This makes Amplitude Modulated Pulse Train, which can be regarded as the discretized wave of the signal. Pulse Train can be defined by frequency and duty cycle, which can be temporarily changed, referring to PWM.
Dependents: Interference_Simple
AMSignal.h
00001 /** Defining Amplide Modulated Pulse Train Model 00002 * 00003 * \file AMSignal.h 00004 * \author Akifumi Takahashi 00005 * \date 2019/12/02 - 00006 * \version 1.0.2019.Dec 00007 */ 00008 00009 #ifndef AM_SIGNAL_H 00010 #define AM_SIGNAL_H 00011 00012 00013 #include "mbed.h" 00014 00015 /** \Class Amplide Modulated Signal Model for AM Pulse Train Model 00016 * 00017 * All parameter data is dealed as unsigned 16 bit int. 00018 * \version alluint16 00019 */ 00020 class AMSignal 00021 { 00022 public: 00023 /// Constructor 00024 AMSignal( 00025 uint16_t const arg_freq = 50, 00026 uint16_t const arg_freq_max = 100, 00027 uint16_t const arg_ampl_max_u16 = 4095 00028 ): 00029 FREQ_MAX(arg_freq_max), 00030 AMPL_MAX_u16(arg_ampl_max_u16) 00031 { 00032 setFrequency(arg_freq); 00033 attachAMSignalExpression(constantDC_defaltSignal); 00034 } 00035 00036 /** Amplitude Modified Signal / Pulse Hight Writing Handler 00037 * 00038 * For fast processing, no float culc nor div culc is recommended 00039 */ 00040 void attachAMSignalExpression( 00041 /** Callback 00042 * 00043 * \arg AMSignal* contains parameters of this. 00044 * \retval is dealed as a factor the argument of Callback as Pulse rising. 00045 */ 00046 Callback<uint16_t(AMSignal*)> arg_signal 00047 ) 00048 { 00049 m_AMSignalExpression = arg_signal; 00050 } 00051 00052 uint16_t getAMSinalValue() 00053 { 00054 return m_AMSignalExpression(this); 00055 } 00056 00057 00058 private: 00059 uint16_t m_ampl_u16; 00060 uint16_t m_freq; 00061 uint16_t m_period_us; 00062 template <typename T> 00063 static T velidateRange(T const arg_val, T const arg_min, T const arg_max); 00064 00065 Callback<uint16_t(AMSignal*)> m_AMSignalExpression; 00066 00067 /// Default amplitude modulation signal 00068 static uint16_t constantDC_defaltSignal (AMSignal* arg) 00069 { 00070 return 1; 00071 } 00072 00073 00074 public: 00075 // constants 00076 uint16_t const FREQ_MAX; 00077 uint16_t const AMPL_MAX_u16; 00078 00079 /** Frequency could be slightly modulated because it depends on the carrier freq 00080 */ 00081 void setFrequency(uint16_t const arg_freq); 00082 00083 /** Set Amplitude Paramiter 00084 * 00085 * - Crumping a value within [0f,1f], converted to [0, 0xFFFF] 00086 * - Note that this is a parameter which affects a pulse hight, 00087 * but isn't the pulse hight itself 00088 * - The Pulse hight is defined in callback attached with attachAMSignalExpression() 00089 */ 00090 void setAmplitude(float const arg_ampl); 00091 00092 void setAmplitude(uint16_t const arg_ampl); 00093 00094 /// Get a parameter which defines the size of pulse hight axis with in [0,1] 00095 float getAmplitude_uf(); //inline 00096 00097 /// Get a parameter which defines the size of pulse hight axis with in [0, 4096] 00098 uint16_t getAmplitude_u16(); //inline 00099 00100 uint16_t getFrequency(); //inline 00101 00102 uint16_t getPeriod_us(); //inline 00103 00104 }; 00105 #endif
Generated on Tue Jul 12 2022 22:17:22 by
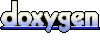