
Bumped Mbed FW version to 01.20.0080
Embed:
(wiki syntax)
Show/hide line numbers
ADMW1001_REGISTERS_typedefs.h
00001 /* ================================================================================ 00002 00003 Created by : 00004 Created on : 2020 Mar 12, 16:15 GMT Standard Time 00005 00006 Project : ADMW1001_REGISTERS 00007 File : ADMW1001_REGISTERS_typedefs.h 00008 Description : C Register Structures 00009 00010 !! ADI Confidential !! 00011 INTERNAL USE ONLY 00012 00013 Copyright (c) 2020 Analog Devices, Inc. All Rights Reserved. 00014 This software is proprietary and confidential to Analog Devices, Inc. and 00015 its licensors. 00016 00017 This file was auto-generated. Do not make local changes to this file. 00018 00019 Auto generation script information: 00020 Script: C:\Program Files (x86)\Yoda-19.05.01\generators\inc\genHeaders 00021 Last modified: 26-SEP-2017 00022 00023 ================================================================================ */ 00024 00025 #ifndef _ADMW1001_REGISTERS_TYPEDEFS_H 00026 #define _ADMW1001_REGISTERS_TYPEDEFS_H 00027 00028 /* pickup integer types */ 00029 #if defined(_LANGUAGE_C) || (defined(__GNUC__) && !defined(__ASSEMBLER__)) 00030 #include <stdint.h> 00031 #endif /* _LANGUAGE_C */ 00032 00033 #if defined ( __CC_ARM ) 00034 #pragma push 00035 #pragma anon_unions 00036 #endif 00037 00038 /** @defgroup Interface_Config_A Interface Configuration A (Interface_Config_A) Register 00039 * Interface Configuration A (Interface_Config_A) Register. 00040 * @{ 00041 */ 00042 00043 /* ========================================================================= 00044 *! \enum ADMW_SPI_Interface_Config_A_Addr_Ascension 00045 *! \brief Determines Sequential Addressing Behavior (Addr_Ascension) Enumerations 00046 * ========================================================================= */ 00047 typedef enum 00048 { 00049 SPI_INTERFACE_CONFIG_A_DESCEND = 0, /**< Address accessed is decremented by one for each data byte when streaming */ 00050 SPI_INTERFACE_CONFIG_A_ASCEND = 1 /**< Address accessed is incremented by one for each data byte when streaming */ 00051 } ADMW_SPI_Interface_Config_A_Addr_Ascension ; 00052 00053 00054 /* ========================================================================== 00055 *! \struct ADMW_SPI_Interface_Config_A_Struct 00056 *! \brief Interface Configuration A Register bit field structure 00057 * ========================================================================== */ 00058 typedef struct _ADMW_SPI_Interface_Config_A_t { 00059 union { 00060 struct { 00061 uint8_t SW_ResetX : 1; /**< Second of Two of the SW_RESET Bits. */ 00062 uint8_t reserved1 : 3; 00063 uint8_t SDO_Enable : 1; /**< Serial Data Output Pin Enable */ 00064 uint8_t Addr_Ascension : 1; /**< Determines Sequential Addressing Behavior */ 00065 uint8_t reserved6 : 1; 00066 uint8_t SW_Reset : 1; /**< First of Two of the SW_RESET Bits. */ 00067 }; 00068 uint8_t VALUE8; 00069 }; 00070 } ADMW_SPI_Interface_Config_A_t; 00071 00072 /*@}*/ 00073 00074 /** @defgroup Chip_Type Chip Type (Chip_Type) Register 00075 * Chip Type (Chip_Type) Register. 00076 * @{ 00077 */ 00078 00079 /* ========================================================================== 00080 *! \struct ADMW_SPI_Chip_Type_Struct 00081 *! \brief Chip Type Register bit field structure 00082 * ========================================================================== */ 00083 typedef struct _ADMW_SPI_Chip_Type_t { 00084 union { 00085 struct { 00086 uint8_t Chip_Type : 4; /**< Precision ADC */ 00087 uint8_t reserved4 : 4; 00088 }; 00089 uint8_t VALUE8; 00090 }; 00091 } ADMW_SPI_Chip_Type_t; 00092 00093 /*@}*/ 00094 00095 /** @defgroup Product_ID_L Product ID Low (Product_ID_L) Register 00096 * Product ID Low (Product_ID_L) Register. 