
ネットワークアップデート機能とか、Pachubeへの情報登録とかの処理を追加しています
Dependencies: Terminal EthernetNetIf Pachube TextLCD mbed ConfigFile FirmwareUpdater
main.cpp
00001 #include "mbed.h" 00002 #include "geigercounter_sbm_20.h" 00003 #include "trans.h" 00004 #include "TextLCD.h" 00005 00006 00007 #include "mbed.h" 00008 #include "PachubeV2CSV.h" 00009 #include "EthernetNetIf.h" 00010 #include "HTTPClient.h" 00011 #include "appconf.h" 00012 #include "FirmwareUpdater.h" 00013 00014 00015 00016 extern "C" void mbed_reset(); 00017 00018 /* 00019 * Definitions for a configuration file. 00020 */ 00021 #define CONFIG_FILENAME "/local/PACHUBE.CFG" 00022 const int PACHUBE_CODE_OK = 200; 00023 00024 LocalFileSystem localfs("local"); 00025 00026 EthernetNetIf netif; 00027 00028 FirmwareUpdater fwup("http://mbed.org/media/uploads/abe00makoto", "geiger", true); 00029 00030 TextLCD output( p24, p26, p27, p28, p29, p30 ); // rs, e, d0-d3 00031 Geigercounter_SBM_20 geiger(p18,p22);//Geiger pin,Spekaer pin 00032 Trans trans(p21,0.16/1000.0,0.68/*0.76*/); //Trans pin,period,duty 00033 00034 static appconf_t appconf; 00035 00036 00037 /** 00038 * Convert double to char. 00039 * 00040 * @param val Value. 00041 * @param buf A pointer to a buffer. 00042 * @param bufsiz The buffer size. 00043 */ 00044 void convertDoubleToChar(double val, char *buf, size_t bufsiz) { 00045 snprintf(buf, bufsiz, "%f", val); 00046 } 00047 00048 /** 00049 * Post to the feed on Pachube. 00050 * 00051 * @param web Pointer to a Pachube object. 00052 * @param feed_id Feed ID. 00053 * @param stream_no Stream number. 00054 * @param value value. 00055 * 00056 * @return Pachube code. 00057 */ 00058 int web_post(PachubeV2CSV *web, int feed_id, int stream_no, double value) { 00059 char value_text[16]; 00060 convertDoubleToChar(value, value_text, sizeof(value_text)); 00061 char stream_no_text[8]; 00062 stream_no_text[0] = "0123456789"[stream_no]; 00063 stream_no_text[1] = '\0'; 00064 return web->updateDataStream(feed_id, stream_no_text, std::string(value_text)); 00065 } 00066 00067 00068 00069 //firm ware updater 00070 void check_newfirm() { 00071 if (fwup.exist() == 0) { 00072 output.printf("Found a new firmware.\n"); 00073 if (fwup.execute() == 0) { 00074 output.printf("Update succeed.\n"); 00075 wait(10); 00076 output.printf("Resetting this system.\n"); 00077 wait(10); 00078 fwup.reset(); 00079 } else { 00080 output.printf("Update failed!\n"); 00081 00082 } 00083 }else{ 00084 output.printf("not found update.\n"); 00085 } 00086 wait(5); 00087 } 00088 00089 void print_geigerdata() 00090 { 00091 output.cls(); 00092 output.printf("%.3fuSv/h\n",geiger.getusv()); 00093 output.printf("%.3fCPM",geiger.getcpm()); 00094 } 00095 00096 00097 00098 00099 int main() { 00100 00101 bool etherconnect; 00102 output.cls(); 00103 output.printf("Hello."); 00104 wait(1); 00105 set_time(0); //mbed first run dont use time(); 00106 00107 00108 /* 00109 * Initialize ethernet interface. 00110 */ 00111 output.cls(); 00112 output.locate(0, 0); 00113 output.printf("Initializing..."); 00114 output.locate(0, 1); 00115 output.printf("Ethernet: "); 00116 00117 EthernetErr ethErr = netif.setup(); 00118 if (ethErr) { 00119 output.printf("[NG]"); 00120 //error("Ethernet setup failed. Done with code %d.