle; 00084 }; 00085 00086 #endif // ifndef __GATT_SERVICE_H__
Generated on Fri Jul 15 2022 20:49:45 by
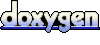