00097 * @{ 00098 */ 00099 00100 /* ========================================================================== 00101 *! \struct ADMW_SPI_Product_ID_L_Struct 00102 *! \brief Product ID Low Register bit field structure 00103 * ========================================================================== */ 00104 typedef struct _ADMW_SPI_Product_ID_L_t { 00105 union { 00106 struct { 00107 uint8_t Product_ID : 8; /**< Product_ID[7:0] The Device Chip Type and Family */ 00108 }; 00109 uint8_t VALUE8; 00110 }; 00111 } ADMW_SPI_Product_ID_L_t; 00112 00113 /*@}*/ 00114 00115 /** @defgroup Product_ID_H Product ID High (Product_ID_H) Register 00116 * Product ID High (Product_ID_H) Register. 00117 * @{ 00118 */ 00119 00120 /* ========================================================================== 00121 *! \struct ADMW_SPI_Product_ID_H_Struct 00122 *! \brief Product ID High Register bit field structure 00123 * ========================================================================== */ 00124 typedef struct _ADMW_SPI_Product_ID_H_t { 00125 union { 00126 struct { 00127 uint8_t Product_ID : 8; /**< Product_ID[15:8] The Device Chip Type and Family */ 00128 }; 00129 uint8_t VALUE8; 00130 }; 00131 } ADMW_SPI_Product_ID_H_t; 00132 00133 /*@}*/ 00134 00135 /** @defgroup Scratch_Pad Scratch Pad (Scratch_Pad) Register 00136 * Scratch Pad (Scratch_Pad) Register. 00137 * @{ 00138 */ 00139 00140 /* ========================================================================== 00141 *! \struct ADMW_SPI_Scratch_Pad_Struct 00142 *! \brief Scratch Pad Register bit field structure 00143 * ========================================================================== */ 00144 typedef struct _ADMW_SPI_Scratch_Pad_t { 00145 union { 00146 struct { 00147 uint8_t Scratch_Value : 8; /**< Software Scratchpad */ 00148 }; 00149 uint8_t VALUE8; 00150 }; 00151 } ADMW_SPI_Scratch_Pad_t; 00152 00153 /*@}*/ 00154 00155 /** @defgroup SPI_Revision SPI Revision (SPI_Revision) Register 00156 * SPI Revision (SPI_Revision) Register. 00157 * @{ 00158 */ 00159 00160 /* ========================================================================= 00161 *! \enum ADMW_SPI_SPI_Revision_Version 00162 *! \brief SPI Version (Version) Enumerations 00163 * ========================================================================= */ 00164 typedef enum 00165 { 00166 SPI_SPI_REVISION_REV1_0 = 2 /**< Revision 1.0 */ 00167 } ADMW_SPI_SPI_Revision_Version ; 00168 00169 00170 /* ========================================================================= 00171 *! \enum ADMW_SPI_SPI_Revision_SPI_Type 00172 *! \brief Always Reads as 0x2 (SPI_Type) Enumerations 00173 * ========================================================================= */ 00174 typedef enum 00175 { 00176 SPI_SPI_REVISION_ADI_SPI = 0, /**< ADI_SPI */ 00177 SPI_SPI_REVISION_LPT_SPI = 2 /**< LPT_SPI */ 00178 } ADMW_SPI_SPI_Revision_SPI_Type ; 00179 00180 00181 /* ========================================================================== 00182 *! \struct ADMW_SPI_SPI_Revision_Struct 00183 *! \brief SPI Revision Register bit field structure 00184 * ========================================================================== */ 00185 typedef struct _ADMW_SPI_SPI_Revision_t { 00186 union { 00187 struct { 00188 uint8_t Version : 6; /**< SPI Version */ 00189 uint8_t SPI_Type : 2; /**< Always Reads as 0x2 */ 00190 }; 00191 uint8_t VALUE8; 00192 }; 00193 } ADMW_SPI_SPI_Revision_t; 00194 00195 /*@}*/ 00196 00197 /** @defgroup Vendor_L Vendor ID Low (Vendor_L) Register 00198 * Vendor ID Low (Vendor_L) Register. 