
Simple demo for the Raio RA8875 Based Display with a 4-wire SPI interface.
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 // 00002 // Demo Program 00003 // mbed version Graphics Working PrintScreen Working? "/local/file.bmp" 00004 // v148 Yes No, FILE *Image = fopen(Name_BMP, "wb"); fails 00005 // v142 Yes No, FILE *Image = fopen(Name_BMP, "wb"); fails 00006 // v140 Yes No, FILE *Image = fopen(Name_BMP, "wb"); fails 00007 // v138 Yes No, FILE *Image = fopen(Name_BMP, "wb"); fails 00008 // v137 Yes Yes 00009 // v136 Yes Yes 00010 // v128 Yes Yes 00011 // 00012 #include "mbed.h" // testing: v147 - working: v146, v145, v142, v136, v128, fails: v148 00013 #include "RA8875.h" // v138 - tested working 00014 #include "MyFont18x32.h" 00015 #include "BPG_Arial08x08.h" 00016 #include "BPG_Arial10x10.h" 00017 #include "BPG_Arial20x20.h" 00018 #include "BPG_Arial31x32.h" 00019 #include "BPG_Arial63x63.h" 00020 00021 // These two defines can be enabled, or commented out 00022 //#define BIG_SCREEN 00023 //#define CAP_TOUCH 00024 #define LCD_C 16 // color - bits per pixel 00025 00026 #ifdef CAP_TOUCH 00027 RA8875 lcd(p5,p6,p7,p12,NC, p9,p10,p13, "tft"); // MOSI,MISO,SCK,/ChipSelect,/reset, SDA,SCL,/IRQ, name 00028 #else 00029 RA8875 lcd(p5,p6,p7,p12,NC, "tft"); //MOSI, MISO, SCK, /ChipSelect, /reset, name 00030 #endif 00031 LocalFileSystem local("local"); // access to calibration file for resistive touch and printscreen 00032 00033 #ifdef BIG_SCREEN 00034 #define LCD_W 800 00035 #define LCD_H 480 00036 #else 00037 #define LCD_W 480 00038 #define LCD_H 272 00039 #endif 00040 00041 Serial pc(USBTX, USBRX); // And a little feedback 00042 Timer measurement; 00043 00044 RetCode_t callback(RA8875::filecmd_t cmd, uint8_t * buffer, uint16_t size) 00045 { 00046 static FILE * fh = NULL; 00047 static unsigned int bytesDone = 0; 00048 static unsigned int totalBytes = 0; 00049 00050 switch(cmd) { 00051 case RA8875::OPEN: 00052 bytesDone = 0; 00053 totalBytes = *(uint32_t *)buffer; 00054 pc.printf("PrintScreen callback to write %u bytes\r\n", totalBytes); 00055 fh = fopen("/local/file.bmp", "w+b"); 00056 if (!fh) 00057 return(file_not_found); 00058 break; 00059 case RA8875::WRITE: 00060 bytesDone += size; 00061 pc.printf(" Write %d bytes => %d of %d\r\n", size, bytesDone, totalBytes); 00062 fwrite(buffer, 1, size, fh); 00063 pc.printf(" %3d %% complete\r\n", ((100 * bytesDone)/totalBytes)); 00064 break; 00065 case RA8875::CLOSE: 00066 fclose(fh); 00067 pc.printf("PrintScreen Closed.\r\n"); 00068 break; 00069 default: 00070 pc.printf("Unexpected callback %d\r\n", cmd); 00071 break; 00072 } 00073 return noerror; 00074 } 00075 00076 00077 int main() 00078 { 00079 pc.baud(460800); // I like a snappy terminal, so crank it up! 00080 pc.printf("\r\nRA8875 Soft Fonts - Build " __DATE__ " " __TIME__ "\r\n"); 00081 00082 measurement.start(); 00083 lcd.frequency(500000); 00084 lcd.Reset(); 00085 lcd.init(LCD_W, LCD_H, LCD_C, 40); 00086 00087 // ************************** 00088 //RunTestSet(lcd, pc); // If the library was compiled for test mode... 00089 00090 lcd.foreground(RGB(255,255,0)); 00091 lcd.puts(0,100, "RA8875 Soft Fonts - Build " __DATE__ " " __TIME__ "\r\n"); 00092 00093 lcd.SelectUserFont(Dave_Smart18x32); 00094 lcd.puts("**** ! Soft Fonts ! **** 0123456789\r\n"); 00095 lcd.puts("ABCDEFGHIJKLMNOPQRSTUVWXYZ\r\n"); 00096 //lcd.puts("abcdefghijklmnopqrstuvwxyz\r\n"); 00097 lcd.SelectUserFont(); 00098 lcd.puts("Back to normal\r\n"); 00099 lcd.SelectUserFont(BPG_Arial08x08); 00100 lcd.puts("BPG_Arial08x08 ABCDEFGHIJKLMNOPQRSTUVWXYZ\r\n"); 00101 //lcd.puts("BPG_Arial08x08 abcdefghijklmnopqrstuvwxyz\r\n"); 00102 lcd.SelectUserFont(BPG_Arial10x10); 00103 lcd.puts("BPG_Arial10x10 ABCDEFGHIJKLMNOPQRSTUVWXYZ\r\n"); 00104 //lcd.puts("BPG_Arial10x10 abcdefghijklmnopqrstuvwxyz\r\n"); 00105 lcd.SelectUserFont(BPG_Arial20x20); 00106 lcd.puts("BPG_Arial20x20 "); 00107 lcd.SelectUserFont(BPG_Arial31x32); 00108 lcd.puts("BPG_Arial31x32\r\n"); 00109 lcd.SelectUserFont(BPG_Arial63x63); 00110 lcd.puts("BPG_Arial63x63"); 00111 pc.printf("Time trial completed in %d uSec\r\n", measurement.read_us()); 00112 00113 lcd.rect(0,0, 10,10, BrightRed); 00114 00115 pc.printf("PrintScreen activated ...\r\n"); 00116 RetCode_t r = lcd.PrintScreen(0,0,LCD_W,LCD_H,"/local/file.bmp", 8); 00117 pc.printf(" PrintScreen returned %d - %s\r\n", r, lcd.GetErrorMessage(r)); 00118 00119 //lcd.AttachPrintHandler(callback); 00120 //lcd.PrintScreen(0,0,LCD_W,LCD_H,8); 00121 00122 00123 while(1) { 00124 ; // end 00125 } 00126 }
Generated on Tue Jul 12 2022 18:27:56 by
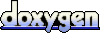