add "LE Device Address" 0x1B to advertising data types
Fork of BLE_API by
GattServer.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef __GATT_SERVER_H__ 00018 #define __GATT_SERVER_H__ 00019 00020 #include "Gap.h" 00021 #include "GattService.h" 00022 #include "GattAttribute.h" 00023 #include "GattServerEvents.h" 00024 #include "GattCallbackParamTypes.h" 00025 #include "CallChainOfFunctionPointersWithContext.h" 00026 00027 class GattServer { 00028 public: 00029 /* Event callback handlers. */ 00030 typedef FunctionPointerWithContext<unsigned> DataSentCallback_t; 00031 typedef CallChainOfFunctionPointersWithContext<unsigned> DataSentCallbackChain_t; 00032 00033 typedef FunctionPointerWithContext<const GattWriteCallbackParams*> DataWrittenCallback_t; 00034 typedef CallChainOfFunctionPointersWithContext<const GattWriteCallbackParams*> DataWrittenCallbackChain_t; 00035 00036 typedef FunctionPointerWithContext<const GattReadCallbackParams*> DataReadCallback_t; 00037 typedef CallChainOfFunctionPointersWithContext<const GattReadCallbackParams *> DataReadCallbackChain_t; 00038 00039 typedef FunctionPointerWithContext<const GattServer *> GattServerShutdownCallback_t; 00040 typedef CallChainOfFunctionPointersWithContext<const GattServer *> GattServerShutdownCallbackChain_t; 00041 00042 typedef FunctionPointerWithContext<GattAttribute::Handle_t> EventCallback_t; 00043 00044 protected: 00045 GattServer() : 00046 serviceCount(0), 00047 characteristicCount(0), 00048 dataSentCallChain(), 00049 dataWrittenCallChain(), 00050 dataReadCallChain(), 00051 updatesEnabledCallback(NULL), 00052 updatesDisabledCallback(NULL), 00053 confirmationReceivedCallback(NULL) { 00054 /* empty */ 00055 } 00056 00057 /* 00058 * The following functions are meant to be overridden in the platform-specific sub-class. 00059 */ 00060 public: 00061 00062 /** 00063 * Add a service declaration to the local server ATT table. Also add the 00064 * characteristics contained within. 00065 */ 00066 virtual ble_error_t addService(GattService &service) { 00067 /* Avoid compiler warnings about unused variables. */ 00068 (void)service; 00069 00070 return BLE_ERROR_NOT_IMPLEMENTED; /* Requesting action from porters: override this API if this capability is supported. */ 00071 } 00072 00073 /** 00074 * Read the value of a characteristic from the local GATT server. 00075 * @param[in] attributeHandle 00076 * Attribute handle for the value attribute of the characteristic. 00077 * @param[out] buffer 00078 * A buffer to hold the value being read. 00079 * @param[in/out] lengthP 00080 * Length of the buffer being supplied. If the attribute 00081 * value is longer than the size of the supplied buffer, 00082 * this variable will hold upon return the total attribute value length 00083 * (excluding offset). The application may use this 00084 * information to allocate a suitable buffer size. 00085 * 00086 * @return BLE_ERROR_NONE if a value was read successfully into the buffer. 00087 */ 00088 virtual ble_error_t read(GattAttribute::Handle_t attributeHandle, uint8_t buffer[], uint16_t *lengthP) { 00089 /* Avoid compiler warnings about unused variables. */ 00090 (void)attributeHandle; 00091 (void)buffer; 00092 (void)lengthP; 00093 00094 return BLE_ERROR_NOT_IMPLEMENTED; /* Requesting action from porters: override this API if this capability is supported. */ 00095 } 00096 00097 /** 00098 * Read the value of a characteristic from the local GATT server. 00099 * @param[in] connectionHandle 00100 * Connection handle. 00101 * @param[in] attributeHandle 00102 * Attribute handle for the value attribute of the characteristic. 