
Sample program for lpc1768 with 8ch ADC and Library TRP105F_Spline.
Dependencies: mbed TRP105F_Spline
main.cpp
00001 #include "mbed.h" 00002 #include "TRP105F_Spline.h" 00003 #include "TRP105FS_SPIWrapper.h" 00004 00005 Serial pc(USBTX, USBRX); // tx, rx ( the usb serial communication ) 00006 extern AnalogIn* g_Sensor_Voltage; 00007 DigitalOut myled1(LED1),myled2(LED2); 00008 Timer t; 00009 TRP105FS TRP(5, AsDEBUG, p16); 00010 00011 00012 SPI_TRP105FS sensor[8] = { 00013 SPI_TRP105FS(0), 00014 SPI_TRP105FS(1), 00015 SPI_TRP105FS(2), 00016 SPI_TRP105FS(3), 00017 SPI_TRP105FS(4), 00018 SPI_TRP105FS(5), 00019 SPI_TRP105FS(6), 00020 SPI_TRP105FS(7) 00021 }; 00022 00023 // A sensor Calibration 00024 // 個別キャリブレーション 00025 void calibrateSensor(unsigned int ch)//channel 00026 { 00027 char c; 00028 00029 printf("\nload save-data or calibration[l/c]>"); 00030 c = pc.getc(); 00031 if(c == 'l') { 00032 printf("%c\n",c); 00033 printf("Now loading...\n"); 00034 sensor[ch].loaddata(); 00035 printf("Done.\n"); 00036 } else { 00037 printf("%c\n",c); 00038 for(int i = 0; i < sensor[ch].getNsample(); i++) { 00039 printf("press ENTER holding the distance at <%d>", i * 255 / 4); 00040 do { 00041 c = pc.getc(); 00042 } while (!( c == 0x0a || c == 0x0d )); 00043 printf("\n"); 00044 sensor[ch].setSample(i * 255 / 4); 00045 } 00046 printf("Done.\n"); 00047 printf("Now Calibrating...\n"); 00048 sensor[ch].calibrate(); 00049 printf("Done.\n"); 00050 printf("Calibration Result being saved...\n"); 00051 sensor[ch].savedata(); 00052 printf("Done.\n"); 00053 } 00054 } 00055 00056 // Multiple Sensors Calibration(Not Tested) 00057 // 一斉キャリブレーション(動作未確認) 00058 void calibrateSensor(unsigned int ch_first, unsigned int ch_end, unsigned int ch_teacher) 00059 { 00060 printf("Calibration at once"); 00061 unsigned int nsample = sensor[ch_teacher].getNsample(); 00062 unsigned short dst; 00063 bool* bnsample = new bool[nsample]; 00064 bool flag = false; 00065 unsigned int n_ui; 00066 00067 for (int i = 0; i < nsample; i++) bnsample[i] = false; 00068 printf("Press keys at once..."); 00069 do { 00070 dst = sensor[ch_teacher].getDistance(); 00071 00072 for (unsigned int i = 0; i < nsample; i++) { 00073 if(dst == i * 255 / (nsample - 1)) { 00074 flag = true; 00075 n_ui = i; 00076 } 00077 } 00078 00079 if(flag || (!bnsample[n_ui])) { 00080 for(unsigned int ch = ch_first; ch < ch_end + 1; ch++) { 00081 if(ch != ch_teacher)sensor[ch].setSample(dst); 00082 } 00083 bnsample[n_ui] = true; 00084 } 00085 for (unsigned int i = 0; i < nsample; i++) { 00086 if(bnsample[i] == false) flag = false; 00087 } 00088 } while(!flag); 00089 printf("Done.\n"); 00090 for (unsigned int ch = 0; ch < nsample; ch++) { 00091 sensor[ch].calibrate(); 00092 sensor[ch].savedata(); 00093 } 00094 00095 delete[] bnsample; 00096 } 00097 00098 //Main 00099 int main() 00100 { 00101 // Setup the spi for 7 bit data, high steady state clock, 00102 // second edge capture, with a 1MHz clock rate 00103 sensor[0].spi.format(16,0); 00104 sensor[0].spi.frequency(1000000); 00105 00106 calibrateSensor(0); //calibration sensor channel 0 00107 //calibrateSensor(1); //calibration sensor channel 1 00108 //calibrateSensor(1,2,0); //Multiple Calibration channel 1-2, sensor channel 0 is teacher. 00109 00110 myled1 = 1; 00111 myled2 = 1; 00112 unsigned short dst; 00113 while(1) { 00114 t.start(); 00115 dst = sensor[0].getDistance(); 00116 //dst = sensor[1].getDistance(); 00117 00118 t.stop(); 00119 printf("dst:%d(%f s)\n",dst, t.read()); 00120 t.reset(); 00121 //printf("vol:%d\n",SPI_TPR105FS::ADread(0)); 00122 00123 wait(0.1); 00124 pc.putc('\n'); 00125 // if 'p' is pressed quit the loop 00126 if(pc.readable())if(pc.getc() == 'q') break; 00127 } 00128 myled1 = myled2 = 0; 00129 00130 }
Generated on Fri Aug 26 2022 17:33:51 by
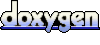