00199 * @{ 00200 */ 00201 00202 /* ========================================================================== 00203 *! \struct ADMW_SPI_Vendor_L_Struct 00204 *! \brief Vendor ID Low Register bit field structure 00205 * ========================================================================== */ 00206 typedef struct _ADMW_SPI_Vendor_L_t { 00207 union { 00208 struct { 00209 uint8_t VID : 8; /**< VID[7:0] Analog Devices Vendor ID */ 00210 }; 00211 uint8_t VALUE8; 00212 }; 00213 } ADMW_SPI_Vendor_L_t; 00214 00215 /*@}*/ 00216 00217 /** @defgroup Vendor_H Vendor ID High (Vendor_H) Register 00218 * Vendor ID High (Vendor_H) Register. 00219 * @{ 00220 */ 00221 00222 /* ========================================================================== 00223 *! \struct ADMW_SPI_Vendor_H_Struct 00224 *! \brief Vendor ID High Register bit field structure 00225 * ========================================================================== */ 00226 typedef struct _ADMW_SPI_Vendor_H_t { 00227 union { 00228 struct { 00229 uint8_t VID : 8; /**< VID[15:8] Analog Devices Vendor ID */ 00230 }; 00231 uint8_t VALUE8; 00232 }; 00233 } ADMW_SPI_Vendor_H_t; 00234 00235 /*@}*/ 00236 00237 /** @defgroup Stream_Mode Stream Mode (Stream_Mode) Register 00238 * Stream Mode (Stream_Mode) Register. 00239 * @{ 00240 */ 00241 00242 /* ========================================================================== 00243 *! \struct ADMW_SPI_Stream_Mode_Struct 00244 *! \brief Stream Mode Register bit field structure 00245 * ========================================================================== */ 00246 typedef struct _ADMW_SPI_Stream_Mode_t { 00247 union { 00248 struct { 00249 uint8_t Loop_Count : 8; /**< Set the Data Byte Count Before Looping to Start Address */ 00250 }; 00251 uint8_t VALUE8; 00252 }; 00253 } ADMW_SPI_Stream_Mode_t; 00254 00255 /*@}*/ 00256 00257 /** @defgroup Interface_Status_A Interface Status A (Interface_Status_A) Register 00258 * Interface Status A (Interface_Status_A) Register. 00259 * @{ 00260 */ 00261 00262 /* ========================================================================== 00263 *! \struct ADMW_SPI_Interface_Status_A_Struct 00264 *! \brief Interface Status A Register bit field structure 00265 * ========================================================================== */ 00266 typedef struct _ADMW_SPI_Interface_Status_A_t { 00267 union { 00268 struct { 00269 uint8_t Address_Invalid_Error : 1; /**< Attempt to Read/Write Nonexistent Register Address */ 00270 uint8_t Register_Partial_Access_Error : 1; /**< Set When Fewer Than Expected Number of Bytes Read/Written */ 00271 uint8_t Wr_To_Rd_Only_Reg_Error : 1; /**< Write to Read Only Register Attempted */ 00272 uint8_t CRC_Error : 1; /**< Invalid/No CRC Received */ 00273 uint8_t Clock_Count_Error : 1; /**< Incorrect Number of Clocks Detected in a Transaction */ 00274 uint8_t reserved5 : 2; 00275 uint8_t Not_Ready_Error : 1; /**< Device Not Ready for Transaction */ 00276 }; 00277 uint8_t VALUE8; 00278 }; 00279 } ADMW_SPI_Interface_Status_A_t; 00280 00281 /*@}*/ 00282 00283 /** @defgroup Command Special Command Register (Command) Register 00284 * Special Command Register (Command) Register. 