00103 * @param[out] buffer 00104 * A buffer to hold the value being read. 00105 * @param[in/out] lengthP 00106 * Length of the buffer being supplied. If the attribute 00107 * value is longer than the size of the supplied buffer, 00108 * this variable will hold upon return the total attribute value length 00109 * (excluding offset). The application may use this 00110 * information to allocate a suitable buffer size. 00111 * 00112 * @return BLE_ERROR_NONE if a value was read successfully into the buffer. 00113 * 00114 * @note This API is a version of the above, with an additional connection handle 00115 * parameter to allow fetches for connection-specific multivalued 00116 * attributes (such as the CCCDs). 00117 */ 00118 virtual ble_error_t read(Gap::Handle_t connectionHandle, GattAttribute::Handle_t attributeHandle, uint8_t *buffer, uint16_t *lengthP) { 00119 /* Avoid compiler warnings about unused variables. */ 00120 (void)connectionHandle; 00121 (void)attributeHandle; 00122 (void)buffer; 00123 (void)lengthP; 00124 00125 return BLE_ERROR_NOT_IMPLEMENTED; /* Requesting action from porters: override this API if this capability is supported. */ 00126 } 00127 00128 /** 00129 * Update the value of a characteristic on the local GATT server. 00130 * 00131 * @param[in] attributeHandle 00132 * Handle for the value attribute of the characteristic. 00133 * @param[in] value 00134 * A pointer to a buffer holding the new value. 00135 * @param[in] size 00136 * Size of the new value (in bytes). 00137 * @param[in] localOnly 00138 * Should this update be kept on the local 00139 * GATT server regardless of the state of the 00140 * notify/indicate flag in the CCCD for this 00141 * Characteristic? If set to true, no notification 00142 * or indication is generated. 00143 * 00144 * @return BLE_ERROR_NONE if we have successfully set the value of the attribute. 00145 */ 00146 virtual ble_error_t write(GattAttribute::Handle_t attributeHandle, const uint8_t *value, uint16_t size, bool localOnly = false) { 00147 /* Avoid compiler warnings about unused variables. */ 00148 (void)attributeHandle; 00149 (void)value; 00150 (void)size; 00151 (void)localOnly; 00152 00153 return BLE_ERROR_NOT_IMPLEMENTED; /* Requesting action from porters: override this API if this capability is supported. */ 00154 } 00155 00156 /** 00157 * Update the value of a characteristic on the local GATT server. A version 00158 * of the same as the above, with a connection handle parameter to allow updates 00159 * for connection-specific multivalued attributes (such as the CCCDs). 00160 * 00161 * @param[in] connectionHandle 00162 * Connection handle. 00163 * @param[in] attributeHandle 00164 * Handle for the value attribute of the characteristic. 00165 * @param[in] value 00166 * A pointer to a buffer holding the new value. 00167 * @param[in] size 00168 * Size of the new value (in bytes). 00169 * @param[in] localOnly 00170 * Should this update be kept on the local 00171 * GattServer regardless of the state of the 00172 * notify/indicate flag in the CCCD for this 00173 * Characteristic? If set to true, no notification 00174 * or indication is generated. 00175 * 00176 * @return BLE_ERROR_NONE if we have successfully set the value of the attribute. 00177 */ 00178 virtual ble_error_t write(Gap::Handle_t connectionHandle, GattAttribute::Handle_t attributeHandle, const uint8_t *value, uint16_t size, bool localOnly = false) { 00179 /* Avoid compiler warnings about unused variables. */ 00180 (void)connectionHandle; 00181 (void)attributeHandle; 00182 (void)value; 00183 (void)size; 00184 (void)localOnly; 00185 00186 return BLE_ERROR_NOT_IMPLEMENTED; /* Requesting action from porters: override this API if this capability is supported. */ 00187 } 00188 00189 /** 00190 * Determine the updates-enabled status (notification or indication) for the current connection from a characteristic's CCCD. 00191 * 00192 * @param characteristic 00193 * The characteristic. 