A stack which works with or without an Mbed os library. Provides IPv4 or IPv6 with a full 1500 byte buffer.
Dependents: oldheating gps motorhome heating
link.c
00001 #include <stdbool.h> 00002 00003 #include "gpio.h" 00004 #include "log.h" 00005 #include "eth.h" 00006 #include "action.h" 00007 #include "mac.h" 00008 #include "net.h" 00009 #include "link.h" 00010 #include "nic.h" 00011 #include "led.h" 00012 #include "restart.h" 00013 #include "jack.h" 00014 00015 #define LINK_PIN FIO1PIN(25) 00016 #define SPEED_PIN FIO1PIN(26) 00017 00018 bool LinkTrace = false; 00019 00020 void LinkMain() 00021 { 00022 int lastRestartPoint = RestartPoint; 00023 RestartPoint = FAULT_POINT_LinkMain; 00024 00025 //Wait until the network is up 00026 if (!NicLinkIsUp()) 00027 { 00028 RestartPoint = lastRestartPoint; 00029 return; 00030 } 00031 00032 //Reset the trace of host at the very start 00033 NetTraceHostResetMatched(); 00034 00035 //Handle packets 00036 int sizeRx; 00037 int sizeTx; 00038 char* pRx = NicGetReceivedPacketOrNull(&sizeRx); 00039 char* pTx = NicGetTransmitPacketOrNull(&sizeTx); 00040 00041 int action = DO_NOTHING; 00042 bool activity = false; 00043 if (pRx) 00044 { 00045 activity = true; 00046 00047 if (pTx) action = EthHandlePacket(pRx, sizeRx, pTx, &sizeTx); 00048 00049 NicReleaseReceivedPacket(); 00050 } 00051 00052 if (pTx) 00053 { 00054 if (!action) action = EthPollForPacketToSend(pTx, &sizeTx); 00055 00056 if ( action) 00057 { 00058 activity = true; 00059 NicSendTransmitPacket(sizeTx); 00060 } 00061 } 00062 00063 //Flash lights 00064 JackLeds(!LINK_PIN, !SPEED_PIN, activity); 00065 00066 //Finish 00067 RestartPoint = lastRestartPoint; 00068 } 00069 00070 void LinkInit() 00071 { 00072 JackInit(); 00073 NicInit(); 00074 NicLinkAddress(MacLocal); 00075 if (LinkTrace) 00076 { 00077 LogTime("MAC "); 00078 MacLog(MacLocal); 00079 Log("\r\n"); 00080 } 00081 }
Generated on Tue Jul 12 2022 18:53:40 by
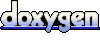