00285 * @{ 00286 */ 00287 00288 /* ========================================================================= 00289 *! \enum ADMW_CORE_Command_Special_Command 00290 *! \brief Special Command (Special_Command) Enumerations 00291 * ========================================================================= */ 00292 typedef enum 00293 { 00294 CORE_COMMAND_NOP = 0, /**< No command */ 00295 CORE_COMMAND_CONVERT = 1, /**< Start ADC conversions */ 00296 CORE_COMMAND_CONVERT_WITH_RAW = 2, /**< Start conversions with added raw ADC data */ 00297 CORE_COMMAND_LATCH_CONFIG = 7, /**< Latch configuration. */ 00298 CORE_COMMAND_LOAD_LUT = 8, /**< Load LUT from flash */ 00299 CORE_COMMAND_SAVE_LUT = 9, /**< Save LUT to flash */ 00300 CORE_COMMAND_LOAD_CONFIG_1 = 24, /**< Load registers with configuration from flash */ 00301 CORE_COMMAND_SAVE_CONFIG_1 = 25 /**< Store current registers to flash configuration */ 00302 } ADMW_CORE_Command_Special_Command ; 00303 00304 00305 /* ========================================================================== 00306 *! \struct ADMW_CORE_Command_Struct 00307 *! \brief Special Command Register bit field structure 00308 * ========================================================================== */ 00309 typedef struct _ADMW_CORE_Command_t { 00310 union { 00311 struct { 00312 uint8_t Special_Command : 8; /**< Special Command */ 00313 }; 00314 uint8_t VALUE8; 00315 }; 00316 } ADMW_CORE_Command_t; 00317 00318 /*@}*/ 00319 00320 /** @defgroup Mode Operating Mode and DRDY Control (Mode) Register 00321 * Operating Mode and DRDY Control (Mode) Register. 00322 * @{ 00323 */ 00324 00325 /* ========================================================================= 00326 *! \enum ADMW_CORE_Mode_Conversion_Mode 00327 *! \brief Conversion Mode (Conversion_Mode) Enumerations 00328 * ========================================================================= */ 00329 typedef enum 00330 { 00331 CORE_MODE_SINGLECYCLE = 0, /**< Single cycle conversion mode. A cycle is completed every time a convert command is issued */ 00332 CORE_MODE_RESERVED = 1, /**< Reserved for future use */ 00333 CORE_MODE_CONTINUOUS = 2 /**< Continuous conversion mode. A cycle is started repeatedly at time specified in cycle time */ 00334 } ADMW_CORE_Mode_Conversion_Mode ; 00335 00336 00337 /* ========================================================================= 00338 *! \enum ADMW_CORE_Mode_Drdy_Mode 00339 *! \brief Indicates Behavior of DRDY Pin (Drdy_Mode) Enumerations 00340 * ========================================================================= */ 00341 typedef enum 00342 { 00343 CORE_MODE_DRDY_PER_CONVERSION = 0, /**< Data ready per conversion */ 00344 CORE_MODE_DRDY_PER_CYCLE = 1, /**< Data ready per cycle */ 00345 CORE_MODE_DRDY_PER_FIFO_FILL = 2 /**< Data ready per FIFO fill */ 00346 } ADMW_CORE_Mode_Drdy_Mode ; 00347 00348 00349 /* ========================================================================== 00350 *! \struct ADMW_CORE_Mode_Struct 00351 *! \brief Operating Mode and DRDY Control Register bit field structure 00352 * ========================================================================== */ 00353 typedef struct _ADMW_CORE_Mode_t { 00354 union { 00355 struct { 00356 uint8_t Conversion_Mode : 2; /**< Conversion Mode */ 00357 uint8_t Drdy_Mode : 2; /**< Indicates Behavior of DRDY Pin */ 00358 uint8_t reserved4 : 4; 00359 }; 00360 uint8_t VALUE8; 00361 }; 00362 } ADMW_CORE_Mode_t; 00363 00364 /*@}*/ 00365 00366 /** @defgroup Power_Config Power Configuration (Power_Config) Register 00367 * Power Configuration (Power_Config) Register. 