Library to control a Graphics TFT connected to 4-wire SPI - revised for the Raio RA8875 Display Controller.
Dependents: FRDM_RA8875_mPaint RA8875_Demo RA8875_KeyPadDemo SignalGenerator ... more
Fork of SPI_TFT by
FontMods.h
00001 // This file contains a perl script used to modify the mikroe generated fonts. 00002 // 00003 // Do not "include" this file into your project - just copy everything from 00004 // the #if to the #endif and put it into a .pl file on your local PC to 00005 // run it (this script requires that you have Perl installed). 00006 #if 0 00007 00008 # ParseMikroeFont.pl 00009 # 00010 # Parse and Modify Mikroe Font File and make a few strategic changes 00011 # 00012 # Copyright (c) 2019 by Smartware Computing, all rights reserved. 00013 # 00014 use strict; 00015 use warnings; 00016 00017 use POSIX; 00018 00019 my @HelpInfo = ( 00020 " ", 00021 "This script modifies a font file which was generated with a tool by ", 00022 "MikroElektronika - GLD Font Creator. ", 00023 " ", 00024 "That tool creates the font data set for an embedded system from a Windows ", 00025 "True Type font. The user is encouraged to ensure that the font used is ", 00026 "properly licensed, or drawn from a source that does not have a license ", 00027 "restriction. ", 00028 " ", 00029 "This script will read and then modify the file for a few specific purposes:", 00030 " * <space> character is redefined to set the width to 1/4 the height, ", 00031 " because the normal behavior sets it much too narrow. ", 00032 " * '0' - '9' characters are redefined to set the width equal to the width ", 00033 " of the widest digit, or to the user override value. ", 00034 " ", 00035 "And just because it can, it then improves upon the documentation in the ", 00036 "resulting data structure. ", 00037 " ", 00038 "This script was created by Smartware Computing, and is provided 'as is' ", 00039 "with no warranty or suitability of fitness for any purpose. Anyone may use ", 00040 "or modify it subject to the agreement that: ", 00041 " * The Smartware copyright statement remains intact. ", 00042 " * Modifications for derivative use are clearly stated in this header. ", 00043 " ", 00044 "Modifications from the original: ", 00045 " * none. ", 00046 " ", 00047 ); 00048 00049 00050 my $FixFile = 1; # Fix the file with planned changes 00051 my $Details = 1; # Emit more detailed information 00052 my $Debug = 0; # 0=None, 1=Some, 2=Detailed 00053 00054 my $DigitWidth = 0; # can be set on the command line, or it uses width('0') 00055 my $ff = ""; # FontFile 00056 my $of = ""; # Output File - otherwise stdout 00057 my $OH; # Handle to the output file 00058 00059 my $prg = $0; 00060 $prg =~ s/.*[\\\/]//; 00061 $prg =~ s/\.pl//i; 00062 my $args = join(" ", @ARGV); 00063 00064 if (!@ARGV) { 00065 ShowHelp(); 00066 exit; 00067 } 00068 foreach (@ARGV) { 00069 if (/-0=(\d+)/) { 00070 $DigitWidth = $1; 00071 } elsif (/-d=(\d+)/) { 00072 $Debug = $1; 00073 } elsif ($ff eq "") { 00074 $ff = $_; 00075 } elsif ($of eq "") { 00076 $of = $_; 00077 } else { 00078 ShowHelp(); 00079 exit; 00080 } 00081 } 00082 if (! -e $ff) { 00083 printf("Can't read $ff\n"); 00084 ShowHelp(); 00085 exit; 00086 } 00087 if (! -T $ff) { 00088 printf("File $ff isn't text.