BLDC control for jumping robot.
Dependencies: CRC MODSERIAL mbed-dev mbed-rtos
Fork of mbed_BLDC_driver_KL25Z by
packet_parser.cpp
00001 #include "packet_parser.h" 00002 00003 00004 Thread* global_thread_ = NULL; 00005 00006 void dma_complete_signal(MODSERIAL_IRQ_INFO *q) { 00007 if (global_thread_ != NULL) { 00008 global_thread_->signal_set(DMA_COMPLETE_FLAG); 00009 } 00010 } 00011 00012 PacketParser::PacketParser( 00013 uint32_t baudrate, PinName tx_pin, PinName rx_pin, PinName tx_led, PinName rx_led) : 00014 pc_(tx_pin, rx_pin), /*dma_(),*/ 00015 tx_led_(tx_led), send_thread_(&PacketParser::thread_starter, this), 00016 rx_led_(rx_led) { 00017 00018 pc_.baud(baudrate); 00019 //pc_.MODDMA(&dma_); 00020 //pc_.attach_dmaSendComplete(this, &PacketParser::send_complete); 00021 //pc_.attach_dmaSendComplete(&dma_complete_signal); 00022 global_thread_ = &send_thread_; 00023 00024 out_pkt_ = NULL; 00025 tx_sequence_ = 0; 00026 00027 pc_.attach(this, &PacketParser::receive_callback, MODSERIAL::RxIrq); 00028 in_pkt_ = (packet_union_t*)in_box_.alloc(); 00029 in_pkt_idx_ = 0; 00030 in_pkt_len_ = MAX_PACKET_LENGTH; 00031 in_pkt_crc_ = 0; 00032 00033 send_thread_.signal_set(START_THREAD_FLAG); 00034 } 00035 00036 packet_union_t* PacketParser::get_received_packet(void) { 00037 osEvent evt = in_box_.get(0); 00038 if (evt.status == osEventMail) { 00039 return (packet_union_t*)evt.value.p; 00040 } else { 00041 return NULL; 00042 } 00043 } 00044 00045 void PacketParser::free_received_packet(packet_union_t* packet) { 00046 in_box_.free(packet); 00047 } 00048 00049 packet_union_t* PacketParser::get_send_packet(void) { 00050 return (packet_union_t*)out_box_.alloc(); 00051 } 00052 00053 void PacketParser::send_packet(packet_union_t* packet) { 00054 out_box_.put(packet); 00055 } 00056 00057 void PacketParser::thread_starter(void const *p) { 00058 PacketParser* instance = (PacketParser*)p; 00059 instance->send_worker(); 00060 } 00061 00062 void PacketParser::send_worker(void) { 00063 send_thread_.signal_wait(START_THREAD_FLAG); 00064 while(true) { 00065 osEvent evt = out_box_.get(); 00066 if (evt.status == osEventMail) { 00067 tx_led_ = 1; 00068 out_pkt_ = (packet_union_t*)evt.value.p; 00069 out_pkt_->packet.header.start = 0; 00070 out_pkt_->packet.header.sequence = tx_sequence_++; 00071 uint8_t crc_value = calculate_crc8(out_pkt_->raw, out_pkt_->packet.header.length-1); 00072 out_pkt_->raw[out_pkt_->packet.header.length-1] = crc_value; 00073 //pc_.dmaSend(out_pkt_->raw, out_pkt_->packet.header.length); 00074 for (int i = 0; i < out_pkt_->packet.header.length; i++) { 00075 pc_.putc(out_pkt_->raw[i]); 00076 } 00077 tx_led_ = 0; 00078 send_thread_.signal_wait(DMA_COMPLETE_FLAG); 00079 tx_led_ = 1; 00080 send_thread_.signal_clr(DMA_COMPLETE_FLAG); 00081 out_box_.free(out_pkt_); 00082 out_pkt_ = NULL; 00083 tx_led_ = 0; 00084 } 00085 Thread::yield(); 00086 } 00087 } 00088 00089 void PacketParser::send_complete(MODSERIAL_IRQ_INFO *q) { 00090 tx_led_ = 1; 00091 if (out_pkt_ != NULL) { 00092 out_box_.free(out_pkt_); 00093 out_pkt_ = NULL; 00094 } 00095 tx_led_ = 0; 00096 } 00097 00098 void PacketParser::receive_callback(MODSERIAL_IRQ_INFO *q) { 00099 rx_led_ = 1; 00100 MODSERIAL* serial = q->serial; 00101 00102 if (in_pkt_ != NULL) { 00103 while(serial->readable()) { 00104 char c = serial->getc(); 00105 00106 // If we just received the second character, set packet length 00107 if (in_pkt_idx_ == 1) { 00108 in_pkt_len_ = c; 00109 } 00110 00111 // If there has been a parse error, reset packet buffer 00112 if ((in_pkt_idx_ == 0 && c != 0) || in_pkt_len_ < sizeof(header_t)+1 ) { 00113 in_pkt_idx_ = 0; 00114 in_pkt_len_ = MAX_PACKET_LENGTH; 00115 in_pkt_crc_ = 0; 00116 00117 // Store byte in packet buffer and update CRC 00118 } else { 00119 in_pkt_->raw[in_pkt_idx_++] = c; 00120 in_pkt_crc_ = update_crc8(in_pkt_crc_, c); 00121 } 00122 00123 // If we just received the last character, put valid packets in mailbox 00124 // and reset packet buffer 00125 if (in_pkt_idx_ == in_pkt_len_) { 00126 if (in_pkt_crc_ == 0) { 00127 in_box_.put(in_pkt_); 00128 in_pkt_ = (packet_union_t*)in_box_.alloc(); 00129 } 00130 in_pkt_idx_ = 0; 00131 in_pkt_len_ = MAX_PACKET_LENGTH; 00132 in_pkt_crc_ = 0; 00133 } 00134 } 00135 } else { 00136 in_pkt_ = (packet_union_t*)in_box_.alloc(); 00137 } 00138 00139 rx_led_ = 0; 00140 }
Generated on Sun Jul 17 2022 03:06:54 by
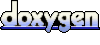