This makes Amplitude Modulated Pulse Train, which can be regarded as the discretized wave of the signal. Pulse Train can be defined by frequency and duty cycle, which can be temporarily changed, referring to PWM.
Dependents: Interference_Simple
AMPulseTrain.h
00001 /** Defining Amplide Modulated Pulse Train Model 00002 * 00003 * \file AMPulseTrain.h 00004 * \author Akifumi Takahashi 00005 * \date 2019/12/02 - 00006 * \version 1.0.2019.Dec 00007 */ 00008 00009 #ifndef AM_PULSE_TRAIN_H 00010 #define AM_PULSE_TRAIN_H 00011 00012 00013 #include "mbed.h" 00014 #include "AMSignal.h" 00015 #include "PulseTrain.h" 00016 00017 /** \Class Amplide Modulated Pulse Train Model 00018 * 00019 * Pulse Train Model which clock is defined in scale of us; 00020 * the model pulses' height can be modulated as a product with m_ampl and any function 00021 * returning a int as the coefficeincy. 00022 * You can define the carrier pulse train's freq and duty cycle like PWM. 00023 * 00024 */ 00025 class AMPulseTrain 00026 { 00027 public: 00028 /** Constractor 00029 */ 00030 AMPulseTrain( 00031 /// Carrier Pulse Train 00032 PulseTrain * arg_carrier = new PulseTrain(), 00033 /// Initial AM Signal expression 00034 AMSignal * arg_signal = new AMSignal() 00035 ); 00036 00037 /// Carrier Pulse Train 00038 PulseTrain * Carrier; 00039 00040 /// AM Signal 00041 AMSignal * Signal; 00042 00043 /// register callback called every clock (not carrier pulse edges) 00044 void attachCallback_asClock( 00045 Callback<void(bool, AMPulseTrain*)> arg_callback 00046 ); 00047 00048 /// register callback called every carrier pulse edges 00049 void attachCallback_asPulseEdge( 00050 Callback<void(bool, AMPulseTrain*)> arg_callback 00051 ); 00052 00053 /// register callback as AMSignalExpression 00054 void attachAMSignalExpression( 00055 Callback<uint16_t(AMPulseTrain*)> arg_callback 00056 ); 00057 00058 void setFrequency_Carrier( uint32_t const arg_freq ) 00059 { 00060 Carrier->setFrequency(arg_freq); 00061 RecalcPulsebaseParameter(); 00062 } 00063 00064 void setFrequency_Signal( uint32_t const arg_freq ) 00065 { 00066 Signal->setFrequency(arg_freq); 00067 RecalcPulsebaseParameter(); 00068 } 00069 00070 uint32_t getFrequency_Carrier() 00071 { 00072 return Carrier->getFrequency(); 00073 } 00074 00075 uint16_t getClockperiod_us(); 00076 00077 void incrementClock(); 00078 00079 uint16_t getPeriod_pPulse_Signal() 00080 { 00081 return m_AMSIGNAL_PERIOD_PER_PULSE; 00082 } 00083 00084 uint16_t getPWidth_pPulse_Signal() 00085 { 00086 return m_AMSIGNAL_PWIDTH_PER_PULSE; 00087 } 00088 00089 private: 00090 00091 Callback<void(bool, AMPulseTrain*)> m_callback_asClock; 00092 void CallbackWrapper_asClock(bool arg_pulsestate); 00093 00094 Callback<void(bool, AMPulseTrain*)> m_callback_asPulseEdge; 00095 void CallbackWrapper_asPulseEdge(bool arg_pulsestate); 00096 00097 Callback<uint16_t(AMPulseTrain*)> m_AMSignalExpression; 00098 uint16_t CallbackWrapper_AMSignalExpression(AMSignal* arg_signal); 00099 00100 uint16_t m_AMSIGNAL_PERIOD_PER_PULSE; 00101 uint16_t m_AMSIGNAL_PWIDTH_PER_PULSE; 00102 void RecalcPulsebaseParameter() 00103 { 00104 m_AMSIGNAL_PERIOD_PER_PULSE = Signal->getPeriod_us() / Carrier->getPeriod_us(); 00105 m_AMSIGNAL_PWIDTH_PER_PULSE = 500 / Carrier->getPeriod_us(); 00106 } 00107 }; 00108 00109 #endif
Generated on Tue Jul 12 2022 22:17:22 by
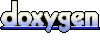