
TextLCD library for controlling various LCD panels based on the HD44780 4-bit interface
Fork of TextLCD by
mainLCD.cpp
00001 #include "mbed.h" 00002 #include "TextLCD.h" 00003 00004 TextLCD lcd(D2, D3, D4, D5, D6, D7); // rs, e, d4-d7 00005 00006 DigitalOut col1 (D8); 00007 DigitalOut col2 (D9); 00008 DigitalOut col3 (D10); 00009 DigitalOut col4 (D11); 00010 DigitalIn fila1 (A5); 00011 DigitalIn fila2 (A4); 00012 DigitalIn fila3 (A3); 00013 DigitalIn fila4 (A2); 00014 DigitalIn Reset (D12); 00015 DigitalIn Next (USER_BUTTON); 00016 00017 char Simbolos[4][4] = { 00018 {'1','2','3','A'}, 00019 {'4','5','6','B'}, 00020 {'7','8','9','C'}, 00021 {'*','0','#','D'} 00022 }; 00023 int usrInput(); 00024 void turnOffteclado(); 00025 int * teclado(); 00026 void printLCD(int a, int b); 00027 00028 00029 int n = 0; 00030 00031 int main() { 00032 00033 int j = 0; 00034 while(1){ 00035 if (n == 0) 00036 { 00037 j=0; 00038 lcd.cls(); 00039 wait(1); 00040 lcd.printf("A"); 00041 while (j == 0) 00042 { 00043 if (Next == 0) 00044 { 00045 j = 1; 00046 n = 1; 00047 } 00048 } 00049 } 00050 if (n == 1) 00051 { 00052 j = 0; 00053 lcd.cls(); 00054 wait(1); 00055 lcd.printf("Unas kguamas o k"); 00056 while (j == 0) 00057 { 00058 if (Next == 0) 00059 { 00060 j = 1; 00061 n =2; 00062 } 00063 } 00064 } 00065 while (n == 2) 00066 { 00067 j = 0; 00068 lcd.cls(); 00069 wait(1); 00070 lcd.printf("Unas kguamas o kDos kguamas o k?"); 00071 while (j == 0) 00072 { 00073 if (Next == 0) 00074 { 00075 j = 1; 00076 n =3; 00077 } 00078 } 00079 } 00080 lcd.cls(); 00081 wait(1); 00082 while (n == 3) 00083 { 00084 j = 0; 00085 int *Index ; 00086 Index = teclado(); 00087 printLCD(Index[0],Index[1]); 00088 00089 } 00090 00091 00092 } 00093 } 00094 00095 void printLCD(int a, int b) 00096 { 00097 lcd.printf("%c",Simbolos[a][b]); 00098 wait(.3); 00099 } 00100 00101 int * teclado() 00102 { 00103 static int r[2]; 00104 turnOffteclado(); 00105 int numero; 00106 while(1) 00107 { 00108 if (Next == 0) 00109 { 00110 lcd.cls(); 00111 n = 0; 00112 wait_ms(30); 00113 break; 00114 } 00115 00116 col1 = 1; 00117 numero = usrInput(); 00118 if (numero != 0) 00119 { 00120 r[0]=numero-1; 00121 r[1]=0; 00122 //wait_ms(30); 00123 return r; 00124 } 00125 turnOffteclado(); 00126 col2 = 1; 00127 numero = usrInput(); 00128 if (numero != 0) 00129 { 00130 r[0]=numero-1; 00131 r[1]=1; 00132 //wait_ms(30); 00133 return r; 00134 } 00135 turnOffteclado(); 00136 col3 = 1; 00137 numero = usrInput(); 00138 if (numero != 0) 00139 { 00140 r[0]=numero-1; 00141 r[1]=2; 00142 //wait_ms(30); 00143 return r; 00144 } 00145 turnOffteclado(); 00146 col4 = 1; 00147 numero = usrInput(); 00148 if (numero != 0) 00149 { 00150 r[0]=numero-1; 00151 r[1]=3; 00152 //wait_ms(30); 00153 return r; 00154 } 00155 turnOffteclado(); 00156 00157 } 00158 } 00159 00160 void turnOffteclado() 00161 { 00162 col1 = col2 = col3 = col4 = 0; 00163 } 00164 00165 int usrInput() 00166 { 00167 if (fila1 == 1) 00168 { 00169 return 1; 00170 } 00171 else if (fila2 == 1) 00172 { 00173 return 2; 00174 } 00175 else if (fila3 == 1) 00176 { 00177 return 3; 00178 } 00179 else if (fila4 == 1) 00180 { 00181 return 4; 00182 } 00183 else{ 00184 return 0; 00185 } 00186 }
Generated on Sun Jul 31 2022 21:32:47 by
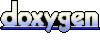