Library to control a Graphics TFT connected to 4-wire SPI - revised for the Raio RA8875 Display Controller.
Dependents: FRDM_RA8875_mPaint RA8875_Demo RA8875_KeyPadDemo SignalGenerator ... more
Fork of SPI_TFT by
GraphicsDisplayJPEG.h
00001 /// @page TinyJPEGDecompressor_Copyright Tiny JPEG Decompressor 00002 /// 00003 /// TJpgDec - Tiny JPEG Decompressor R0.01b (C)ChaN, 2012 00004 ///-----------------------------------------------------------------------------/ 00005 /// The TJpgDec is a generic JPEG decompressor module for tiny embedded systems. 00006 /// This is a free software that opened for education, research and commercial 00007 /// developments under license policy of following terms. 00008 /// 00009 /// @copyright © 2012, ChaN, all right reserved. 00010 /// 00011 /// * The TJpgDec module is a free software and there is NO WARRANTY. 00012 /// * No restriction on use. You can use, modify and redistribute it for 00013 /// personal, non-profit or commercial products UNDER YOUR RESPONSIBILITY. 00014 /// * Redistributions of source code must retain the above copyright notice. 00015 /// 00016 ///-----------------------------------------------------------------------------/ 00017 /// Oct 04,'11 R0.01 First release. 00018 /// Feb 19,'12 R0.01a Fixed decompression fails when scan starts with an escape seq. 00019 /// Sep 03,'12 R0.01b Added JD_TBLCLIP option. 00020 ///----------------------------------------------------------------------------*/ 00021 00022 #ifndef GraphicsDisplayJPEG_H 00023 #define GraphicsDisplayJPEG_H 00024 00025 /*----------------------------------------------------------------------------/ 00026 / TJpgDec - Tiny JPEG Decompressor include file (C)ChaN, 2012 00027 /----------------------------------------------------------------------------*/ 00028 #ifndef _TJPGDEC 00029 #define _TJPGDEC 00030 00031 /*---------------------------------------------------------------------------*/ 00032 /* System Configurations */ 00033 00034 #define JD_SZBUF 512 /* Size of stream input buffer */ 00035 #define JD_FORMAT 1 /* Output pixel format 0:RGB888 (3 BYTE/pix), 1:RGB565 (1 WORD/pix) */ 00036 #define JD_USE_SCALE 1 /* Use descaling feature for output */ 00037 #define JD_TBLCLIP 1 /* Use table for saturation (might be a bit faster but increases 1K bytes of code size) */ 00038 00039 /*---------------------------------------------------------------------------*/ 00040 00041 #include "DisplayDefs.h" 00042 00043 /// Error code results for the jpeg engine 00044 typedef enum { 00045 JDR_OK = noerror, ///< 0: Succeeded 00046 JDR_INTR = external_abort, ///< 1: Interrupted by output function 00047 JDR_INP = bad_parameter, ///< 2: Device error or wrong termination of input stream 00048 JDR_MEM1 = not_enough_ram, ///< 3: Insufficient memory pool for the image 00049 JDR_MEM2 = not_enough_ram, ///< 4: Insufficient stream input buffer 00050 JDR_PAR = bad_parameter, ///< 5: Parameter error 00051 JDR_FMT1 = not_supported_format, ///< 6: Data format error (may be damaged data) 00052 JDR_FMT2 = not_supported_format, ///< 7: Right format but not supported 00053 JDR_FMT3 = not_supported_format ///< 8: Not supported JPEG standard 00054 } JRESULT; 00055 00056 00057 00058 /// Rectangular structure definition for the jpeg engine 00059 typedef struct { 00060 loc_t left; ///< left coord 00061 loc_t right; ///< right coord 00062 loc_t top; ///< top coord 00063 loc_t bottom; ///< bottom coord 00064 } JRECT; 00065 00066 00067 /// Decompressor object structure for the jpeg engine 00068 typedef struct JDEC JDEC; 00069 00070 /// Internal structure for the jpeg engine 00071 struct JDEC { 00072 uint16_t dctr; ///< Number of bytes available in the input buffer 00073 uint8_t * dptr; ///< Current data read ptr 00074 uint8_t * inbuf; ///< Bit stream input buffer 00075 uint8_t dmsk; ///< Current bit in the current read byte 00076 uint8_t scale; ///< Output scaling ratio 00077 uint8_t msx; ///< MCU size in unit of block (width, ...) 00078 uint8_t msy; ///< MCU size in unit of block (..., height) 00079 uint8_t qtid[3]; ///< Quantization table ID of each component 00080 int16_t dcv[3]; ///< Previous DC element of each component 00081 uint16_t nrst; ///< Restart inverval 00082 uint16_t width; ///< Size of the input image (pixel width, ...) 00083 uint16_t height; ///< Size of the input image (..., pixel height) 00084 uint8_t * huffbits[2][2]; ///< Huffman bit distribution tables [id][dcac] 00085 uint16_t * huffcode[2][2]; ///< Huffman code word tables [id][dcac] 00086 uint8_t * huffdata[2][2]; ///< Huffman decoded data tables [id][dcac] 00087 int32_t * qttbl[4]; ///< Dequaitizer tables [id] 00088 void * workbuf; ///< Working buffer for IDCT and RGB output 00089 uint8_t * mcubuf; ///< Working buffer for the MCU 00090 void * pool; ///< Pointer to available memory pool 00091 uint16_t sz_pool; ///< Size of momory pool (bytes available) 00092 uint16_t (*infunc)(JDEC * jd, uint8_t * buffer, uint16_t bufsize); ///< Pointer to jpeg stream input function 00093 void * device; ///< Pointer to I/O device identifiler for the session 00094 }; 00095 00096 00097 #endif /* _TJPGDEC */ 00098 00099 #endif // GraphicsDisplayJPEG_H
Generated on Tue Jul 12 2022 17:28:36 by
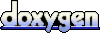