
avant le lab
Dependencies: X_NUCLEO_6180XA1 mbed
Fork of 247-436-M1-S1-LAB-1 by
main.cpp
00001 #include "mbed.h" 00002 #include "XNucleo6180XA1.h" 00003 #include <string.h> 00004 #include <stdlib.h> 00005 #include <stdio.h> 00006 #include <assert.h> 00007 00008 //options de compilation 00009 //#define TEST 00010 00011 //intégration 00012 // représentation 00013 // G: gauche, D: droit, H: haut, B: bas 00014 // E: écran, T: touch 00015 // Ecran: 00016 // (XE_G,YE_H) ___ (XE_D,YE_H) 00017 // | | 00018 // | | 00019 // (XE_G,YE_B) |___| (XE_G,YE_B) 00020 // 00021 // représentation du touch 00022 // (XT_G,YT_H) ___ (XT_D,YT_H) 00023 // | | 00024 // | | 00025 // (XT_G,YT_B) |___| (XT_G,YT_B) 00026 00027 // équations pour conversion 00028 // XT: x touch lu, YT: y touch lu, XE: x ecran, YE: y ecran 00029 // XE = (XE_D - XE_G)/(XT_D - XT_G)*(XT - XT_G) + XE_G 00030 // YE = (YE_H - YE_B)/(YT_H - YT_B)*(YT - YT_G) + YE_G 00031 00032 // l'application présente 3 boutons et une surface de dessin 00033 // bouton noir: effacer 00034 // bouton rouge: dessiner en rouge 00035 // bouton blanc: dessiner en blanc 00036 00037 //touchscreen 00038 #define IDLE 1 00039 #define ACTIVE 0 00040 #define COMMAND 0 00041 #define DATA 1 00042 00043 #define YP A3 00044 #define XM A2 00045 #define YM D9 00046 #define XP D8 00047 00048 #define TOUCH_SEUIL_HAUT 3000 00049 #define TOUCH_SEUIL_BAS 16 00050 00051 00052 #define TFTWIDTH 240 00053 #define TFTHEIGHT 320 00054 00055 #define LCD_CS A3 00056 #define LCD_CD A2 00057 #define LCD_WR A1 00058 #define LCD_RD A0 00059 #define LCD_RESET A4 00060 00061 #define TFT_NORTH SPFD5408_MADCTL_MY | SPFD5408_MADCTL_BGR 00062 00063 #define BLACK 0x0000 00064 #define BLUE 0x001F 00065 #define RED 0xF800 00066 #define GREEN 0x07E0 00067 #define CYAN 0x07FF 00068 #define MAGENTA 0xF81F 00069 #define YELLOW 0xFFE0 00070 #define WHITE 0xFFFF 00071 00072 #define SPFD5408_SOFTRESET 0x01 00073 #define SPFD5408_SLEEPOUT 0x11 00074 00075 #define SPFD5408_DISPLAYON 0x29 00076 #define SPFD5408_COLADDRSET 0x2A 00077 #define SPFD5408_PAGEADDRSET 0x2B 00078 #define SPFD5408_MEMORYWRITE 0x2C 00079 #define SPFD5408_PIXELFORMAT 0x3A 00080 #define SPFD5408_FRAMECONTROL 0xB1 00081 #define SPFD5408_MEMCONTROL 0x36 00082 #define SPFD5408_MADCTL_MY 0x80 00083 #define SPFD5408_MADCTL_BGR 0x08 00084 00085 #define IDLE 1 00086 #define ACTIVE 0 00087 #define COMMAND 0 00088 #define DATA 1 00089 00090 #define TEMPS 40 00091 00092 //VL6180X 00093 #define VL6180X_I2C_SDA D14 00094 #define VL6180X_I2C_SCL D15 00095 00096 #define VL6180X_I2C_SDA_MODE PullUp 00097 #define VL6180X_I2C_SCL_MODE PullUp 00098 00099 00100 //integration 00101 00102 #define XE_G 0 00103 #define XE_D 240 00104 #define YE_H 0 00105 #define YE_B 320 00106 #define XT_G 3700 00107 #define XT_D 600 00108 #define YT_H 600 00109 #define YT_B 3700 00110 00111 #define MODE_COULEUR_CONSTANTE 0 00112 #define MODE_COULEUR_VARIABLE 1 00113 //definitions de variables 00114 //touchscreen 00115 uint16_t x; 00116 uint16_t y; 00117 uint16_t z; 00118 00119 //tftspfd5408 00120 DigitalOut pinRD(LCD_RD); //PA_0; 00121 DigitalOut pinWR(LCD_WR); //PA_1; 00122 DigitalOut pinCD(LCD_CD); //PA_4; 00123 DigitalOut pinCS(LCD_CS); //PB_0; 00124 DigitalOut pinReset(LCD_RESET); //PC_1; 