Library to control a Graphics TFT connected to 4-wire SPI - revised for the Raio RA8875 Display Controller.
Dependents: FRDM_RA8875_mPaint RA8875_Demo RA8875_KeyPadDemo SignalGenerator ... more
Fork of SPI_TFT by
RA8875_Touch_FT5206.cpp
00001 00002 00003 #include "RA8875.h" 00004 00005 //#define DEBUG "RAFT" // RA8875 FT5206 00006 // ... 00007 // INFO("Stuff to show %d", var); // new-line is automatically appended 00008 // 00009 #if (defined(DEBUG) && !defined(TARGET_LPC11U24)) 00010 #define INFO(x, ...) std::printf("[INF %s %4d] " x "\r\n", DEBUG, __LINE__, ##__VA_ARGS__); 00011 #define WARN(x, ...) std::printf("[WRN %s %4d] " x "\r\n", DEBUG, __LINE__, ##__VA_ARGS__); 00012 #define ERR(x, ...) std::printf("[ERR %s %4d] " x "\r\n", DEBUG, __LINE__, ##__VA_ARGS__); 00013 static void HexDump(const char * title, const uint8_t * p, int count) 00014 { 00015 int i; 00016 char buf[100] = "0000: "; 00017 00018 if (*title) 00019 INFO("%s", title); 00020 for (i=0; i<count; ) { 00021 sprintf(buf + strlen(buf), "%02X ", *(p+i)); 00022 if ((++i & 0x0F) == 0x00) { 00023 INFO("%s", buf); 00024 if (i < count) 00025 sprintf(buf, "%04X: ", i); 00026 else 00027 buf[0] = '\0'; 00028 } 00029 } 00030 if (strlen(buf)) 00031 INFO("%s", buf); 00032 } 00033 #else 00034 #define INFO(x, ...) 00035 #define WARN(x, ...) 00036 #define ERR(x, ...) 00037 #define HexDump(a, b, c) 00038 #endif 00039 00040 00041 // Translate from FT5206 Event Flag to Touch Code to API-match the 00042 // alternate resistive touch screen driver common in the RA8875 00043 // displays. 00044 static const TouchCode_t FT5206_EventFlagToTouchCode[4] = { 00045 touch, // 00b Put Down 00046 release, // 01b Put Up 00047 held, // 10b Contact 00048 no_touch // 11b Reserved 00049 }; 00050 00051 00052 RetCode_t RA8875::FT5206_Init() { 00053 const char data[] = {FT5206_DEVICE_MODE, 0}; 00054 //const char data[] = {0xC3, 0x55, 0x11, 0x33, 0xAA}; 00055 00056 #ifdef DEBUG 00057 int count = 0; 00058 for (int address=0; address<256; address+=2) { 00059 if (!m_i2c->write(address, NULL, 0)) { // 0 returned is ok 00060 INFO("I2C address 0x%02X", address); 00061 count++; 00062 } 00063 } 00064 INFO("%d devices found", count); 00065 #endif 00066 00067 00068 INFO("FT5206_Init: Addr %02X", m_addr); 00069 HexDump("FT5206 Init", (uint8_t *)data, sizeof(data)/sizeof(data[0])); 00070 int err = m_i2c->write(m_addr, data, sizeof(data)/sizeof(data[0]), true); 00071 if (err) { 00072 ERR(" result: %d", err); 00073 } 00074 return noerror; 00075 } 00076 00077 uint8_t RA8875::FT5206_TouchPositions(void) { 00078 uint8_t valXH; 00079 uint8_t valYH; 00080 00081 INFO("FT5206_TouchPositions()"); 00082 