1
Dependents: internet_radio_leo
Fork of WIZnetInterface by
W5500.cpp
00001 /* Copyright (C) 2012 mbed.org, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00005 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00006 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00007 * furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 #include "eth_arch.h" 00019 #if (not defined TARGET_WIZwiki_W7500) && (not defined TARGET_WIZwiki_W7500P) 00020 00021 00022 #include "mbed.h" 00023 #include "mbed_debug.h" 00024 #include "DNSClient.h" 00025 00026 00027 //Debug is disabled by default 00028 #if 0 00029 #define DBG(...) do{debug("%p %d %s ", this,__LINE__,__PRETTY_FUNCTION__); debug(__VA_ARGS__); } while(0); 00030 //#define DBG(x, ...) debug("[W5500:DBG]"x"\r\n", ##__VA_ARGS__); 00031 #define WARN(x, ...) debug("[W5500:WARN]"x"\r\n", ##__VA_ARGS__); 00032 #define ERR(x, ...) debug("[W5500:ERR]"x"\r\n", ##__VA_ARGS__); 00033 #else 00034 #define DBG(x, ...) 00035 #define WARN(x, ...) 00036 #define ERR(x, ...) 00037 #endif 00038 00039 #if 1 00040 #define INFO(x, ...) debug("[W5500:INFO]"x"\r\n", ##__VA_ARGS__); 00041 #else 00042 #define INFO(x, ...) 00043 #endif 00044 00045 #define DBG_SPI 0 00046 00047 WIZnet_Chip* WIZnet_Chip::inst; 00048 00049 WIZnet_Chip::WIZnet_Chip(PinName mosi, PinName miso, PinName sclk, PinName _cs, PinName _reset): 00050 cs(_cs), reset_pin(_reset) 00051 { 00052 spi = new SPI(mosi, miso, sclk); 00053 cs = 1; 00054 reset_pin = 1; 00055 inst = this; 00056 sock_any_port = SOCK_ANY_PORT_NUM; 00057 } 00058 00059 WIZnet_Chip::WIZnet_Chip(SPI* spi, PinName _cs, PinName _reset): 00060 cs(_cs), reset_pin(_reset) 00061 { 00062 this->spi = spi; 00063 cs = 1; 00064 reset_pin = 1; 00065 inst = this; 00066 sock_any_port = SOCK_ANY_PORT_NUM; 00067 } 00068 00069 bool WIZnet_Chip::setmac() 00070 { 00071 00072 for (int i =0; i < 6; i++) reg_wr<uint8_t>(SHAR+i, mac[i]); 00073 00074 return true; 00075 } 00076 00077 // Set the IP 00078 bool WIZnet_Chip::setip() 00079 { 00080 reg_wr<uint32_t>(SIPR, ip); 00081 reg_wr<uint32_t>(GAR, gateway); 00082 reg_wr<uint32_t>(SUBR, netmask); 00083 return true; 00084 } 00085 00086 bool WIZnet_Chip::setProtocol(int socket, Protocol p) 00087 { 00088 if (socket < 0) { 00089 return false; 00090 } 00091 sreg<uint8_t>(socket, Sn_MR, p); 00092 return true; 00093 } 00094 00095 bool WIZnet_Chip::connect(int socket, const char * host, int port, int timeout_ms) 00096 { 00097 if (socket < 0) { 00098 return false; 00099 } 00100 sreg<uint8_t>(socket, Sn_MR, TCP); 00101 scmd(socket, OPEN); 00102 sreg_ip(socket, Sn_DIPR, host); 00103 sreg<uint16_t>(socket, Sn_DPORT, port); 00104 sreg<uint16_t>(socket, Sn_PORT, new_port()); 00105 scmd(socket, CONNECT); 00106 Timer t; 00107 t.reset(); 00108 t.start(); 00109 while(!is_connected(socket)) { 00110 if (t.read_ms() > timeout_ms) { 00111 return false; 00112 } 00113 } 00114 return true; 00115 } 00116 00117 bool WIZnet_Chip::gethostbyname(const char* host, uint32_t* ip) 00118 { 00119 uint32_t addr = str_to_ip(host); 00120 char buf[17]; 00121 snprintf(buf, sizeof(buf), "%d.%d.%d.