00125 00126 //VL6180X 00127 DigitalOut enableVL6180X(D12); 00128 static XNucleo6180XA1 *board = NULL; 00129 uint32_t dist; 00130 00131 00132 //integration 00133 #ifdef TEST 00134 Serial pc(USBTX, USBRX, 9600); //SERIAL_TX, SERIAL_RX, 9600); 00135 #endif 00136 DigitalOut myled(LED1); 00137 uint16_t couleurVariable; 00138 uint16_t tableDeCouleur[]= 00139 { 00140 BLACK, RED, YELLOW, GREEN, CYAN, BLUE, MAGENTA, WHITE 00141 }; 00142 00143 00144 //declarations de fonctions 00145 //touchscreen 00146 void restoreXY(void); 00147 uint16_t readTouchX(void); 00148 uint16_t readTouchY(void); 00149 uint16_t detectTouch(void); 00150 00151 //tftspfd5408 00152 void WriteCommand(uint8_t c); 00153 void WriteData(uint8_t d); 00154 void begin(void); 00155 void setAddrWindow(int x1, int y1, int x2, int y2); 00156 void fillRect(uint16_t x1, uint16_t y1, uint16_t w, uint16_t h, uint16_t fillcolor); 00157 00158 //definitions de fonctions 00159 //touchscreen 00160 void restoreXY(void) 00161 { 00162 DigitalOut pinXP(XP); 00163 DigitalOut pinXM(XM); 00164 DigitalOut pinYP(YP); 00165 DigitalOut pinYM(YM); 00166 00167 pinXP = 1; 00168 pinXM = 1; 00169 pinYP = 1; 00170 pinYM = 1; 00171 wait_ms(1); 00172 } 00173 00174 uint16_t readTouchX(void) 00175 { 00176 uint16_t value; 00177 DigitalOut pinXP(XP); 00178 DigitalOut pinXM(XM); 00179 AnalogIn pinYP(YP); 00180 DigitalIn pinYM(YM); 00181 00182 pinXP = 0; 00183 pinXM = 1; 00184 pinYM.mode(OpenDrain); 00185 value = pinYP.read_u16() >> 4; 00186 restoreXY(); 00187 return value; 00188 } 00189 00190 uint16_t readTouchY(void) 00191 { 00192 uint16_t value; 00193 DigitalIn pinXP(XP); 00194 AnalogIn pinXM(XM); 00195 DigitalOut pinYP(YP); 00196 DigitalOut pinYM(YM); 00197 00198 pinYP = 1; 00199 pinYM = 0; 00200 pinXP.mode(OpenDrain); 00201 value = pinXM.read_u16() >> 4; 00202 restoreXY(); 00203 return value; 00204 } 00205 00206 uint16_t detectTouch(void) 00207 { 00208 uint16_t firstValue; 00209 uint16_t secondValue; 00210 DigitalOut pinXP(XP); 00211 DigitalOut pinXM(XM); 00212 AnalogIn pinYP(YP); 00213 DigitalIn pinYM(YM); 00214 00215 pinYM.mode(OpenDrain); 00216 pinXP = 1; 00217 pinXM = 0; 00218 firstValue = pinYP.read_u16() >> 4; 00219 00220 pinXP = 0; 00221 pinXM = 1; 00222 secondValue = pinYP.read_u16() >> 4; 00223 00224 restoreXY(); 00225 00226 if (secondValue > firstValue) 00227 { 00228 return firstValue; 00229 } 00230 return secondValue; 00231 } 00232 00233 //tftspfd5408 00234 void WriteCommand(uint8_t c) 00235 { 00236 BusInOut portTFT(D8, D9, D2, D3, D4, D5, D6, D7); 00237 portTFT.output(); 00238 pinCD = COMMAND; 00239 pinWR = ACTIVE; 00240 portTFT = c; 00241 pinWR = IDLE; 00242 } 00243 00244 void WriteData(uint8_t d) 00245 { 00246 BusInOut portTFT(D8, D9, D2, D3, D4, D5, D6, D7); 00247 portTFT.output(); 00248 pinCD = DATA; 00249 pinWR = ACTIVE; 00250 portTFT = d; 00251 pinWR = IDLE; 00252 } 00253 00254 void begin(void) 00255 { 00256 pinCS = IDLE; 00257 pinCD = DATA; 00258 pinWR = IDLE; 00259 pinRD = IDLE; 00260 00261 pinReset = ACTIVE; 00262 wait_ms(100); 00263 pinReset = IDLE; 00264 wait_ms(100); 00265 pinCS = ACTIVE; 00266 00267 WriteCommand(SPFD5408_SOFTRESET); 00268 WriteData(0); 00269 wait_ms(50); 00270 00271 WriteCommand(SPFD5408_MEMCONTROL); 00272 