numberOfTouchPoints = FT5206_ReadRegU8(FT5206_TD_STATUS) & 0xF; 00083 INFO(" numOfTouchPoints %d", numberOfTouchPoints); 00084 gesture = FT5206_ReadRegU8(FT5206_GEST_ID); 00085 INFO(" gesture %d", gesture); 00086 00087 00088 valXH = FT5206_ReadRegU8(FT5206_TOUCH5_XH); 00089 valYH = FT5206_ReadRegU8(FT5206_TOUCH5_YH); 00090 touchInfo[4].touchCode = FT5206_EventFlagToTouchCode[valXH >> 6]; 00091 INFO(" touchCode %d", touchInfo[4].touchCode); 00092 touchInfo[4].touchID = (valYH >> 4); 00093 touchInfo[4].coordinates = TranslateOrientation( 00094 (valXH & 0x0f)*256 + FT5206_ReadRegU8(FT5206_TOUCH5_XL), 00095 (valYH & 0x0f)*256 + FT5206_ReadRegU8(FT5206_TOUCH5_YL) ); 00096 00097 00098 valXH = FT5206_ReadRegU8(FT5206_TOUCH4_XH); 00099 valYH = FT5206_ReadRegU8(FT5206_TOUCH4_YH); 00100 touchInfo[3].touchCode = FT5206_EventFlagToTouchCode[valXH >> 6]; 00101 INFO(" touchCode %d", touchInfo[3].touchCode); 00102 touchInfo[3].touchID = (valYH >> 4); 00103 touchInfo[3].coordinates = TranslateOrientation( 00104 (valXH & 0x0f)*256 + FT5206_ReadRegU8(FT5206_TOUCH4_XL), 00105 (valYH & 0x0f)*256 + FT5206_ReadRegU8(FT5206_TOUCH4_YL) ); 00106 00107 00108 valXH = FT5206_ReadRegU8(FT5206_TOUCH3_XH); 00109 valYH = FT5206_ReadRegU8(FT5206_TOUCH3_YH); 00110 touchInfo[2].touchCode = FT5206_EventFlagToTouchCode[valXH >> 6]; 00111 INFO(" touchCode %d", touchInfo[2].touchCode); 00112 touchInfo[2].touchID = (valYH >> 4); 00113 touchInfo[2].coordinates = TranslateOrientation( 00114 (valXH & 0x0f)*256 + FT5206_ReadRegU8(FT5206_TOUCH3_XL), 00115 (valYH & 0x0f)*256 + FT5206_ReadRegU8(FT5206_TOUCH3_YL) ); 00116 00117 00118 valXH = FT5206_ReadRegU8(FT5206_TOUCH2_XH); 00119 valYH = FT5206_ReadRegU8(FT5206_TOUCH2_YH); 00120 touchInfo[1].touchCode = FT5206_EventFlagToTouchCode[valXH >> 6]; 00121 INFO(" touchCode %d", touchInfo[1].touchCode); 00122 touchInfo[1].touchID = (valYH >> 4); 00123 touchInfo[1].coordinates = TranslateOrientation( 00124 (valXH & 0x0f)*256 + FT5206_ReadRegU8(FT5206_TOUCH2_XL), 00125 (valYH & 0x0f)*256 + FT5206_ReadRegU8(FT5206_TOUCH2_YL) ); 00126 00127 00128 valXH = FT5206_ReadRegU8(FT5206_TOUCH1_XH); 00129 valYH = FT5206_ReadRegU8(FT5206_TOUCH1_YH); 00130 touchInfo[0].touchCode = FT5206_EventFlagToTouchCode[valXH >> 6]; 00131 INFO(" touchCode %d", touchInfo[0].touchCode); 00132 touchInfo[0].touchID = (valYH >> 4); 00133 touchInfo[0].coordinates = TranslateOrientation( 00134 (valXH & 0x0f)*256 + FT5206_ReadRegU8(FT5206_TOUCH1_XL), 00135 (valYH & 0x0f)*256 + FT5206_ReadRegU8(FT5206_TOUCH1_YL) ); 00136 00137 return numberOfTouchPoints; 00138 }
Generated on Tue Jul 12 2022 17:28:36 by
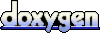