00368 * @{ 00369 */ 00370 00371 /* ========================================================================= 00372 *! \enum ADMW_CORE_Power_Config_Power_Mode_MCU 00373 *! \brief MCU Power Mode (Power_Mode_MCU) Enumerations 00374 * ========================================================================= */ 00375 typedef enum 00376 { 00377 CORE_POWER_CONFIG_ACTIVE_MODE = 0, /**< ADMW1001 is fully power up and ready to convert */ 00378 CORE_POWER_CONFIG_HIBERNATION = 1 /**< Lowest power mode. wakeup pin required to enter active mode. SPI powered down */ 00379 } ADMW_CORE_Power_Config_Power_Mode_MCU ; 00380 00381 00382 /* ========================================================================== 00383 *! \struct ADMW_CORE_Power_Config_Struct 00384 *! \brief Power Configuration Register bit field structure 00385 * ========================================================================== */ 00386 typedef struct _ADMW_CORE_Power_Config_t { 00387 union { 00388 struct { 00389 uint8_t Power_Mode_MCU : 1; /**< MCU Power Mode */ 00390 uint8_t reserved1 : 7; 00391 }; 00392 uint8_t VALUE8; 00393 }; 00394 } ADMW_CORE_Power_Config_t; 00395 00396 /*@}*/ 00397 00398 /** @defgroup Cycle_Control Measurement Cycle (Cycle_Control) Register 00399 * Measurement Cycle (Cycle_Control) Register. 00400 * @{ 00401 */ 00402 00403 /* ========================================================================= 00404 *! \enum ADMW_CORE_Cycle_Control_GND_SW_CTRL 00405 *! \brief Ground Switch Cycle Control (GND_SW_CTRL) Enumerations 00406 * ========================================================================= */ 00407 typedef enum 00408 { 00409 CORE_CYCLE_CONTROL_OPEN_SW = 0, /**< Ground Switch Opens outside of measurement cycle to conserve power */ 00410 CORE_CYCLE_CONTROL_CLOSE_SW = 1 /**< Ground Switch Closed */ 00411 } ADMW_CORE_Cycle_Control_GND_SW_CTRL ; 00412 00413 00414 /* ========================================================================= 00415 *! \enum ADMW_CORE_Cycle_Control_Vbias 00416 *! \brief Voltage Bias Global Enable (Vbias) Enumerations 00417 * ========================================================================= */ 00418 typedef enum 00419 { 00420 CORE_CYCLE_CONTROL_VBIAS_DISABLE = 0, /**< Vbias disabled */ 00421 CORE_CYCLE_CONTROL_VBIAS_ENABLE = 1 /**< Enable Vbias output for the duration of a cycle */ 00422 } ADMW_CORE_Cycle_Control_Vbias ; 00423 00424 00425 /* ========================================================================= 00426 *! \enum ADMW_CORE_Cycle_Control_Cycle_Time_Units 00427 *! \brief Units for Cycle Time (Cycle_Time_Units) Enumerations 00428 * ========================================================================= */ 00429 typedef enum 00430 { 00431 CORE_CYCLE_CONTROL_MILLISECONDS = 0, /**< Milli-seconds */ 00432 CORE_CYCLE_CONTROL_SECONDS = 1 /**< Seconds */ 00433 } ADMW_CORE_Cycle_Control_Cycle_Time_Units ; 00434 00435 00436 /* ========================================================================= 00437 *! \enum ADMW_CORE_Cycle_Control_PST_MEAS_EXC_CTRL 00438 *! \brief Disable Current Sources After Measurement Completes (PST_MEAS_EXC_CTRL) Enumerations 00439 * ========================================================================= */ 00440 typedef