
Program an AVR microcontroller using mbed.
Embed:
(wiki syntax)
Show/hide line numbers
AVR910.h
00001 /** 00002 * @author Aaron Berk 00003 * 00004 * @section LICENSE 00005 * 00006 * Copyright (c) 2010 Aaron Berk 00007 * 00008 * Permission is hereby granted, free of charge, to any person obtaining a copy 00009 * of this software and associated documentation files (the "Software"), to deal 00010 * in the Software without restriction, including without limitation the rights 00011 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00012 * copies of the Software, and to permit persons to whom the Software is 00013 * furnished to do so, subject to the following conditions: 00014 * 00015 * The above copyright notice and this permission notice shall be included in 00016 * all copies or substantial portions of the Software. 00017 * 00018 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00019 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00020 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00021 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00022 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00023 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00024 * THE SOFTWARE. 00025 * 00026 * @section DESCRIPTION 00027 * 00028 * Program AVR chips with the AVR910 ISP (in-system programming) protocol, 00029 * using an mbed. 00030 * 00031 * AVR910 Application Note: 00032 * 00033 * http://www.atmel.com/dyn/resources/prod_documents/doc0943.pdf 00034 */ 00035 00036 #ifndef MBED_AVR910_H 00037 #define MBED_AVR910_H 00038 00039 /** 00040 * Includes 00041 */ 00042 #include "mbed.h" 00043 00044 /** 00045 * Defines 00046 */ 00047 00048 #define PATH_TO_BINARY "/local/AVRCODE.bin" 00049 00050 //Commands 00051 #define ATMEL_VENDOR_CODE 0x1E 00052 #define DEVICE_LOCKED 0x00 00053 #define WRITE_HIGH_BYTE 0x48 00054 #define WRITE_LOW_BYTE 0x40 00055 #define READ_HIGH_BYTE 0x28 00056 #define READ_LOW_BYTE 0x20 00057 #define WRITE_HIGH_FLASH_BYTE 0x68 00058 #define WRITE_LOW_FLASH_BYTE 0x60 00059 00060 #define PAGE_SIZE ATMEGA328P_PAGESIZE 00061 #define NUM_PAGES ATMEGA328P_NUM_PAGES 00062 00063 //ATMega328P 00064 #define ATMEGA328P_PAGESIZE 64 //Size in words. 00065 #define ATMEGA328P_NUM_PAGES 256 00066 00067 /** 00068 * AVR910 ISP 00069 */ 00070 class AVR910 { 00071 00072 public: 00073 00074 /** 00075 * Constructor. 00076 * 00077 * @param mosi mbed pin for MOSI SPI line. 00078 * @param miso mbed pin for MISO SPI line. 00079 * @param sclk mbed pin for SCLK SPI line. 00080 * @param nReset mbed pin for not reset line on the ISP interface. 00081 * 00082 * Sends an enable programming command, allowing device registers to be 00083 * read and commands sent. 00084 */ 00085 AVR910(PinName mosi, PinName miso, PinName sclk, PinName nReset); 00086 00087 /** 00088 * Program the AVR microcontroller connected to the mbed. 00089 * 00090 * Sends a chip erase command followed by writing the binary to the AVR 00091 * page buffer and writing the page buffer to flash memory whenever it is 00092 * full. 00093 * 00094 * @param binary File pointer to the binary file to be loaded onto the 00095 * AVR microcontroller. 00096 * @param pageSize The size of one page on the device in words. If the 00097 * device does not use paged memory, set this as the size 00098 * of memory of the device in words. 00099 * @param numPages The number of pages on the device. If the device does 00100 * not use paged memory, set this to 1 (default). 00101 * 00102 * @return 0 => AVR microcontroller programmed successfully. 00103 * -1 => Problem during programming. 00104 */ 00105 int program(FILE* binary, int pageSize, int numPages = 1); 00106 00107 /** 00108 * Set the frequency of the SPI communication. 00109 * 00110 * (Wrapper for SPI::frequency) 00111 * 00112 * @param frequency Frequency of the SPI communication in hertz. 00113 */ 00114 void setFrequency(int frequency); 00115 00116 /** 00117 * Read the vendor code of the device. 00118 * 00119 * @return The vendor code - should be 0x1E for Atmel. 00120 * 0x00 -> Device is locked. 00121 */ 00122 int readVendorCode(void); 00123 00124 /** 00125 * Read the part family and flash size of the device. 00126 * 00127 * @return Code indicating the family of AVR microcontrollers the device comes 00128 * from and how much flash memory it contains. 00129 * 0xFF -> Device code erased or target missing. 00130 * 0x01 -> Device is locked. 00131 */ 00132 int readPartFamilyAndFlashSize(void); 00133 00134 /** 00135 * Read the part number. 00136 * 00137 * @return Code identifying the part number. 00138 * 0xFF -> Device code erased or target missing. 00139 * 0x02 -> Device is locked. 00140 */ 00141 int readPartNumber(void); 00142 00143 private: 00144 00145 /** 00146 * Issue an enable programming command to the AVR microcontroller. 00147 * 00148 * @param 0 to indicate programming was enabled successfully. 00149 * -1 to indicate programming was not enabled. 00150 */ 00151 int enableProgramming(void); 00152 00153 /** 00154 * Poll the device until it has finished its current operation. 00155 */ 00156 void poll(void); 00157 00158 /** 00159 * Issue a chip erase command to the AVR microcontroller. 00160 */ 00161 void chipErase(void); 00162 00163 /** 00164 * Load a byte into the memory page buffer. 00165 * 00166 * @param highLow Indicate whether the byte being loaded is a high or low 00167 * byte. 00168 * @param data The data byte to load. 00169 */ 00170 void loadMemoryPage(int highLow, char address, char data); 00171 00172 /** 00173 * Write a byte into the flash memory. 00174 * 00175 * @param highLow Indicate whether the byte being loaded is a high or low 00176 * byte. 00177 * @param address The address to load the byte at. 00178 * @param data The data byte to load. 00179 */ 00180 void writeFlashMemoryByte(int highLow, int address, char data); 00181 00182 /** 00183 * Write the memory page buffer to flash memory. 00184 * 00185 * @param pageNumber The page number to write to in flash memory. 00186 */ 00187 void writeFlashMemoryPage(char pageNumber); 00188 00189 /** 00190 * Read a byte from program memory. 00191 * 00192 * @param highLow Indicate whether the byte being read is a low or high byte. 00193 * @param pageNumber The page number to read from. 00194 * @param pageOffset Address of byte in the page. 00195 * 00196 * @return The byte at the specified memory location. 00197 */ 00198 char readProgramMemory(int highLow, char pageNumber, char pageOffset); 00199 00200 /** 00201 * Check the binary has been written correctly. 00202 * 00203 * @param numPages The number of pages written to the AVR microcontroller. 00204 * @param binary File pointer to the binary used. 00205 * 00206 * @return 0 -> No inconsistencies between binary file and AVR flash memory. 00207 * -1 -> Binary file was not written correctly. 00208 */ 00209 int checkMemory(int numPages, FILE* binary); 00210 00211 SPI spi_; 00212 DigitalOut nReset_; 00213 00214 }; 00215 00216 #endif /* AVR910_H */
Generated on Tue Jul 12 2022 14:07:14 by
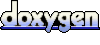