%d", (addr>>24)&0xff, (addr>>16)&0xff, (addr>>8)&0xff, addr&0xff); 00122 if (strcmp(buf, host) == 0) { 00123 *ip = addr; 00124 return true; 00125 } 00126 DNSClient client; 00127 if(client.lookup(host)) { 00128 *ip = client.ip; 00129 return true; 00130 } 00131 return false; 00132 } 00133 00134 bool WIZnet_Chip::disconnect() 00135 { 00136 return true; 00137 } 00138 00139 bool WIZnet_Chip::is_connected(int socket) 00140 { 00141 /* 00142 if (sreg<uint8_t>(socket, Sn_SR) == SOCK_ESTABLISHED) { 00143 return true; 00144 } 00145 */ 00146 uint8_t tmpSn_SR; 00147 tmpSn_SR = sreg<uint8_t>(socket, Sn_SR); 00148 // packet sending is possible, when state is SOCK_CLOSE_WAIT. 00149 if ((tmpSn_SR == SOCK_ESTABLISHED) || (tmpSn_SR == SOCK_CLOSE_WAIT)) { 00150 return true; 00151 } 00152 return false; 00153 } 00154 00155 // Reset the chip & set the buffer 00156 void WIZnet_Chip::reset() 00157 { 00158 #if defined(USE_WIZ550IO_MAC) 00159 //read the MAC address inside the module 00160 reg_rd_mac(SHAR, mac); 00161 #endif 00162 // hw reset 00163 reset_pin = 1; 00164 reset_pin = 0; 00165 wait_us(500); // 500us (w5500) 00166 reset_pin = 1; 00167 wait_ms(400); // 400ms (w5500) 00168 #if defined(USE_WIZ550IO_MAC) 00169 // write MAC address inside the WZTOE MAC address register 00170 reg_wr_mac(SHAR, mac); 00171 #endif 00172 // set RX and TX buffer size 00173 #if 0 00174 for (int socket = 0; socket < MAX_SOCK_NUM; socket++) { 00175 sreg<uint8_t>(socket, Sn_RXBUF_SIZE, 2); 00176 sreg<uint8_t>(socket, Sn_TXBUF_SIZE, 2); 00177 } 00178 #endif 00179 } 00180 00181 00182 bool WIZnet_Chip::close(int socket) 00183 { 00184 if (socket < 0) { 00185 return false; 00186 } 00187 // if not connected, return 00188 if (sreg<uint8_t>(socket, Sn_SR) == SOCK_CLOSED) { 00189 return true; 00190 } 00191 if (sreg<uint8_t>(socket, Sn_MR) == TCP) { 00192 scmd(socket, DISCON); 00193 } 00194 scmd(socket, CLOSE); 00195 sreg<uint8_t>(socket, Sn_IR, 0xff); 00196 return true; 00197 } 00198 00199 int WIZnet_Chip::wait_readable(int socket, int wait_time_ms, int req_size) 00200 { 00201 if (socket < 0) { 00202 return -1; 00203 } 00204 Timer t; 00205 t.reset(); 00206 t.start(); 00207 while(1) { 00208 //int size = sreg<uint16_t>(socket, Sn_RX_RSR); 00209 // during the reading Sn_RX_RXR, it has the possible change of this register. 00210 // so read twice and get same value then use size information. 00211 int size, size2; 00212 do { 00213 size = sreg<uint16_t>(socket, Sn_RX_RSR); 00214 size2 = sreg<uint16_t>(socket, Sn_RX_RSR); 00215 } while (size != size2); 00216 00217 if (size > req_size) { 00218 return size; 00219 } 00220 if (wait_time_ms != (-1) && t.read_ms() > wait_time_ms) { 00221 break; 00222 } 00223 } 00224 return -1; 00225 } 00226 00227 int WIZnet_Chip::wait_writeable(int socket, int wait_time_ms, int req_size) 00228 { 00229 if (socket < 0) { 00230 return -1; 00231 } 00232 Timer t; 00233 t.reset(); 00234 t.start(); 00235 while(1) { 00236 //int size = sreg<uint16_t>(socket, Sn_TX_FSR); 00237 // during the reading Sn_TX_FSR, it has the possible change of this register. 00238 // so read twice and get same value then use size information. 