00194 * @param[out] enabledP 00195 * Upon return, *enabledP is true if updates are enabled, else false. 00196 * 00197 * @return BLE_ERROR_NONE if the connection and handle are found. False otherwise. 00198 */ 00199 virtual ble_error_t areUpdatesEnabled(const GattCharacteristic &characteristic, bool *enabledP) { 00200 /* Avoid compiler warnings about unused variables. */ 00201 (void)characteristic; 00202 (void)enabledP; 00203 00204 return BLE_ERROR_NOT_IMPLEMENTED; /* Requesting action from porters: override this API if this capability is supported. */ 00205 } 00206 00207 /** 00208 * Determine the connection-specific updates-enabled status (notification or indication) from a characteristic's CCCD. 00209 * 00210 * @param connectionHandle 00211 * The connection handle. 00212 * @param[out] enabledP 00213 * Upon return, *enabledP is true if updates are enabled, else false. 00214 * 00215 * @param characteristic 00216 * The characteristic. 00217 * 00218 * @return BLE_ERROR_NONE if the connection and handle are found. False otherwise. 00219 */ 00220 virtual ble_error_t areUpdatesEnabled(Gap::Handle_t connectionHandle, const GattCharacteristic &characteristic, bool *enabledP) { 00221 /* Avoid compiler warnings about unused variables. */ 00222 (void)connectionHandle; 00223 (void)characteristic; 00224 (void)enabledP; 00225 00226 return BLE_ERROR_NOT_IMPLEMENTED; /* Requesting action from porters: override this API if this capability is supported. */ 00227 } 00228 00229 /** 00230 * A virtual function to allow underlying stacks to indicate if they support 00231 * onDataRead(). It should be overridden to return true as applicable. 00232 */ 00233 virtual bool isOnDataReadAvailable() const { 00234 return false; /* Requesting action from porters: override this API if this capability is supported. */ 00235 } 00236 00237 /* 00238 * APIs with non-virtual implementations. 00239 */ 00240 public: 00241 /** 00242 * Add a callback for the GATT event DATA_SENT (which is triggered when 00243 * updates are sent out by GATT in the form of notifications). 00244 * 00245 * @Note: It is possible to chain together multiple onDataSent callbacks 00246 * (potentially from different modules of an application) to receive updates 00247 * to characteristics. 00248 * 00249 * @Note: It is also possible to set up a callback into a member function of 00250 * some object. 00251 */ 00252 void onDataSent(const DataSentCallback_t& callback) {dataSentCallChain.add(callback);} 00253 template <typename T> 00254 void onDataSent(T *objPtr, void (T::*memberPtr)(unsigned count)) { 00255 dataSentCallChain.add(objPtr, memberPtr); 00256 } 00257 00258 /** 00259 * @brief get the callback chain called when the event DATA_EVENT is triggered. 00260 */ 00261 DataSentCallbackChain_t& onDataSent() { 00262 return dataSentCallChain; 00263 } 00264 00265 /** 00266 * Set up a callback for when an attribute has its value updated by or at the 00267 * connected peer. For a peripheral, this callback is triggered when the local 00268 * GATT server has an attribute updated by a write command from the peer. 00269 * For a central, this callback is triggered when a response is received for 00270 * a write request. 00271 * 00272 * @Note: It is possible to chain together multiple onDataWritten callbacks 00273 * (potentially from different modules of an application) to receive updates 00274 * to characteristics. Many services, such as DFU and UART, add their own 00275 * onDataWritten callbacks behind the scenes to trap interesting events. 00276 * 00277 * @Note: It is also possible to set up a callback into a member function of 00278 * some object. 