Library to control a Graphics TFT connected to 4-wire SPI - revised for the Raio RA8875 Display Controller.
Dependents: FRDM_RA8875_mPaint RA8875_Demo RA8875_KeyPadDemo SignalGenerator ... more
Fork of SPI_TFT by
TextDisplay.cpp
00001 // mbed TextDisplay Display Library Base Class 00002 // Copyright © 2007-2009 sford 00003 // Released under the MIT License: http://mbed.org/license/mit 00004 00005 #include "TextDisplay.h" 00006 00007 //#define DEBUG "Text" 00008 // ... 00009 // INFO("Stuff to show %d", var); // new-line is automatically appended 00010 // 00011 #if (defined(DEBUG) && !defined(TARGET_LPC11U24)) 00012 #define INFO(x, ...) std::printf("[INF %s %3d] " x "\r\n", DEBUG, __LINE__, ##__VA_ARGS__); 00013 #define WARN(x, ...) std::printf("[WRN %s %3d] " x "\r\n", DEBUG, __LINE__, ##__VA_ARGS__); 00014 #define ERR(x, ...) std::printf("[ERR %s %3d] " x "\r\n", DEBUG, __LINE__, ##__VA_ARGS__); 00015 #else 00016 #define INFO(x, ...) 00017 #define WARN(x, ...) 00018 #define ERR(x, ...) 00019 #endif 00020 00021 TextDisplay::TextDisplay(const char *name) : Stream(name) 00022 { 00023 _row = 0; 00024 _column = 0; 00025 _foreground = 0; 00026 _background = 0; 00027 if (name == NULL) { 00028 _path = NULL; 00029 } else { 00030 int len = strlen(name) + 2; 00031 _path = new char[len]; 00032 snprintf(_path, len, "/%s", name); 00033 } 00034 } 00035 00036 TextDisplay::~TextDisplay() 00037 { 00038 delete [] _path; 00039 } 00040 00041 int TextDisplay::_putc(int value) 00042 { 00043 INFO("_putc(%d)", value); 00044 if(value == '\n') { 00045 _column = 0; 00046 _row++; 00047 if(_row >= rows()) { 00048 _row = 0; 00049 } 00050 } else { 00051 character(_column, _row, value); 00052 _column++; 00053 if(_column >= columns()) { 00054 _column = 0; 00055 _row++; 00056 if(_row >= rows()) { 00057 _row = 0; 00058 } 00059 } 00060 } 00061 return value; 00062 } 00063 00064 // crude cls implementation, should generally be overwritten in derived class 00065 RetCode_t TextDisplay::cls(uint16_t layers) 00066 { 00067 INFO("cls()"); 00068 locate(0, 0); 00069 for(int i=0; i<columns()*rows(); i++) { 00070 putc(' '); 00071 } 00072 return noerror; 00073 } 00074 00075 point_t TextDisplay::locate(textloc_t column, textloc_t row) 00076 { 00077 point_t oldPt; 00078 00079 oldPt.x = _column; oldPt.y = _row; 00080 INFO("locate(%d,%d)", column, row); 00081 _column = column; 00082 _row = row; 00083 return oldPt; 00084 } 00085 00086 int TextDisplay::_getc() 00087 { 00088 return -1; 00089 } 00090 00091 color_t TextDisplay::foreground(uint16_t color) 00092 { 00093 color_t oldColor = _foreground; 00094 //INFO("foreground(%4X)", color); 00095 _foreground = color; 00096 return oldColor; 00097 } 00098 00099 color_t TextDisplay::background(uint16_t color) 00100 { 00101 color_t oldColor = _background; 00102 //INFO("background(%4X)", color); 00103 _background = color; 00104 return oldColor; 00105 } 00106 00107 bool TextDisplay::claim(FILE *stream) 00108 { 00109 if ( _path == NULL) { 00110 fprintf(stderr, "claim requires a name to be given in the instantiator of the TextDisplay instance!\r\n"); 00111 return false; 00112 } 00113 if (freopen(_path, "w", stream) == NULL) { 00114 return false; // Failed, should not happen 00115 } 00116 // make sure we use line buffering 00117 setvbuf(stdout, NULL, _IOLBF, columns()); 00118 return true; 00119 } 00120
Generated on Tue Jul 12 2022 17:28:36 by
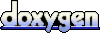