WriteData(TFT_NORTH); //(SPFD5408_MADCTL_MY | SPFD5408_MADCTL_BGR); 00273 00274 WriteCommand(SPFD5408_PIXELFORMAT); 00275 WriteData(0x55); 00276 00277 WriteCommand(SPFD5408_FRAMECONTROL); 00278 WriteData(0x00); 00279 WriteData(0x1B); 00280 00281 WriteCommand(SPFD5408_SLEEPOUT); 00282 WriteData(0); 00283 00284 WriteCommand(SPFD5408_DISPLAYON); 00285 WriteData(0); 00286 } 00287 00288 void setAddrWindow(int x1, int y1, int x2, int y2) { 00289 pinCS = ACTIVE; 00290 wait_us(TEMPS); 00291 WriteCommand(SPFD5408_COLADDRSET); 00292 WriteData(x1 >> 8); 00293 WriteData(x1); 00294 WriteData(x2 >> 8); 00295 WriteData(x2); 00296 wait_us(TEMPS); 00297 pinCS = IDLE; 00298 00299 pinCS = ACTIVE; 00300 wait_us(TEMPS); 00301 WriteCommand(SPFD5408_PAGEADDRSET); 00302 WriteData(y1 >> 8); 00303 WriteData(y1); 00304 WriteData(y2 >> 8); 00305 WriteData(y2); 00306 pinCS = IDLE; 00307 } 00308 00309 void fillRect(uint16_t x1, uint16_t y1, uint16_t w, uint16_t h, uint16_t fillcolor) 00310 { 00311 BusInOut portTFT(D8, D9, D2, D3, D4, D5, D6, D7); 00312 uint8_t hi, lo; 00313 uint16_t x2, y2; 00314 uint16_t i, j; 00315 00316 portTFT.output(); 00317 00318 x2 = x1 + w - 1; 00319 y2 = y1 + h - 1; 00320 setAddrWindow(x1, y1, x2, y2); 00321 00322 hi = fillcolor >> 8; 00323 lo = fillcolor; 00324 00325 pinCS = ACTIVE; 00326 00327 WriteCommand(SPFD5408_MEMORYWRITE); 00328 pinCD = DATA; 00329 for (i = h; i > 0; i--) 00330 { 00331 for (j = w; j > 0; j--) 00332 00333 { 00334 pinWR = ACTIVE; 00335 portTFT = hi; 00336 pinWR = IDLE; 00337 pinWR = ACTIVE; 00338 portTFT = lo; 00339 pinWR = IDLE; 00340 } 00341 } 00342 pinCS = IDLE; 00343 } 00344 00345 //intégration 00346 00347 int32_t conversion(int32_t x, int32_t x0, int32_t y0, int32_t x1, int32_t y1) 00348 { 00349 int32_t retour; 00350 int32_t limiteInferieure; 00351 int32_t limiteSuperieure; 00352 if (y0 < y1) 00353 { 00354 limiteInferieure = y0; 00355 limiteSuperieure = y1; 00356 } 00357 else 00358 { 00359 limiteInferieure = y1; 00360 limiteSuperieure = y0; 00361 } 00362 retour = (x - x0) * (y1 -y0) / (x1 -x0) + y0; 00363 00364 if (retour < limiteInferieure) 00365 { 00366 return limiteInferieure; 00367 } 00368 if (retour > limiteSuperieure) 00369 { 00370 return limiteSuperieure; 00371 } 00372 return retour; 00373 }; 00374 00375 uint16_t determineLaCouleur(void) 00376 { 00377 board->sensor_top->get_distance(&dist); 00378 if (dist > 210) //en millimetres 00379 { 00380 dist = 210; 00381 } 00382 // board->sensor_top->get_lux(&lux); 00383 00384 return tableDeCouleur[(int)(dist/30)]; 00385 } 00386 00387 int main() 00388 { 00389 //variables locales du touchscreen 00390 uint16_t couleur; 00391 00392 //variables locales du tftspfd5408 00393 00394 //variables locales pour le VL6180X 00395 int status; 00396 uint32_t lux; 00397 00398 DevI2C *device_i2c = new DevI2C(VL6180X_I2C_SDA, VL6180X_I2C_SCL); 00399 //variables locales pour l'integration 00400 uint32_t mode; 00401 00402 //initialisation du touchscreen 00403 restoreXY(); 00404 00405 //initialisation du tftspfd5408 00406 begin(); 00407 00408 //initialisation du VL6180X 00409 // printf ("Initialisation *********\n"); 00410 //yr 00411 // pin_mode(VL6180X_I2C_SDA, (PinMode)PullUp); 00412 // pin_mode(VL6180X_I2C_SCL, (PinMode)PullUp); 00413 00414 enableVL6180X = 1; 00415 wait_ms(1); 00416 enableVL6180X = 0; 00417 wait_ms(1); 00418 enableVL6180X = 1; 00419 wait_ms(1); 00420 00421 /* Creates the 6180XA1 expansion board singleton obj. */ 00422 board = XNucleo6180XA1::instance(device_i2c, D11, D10, D13, D2); 00423 00424 /* Initializes the 6180XA1 expansion board with default values. */ 00425 status = board->init_board(); 00426 if (status) { 00427 printf("Failed to init board!