
a
Embed:
(wiki syntax)
Show/hide line numbers
controller.hpp
00001 /** 00002 ****************************************************************************** 00003 * @file Controller.hpp 00004 * @author RBRO/PJ-IU 00005 * @version V1.0.0 00006 * @date day-month-year 00007 * @brief This file contains the class declaration for the controller 00008 * functionality. 00009 ****************************************************************************** 00010 */ 00011 00012 /* Include guard */ 00013 00014 #ifndef CONTROLLER_HPP 00015 #define CONTROLLER_HPP 00016 #include<cmath> 00017 #include<Controllers/pidcontroller.hpp> 00018 00019 #include <Encoders/encoderinterface.hpp > 00020 #include <Controllers/converters.hpp > 00021 00022 #include <mbed.h> 00023 00024 namespace controllers 00025 { 00026 00027 /** CControllerSiso class 00028 * @brief It implements a controller with a single input and a single output. It needs an encoder getter interface to get the measured values, a controller to calculate the control signal. It can be completed with a converter to convert the measaurment unit of the control signal. 00029 * 00030 */ 00031 class CControllerSiso 00032 { 00033 /* PID controller declaration*/ 00034 template <class T> 00035 using ControllerType=siso::IController<T>; 00036 00037 public: 00038 /* Construnctor */ 00039 CControllerSiso(encoders::IEncoderGetter& f_encoder 00040 ,ControllerType<double>& f_pid 00041 ,controllers::IConverter* f_converter=NULL); 00042 /* Set controller reference value */ 00043 void setRef(double f_RefRps); 00044 /* Get controller reference value */ 00045 double getRef(); 00046 /* Get control value */ 00047 double get(); 00048 /* Get error */ 00049 double getError(); 00050 /* Clear PID parameters */ 00051 void clear(); 00052 /* Control action */ 00053 bool control(); 00054 00055 private: 00056 /* PWM onverter */ 00057 double converter(double f_u); 00058 00059 /* Enconder object reference */ 00060 encoders::IEncoderGetter& m_encoder; 00061 /* PID object reference */ 00062 ControllerType<double>& m_pid; 00063 /* Controller reference */ 00064 double m_RefRps; 00065 /* Control value */ 00066 double m_u; 00067 /* Error */ 00068 double m_error; 00069 /* Converter */ 00070 controllers::IConverter* m_converter; 00071 /* Scaled PWM control signal limits */ 00072 const float m_control_sup; 00073 const float m_control_inf; 00074 /* Inferior limit of absolut reference */ 00075 const float m_ref_abs_inf; 00076 /* Inferior and superior limits of absolute speed measurment for standing state */ 00077 const float m_mes_abs_inf; 00078 const float m_mes_abs_sup; 00079 00080 }; 00081 }; // namespace controllers 00082 00083 #endif
Generated on Tue Jul 12 2022 22:40:50 by
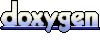