
Codebase from CC1101_Transceiver, ported to LPC1114/LPC824/STM32F103 and other micros, will be merged with panStamp project to replace AVR/MSP.
Fork of CC1101_Transceiver_LPC1114 by
CC1101 Class Reference
CC1101 Low-Power Sub-1 GHz RF Transceiver . More...
#include <CC1101.h>
Public Member Functions | |
CC1101 (PinName mosi, PinName miso, PinName clk, PinName csn, PinName RDmiso) | |
Constructor. | |
void | init (void) |
Initialize CC1101 parameters. | |
unsigned char | ReadReg (unsigned char addr) |
This function gets the value of a single specified CCxxx0 register. | |
void | ReadBurstReg (unsigned char addr, unsigned char *buffer, unsigned char count) |
This function reads multiple CCxxx0 register, using SPI burst access. | |
void | WriteReg (unsigned char addr, unsigned char value) |
Function for writing to a single CCxxx0 register. | |
void | WriteBurstReg (unsigned char addr, unsigned char *buffer, unsigned char count) |
This function writes to multiple CCxxx0 register, using SPI burst access. | |
void | SendPacket (unsigned char *txBuffer, unsigned char size) |
This function can be used to transmit a packet with packet length up to 63 bytes. | |
unsigned char | TxFifoEmpty (void) |
This function check if the TX FIFO is empty. | |
int | ReceivePacket (unsigned char *rxBuffer, unsigned char *length) |
This function can be used to receive a packet of variable packet length (first byte in the packet must be the length byte). | |
unsigned char | RxFifoEmpty (void) |
This function check if the RX FIFO is empty. | |
unsigned char | ReadChipStatusRX (void) |
This function returns the Chip Status RX register. | |
unsigned char | ReadChipStatusTX (void) |
This function returns the Chip Status TX register. | |
unsigned char | RdRSSI (void) |
This function returns the RSSI value based from the last packet received. | |
unsigned char | RdLQI (void) |
This function returns the LQI value based from the last packet received. | |
void | FlushRX (void) |
This function flushes the RX FIFO buffer. | |
void | FlushTX (void) |
This function flushes the TX FIFO buffer. | |
void | RXMode (void) |
This function change the state of CC1101 to RX mode. |
Detailed Description
CC1101 Low-Power Sub-1 GHz RF Transceiver .
Definition at line 211 of file CC1101.h.
Constructor & Destructor Documentation
CC1101 | ( | PinName | mosi, |
PinName | miso, | ||
PinName | clk, | ||
PinName | csn, | ||
PinName | RDmiso | ||
) |
Constructor.
- Parameters:
-
mosi mbed pin to use for MOSI line of SPI interface. miso mbed pin to use for MISO line of SPI interface. clk mbed pin to use for SCK line of SPI interface. csn mbed pin to use for not chip select line of SPI interface. RDmiso mbed pin connected to SPI MISO pin for CC1101 RDY read.
Definition at line 7 of file CC1101.cpp.
Member Function Documentation
void FlushRX | ( | void | ) |
This function flushes the RX FIFO buffer.
Definition at line 516 of file CC1101.cpp.
void FlushTX | ( | void | ) |
This function flushes the TX FIFO buffer.
Definition at line 525 of file CC1101.cpp.
void init | ( | void | ) |
Initialize CC1101 parameters.
Definition at line 214 of file CC1101.cpp.
unsigned char RdLQI | ( | void | ) |
This function returns the LQI value based from the last packet received.
- Returns:
- Return the LQI value.
Definition at line 349 of file CC1101.cpp.
unsigned char RdRSSI | ( | void | ) |
This function returns the RSSI value based from the last packet received.
- Returns:
- Return the RSSI value.
Definition at line 330 of file CC1101.cpp.
void ReadBurstReg | ( | unsigned char | addr, |
unsigned char * | buffer, | ||
unsigned char | count | ||
) |
This function reads multiple CCxxx0 register, using SPI burst access.
- Parameters:
-
addr Value of the accessed CCxxx0 register *buffer Pointer to a byte array which stores the values read from a corresponding range of CCxxx0 registers. count Number of bytes to be read from the subsequent CCxxx0 registers.
Definition at line 261 of file CC1101.cpp.
unsigned char ReadChipStatusRX | ( | void | ) |
This function returns the Chip Status RX register.
- Returns:
- Return the Chip Status RX register
Definition at line 503 of file CC1101.cpp.
unsigned char ReadChipStatusTX | ( | void | ) |
This function returns the Chip Status TX register.
- Returns:
- Return the Chip Status TX register
Definition at line 495 of file CC1101.cpp.
unsigned char ReadReg | ( | unsigned char | addr ) |
This function gets the value of a single specified CCxxx0 register.
- Parameters:
-
addr Value of the accessed CCxxx0 register
- Returns:
- Value of the accessed CCxxx0 register
Definition at line 233 of file CC1101.cpp.
int ReceivePacket | ( | unsigned char * | rxBuffer, |
unsigned char * | length | ||
) |
This function can be used to receive a packet of variable packet length (first byte in the packet must be the length byte).
The packet length should not exceed the RX FIFO size.
- Parameters:
-
*rxBuffer Pointer to the buffer where the incoming data should be stored *length Pointer to a variable containing the size of the buffer where the incoming data should be stored. After this function returns, that variable holds the packet length.
- Returns:
- Return value is 1 if CRC OK or else 0 if CRC NOT OK (or no packet was put in the RX FIFO due to filtering)
Definition at line 401 of file CC1101.cpp.
unsigned char RxFifoEmpty | ( | void | ) |
This function check if the RX FIFO is empty.
- Returns:
- Return value is 1 if the RX FIFO buffer is empty or else 0
Definition at line 357 of file CC1101.cpp.
void RXMode | ( | void | ) |
This function change the state of CC1101 to RX mode.
Definition at line 534 of file CC1101.cpp.
void SendPacket | ( | unsigned char * | txBuffer, |
unsigned char | size | ||
) |
This function can be used to transmit a packet with packet length up to 63 bytes.
- Parameters:
-
*txBuffer Pointer to a buffer containing the data that are going to be transmitted size The size of the txBuffer
Definition at line 482 of file CC1101.cpp.
unsigned char TxFifoEmpty | ( | void | ) |
This function check if the TX FIFO is empty.
- Returns:
- Return value is 1 if the TX FIFO buffer is empty or else 0
Definition at line 447 of file CC1101.cpp.
void WriteBurstReg | ( | unsigned char | addr, |
unsigned char * | buffer, | ||
unsigned char | count | ||
) |
This function writes to multiple CCxxx0 register, using SPI burst access.
- Parameters:
-
addr Address of the first CCxxx0 register to be accessed. *buffer Array of bytes to be written into a corresponding range of CCxx00 registers, starting by the address specified in _addr_. count Number of bytes to be written to the subsequent CCxxx0 registers.
Definition at line 314 of file CC1101.cpp.
void WriteReg | ( | unsigned char | addr, |
unsigned char | value | ||
) |
Function for writing to a single CCxxx0 register.
- Parameters:
-
addr Address of the first CCxxx0 register to be accessed. value Value to be written to the specified CCxxx0 register.
Definition at line 288 of file CC1101.cpp.
Generated on Tue Jul 12 2022 19:03:21 by
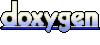