Debouncing library (not mine)
Dependents: ElecPneuShifter_4 tarea1 tarea_miercoles Rampa ... more
DebouncedIn.cpp
00001 #include "DebouncedIn.h" 00002 #include "mbed.h" 00003 00004 /* 00005 * Constructor 00006 */ 00007 DebouncedIn::DebouncedIn(PinName in) 00008 : _in(in) { 00009 00010 // reset all the flags and counters 00011 _samples = 0; 00012 _output = 0; 00013 _output_last = 0; 00014 _rising_flag = 0; 00015 _falling_flag = 0; 00016 _state_counter = 0; 00017 00018 // Attach ticker 00019 _ticker.attach(this, &DebouncedIn::_sample, 0.005); 00020 } 00021 00022 void DebouncedIn::_sample() { 00023 00024 // take a sample 00025 _samples = _samples >> 1; // shift left 00026 00027 if (_in) { 00028 _samples |= 0x80; 00029 } 00030 00031 // examine the sample window, look for steady state 00032 if (_samples == 0x00) { 00033 _output = 0; 00034 } 00035 else if (_samples == 0xFF) { 00036 _output = 1; 00037 } 00038 00039 00040 // Rising edge detection 00041 if ((_output == 1) && (_output_last == 0)) { 00042 _rising_flag++; 00043 _state_counter = 0; 00044 } 00045 00046 // Falling edge detection 00047 else if ((_output == 0) && (_output_last == 1)) { 00048 _falling_flag++; 00049 _state_counter = 0; 00050 } 00051 00052 // steady state 00053 else { 00054 _state_counter++; 00055 } 00056 00057 // update the output 00058 _output_last = _output; 00059 00060 } 00061 00062 00063 00064 // return number of rising edges 00065 int DebouncedIn::rising(void) { 00066 int return_value = _rising_flag; 00067 _rising_flag = 0; 00068 return(return_value); 00069 } 00070 00071 // return number of falling edges 00072 int DebouncedIn::falling(void) { 00073 int return_value = _falling_flag; 00074 _falling_flag = 0; 00075 return(return_value); 00076 } 00077 00078 // return number of ticsk we've bene steady for 00079 int DebouncedIn::steady(void) { 00080 return(_state_counter); 00081 } 00082 00083 // return the debounced status 00084 int DebouncedIn::read(void) { 00085 return(_output); 00086 } 00087 00088 // shorthand for read() 00089 DebouncedIn::operator int() { 00090 return read(); 00091 }
Generated on Wed Jul 13 2022 12:41:28 by
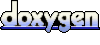