This makes Amplitude Modulated Pulse Train, which can be regarded as the discretized wave of the signal. Pulse Train can be defined by frequency and duty cycle, which can be temporarily changed, referring to PWM.
Dependents: Interference_Simple
PulseTrain.h
00001 /** Defining Amplide Modulated Pulse Train Model 00002 * 00003 * \file PulseTrain.h 00004 * \author Akifumi Takahashi 00005 * \date 2019/12/02 - 00006 * \version 1.0.2019.Dec 00007 */ 00008 00009 #ifndef PULSE_TRAIN_H 00010 #define PULSE_TRAIN_H 00011 00012 #include "mbed.h" 00013 /** \Class Pulse Train Model 00014 * 00015 * Pulse Train Model which clock is defined in scale of [us]; 00016 * You can define the carrier pulse train's freq and duty cycle like PWM. 00017 * Kinds of Frequency is dealed as unsigned 32bit int 00018 * For the other int vars, allocated is 32bit. 00019 */ 00020 class PulseTrain 00021 { 00022 public: 00023 /** Constractor 00024 */ 00025 PulseTrain( 00026 /// Initial carrier pulse frequency 00027 uint32_t const arg_freq_init = 4000, 00028 /// Initial carrier pulse duty cycle 00029 float const arg_duty_init = 0.5, 00030 /// Initialize FREQ_MAX 00031 uint32_t const arg_freq_max = 8000 00032 ); 00033 /** Increment the clock to let go ahead the wave state 00034 * 00035 * If callback as rising/falling is also called, 00036 * this "asClock" is called earlier 00037 */ 00038 void incrementClock(); 00039 00040 /** Executes a callback fanction called as clock is incremented 00041 * 00042 * If callback as rising/falling is also called, 00043 * this "asClock" is called at the last. 00044 */ 00045 void attachCallback_asClock( 00046 /** Called back as clock incremented 00047 * 00048 * \arg <pulsestate> indicate whther pulse 00049 * (not clock pulse but one of pulse train) has risen/fallen 00050 */ 00051 Callback<void(bool)> arg_callback 00052 ); 00053 00054 void attachCallback_asPulseEdge ( 00055 /** Called back as a pulse rising/falling 00056 * 00057 * \arg <pulsestate> indicate whther pulse has risen/fallen 00058 */ 00059 Callback<void(bool)> arg_callback 00060 ); 00061 00062 void setFrequency(uint32_t const arg_freq); 00063 00064 void setDutycycle(float const arg_duty); 00065 00066 bool 00067 getState(); //inline 00068 uint32_t 00069 getFrequency(); //inline 00070 float 00071 getDutycycle(); //inline 00072 uint32_t 00073 getPeriod_us(); //inline 00074 uint32_t 00075 getClockperiod_us(); //inline 00076 00077 00078 uint32_t const FREQ_MAX; 00079 00080 template <typename T> 00081 static T velidateRange(T const arg_val, T const arg_min, T const arg_max); 00082 00083 private: 00084 void init(); 00085 00086 /// Frequency of the Carrier Pulse (Hz) 00087 uint32_t m_freq; 00088 00089 /// Duty cycle 00090 float m_duty; 00091 00092 /// Period 00093 uint32_t m_period_us; 00094 00095 /// GCD of the pulse period and pulse width 00096 uint32_t m_clock_period_us; 00097 00098 /** Period per clock period 00099 * 00100 * The unit is what times the clock tickes 00101 */ 00102 uint32_t m_period_pcp; 00103 00104 /// Timing [us] a pulse rising within a period [us] 00105 static uint32_t const m_raising = 0; 00106 00107 /** Timing [us] a pulse falling within a period [us] 00108 * 00109 * Calculated in init() 00110 */ 00111 uint32_t m_falling; 00112 00113 /// Flag if a palse is raised (High) or not (Low) 00114 bool m_pulsestate; 00115 00116 /** Called back as clock incremented 00117 * 00118 * \arg <pulsestate> indicate whther pulse 00119 * (not clock pulse but one of pulse train) has risen/fallen 00120 */ 00121 Callback<void(bool)> m_callback_asClock; 00122 00123 /** Called back as a pulse rising/falling 00124 * 00125 * \arg <pulsestate> indicate whther pulse has risen/fallen 00126 */ 00127 Callback<void(bool)> m_callback_asPulseEdge; 00128 00129 static void doNothing(bool arg_b) {;} 00130 00131 }; 00132 #endif
Generated on Tue Jul 12 2022 22:17:22 by
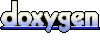