\n"); 00089 ShowHelp(); 00090 exit; 00091 } 00092 if ($of ne "") { 00093 open($OH, ">$of") || die("Can't write to $of"); 00094 select($OH); 00095 } 00096 00097 my @data = (); # Raw byte stream from the source file 00098 my @FileTop; 00099 my $FontDeclaration; 00100 my @FileBot; 00101 my %charData; # charData{$char}{width}, {$char}{data} 00102 00103 ImportFontFile(); 00104 DumpRawData() if ($Debug >= 2); 00105 00106 my $unk_0 = GetValueAt_Size_(0,1); 00107 my $unk_1 = GetValueAt_Size_(1,1); 00108 00109 my $firstChar = GetValueAt_Size_(2,2); 00110 my $lastChar = GetValueAt_Size_(4,2); 00111 my $fontHeight = GetValueAt_Size_(6,1); 00112 my $unk_2 = GetValueAt_Size_(7,1); 00113 00114 printf("// Char Range [%4X - %4X]\n", $firstChar, $lastChar) if ($Debug); 00115 00116 for (my $char = $firstChar; $char <= $lastChar; $char++) { 00117 my $offsetToChar = 8 + 4 * ($char - $firstChar); 00118 my $charWidth = GetValueAt_Size_($offsetToChar,1); 00119 my $charDataNdx = GetValueAt_Size_($offsetToChar + 1, 2); 00120 $charData{$char}{width} = $charWidth; 00121 my $count = (floor(($charWidth+7)/8)); 00122 @{$charData{$char}{data}} = GetByteArrayAt_Size_($charDataNdx, $count * $fontHeight); 00123 } 00124 00125 ShowFonts() if ($Debug >= 2); # Before Modifications 00126 FixChars() if ($FixFile); 00127 ShowFonts() if ($Debug); # After Modifications 00128 EmitFile(); 00129 if ($of ne "") { 00130 select(STDOUT); 00131 close($OH); 00132 } 00133 exit; 00134 00135 ######################################################################### 00136 00137 sub FixChars { 00138 my @newDat; 00139 my $char; 00140 my $charWidth; 00141 my $BytesWide; 00142 00143 # 00144 # * <space> character is redefined to set the width to 1/4 the height. 00145 # 00146 $char = 0x20; # Fix <space> 00147 $charWidth = floor($fontHeight/4); 00148 $charData{$char}{width} = $charWidth; 00149 $BytesWide = floor($charWidth/8); 00150 for (my $i=0; $i<($BytesWide*$fontHeight); $i++) { 00151 $charData{$char}{data}[$i] = 0x00; 00152 } 00153 00154 00155 # 00156 # * '0' - '9' characters are redefined to set the width equal to width('0') 00157 # or to the user override value. 00158 00159 # 00160 if ($DigitWidth > 0) { 00161 $charWidth = $DigitWidth; # User override option 00162 } else { 00163 $charWidth = DigitMaxWidth(); # Set it to the width of the widest digit 00164 } 00165 $BytesWide = floor(($charWidth+7)/8); 00166 #printf("Set Width = $charWidth, BytesWide = $BytesWide, Height = $fontHeight\n"); 00167 for ($char = 0x30; $char <= 0x39; $char++) { 00168 for (my $h=0; $h<$fontHeight; $h++) { 00169 for (my $w=0; $w<$BytesWide; $w++) { 00170 my $pDst = $h * $BytesWide + $w; 00171 if ($w < ceil($charData{$char}{width}/8)) { 00172 my $pSrc = $h * floor(($charData{$char}{width}+7)/8) + $w; 00173 $newDat[$pDst] = $charData{$char}{data}[$pSrc]; 00174 } else { 00175 $newDat[$pDst] = 0x00; 00176 } 00177 } 00178 } 00179 $charData{$char}{width} = $charWidth; 00180 for (my $i=0; $i<($fontHeight * $BytesWide); $i++) { 00181 $charData{$char}{data}[$i] = $newDat[$i]; 00182 } 00183 #RenderChar($char); 00184 #<stdin>; 00185 } 00186 } 00187 00188 sub DigitMaxWidth { 00189 my $max = 0; 00190 for (my $char=0x30; $char <= 0x39; $char++) { 00191 $max = $charData{$char}{width} if ($max < $charData{$char}{width}); 00192 } 00193 return $max; 00194 } 00195 00196 sub ImportFontFile { 00197 # 0 = scanning 00198 # 1 = after '{' 00199 # 2 = found '}' 00200 my $state = 0; 00201 00202 open(FH, "<$ff") || die("Can't open $ff"); 00203 while (<FH>) { 00204 my $rec = $_; 00205 chomp $rec; 00206 if ($state == 0) { 00207 if ($rec =~ /^const .