
This is a program to drive a stepper servomotor from SerialUSB without any other interrution but the serial one.
L293D.h
00001 #ifndef L293D_H 00002 #define L293d_H 00003 00004 #include "mbed.h" 00005 00006 /**@brief Class to handle L293D in two modes, Pwm or Digital. 00007 * Pwm Mode requires constructing an object with two PwmOut'puts to handle Speed. 00008 * Enable, or Digital Mode requires One DigitalOut'put to Enable or Disable both sides 00009 * of the L293D driver. 00010 * 00011 * This of course requires an 'special' wiring of the driver, using one transistor for each side. 00012 * The transistor is effectively a NOT gate, assuring that the direction of the current of the 00013 * driver output will be controlled using just one pin. 00014 * _____________ 00015 * Enable/ Pwm --->[1] 00016 * _5V__ | L 00017 * | _______________ [2] 00018 * _|_ / | 2 00019 * | | | ---------[3] 00020 * |1k| | | | 9 00021 * |__| | | (GND)[4] 00022 * /_________/ ( ~ ) | 3 00023 * _left |/ | (GND)[5] 00024 * -.---|470ohm|----|\ (Q 2N3904) | | 00025 * | | \ | -------[6] D 00026 * | |_(GND) | 00027 * | | 00028 * \___________________________________________ [7] 00029 * | 00030 * 5~15V-->[8]_____________ 00031 * 00032 * Hope you get my ASCII Art. same goes for the other side, thus allowing a complete control of the driver 00033 * with a few pins. 00034 * 00035 * Also for Sake of Simplicity SetSpeedLeft/Right will control Motor Speed and Direction, from -1.0 to 1.0 00036 * Reverse if < 0. 00037 */ 00038 class L293D{ 00039 00040 public: 00041 00042 /**@brief 00043 * Two PWM for speed Cpntrol & two digital outs needed for setting direction 00044 * on each side of the L293D. 00045 * @param left: Output to the left side of the driver 00046 * @param right: Output to the right side of the driver 00047 * @param pwmLeft: Left side PWM. 00048 * @param pwmRight: Right side PWM. 00049 */ 00050 L293D( PwmOut pwmLeft, PwmOut right, DigitalOut left, DigitalOut right ); 00051 00052 00053 /**@brief 00054 * Set the left PWM to current speed and Direction. 00055 * Values from -1.0 to 1.0, to control direction and speed, 00056 * if Enable mode, only use -1.0 & 1.0 00057 */ 00058 void setSpeedLeft( float speed ); 00059 00060 00061 /**@brief 00062 * Set the right PWM to current speed and direction. 00063 * Values from -1.0 to 1.0, to control direction and speed, 00064 * if Enable mode, only use -1.0 & 1.0 00065 * Only to use when PWM mode is declared 00066 */ 00067 void setSpeedRight( float speed ); 00068 00069 00070 /**@brief 00071 * Read the left PWM current speed & direction 00072 * Ranges from -1.0 to 1.0, as being set. 00073 * @return Current PWM duty cycle. 00074 */ 00075 float getSpeedLeft(); 00076 00077 00078 /**@brief 00079 * Read the left PWM current speed & direction. 00080 * Ranges from -1.0 to 1.0 as being set. 00081 * @return Current PWM duty cycle. 00082 */ 00083 float getSpeedRight(); 00084 00085 00086 private: 00087 00088 DigitalOut _left; 00089 DigitalOut _right; 00090 PwmOut _pwmLeft; 00091 PwmOut _pwmRight; 00092 00093 float _speedLeft; 00094 float _speedRight; 00095 00096 }; 00097 00098 #endif
Generated on Mon Jul 25 2022 22:31:17 by
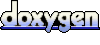