
This is a demo for the WiflyInterface Library.
Dependencies: WiflyInterface mbed
main.cpp
00001 #include "mbed.h" 00002 #include "RawSerial.h" 00003 00004 #include "WiflyInterface.h" 00005 00006 // Used later in hw adaptation 00007 #define MBED_APP_BOARD 1 /* http://mbed.org/components/mbed-Application-Board/ */ 00008 #define SMART_BOARD 2 /* http://mbed.org/users/WiredHome/notebook/SmartBoard-baseboard/ */ 00009 00010 // ######################################################################## 00011 #define HW_ADAPTER SMART_BOARD /* Which board are we compiling against? */ 00012 // ######################################################################## 00013 00014 00015 #define SSID "ssid" 00016 #define PASS "pass" 00017 00018 const char PROGINFO[] = "\r\n\r\nWifly Demo - built " __DATE__ " " __TIME__ "\r\n\r\n"; 00019 00020 char Message[] = "Hello World"; 00021 char BCASTADDR[] = "255.255.255.255"; 00022 int Port = 40000; 00023 00024 #if HW_ADAPTER == MBED_APP_BOARD 00025 WiflyInterface eth( p9, p10, p30, p29); 00026 #elif HW_ADAPTER == SMART_BOARD 00027 WiflyInterface eth(p28, p27, p23, p24); 00028 #endif 00029 00030 RawSerial pc(USBTX, USBRX); 00031 DigitalOut myled(LED1); 00032 00033 extern "C" void mbed_reset(); 00034 00035 void ShowIPAddress(bool show) 00036 { 00037 pc.printf("Ethernet connected as %s\r\n", show ? eth.getIPAddress() : "---.---.---.---"); 00038 } 00039 00040 void ShowMACAddress(bool show) 00041 { 00042 pc.printf("Ethernet MAC is %s\r\n", show ? eth.getMACAddress() : "--.--.--.--.--.--"); 00043 } 00044 00045 00046 int main() { 00047 pc.baud(460800); 00048 pc.printf(PROGINFO); 00049 pc.printf("Configuration for %s\r\n\r\n", (HW_ADAPTER == SMART_BOARD) ? "SmartBoard" : "mbed Application Board"); 00050 00051 eth.SetSecurity(SSID, PASS, WPA); 00052 pc.printf("***\r\n"); 00053 pc.printf("Initializing network interface...\r\n"); 00054 if (0 == eth.init()) { //Use DHCP 00055 eth.setName("TestWiFi_01"); 00056 do { 00057 pc.printf("Connecting to network...\r\n"); 00058 if (0 == eth.connect()) { 00059 ShowIPAddress(true); 00060 ShowMACAddress(true); 00061 int speed = eth.get_connection_speed(); 00062 pc.printf("Connected at %d Mb/s\r\n", speed); 00063 pc.printf("Wifly info: %s\r\n", eth.getWiflyVersionString()); 00064 00065 pc.printf("\r\n"); 00066 pc.printf(" ctrl-a to download Wifly firmward v4.41\r\n"); 00067 pc.printf(" ctrl-b to download Wifly firmward v4.75\r\n"); 00068 pc.printf(" ctrl-c to send a broadcast message\r\n"); 00069 while (eth.is_connected()) { 00070 if (eth.readable()) 00071 pc.putc(eth.getc()); 00072 else if (pc.readable()) { 00073 int c = pc.getc(); 00074 if (c == 0x01) { // ctrl-a // Install Software v4.41 00075 pc.printf("Trying to download wifly7-441.mif from microchip...\r\n"); 00076 if (eth.SWUpdateWifly("wifly7-441.mif")) { 00077 pc.printf(" Success - now rebooting to this Wifly image.\r\n"); 00078 wait_ms(100); 00079 mbed_reset(); 00080 } 00081 } else if (c == 0x02) { // ctrl-b // Install Software v4.75 00082 pc.printf("Trying to download wifly7-475.mif from microchip...\r\n"); 00083 if (eth.SWUpdateWifly("wifly7-475.mif")){ 00084 pc.printf(" Success - now rebooting to this Wifly image.\r\n"); 00085 wait_ms(100); 00086 mbed_reset(); 00087 } 00088 } else if (c == 0x03) { // ctrl-c 00089 UDPSocket bcast; 00090 Endpoint ep; 00091 pc.printf("Broadcast sending '%s' on port %d\r\n", Message, Port); 00092 int h = ep.set_address(BCASTADDR, Port); 00093 int i = bcast.bind(40000); 00094 int j = bcast.set_broadcasting(true); 00095 int k = bcast.sendTo(ep, Message, strlen(Message)); 00096 bcast.close(); 00097 pc.printf("done.\r\n"); 00098 } else { 00099 eth.putc(c); 00100 } 00101 } 00102 } 00103 00104 pc.printf("lost connection.\r\n"); 00105 ShowIPAddress(false); 00106 eth.disconnect(); 00107 } 00108 else { 00109 pc.printf(" ... failed to connect.\r\n"); 00110 } 00111 } 00112 while (1); 00113 } 00114 else { 00115 pc.printf(" ... failed to initialize, rebooting...\r\n"); 00116 mbed_reset(); 00117 } 00118 }
Generated on Wed Jul 13 2022 07:22:26 by
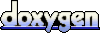