1
Dependents: internet_radio_leo
Fork of VS1053 by
VS1053.h
00001 /** 00002 * ==================================================== Dec 21 2013, kayeks == 00003 * VS1053.cpp 00004 * =========================================================================== 00005 * Just a simple library for VLSI's mp3/midi codec chip 00006 * - Minimal and simple implementation (and dirty too) 00007 * 00008 * Modified on 05 September 2015 by Vassilis Serasidis. 00009 * - Added a patch for playing MP3 files on some "LC Technology" VS1053 boards. 00010 * 00011 * 00012 */ 00013 00014 #ifndef KAYX_VS1053_H_ 00015 #define KAYX_VS1053_H_ 00016 00017 /** Class VS1053. Drives VLSI's mp3/midi codec chip. */ 00018 class VS1053 { 00019 private: 00020 SPI spi; 00021 DigitalOut cs; 00022 DigitalOut bsync; 00023 DigitalIn dreq; 00024 DigitalOut rst; 00025 00026 public: 00027 static const uint8_t SCI_MODE = 0x00; 00028 static const uint8_t SCI_STATUS = 0x01; 00029 static const uint8_t SCI_BASS = 0x02; 00030 static const uint8_t SCI_CLOCKF = 0x03; 00031 static const uint8_t SCI_DECODE_TIME = 0x04; 00032 static const uint8_t SCI_AUDATA = 0x05; 00033 static const uint8_t SCI_WRAM = 0x06; 00034 static const uint8_t SCI_WRAMADDR = 0x07; 00035 static const uint8_t SCI_HDAT0 = 0x08; 00036 static const uint8_t SCI_HDAT1 = 0x09; 00037 static const uint8_t SCI_AIADDR = 0x0a; 00038 static const uint8_t SCI_VOL = 0x0b; 00039 static const uint8_t SCI_AICTRL0 = 0x0c; 00040 static const uint8_t SCI_AICTRL1 = 0x0d; 00041 static const uint8_t SCI_AICTRL2 = 0x0e; 00042 static const uint8_t SCI_AICTRL3 = 0x0f; 00043 00044 static const uint8_t SM_RESET = 2; 00045 static const uint8_t SM_SDINEW = 11; 00046 00047 VS1053(PinName mosiPin, PinName misoPin, PinName sckPin, 00048 PinName csPin, PinName bsyncPin, PinName dreqPin, PinName rstPin, 00049 uint32_t spiFrequency=1000000); 00050 ~VS1053(); 00051 void hardwareReset(); 00052 void modeSwitch(void); 00053 void sendDataByte(char data); 00054 size_t sendDataBlock(char* data, size_t length); 00055 void clockUp(); 00056 bool sendCancel(); 00057 bool stop(); 00058 00059 private: 00060 void writeReg(uint8_t, uint16_t); 00061 uint16_t readReg(uint8_t); 00062 }; 00063 00064 #endif
Generated on Sun Jul 17 2022 11:40:55 by
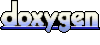