\n\r"); 00428 return 0; 00429 } 00430 00431 //intégration 00432 mode = MODE_COULEUR_CONSTANTE; 00433 couleur = RED; 00434 couleurVariable = BLUE; 00435 fillRect(0, 0, 240, 280, BLACK); //surface de dessin 00436 //bouton noir 00437 fillRect(0, 280, 80, 2, WHITE); // ligne horizontale haut 00438 fillRect(0, 282, 2, 38, WHITE); // ligne verticale gauche 00439 fillRect(79, 282, 1, 38, WHITE); // ligne verticale droite 00440 fillRect(2, 318, 77, 2, WHITE); //ligne horizontale bas 00441 fillRect(2, 282, 77, 36, BLACK); // centre noir 00442 //bouton rouge 00443 fillRect(80, 280, 80, 40, WHITE); // moins d'instructions mais plus long 00444 fillRect(81, 282, 78, 36, RED); // bouton rouge 00445 //bouton cyan 00446 fillRect(160, 280, 80, 40, WHITE); // moins d'instructions mais plus long 00447 fillRect(161, 282, 77, 36, BLUE); //bouton bleu 00448 //indicateur 00449 fillRect(81, 282, 10, 10, GREEN); //couleur rouge par défaut 00450 00451 while(1) { 00452 00453 #ifdef TEST 00454 printf ("Distance: %d, Lux: %d\n\r", dist, lux); 00455 #endif 00456 00457 //tâches du touchscreen 00458 myled = !myled; 00459 00460 x = readTouchX(); 00461 y = readTouchY(); 00462 z = detectTouch(); 00463 //tâches du tftspfd5408 00464 00465 00466 //tâches d'intégration 00467 couleurVariable = determineLaCouleur(); 00468 // fillRect(228, 282, 10, 10, couleurVariable); //indicateur de couleur 00469 00470 if ((z > TOUCH_SEUIL_BAS)&&(z < TOUCH_SEUIL_HAUT)) 00471 { 00472 #ifdef TEST 00473 pc.printf("x lu %u\t ", x); 00474 pc.printf("y lu %u\t ", y); 00475 pc.printf("z lu %u\t ", z); 00476 #endif 00477 float u = conversion((float)x, XT_G, XE_G, XT_D, XE_D); 00478 float v = conversion((float)y, YT_B, YE_B, YT_H, YE_H); 00479 00480 #ifdef TEST 00481 pc.printf("x ecran %f\t ", u); 00482 pc.printf("y ecran %f\r\n", v); 00483 #endif 00484 if (v < 280) 00485 { 00486 if (mode == MODE_COULEUR_CONSTANTE) 00487 { 00488 fillRect(u, v, 3, 3, couleur); 00489 } 00490 else 00491 { 00492 fillRect(u, v, 3, 3, couleurVariable); 00493 } 00494 } 00495 else 00496 { 00497 if (u < 80) 00498 { 00499 fillRect(0, 0, 240, 280, BLACK); 00500 } 00501 else 00502 { 00503 if (u < 160) 00504 { 00505 mode = MODE_COULEUR_CONSTANTE; 00506 //indicateur RED 00507 fillRect(81, 282, 10, 10, GREEN); //couleur rouge par défaut 00508 fillRect(161,282, 10, 10, BLUE); 00509 00510 #ifdef TEST 00511 pc.printf("couleur: rouge\n\r"); 00512 #endif 00513 } 00514 else 00515 { 00516 mode = MODE_COULEUR_VARIABLE; 00517 //indicateur AUTRE 00518 fillRect(81, 282, 10, 10, RED); 00519 fillRect(161, 282, 77, 36, BLUE); 00520 fillRect(161, 282, 10, 10, GREEN); 00521 00522 #ifdef TEST 00523 pc.printf("couleur variable\n\r"); 00524 #endif 00525 } 00526 } 00527 } 00528 } 00529 } 00530 }
Generated on Sat Jul 16 2022 23:12:51 by
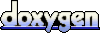