00239 int size, size2; 00240 do { 00241 size = sreg<uint16_t>(socket, Sn_TX_FSR); 00242 size2 = sreg<uint16_t>(socket, Sn_TX_FSR); 00243 } while (size != size2); 00244 00245 if (size > req_size) { 00246 return size; 00247 } 00248 if (wait_time_ms != (-1) && t.read_ms() > wait_time_ms) { 00249 break; 00250 } 00251 } 00252 return -1; 00253 } 00254 00255 int WIZnet_Chip::send(int socket, const char * str, int len) 00256 { 00257 if (socket < 0) { 00258 return -1; 00259 } 00260 uint16_t ptr = sreg<uint16_t>(socket, Sn_TX_WR); 00261 uint8_t cntl_byte = (0x14 + (socket << 5)); 00262 spi_write(ptr, cntl_byte, (uint8_t*)str, len); 00263 sreg<uint16_t>(socket, Sn_TX_WR, ptr + len); 00264 scmd(socket, SEND); 00265 uint8_t tmp_Sn_IR; 00266 while (( (tmp_Sn_IR = sreg<uint8_t>(socket, Sn_IR)) & INT_SEND_OK) != INT_SEND_OK) { 00267 // @Jul.10, 2014 fix contant name, and udp sendto function. 00268 switch (sreg<uint8_t>(socket, Sn_SR)) { 00269 case SOCK_CLOSED : 00270 close(socket); 00271 return 0; 00272 //break; 00273 case SOCK_UDP : 00274 // ARP timeout is possible. 00275 if ((tmp_Sn_IR & INT_TIMEOUT) == INT_TIMEOUT) { 00276 sreg<uint8_t>(socket, Sn_IR, INT_TIMEOUT); 00277 return 0; 00278 } 00279 break; 00280 default : 00281 break; 00282 } 00283 } 00284 /* 00285 while ((sreg<uint8_t>(socket, Sn_IR) & INT_SEND_OK) != INT_SEND_OK) { 00286 if (sreg<uint8_t>(socket, Sn_SR) == CLOSED) { 00287 close(socket); 00288 return 0; 00289 } 00290 } 00291 */ 00292 sreg<uint8_t>(socket, Sn_IR, INT_SEND_OK); 00293 00294 return len; 00295 } 00296 00297 int WIZnet_Chip::recv(int socket, char* buf, int len) 00298 { 00299 if (socket < 0) { 00300 return -1; 00301 } 00302 uint16_t ptr = sreg<uint16_t>(socket, Sn_RX_RD); 00303 uint8_t cntl_byte = (0x18 + (socket << 5)); 00304 spi_read(ptr, cntl_byte, (uint8_t*)buf, len); 00305 sreg<uint16_t>(socket, Sn_RX_RD, ptr + len); 00306 scmd(socket, RECV); 00307 return len; 00308 } 00309 00310 int WIZnet_Chip::new_socket() 00311 { 00312 for(int s = 0; s < MAX_SOCK_NUM; s++) { 00313 if (sreg<uint8_t>(s, Sn_SR) == SOCK_CLOSED) { 00314 return s; 00315 } 00316 } 00317 return -1; 00318 } 00319 00320 uint16_t WIZnet_Chip::new_port() 00321 { 00322 uint16_t port = rand(); 00323 port |= 49152; 00324 return port; 00325 } 00326 00327 bool WIZnet_Chip::link(int wait_time_ms) 00328 { 00329 Timer t; 00330 t.reset(); 00331 t.start(); 00332 while(1) { 00333 int is_link = ethernet_link(); 00334 00335 if (is_link) { 00336 return true; 00337 } 00338 if (wait_time_ms != (-1) && t.read_ms() > wait_time_ms) { 00339 break; 00340 } 00341 } 00342 return 0; 00343 } 00344 00345 void WIZnet_Chip::set_link(PHYMode phymode) 00346 { 00347 int speed = -1; 00348 int duplex = 0; 00349 00350 switch(phymode) { 00351 case AutoNegotiate : speed = -1; duplex = 0; break; 00352 case HalfDuplex10 : speed = 0; duplex = 0; break; 00353 case FullDuplex10 : speed = 0; duplex = 1; break; 00354 case HalfDuplex100 : speed = 1; duplex = 0; break; 00355 case FullDuplex100 : speed = 1; duplex = 1; break; 00356 } 00357 00358 ethernet_set_link(speed, duplex); 00359 } 00360 00361 void WIZnet_Chip::scmd(int socket, Command cmd) 00362 { 00363 sreg<uint8_t>(socket, Sn_CR, cmd); 00364 while(sreg<uint8_t>(socket, Sn_CR)); 00365 } 00366 00367 void WIZnet_Chip::spi_write(uint16_t addr, uint8_t cb, const uint8_t *buf, uint16_t len) 00368 { 00369 cs = 0; 00370 spi->write(addr >> 8); 00371 spi->write(addr & 0xff); 00372 spi->write(cb); 00373 for(int i = 0; i < len; i++) { 00374 spi->write(buf[i]); 00375 } 00376 cs = 1; 00377 00378 #if DBG_SPI 00379 debug("[SPI]W %04x(%02x %d)", addr, cb, len); 00380 for(int i = 0; i < len; i++) { 00381 