USB device stack, fixes for USBSerial & KL25Z
Fork of USBDevice by
USBMouseKeyboard.h
00001 /* Copyright (c) 2010-2011 mbed.org, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without 00005 * restriction, including without limitation the rights to use, copy, modify, merge, publish, 00006 * distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the 00007 * Software is furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 00019 #ifndef USBMOUSEKEYBOARD_H 00020 #define USBMOUSEKEYBOARD_H 00021 00022 #define REPORT_ID_KEYBOARD 1 00023 #define REPORT_ID_MOUSE 2 00024 #define REPORT_ID_VOLUME 3 00025 00026 #include "USBMouse.h" 00027 #include "USBKeyboard.h" 00028 #include "Stream.h" 00029 #include "USBHID.h" 00030 00031 /** 00032 * USBMouseKeyboard example 00033 * @code 00034 * 00035 * #include "mbed.h" 00036 * #include "USBMouseKeyboard.h" 00037 * 00038 * USBMouseKeyboard key_mouse; 00039 * 00040 * int main(void) 00041 * { 00042 * while(1) 00043 * { 00044 * key_mouse.move(20, 0); 00045 * key_mouse.printf("Hello From MBED\r\n"); 00046 * wait(1); 00047 * } 00048 * } 00049 * @endcode 00050 * 00051 * 00052 * @code 00053 * 00054 * #include "mbed.h" 00055 * #include "USBMouseKeyboard.h" 00056 * 00057 * USBMouseKeyboard key_mouse(ABS_MOUSE); 00058 * 00059 * int main(void) 00060 * { 00061 * while(1) 00062 * { 00063 * key_mouse.move(X_MAX_ABS/2, Y_MAX_ABS/2); 00064 * key_mouse.printf("Hello from MBED\r\n"); 00065 * wait(1); 00066 * } 00067 * } 00068 * @endcode 00069 */ 00070 class USBMouseKeyboard: public USBHID, public Stream 00071 { 00072 public: 00073 00074 /** 00075 * Constructor 00076 * 00077 * @param mouse_type Mouse type: ABS_MOUSE (absolute mouse) or REL_MOUSE (relative mouse) (default: REL_MOUSE) 00078 * @param leds Leds bus: first: NUM_LOCK, second: CAPS_LOCK, third: SCROLL_LOCK 00079 * @param vendor_id Your vendor_id (default: 0x1234) 00080 * @param product_id Your product_id (default: 0x0001) 00081 * @param product_release Your preoduct_release (default: 0x0001) 00082 * 00083 */ 00084 USBMouseKeyboard(MOUSE_TYPE mouse_type = REL_MOUSE, uint16_t vendor_id = 0x0021, uint16_t product_id = 0x0011, uint16_t product_release = 0x0001): 00085 USBHID(0, 0, vendor_id, product_id, product_release, false) 00086 { 00087 lock_status = 0; 00088 button = 0; 00089 this->mouse_type = mouse_type; 00090 connect(); 00091 }; 00092 00093 /** 00094 * Write a state of the mouse 00095 * 00096 * @param x x-axis position 00097 * @param y y-axis position 00098 * @param buttons buttons state (first bit represents MOUSE_LEFT, second bit MOUSE_RIGHT and third bit MOUSE_MIDDLE) 00099 * @param z wheel state (>0 to scroll down, <0 to scroll up) 00100 * @returns true if there is no error, false otherwise 00101 */ 00102 bool update(int16_t x, int16_t y, uint8_t buttons, int8_t z); 00103 00104 00105 /** 00106 * Move the cursor to (x, y) 00107 * 00108 * @param x x-axis position 00109 * @param y y-axis position 00110 * @returns true if there is no error, false otherwise 00111 */ 00112 bool move(int16_t x, int16_t y); 00113 00114 /** 00115 * Press one or several buttons 00116 * 00117 * @param button button state (ex: press(MOUSE_LEFT)) 00118 * @returns true if there is no error, false otherwise 00119 */ 00120 bool press(uint8_t button); 00121 00122 /** 00123 * Release one or several buttons 00124 * 00125 * @param button button state (ex: release(MOUSE_LEFT)) 00126 * @returns true if there is no error, false otherwise 00127 */ 00128 bool release(uint8_t button); 00129 00130 /** 00131 * Double click (MOUSE_LEFT) 00132 * 00133 * @returns true if there is no error, false otherwise 00134 */ 00135 bool doubleClick(); 00136 00137 /** 00138 * Click 00139 * 00140 * @param button state of the buttons ( ex: clic(MOUSE_LEFT)) 00141 * @returns true if there is no error, false otherwise 00142 */ 00143 bool click(uint8_t button); 00144 00145 /** 00146 * Scrolling 00147 * 00148 * @param z value of the wheel (>0 to go down, <0 to go up) 00149 * @returns true if there is no error, false otherwise 00150 */ 00151 bool scroll(int8_t z); 00152 00153 /** 00154 * To send a character defined by a modifier(CTRL, SHIFT, ALT) and the key 00155 * 00156 * @code 00157 * //To send CTRL + s (save) 00158 * keyboard.keyCode('s', KEY_CTRL); 00159 * @endcode 00160 * 00161 * @param modifier bit 0: KEY_CTRL, bit 1: KEY_SHIFT, bit 2: KEY_ALT (default: 0) 00162 * @param key character to send 00163 * @returns true if there is no error, false otherwise 00164 */ 00165 bool keyCode(uint8_t key, uint8_t modifier = 0); 00166 00167 /** 00168 * Send a character 00169 * 00170 * @param c character to be sent 00171 * @returns true if there is no error, false otherwise 00172 */ 00173 virtual int _putc(int c); 00174 00175 /** 00176 * Control media keys 00177 * 00178 * @param key media key pressed (KEY_NEXT_TRACK, KEY_PREVIOUS_TRACK, KEY_STOP, KEY_PLAY_PAUSE, KEY_MUTE, KEY_VOLUME_UP, KEY_VOLUME_DOWN) 00179 * @returns true if there is no error, false otherwise 00180 */ 00181 bool mediaControl(MEDIA_KEY key); 00182 00183 /** 00184 * Read status of lock keys. Useful to switch-on/off leds according to key pressed. Only the first three bits of the result is important: 00185 * - First bit: NUM_LOCK 00186 * - Second bit: CAPS_LOCK 00187 * - Third bit: SCROLL_LOCK 00188 * 00189 * @returns status of lock keys 00190 */ 00191 uint8_t lockStatus(); 00192 00193 /* 00194 * To define the report descriptor. Warning: this method has to store the length of the report descriptor in reportLength. 00195 * 00196 * @returns pointer to the report descriptor 00197 */ 00198 virtual uint8_t * reportDesc(); 00199 00200 /* 00201 * Called when a data is received on the OUT endpoint. Useful to switch on LED of LOCK keys 00202 * 00203 * @returns if handle by subclass, return true 00204 */ 00205 virtual bool EP1_OUT_callback(); 00206 00207 00208 private: 00209 bool mouseWrite(int8_t x, int8_t y, uint8_t buttons, int8_t z); 00210 MOUSE_TYPE mouse_type; 00211 uint8_t button; 00212 bool mouseSend(int8_t x, int8_t y, uint8_t buttons, int8_t z); 00213 00214 uint8_t lock_status; 00215 00216 //dummy otherwise it doesn't compile (we must define all methods of an abstract class) 00217 virtual int _getc() { return -1;} 00218 }; 00219 00220 #endif
Generated on Wed Jul 13 2022 17:00:18 by
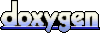