*{/) { 00208 $FontDeclaration = $rec; 00209 $state = 1; 00210 } else { 00211 push @FileTop, $rec; 00212 } 00213 } elsif ($state == 1) { 00214 if ($rec =~ /};/) { 00215 $rec =~ s/^ +(.*)$/$1/ if ($Details); 00216 push @FileBot, $rec; 00217 $state = 2; 00218 } else { 00219 $rec =~ s/( +)/ /g; 00220 next if ($rec =~ /^ *$/); 00221 $rec =~ s# +//.*##; 00222 $rec =~ s/^ +(.*)$/$1/; 00223 $rec =~ s/^(.*),$/$1/; 00224 $rec =~ s/0x//g; 00225 push @data, split(",", $rec); 00226 } 00227 } elsif ($state == 2) { 00228 push @FileBot, $rec; 00229 } 00230 } 00231 close FH; 00232 } 00233 00234 00235 sub ShowHelp { 00236 print "\n\n$prg\n\n"; 00237 foreach (@HelpInfo) { 00238 print " $_\n"; 00239 } 00240 print <<EOM; 00241 $prg <MikroeFontFile> [Options] [<OptionalNewFile>] 00242 00243 Process the MikreFontFile, optionally generating a new file. 00244 00245 Options: 00246 -0=xx Set Digit '0' - '9' width to xx 00247 -d=x Set Debug Level 0=None, 1=Some, 2=More 00248 00249 EOM 00250 } 00251 00252 sub ShowFonts { 00253 for (my $char = $firstChar; $char <= $lastChar; $char++) { 00254 my $charWidth = $charData{$char}{width}; 00255 printf("\n// === %d (0x%2X) === w:%d, h:%d\n", $char, $char, $charWidth, $fontHeight); 00256 RenderChar($char); 00257 } 00258 } 00259 00260 sub EmitFile { 00261 if ($Details) { 00262 foreach (@HelpInfo) { 00263 print "// $_\n"; 00264 } 00265 print "// Script Activation:\n"; 00266 printf("// %s %s\n", $prg, $args); 00267 print "\n"; 00268 } 00269 00270 print join("\n", @FileTop) . "\n"; # Mikroe header 00271 printf("%s\n", $FontDeclaration); 00272 printf(" // Font Info\n") if ($Details); 00273 printf(" 0x%02X, // Unknown #1\n", $unk_0); 00274 printf(" 0x%02X, // Unknown #2\n", $unk_1); 00275 printf(" %s, // FirstChar\n", HexStream($firstChar,2)); 00276 printf(" %s, // LastChar\n", HexStream($lastChar,2)); 00277 printf(" %s, // FontHeight\n", HexStream($fontHeight,1)); 00278 printf(" 0x%02X, // Unknown #3\n", $unk_2); 00279 00280 00281 printf(" // Directory of Chars [Width] [Offset-L] [Offset-M] [Offset-H]\n") if ($Details); 00282 my $offsetToChar = 8 + 4 * ($lastChar - $firstChar + 1); 00283 for (my $char = $firstChar; $char <= $lastChar; $char++) { 00284 my $charWidth = $charData{$char}{width}; 00285 my $charByteCount = floor(($charWidth+7)/8) * $fontHeight; 00286 my $asc = ($char >= 0x20 && $char < 0x7F) ? chr($char) : "<non-printable>"; 00287 $asc = "\\\\" if ($char == 0x5C); 00288 my $details = ($Details) ? sprintf(" // 0x%02X '%s' ", $char, $asc) : ""; 00289 printf(" 0x%02X,%s,0x%02X,%s\n", 00290 $charWidth, HexStream($offsetToChar,2), 0, 00291 $details 00292 ); 00293 $offsetToChar += $charByteCount; 00294 } 00295 printf(" // Chars Bitstream\n") if ($Details); 00296 for (my $char = $firstChar; $char <= $lastChar; $char++) { 00297 my $charWidth = $charData{$char}{width}; 00298 my $charByteCount = floor(($charWidth+7)/8) * $fontHeight; 00299 my $asc = ($char >= 0x20 && $char < 0x7F) ? chr($char) : "<non-printable>"; 00300 $asc = "\\\\" if ($char == 0x5C); 00301 