00279 * 00280 * @Note It is possible to unregister a callback using onDataWritten().detach(callback) 00281 */ 00282 void onDataWritten(const DataWrittenCallback_t& callback) {dataWrittenCallChain.add(callback);} 00283 template <typename T> 00284 void onDataWritten(T *objPtr, void (T::*memberPtr)(const GattWriteCallbackParams *context)) { 00285 dataWrittenCallChain.add(objPtr, memberPtr); 00286 } 00287 00288 /** 00289 * @brief provide access to the callchain of data written event callbacks 00290 * It is possible to register callbacks using onDataWritten().add(callback); 00291 * It is possible to unregister callbacks using onDataWritten().detach(callback) 00292 * @return The data written event callbacks chain 00293 */ 00294 DataWrittenCallbackChain_t& onDataWritten() { 00295 return dataWrittenCallChain; 00296 } 00297 00298 /** 00299 * Setup a callback to be invoked on the peripheral when an attribute is 00300 * being read by a remote client. 00301 * 00302 * @Note: This functionality may not be available on all underlying stacks. 00303 * You could use GattCharacteristic::setReadAuthorizationCallback() as an 00304 * alternative. Refer to isOnDataReadAvailable(). 00305 * 00306 * @Note: It is possible to chain together multiple onDataRead callbacks 00307 * (potentially from different modules of an application) to receive updates 00308 * to characteristics. Services may add their own onDataRead callbacks 00309 * behind the scenes to trap interesting events. 00310 * 00311 * @Note: It is also possible to set up a callback into a member function of 00312 * some object. 00313 * 00314 * @Note It is possible to unregister a callback using onDataRead().detach(callback) 00315 * 00316 * @return BLE_ERROR_NOT_IMPLEMENTED if this functionality isn't available; 00317 * else BLE_ERROR_NONE. 00318 */ 00319 ble_error_t onDataRead(const DataReadCallback_t& callback) { 00320 if (!isOnDataReadAvailable()) { 00321 return BLE_ERROR_NOT_IMPLEMENTED; 00322 } 00323 00324 dataReadCallChain.add(callback); 00325 return BLE_ERROR_NONE; 00326 } 00327 template <typename T> 00328 ble_error_t onDataRead(T *objPtr, void (T::*memberPtr)(const GattReadCallbackParams *context)) { 00329 if (!isOnDataReadAvailable()) { 00330 return BLE_ERROR_NOT_IMPLEMENTED; 00331 } 00332 00333 dataReadCallChain.add(objPtr, memberPtr); 00334 return BLE_ERROR_NONE; 00335 } 00336 00337 /** 00338 * @brief provide access to the callchain of data read event callbacks 00339 * It is possible to register callbacks using onDataRead().add(callback); 00340 * It is possible to unregister callbacks using onDataRead().detach(callback) 00341 * @return The data read event callbacks chain 00342 */ 00343 DataReadCallbackChain_t& onDataRead() { 00344 return dataReadCallChain; 00345 } 00346 00347 /** 00348 * Setup a callback to be invoked to notify the user application that the 00349 * GattServer instance is about to shutdown (possibly as a result of a call 00350 * to BLE::shutdown()). 00351 * 00352 * @Note: It is possible to chain together multiple onShutdown callbacks 00353 * (potentially from different modules of an application) to be notified 00354 * before the GattServer is shutdown. 00355 * 00356 * @Note: It is also possible to set up a callback into a member function of 00357 * some object. 00358 * 00359 * @Note It is possible to unregister a callback using onShutdown().detach(callback) 00360 */ 00361 void onShutdown(const GattServerShutdownCallback_t& callback) { 00362 shutdownCallChain.add(callback); 00363 } 00364 template <typename T> 00365 void onShutdown(T *objPtr, void (T::*memberPtr)(void)) { 00366 shutdownCallChain.add(objPtr, memberPtr); 00367 } 00368 00369 /** 00370 * @brief provide access to the callchain of shutdown event callbacks 00371 * It is possible to register callbacks using onShutdown().add(callback); 00372 * It is possible to unregister callbacks using onShutdown().detach(callback) 00373 * @return The shutdown event callbacks chain 00374 */ 00375 GattServerShutdownCallbackChain_t& onShutdown() { 00376 return shutdownCallChain; 00377 } 00378 00379 /** 00380 * Set up a callback for when notifications or indications are enabled for a 00381 * characteristic on the local GATT server. 