\n", ethErr); 00121 etherconnect=false; 00122 } 00123 else{ 00124 etherconnect=true; 00125 output.printf("[OK]"); 00126 } 00127 00128 wait(2); 00129 if(etherconnect){ 00130 //firmware update 00131 output.cls(); 00132 check_newfirm(); 00133 } 00134 00135 /* 00136 * Read configuration variables from a file. 00137 */ 00138 output.cls(); 00139 output.locate(0, 0); 00140 output.printf("cfg file Reading..."); 00141 output.locate(0, 1); 00142 output.printf("Setup: "); 00143 appconf_init(&appconf); 00144 if (appconf_read(CONFIG_FILENAME, &appconf) != 0) { 00145 output.printf("[NG]"); 00146 error("Failure to read a configuration file.\n"); 00147 } 00148 else{ 00149 output.printf("[OK]"); 00150 wait(3); 00151 } 00152 00153 /* 00154 * Initialize objects. 00155 */ 00156 PachubeV2CSV web(appconf.apikey); 00157 const int feed_id = atoi(appconf.feedid); 00158 00159 00160 00161 //20 minitues loop 00162 for(int min=0;min<(20/5);min++){ 00163 trans.on(); 00164 wait(0.5); 00165 geiger.start(); 00166 //5 minitues geiger run 00167 for(int i=0;i<5*60;i++){ 00168 print_geigerdata(); 00169 wait(1); 00170 } 00171 geiger.stop(); 00172 trans.off(); 00173 output.cls(); 00174 print_geigerdata(); 00175 wait(1); 00176 /* 00177 * Check the pachube feautures. 00178 */ 00179 if(etherconnect){ 00180 00181 if (web_post(&web, feed_id, 0, geiger.getusv()) != PACHUBE_CODE_OK) { 00182 output.cls(); 00183 output.printf("Checking Pachube \nstatus:x"); 00184 wait(10); 00185 00186 } 00187 00188 if (web_post(&web, feed_id, 1, geiger.getcpm()) != PACHUBE_CODE_OK){ 00189 output.cls(); 00190 output.printf("Checking Pachube \nstatus:x"); 00191 wait(10); 00192 } 00193 } 00194 } 00195 00196 if(!etherconnect){ 00197 output.cls(); 00198 output.printf("please!!\n net connect\n"); 00199 wait(10); 00200 output.cls(); 00201 output.printf("mbed reset!!\n"); 00202 wait(10); 00203 mbed_reset(); 00204 } 00205 00206 00207 // 00208 // night running mode. 00209 // 00210 output.cls(); 00211 output.printf("sound off mode.."); 00212 geiger.soundoff(); 00213 00214 int errcnt=0; 00215 while(1){ 00216 00217 for(int min=0;min<(20/5);min++){ 00218 trans.on(); 00219 wait(1); 00220 geiger.start(); 00221 for(int i=0;i<5*60;i++){ 00222 print_geigerdata(); 00223 wait(1); 00224 } 00225 00226 geiger.stop(); 00227 trans.off(); 00228 00229 /* 00230 * Post. 00231 */ 00232 if(etherconnect){ 00233 if (web_post(&web, feed_id, 0, geiger.getusv()) != PACHUBE_CODE_OK) { 00234 output.cls(); 00235 output.printf("Checking Pachube \nstatus:x"); 00236 errcnt++; 00237 wait(10); 00238 } 00239 00240 if (web_post(&web, feed_id, 1, geiger.getcpm()) != PACHUBE_CODE_OK){ 00241 output.cls(); 00242 output.printf("Checking Pachube \nstatus:x"); 00243 errcnt++; 00244 wait(10); 00245 } 00246 }//if(etherconncet) 00247 }//for 00248 00249 //if firmware exist.reset. 00250 if(etherconnect){ 00251 if(fwup.exist()==0)mbed_reset(); 00252 } 00253 00254 //many error!! reset 00255 if(errcnt>20)mbed_reset(); 00256 }//while 00257 }
Generated on Wed Jul 13 2022 02:46:53 by
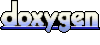