This makes Amplitude Modulated Pulse Train, which can be regarded as the discretized wave of the signal. Pulse Train can be defined by frequency and duty cycle, which can be temporarily changed, referring to PWM.
Dependents: Interference_Simple
AMSignal.cpp
00001 #include "AMSignal.h" 00002 00003 void AMSignal::setFrequency(uint16_t const arg_freq) 00004 { 00005 m_freq = velidateRange<uint16_t>(arg_freq, 1, FREQ_MAX); 00006 m_period_us = 1000000 / m_freq; 00007 if(1000000 % m_freq >= (m_freq/2)) m_period_us += 1; 00008 } 00009 00010 void AMSignal::setAmplitude(float const arg_ampl) 00011 { 00012 m_ampl_u16 = AMPL_MAX_u16 * velidateRange<float>(arg_ampl, 0.0, 1.0); 00013 } 00014 00015 void AMSignal::setAmplitude(uint16_t const arg_ampl) 00016 { 00017 m_ampl_u16 = arg_ampl; 00018 } 00019 00020 /// Get a parameter which defines the size of pulse hight axis with in [0,1] 00021 float AMSignal::getAmplitude_uf() 00022 { 00023 return (float) m_ampl_u16 / (float) AMPL_MAX_u16; 00024 } 00025 00026 uint16_t AMSignal::getAmplitude_u16() 00027 { 00028 return m_ampl_u16; 00029 } 00030 00031 uint16_t AMSignal::getFrequency() 00032 { 00033 return m_freq; 00034 } 00035 00036 uint16_t AMSignal::getPeriod_us() 00037 { 00038 return m_period_us; 00039 } 00040 00041 00042 template <typename T> 00043 T AMSignal::velidateRange(T const arg_val, T const arg_min, T const arg_max) 00044 { 00045 if(arg_val < arg_min) return arg_min; 00046 else if (arg_val <= arg_max) return arg_val; 00047 else return arg_max; 00048 }
Generated on Tue Jul 12 2022 22:17:22 by
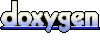