BLDC control for jumping robot.
Dependencies: CRC MODSERIAL mbed-dev mbed-rtos
Fork of mbed_BLDC_driver_KL25Z by
protocol.h
00001 #ifndef PROTOCOL_H 00002 #define PROTOCOL_H 00003 00004 #include <stdint.h> 00005 /** 00006 * Packet type characters. 00007 */ 00008 #define PKT_TYPE_COMMAND 'C' 00009 #define PKT_TYPE_SENSOR 'S' 00010 00011 /** 00012 * Defines the total maximum size of a packet, including header 00013 */ 00014 #define MAX_PACKET_LENGTH 256 00015 00016 /** 00017 * Packet structure definitions 00018 */ 00019 typedef struct header_t { 00020 uint8_t start; 00021 uint8_t length; 00022 char type; 00023 uint8_t flags; 00024 uint32_t sequence; 00025 } header_t; 00026 00027 typedef struct packet_t { 00028 header_t header; 00029 uint8_t data_crc[MAX_PACKET_LENGTH-sizeof(header_t)]; 00030 } packet_t; 00031 00032 typedef union packet_union_t { 00033 packet_t packet; 00034 char raw[MAX_PACKET_LENGTH]; 00035 } packet_union_t; 00036 00037 typedef struct command_data_t { 00038 int32_t position_setpoint; 00039 uint16_t current_setpoint; 00040 } command_data_t; 00041 00042 typedef struct sensor_data_t { 00043 uint32_t time; 00044 int32_t position; 00045 int32_t velocity; 00046 uint16_t current; 00047 uint16_t voltage; 00048 uint16_t temperature; 00049 } sensor_data_t; 00050 00051 #endif
Generated on Sun Jul 17 2022 03:06:54 by
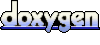