CANMessage is the primitive CAN message object. It supports creation, parsing, formatting of messages. Can be easily integrated with CANPort and CANQueue libraries.
CANmsg Class Reference
A super CAN message object, which has additional capabilities. More...
#include <CANMessage.h>
Public Member Functions | |
CANmsg () | |
Constructor for a CANmsg object, which is a superclass of CANMessage. | |
CANmsg (CANCHANNEL_T ch, CANDIR_T dir, CANMessage msg) | |
Constructor for a CANmsg object, which is a superclass of CANMessage. | |
CANmsg (char *p) | |
Constructor for a CANmsg object, which is a superclass of CANMessage. | |
~CANmsg () | |
Destructor for the CANmsg object. | |
bool | ParseCANMessage (char *p) |
Parse a text string into the CANmsg object, which is a superclass of CANMessage. | |
void | FormatCANMessage (char *buffer, int buflen) |
Formats this CAN message into a text buffer - which should be at least 70. | |
void | SetTimestamp () |
Overrides the timestamp in this message with the current time. | |
uint64_t | GetTimestamp () |
Gets the 64-bit timestamp of this message. | |
Data Fields | |
CANDIR_T | dir |
direction of this CAN message - rcv or xmt | |
CANCHANNEL_T | ch |
channel number of this CAN message |
Detailed Description
A super CAN message object, which has additional capabilities.
This object is derived from CANMessage, however it adds a timestamp and other useful methods.
the CANMessage format element by default is:
- CANStandard = 0,
- CANExtended = 1,
- CANAny = 2 But for certain purposes it will be convenient to use the CANmsg object, which inherits CANMessage, and overload this item with additional values.
- Error = 128
Definition at line 58 of file CANMessage.h.
Constructor & Destructor Documentation
CANmsg | ( | ) |
Constructor for a CANmsg object, which is a superclass of CANMessage.
The CANmsg object included additional information, including direction, channel number, timestamp, and the ability to format into ASCII
Definition at line 52 of file CANMessage.cpp.
CANmsg | ( | CANCHANNEL_T | ch, |
CANDIR_T | dir, | ||
CANMessage | msg | ||
) |
Constructor for a CANmsg object, which is a superclass of CANMessage.
The CANmsg object included additional information, including direction, channel number, timestamp, and the ability to format into ASCII.
- Parameters:
-
ch is the channel number (CH1, CH2) dir is the direction (xmt, rcv) msg is a CANMessage object from which to construct this object
Definition at line 60 of file CANMessage.cpp.
CANmsg | ( | char * | p ) |
Constructor for a CANmsg object, which is a superclass of CANMessage.
The CANmsg object included additional information, including direction, channel number, timestamp, and the ability to format into ASCII. This constructor creates a message from an ascii buffer with the standard message format in it.
/// t xtd 02 1CF00400 08 11 22 33 44 55 66 77 88 0 0 1234.567890 /// If the first letter is not 't' or 'r', transmit is assumed /// xtd 02 1CF00400 08 11 22 33 44 55 66 77 88 0 0 1234.567890 ///
- Parameters:
-
p is a pointer to a text string of the message contents, in the same format as what would be output with the FormatCANMessage method.
Definition at line 81 of file CANMessage.cpp.
~CANmsg | ( | ) |
Destructor for the CANmsg object.
Definition at line 89 of file CANMessage.cpp.
Member Function Documentation
void FormatCANMessage | ( | char * | buffer, |
int | buflen | ||
) |
Formats this CAN message into a text buffer - which should be at least 70.
/// t xtd 02 1CF00400 08 11 22 33 44 55 66 77 88 0 0 1234.567890 ///
- Parameters:
-
buffer is a pointer to the buffer to fill buflen is the size of the buffer (minimum 70 recommended)
- Returns:
- nothing
Definition at line 135 of file CANMessage.cpp.
uint64_t GetTimestamp | ( | ) |
Gets the 64-bit timestamp of this message.
- Returns:
- time in microseconds
Definition at line 148 of file CANMessage.h.
bool ParseCANMessage | ( | char * | p ) |
Parse a text string into the CANmsg object, which is a superclass of CANMessage.
The CANmsg object included additional information, including direction, channel number, timestamp, and the ability to format into ASCII. This constructor creates a message from an ascii buffer with the standard message format in it.
/// t xtd 02 1CF00400 08 11 22 33 44 55 66 77 88 0 0 1234.567890 /// If the first letter is not 't' or 'r', transmit is assumed /// xtd 02 1CF00400 08 11 22 33 44 55 66 77 88 0 0 1234.567890 ///
- Parameters:
-
p is a pointer to a text string of the message contents, in the same format as what would be output with the FormatCANMessage method.
- Returns:
- true
Definition at line 92 of file CANMessage.cpp.
void SetTimestamp | ( | ) |
Overrides the timestamp in this message with the current time.
Definition at line 129 of file CANMessage.cpp.
Field Documentation
channel number of this CAN message
Definition at line 154 of file CANMessage.h.
direction of this CAN message - rcv or xmt
Definition at line 148 of file CANMessage.h.
Generated on Sat Jul 16 2022 14:23:32 by
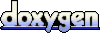