00382 */ 00383 void onUpdatesEnabled(EventCallback_t callback) {updatesEnabledCallback = callback;} 00384 00385 /** 00386 * Set up a callback for when notifications or indications are disabled for a 00387 * characteristic on the local GATT server. 00388 */ 00389 void onUpdatesDisabled(EventCallback_t callback) {updatesDisabledCallback = callback;} 00390 00391 /** 00392 * Set up a callback for when the GATT server receives a response for an 00393 * indication event sent previously. 00394 */ 00395 void onConfirmationReceived(EventCallback_t callback) {confirmationReceivedCallback = callback;} 00396 00397 /* Entry points for the underlying stack to report events back to the user. */ 00398 protected: 00399 void handleDataWrittenEvent(const GattWriteCallbackParams *params) { 00400 dataWrittenCallChain.call(params); 00401 } 00402 00403 void handleDataReadEvent(const GattReadCallbackParams *params) { 00404 dataReadCallChain.call(params); 00405 } 00406 00407 void handleEvent(GattServerEvents::gattEvent_e type, GattAttribute::Handle_t attributeHandle) { 00408 switch (type) { 00409 case GattServerEvents::GATT_EVENT_UPDATES_ENABLED: 00410 if (updatesEnabledCallback) { 00411 updatesEnabledCallback(attributeHandle); 00412 } 00413 break; 00414 case GattServerEvents::GATT_EVENT_UPDATES_DISABLED: 00415 if (updatesDisabledCallback) { 00416 updatesDisabledCallback(attributeHandle); 00417 } 00418 break; 00419 case GattServerEvents::GATT_EVENT_CONFIRMATION_RECEIVED: 00420 if (confirmationReceivedCallback) { 00421 confirmationReceivedCallback(attributeHandle); 00422 } 00423 break; 00424 default: 00425 break; 00426 } 00427 } 00428 00429 void handleDataSentEvent(unsigned count) { 00430 dataSentCallChain.call(count); 00431 } 00432 00433 public: 00434 /** 00435 * Notify all registered onShutdown callbacks that the GattServer is 00436 * about to be shutdown and clear all GattServer state of the 00437 * associated object. 00438 * 00439 * This function is meant to be overridden in the platform-specific 00440 * sub-class. Nevertheless, the sub-class is only expected to reset its 00441 * state and not the data held in GattServer members. This shall be achieved 00442 * by a call to GattServer::reset() from the sub-class' reset() 00443 * implementation. 00444 * 00445 * @return BLE_ERROR_NONE on success. 00446 */ 00447 virtual ble_error_t reset(void) { 00448 /* Notify that the instance is about to shutdown */ 00449 shutdownCallChain.call(this); 00450 shutdownCallChain.clear(); 00451 00452 serviceCount = 0; 00453 characteristicCount = 0; 00454 00455 dataSentCallChain.clear(); 00456 dataWrittenCallChain.clear(); 00457 dataReadCallChain.clear(); 00458 updatesEnabledCallback = NULL; 00459 updatesDisabledCallback = NULL; 00460 confirmationReceivedCallback = NULL; 00461 00462 return BLE_ERROR_NONE; 00463 } 00464 00465 protected: 00466 uint8_t serviceCount; 00467 uint8_t characteristicCount; 00468 00469 private: 00470 DataSentCallbackChain_t dataSentCallChain; 00471 DataWrittenCallbackChain_t dataWrittenCallChain; 00472 DataReadCallbackChain_t dataReadCallChain; 00473 GattServerShutdownCallbackChain_t shutdownCallChain; 00474 EventCallback_t updatesEnabledCallback; 00475 EventCallback_t updatesDisabledCallback; 00476 EventCallback_t confirmationReceivedCallback; 00477 00478 private: 00479 /* Disallow copy and assignment. */ 00480 GattServer(const GattServer &); 00481 GattServer& operator=(const GattServer &); 00482 }; 00483 00484 #endif // ifndef __GATT_SERVER_H__
Generated on Fri Jul 15 2022 20:49:45 by
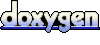