my $string = GetSomeHexBytes($char, 0, 8 * $charByteCount); 00302 printf(" %s%s // 0x%02X '%s'\n", 00303 $string, 00304 00305 ($char != $lastChar) ? "," : "", 00306 $char, $asc 00307 ); 00308 } 00309 print join("\n", @FileBot); 00310 } 00311 00312 sub DumpRawData { 00313 my $i; 00314 print "// "; 00315 for ($i=0; $i<=$#data; $i++) { 00316 printf("%02X ", hex($data[$i])); 00317 print "\n// " if ($i % 16 == 15); 00318 } 00319 print "\n"; 00320 } 00321 00322 00323 sub HexStream { 00324 my ($value, $len) = @_; 00325 my @parts; 00326 while ($len--) { 00327 push @parts, sprintf("0x%02X", $value & 0xFF); 00328 $value >>= 8; 00329 } 00330 return join(",", @parts); 00331 } 00332 00333 sub RenderChar { 00334 my ($char) = shift; 00335 my $h = $fontHeight; 00336 my $w = $charData{$char}{width}; 00337 00338 00339 PrintChar($char,$w,$h,@{$charData{$char}{data}}); 00340 } 00341 00342 sub PrintChar { 00343 my ($char,$w,$h,@datablock) = @_; 00344 00345 00346 printf("// +%s+ \n// ", '-' x $w); 00347 my $row = 0; 00348 my $boolStream = 0; 00349 while ($h--) { 00350 my $pixels = $w; 00351 my $bitmask = 0x01; 00352 my $rowStream = $boolStream; 00353 printf("%02X |", $row++); 00354 my $tail = ""; 00355 while ($pixels) { 00356 my $byte; 00357 $datablock[$rowStream] = 0 if (!defined($datablock[$rowStream])); 00358 $byte = $datablock[$rowStream]; 00359 printf("%s", ($byte & $bitmask) ? "*" : " "); 00360 $bitmask <<= 1; 00361 if ($pixels > 1 && ($bitmask & 0xFF) == 0) { 00362 $bitmask = 0x01; 00363 $rowStream++; 00364 } 00365 $pixels--; 00366 } 00367 printf("| %s\n// ", $tail); 00368 $boolStream += ($rowStream - $boolStream + 1); 00369 } 00370 printf(" +%s+\n", '-' x $w); 00371 } 00372 00373 00374 sub GetSomeHexBytes { 00375 my ($char, $offSet, $w) = @_; 00376 my @out; 00377 my $x = 0; 00378 00379 00380 $w = floor(($w+7)/8); 00381 while ($w--) { 00382 my $c = 0; 00383 $c = $charData{$char}{data}[$offSet + $x++] if (defined($charData{$char}{data}[$offSet + $x])); 00384 push @out, sprintf("0x%02X", $c); 00385 } 00386 return join(",", @out); 00387 } 00388 00389 sub GetSomeBytes { 00390 my ($char, $offSet, $w) = @_; 00391 my $string = ""; 00392 my $x = 0; 00393 00394 00395 $w = floor(($w+7)/8); 00396 while ($w--) { 00397 $string .= sprintf("%02X ", $charData{$char}{data}[$offSet + $x++]); 00398 } 00399 return $string; 00400 } 00401 00402 sub GetValueAt_Size_ { 00403 my ($offset, $size) = @_; 00404 my $value = 0; 00405 while ($size--) { 00406 $value = ($value << 8) | hex($data[$offset + $size]); 00407 } 00408 return $value; 00409 } 00410 00411 sub GetValueFromArray_At_Size_ { 00412 my ($offset, $size, $ary) = @_; 00413 my $value = 0; 00414 while ($size--) { 00415 $value = ($value << 8) | hex($$ary[$offset + $size]); 00416 } 00417 return $value; 00418 } 00419 00420 sub GetByteArrayAt_Size_ { 00421 my ($offset, $size) = @_; 00422 my @bytes; 00423 while ($size--) { 00424 push @bytes, hex($data[$offset++]); 00425 } 00426 return @bytes; 00427 } 00428 00429 sub GetByteSreamAt_Size_ { 00430 my ($offset, $size) = @_; 00431 my $value = ""; 00432 while ($size--) { 00433 $value .= sprintf("%02X ", hex($data[$offset++])); 00434 } 00435 return $value; 00436 } 00437 00438 00439 00440 #endif
Generated on Tue Jul 12 2022 17:28:36 by
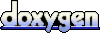