debug(" %02x", buf[i]); 00382 if (i > 16) { 00383 debug(" ..."); 00384 break; 00385 } 00386 } 00387 debug("\r\n"); 00388 #endif 00389 } 00390 00391 void WIZnet_Chip::spi_read(uint16_t addr, uint8_t cb, uint8_t *buf, uint16_t len) 00392 { 00393 cs = 0; 00394 spi->write(addr >> 8); 00395 spi->write(addr & 0xff); 00396 spi->write(cb); 00397 for(int i = 0; i < len; i++) { 00398 buf[i] = spi->write(0); 00399 } 00400 cs = 1; 00401 00402 #if DBG_SPI 00403 debug("[SPI]R %04x(%02x %d)", addr, cb, len); 00404 for(int i = 0; i < len; i++) { 00405 debug(" %02x", buf[i]); 00406 if (i > 16) { 00407 debug(" ..."); 00408 break; 00409 } 00410 } 00411 debug("\r\n"); 00412 if ((addr&0xf0ff)==0x4026 || (addr&0xf0ff)==0x4003) { 00413 wait_ms(200); 00414 } 00415 #endif 00416 } 00417 00418 uint32_t str_to_ip(const char* str) 00419 { 00420 uint32_t ip = 0; 00421 char* p = (char*)str; 00422 for(int i = 0; i < 4; i++) { 00423 ip |= atoi(p); 00424 p = strchr(p, '.'); 00425 if (p == NULL) { 00426 break; 00427 } 00428 ip <<= 8; 00429 p++; 00430 } 00431 return ip; 00432 } 00433 00434 void printfBytes(char* str, uint8_t* buf, int len) 00435 { 00436 printf("%s %d:", str, len); 00437 for(int i = 0; i < len; i++) { 00438 printf(" %02x", buf[i]); 00439 } 00440 printf("\n"); 00441 } 00442 00443 void printHex(uint8_t* buf, int len) 00444 { 00445 for(int i = 0; i < len; i++) { 00446 if ((i%16) == 0) { 00447 printf("%p", buf+i); 00448 } 00449 printf(" %02x", buf[i]); 00450 if ((i%16) == 15) { 00451 printf("\n"); 00452 } 00453 } 00454 printf("\n"); 00455 } 00456 00457 void debug_hex(uint8_t* buf, int len) 00458 { 00459 for(int i = 0; i < len; i++) { 00460 if ((i%16) == 0) { 00461 debug("%p", buf+i); 00462 } 00463 debug(" %02x", buf[i]); 00464 if ((i%16) == 15) { 00465 debug("\n"); 00466 } 00467 } 00468 debug("\n"); 00469 } 00470 00471 int WIZnet_Chip::ethernet_link(void) { 00472 int val = getPHYCFGR(); 00473 return (val&0x01); 00474 } 00475 00476 void WIZnet_Chip::ethernet_set_link(int speed, int duplex) { 00477 uint32_t val=0; 00478 if((speed < 0) || (speed > 1)) { 00479 val = (PHYCFGR_OPMDC_ALLA)<<3; 00480 } else { 00481 val = (((speed&0x01)<<1)+ (duplex&0x01))<<3; 00482 } 00483 setPHYCFGR((uint8_t)(PHYCFGR_RST&(PHYCFGR_OPMD|val))); 00484 wait(0.2); 00485 setPHYCFGR((uint8_t)((~PHYCFGR_RST)|(PHYCFGR_OPMD|val))); 00486 wait(0.2); 00487 } 00488 00489 void WIZnet_Chip::reg_rd_mac(uint16_t addr, uint8_t* data) { 00490 spi_read(addr, 0x00, data, 6); 00491 } 00492 00493 void WIZnet_Chip::reg_wr_ip(uint16_t addr, uint8_t cb, const char* ip) { 00494 uint8_t buf[4]; 00495 char* p = (char*)ip; 00496 for(int i = 0; i < 4; i++) { 00497 buf[i] = atoi(p); 00498 p = strchr(p, '.'); 00499 if (p == NULL) { 00500 break; 00501 } 00502 p++; 00503 } 00504 spi_write(addr, cb, buf, sizeof(buf)); 00505 } 00506 00507 void WIZnet_Chip::sreg_ip(int socket, uint16_t addr, const char* ip) { 00508 reg_wr_ip(addr, (0x0C + (socket << 5)), ip); 00509 } 00510 00511 void WIZnet_Chip::reg_rd_ip_byte(uint16_t addr, uint8_t* data) { 00512 spi_read(addr, 0x00, data, 4); 00513 } 00514 00515 void WIZnet_Chip::reg_wr_ip_byte(uint16_t addr, uint8_t* data) { 00516 spi_write(addr, 0x04, data, 4); 00517 } 00518 00519 00520 #endif 00521
Generated on Wed Jul 13 2022 02:46:21 by
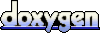