
a
CBezierMotionPlanner Class Reference
CBezierMotionPlanner class. More...
#include <beziermotionplanner.hpp>
Public Member Functions | |
CBezierMotionPlanner () | |
Construct a new CBezierMotionPlanner::CBezierMotionPlanner object. | |
CBezierMotionPlanner (bool isForward, std::complex< float > a, std::complex< float > b, std::complex< float > c, std::complex< float > d, float motion_duration_i, float timestep_i) | |
Construct a new CBezierMotionPlanner::CBezierMotionPlanner object. | |
void | setMotionPlannerParameters (bool isForward, std::complex< float > a, std::complex< float > b, std::complex< float > c, std::complex< float > d, float motion_duration_i, float timestep_i) |
Set motion planner parameters. | |
virtual | ~CBezierMotionPlanner () |
Destroy the CBezierMotionPlanner::CBezierMotionPlanner object. | |
math::BezierCurve< float > | getBezierCurve () |
Get the Bezier curve. | |
std::pair< float, float > | getNextVelocity () |
Get the next control parameters. | |
std::pair< float, float > | getVelocity (float input_value) |
Get the forward velocity and steering angle, base on the given input value. | |
bool | hasValidValue () |
Get the state of the planner. | |
bool | getForward () |
Get the direction of the motion. |
Detailed Description
CBezierMotionPlanner class.
This class implements the functionality of planner to keep the robot on the given Bezier curve.
Definition at line 30 of file beziermotionplanner.hpp.
Constructor & Destructor Documentation
Construct a new CBezierMotionPlanner::CBezierMotionPlanner object.
Definition at line 21 of file beziermotionplanner.cpp.
CBezierMotionPlanner | ( | bool | isForward, |
std::complex< float > | a, | ||
std::complex< float > | b, | ||
std::complex< float > | c, | ||
std::complex< float > | d, | ||
float | motion_duration_i, | ||
float | timestep_i | ||
) |
Construct a new CBezierMotionPlanner::CBezierMotionPlanner object.
- Parameters:
-
isForward Forward movement flag a Point A b Point B c Point C d Point D motion_duration_i The motion duration in second. timestep_i The base period of the planner. (Sample time)
Definition at line 37 of file beziermotionplanner.cpp.
~CBezierMotionPlanner | ( | ) | [virtual] |
Destroy the CBezierMotionPlanner::CBezierMotionPlanner object.
Definition at line 87 of file beziermotionplanner.cpp.
Member Function Documentation
math::BezierCurve< float > getBezierCurve | ( | ) |
Get the Bezier curve.
Definition at line 96 of file beziermotionplanner.cpp.
bool getForward | ( | ) |
Get the direction of the motion.
- Returns:
- true The motion direction is forward.
- false The motion direction is backward.
Definition at line 163 of file beziermotionplanner.cpp.
std::pair< float, float > getNextVelocity | ( | ) |
Get the next control parameters.
It calculates the velocity and angle, and increase input value.
- Returns:
- It returns the next forward velocity and direction angle.
Definition at line 106 of file beziermotionplanner.cpp.
std::pair< float, float > getVelocity | ( | float | input_value ) |
Get the forward velocity and steering angle, base on the given input value.
- Parameters:
-
input_value The input value have to belong to interval [0,1].
- Returns:
- It returns a pair of number, where the fist variable and the second contains the forward velocity and the steering angular, respectively.
Definition at line 118 of file beziermotionplanner.cpp.
bool hasValidValue | ( | ) |
Get the state of the planner.
- Returns:
- true The planner has valid value.
- false The planner finished the last given curve, it cannnot get correct parameters.
Definition at line 152 of file beziermotionplanner.cpp.
void setMotionPlannerParameters | ( | bool | isForward, |
std::complex< float > | a, | ||
std::complex< float > | b, | ||
std::complex< float > | c, | ||
std::complex< float > | d, | ||
float | motion_duration_i, | ||
float | timestep_i | ||
) |
Set motion planner parameters.
- Parameters:
-
isForward Forward movement flag a Point A b Point B c Point C d Point D motion_duration_i The motion duration in second. timestep_i The base period of the planner. (Sample time)
Definition at line 66 of file beziermotionplanner.cpp.
Generated on Tue Jul 12 2022 22:40:51 by
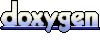