
.
Dependencies: BLE_API TxIR mbed nRF51822
Fork of ir-puck by
main.cpp
00001 #include "mbed.h" 00002 #include "BLEDevice.h" 00003 #include "IR.h" 00004 #include "nRF51822n.h" 00005 00006 BLEDevice ble; 00007 00008 DigitalOut myled(LED1); 00009 DigitalOut yourled(LED2); 00010 nRF51822n nrf; 00011 00012 Serial py(USBTX, USBRX); 00013 00014 const static uint8_t beaconPayload[] = { 00015 0x00, 0x4C, // Company identifier code (0x004C == Apple) 00016 0x02, // ID 00017 0x15, // length of the remaining payload 00018 0xE2, 0x0A, 0x39, 0xF4, 0x73, 0xF5, 0x4B, 0xC4, // UUID 00019 0xA1, 0x2F, 0x17, 0xD1, 0xAD, 0x07, 0xA9, 0x61, 00020 0x13, 0x37, // the major value to differenciate a location 00021 0xFA, 0xCE, // the minor value to differenciate a location 00022 0xC8 // 2's complement of the Tx power (-56dB) 00023 }; 00024 00025 extern GattService ir_service; 00026 extern GattCharacteristic header, one, zero, ptrail, predata, code; 00027 00028 int received_ir_transmission = 0; 00029 void onDataWritten(uint16_t handle) 00030 { 00031 py.printf("Data written! %i\n", handle); 00032 for (int i = 0; i < ir_service.getCharacteristicCount(); i++) { 00033 GattCharacteristic* characteristic = ir_service.getCharacteristic(i); 00034 characteristic->getMaxLength(); 00035 if (characteristic->getHandle() == handle) { 00036 uint16_t max_length = characteristic->getMaxLength(); 00037 ble.readCharacteristicValue(handle, characteristic->getValuePtr(), &max_length); 00038 for (int i=0; i<max_length; i++) { 00039 py.printf("Got value: %d\n", characteristic->getValuePtr()[i]); 00040 } 00041 break; 00042 } 00043 } 00044 00045 if (code.getHandle() == handle) { 00046 received_ir_transmission = 1; 00047 } 00048 } 00049 00050 void disconnectionCallback(void) 00051 { 00052 py.printf("Disconnected!\n"); 00053 py.printf("Restarting the advertising process\n"); 00054 ble.startAdvertising(); 00055 } 00056 00057 void connectionCallback(void) 00058 { 00059 py.printf("Connected!\n"); 00060 } 00061 00062 void onDataSent(uint16_t data) 00063 { 00064 py.printf("onDataSent!\n"); 00065 } 00066 00067 int main() 00068 { 00069 py.printf("Start of main\n"); 00070 00071 ble.init(); 00072 ble.onConnection(connectionCallback); 00073 ble.onDisconnection(disconnectionCallback); 00074 ble.onDataWritten(onDataWritten); 00075 ble.onDataSent(onDataSent); 00076 ble.accumulateAdvertisingPayload(GapAdvertisingData::BREDR_NOT_SUPPORTED); 00077 ble.setAdvertisingType(GapAdvertisingParams::ADV_CONNECTABLE_UNDIRECTED); 00078 ble.setAdvertisingInterval(160); /* 100ms; in multiples of 0.625ms. */ 00079 00080 ble.accumulateAdvertisingPayload(GapAdvertisingData::MANUFACTURER_SPECIFIC_DATA, 00081 beaconPayload, sizeof(beaconPayload)); 00082 00083 ble.startAdvertising(); 00084 00085 ble.addService(ir_service); 00086 00087 myled = 1; 00088 00089 py.printf("Listening..\n"); 00090 00091 while (true) { 00092 ble.waitForEvent(); 00093 if (received_ir_transmission == 1) { 00094 fireIRCode(header.getValuePtr(), one.getValuePtr(), zero.getValuePtr(), ptrail.getValuePtr(), predata.getValuePtr(), code.getValuePtr()); 00095 received_ir_transmission = 0; 00096 } 00097 myled = !myled; 00098 } 00099 }
Generated on Fri Jul 15 2022 18:15:28 by
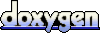