enum 00441 { 00442 CORE_CYCLE_CONTROL_POWERCYCLE = 0, /**< */ 00443 CORE_CYCLE_CONTROL_ALWAYSON = 1 /**< */ 00444 } ADMW_CORE_Cycle_Control_PST_MEAS_EXC_CTRL; 00445 00446 00447 /* ========================================================================== 00448 *! \struct ADMW_CORE_Cycle_Control_Struct 00449 *! \brief Measurement Cycle Register bit field structure 00450 * ========================================================================== */ 00451 typedef struct _ADMW_CORE_Cycle_Control_t { 00452 union { 00453 struct { 00454 uint16_t Cycle_Time : 12; /**< Time Between Measurement Cycles */ 00455 uint16_t GND_SW_CTRL : 1; /**< Ground Switch Cycle Control */ 00456 uint16_t Vbias : 1; /**< Voltage Bias Global Enable */ 00457 uint16_t Cycle_Time_Units : 1; /**< Units for Cycle Time */ 00458 uint16_t PST_MEAS_EXC_CTRL : 1; /**< Disable Current Sources After Measurement Completes */ 00459 }; 00460 uint16_t VALUE16; 00461 }; 00462 } ADMW_CORE_Cycle_Control_t; 00463 00464 /*@}*/ 00465 00466 /** @defgroup Fifo_Num_Cycles Number of Measurement Cycles to Store in FIFO (Fifo_Num_Cycles) Register 00467 * Number of Measurement Cycles to Store in FIFO (Fifo_Num_Cycles) Register. 00468 * @{ 00469 */ 00470 00471 /* ========================================================================== 00472 *! \struct ADMW_CORE_Fifo_Num_Cycles_Struct 00473 *! \brief Number of Measurement Cycles to Store in FIFO Register bit field structure 00474 * ========================================================================== */ 00475 typedef struct _ADMW_CORE_Fifo_Num_Cycles_t { 00476 union { 00477 struct { 00478 uint8_t Fifo_Num_Cycles : 8; /**< Number of Cycles to Fill the FIFO */ 00479 }; 00480 uint8_t VALUE8; 00481 }; 00482 } ADMW_CORE_Fifo_Num_Cycles_t; 00483 00484 /*@}*/ 00485 00486 /** @defgroup Status General Status (Status) Register 00487 * General Status (Status) Register. 00488 * @{ 00489 */ 00490 00491 /* ========================================================================== 00492 *! \struct ADMW_CORE_Status_Struct 00493 *! \brief General Status Register bit field structure 00494 * ========================================================================== */ 00495 typedef struct _ADMW_CORE_Status_t { 00496 union { 00497 struct { 00498 uint8_t Configuration_Error : 1; /**< Indicates Error with Programmed Configuration */ 00499 uint8_t Alert_Active : 1; /**< Indicates One or More Sensor Alerts Active */ 00500 uint8_t Error : 1; /**< Indicates an Error */ 00501 uint8_t Drdy : 1; /**< Indicates New Sensor Result Available to Read */ 00502 uint8_t Cmd_Running : 1; /**< Indicates Special Command Active, Active Low */ 00503 uint8_t FIFO_Error : 1; /**< Indicates Error with FIFO */ 00504 uint8_t Diag_Checksum_Error : 1; /**< Indicates Error on Internal Checksum Calculations */ 00505 uint8_t LUT_Error : 1; /**< Indicates Error with One or More Lookup Tables */ 00506 }; 00507 uint8_t VALUE8; 00508 }; 00509 } ADMW_CORE_Status_t; 00510 00511 /*@}*/ 00512 00513 /** @defgroup Channel_Alert_Status Alert Status Summary (Channel_Alert_Status) Register 00514 * Alert Status Summary (Channel_Alert_Status) Register. 00515 * @{ 00516 */ 00517 00518 /* ========================================================================== 00519 *! \struct ADMW_CORE_Channel_Alert_Status_Struct 00520 *! \brief Alert Status Summary Register bit field structure 00521 * ========================================================================== */ 00522 typedef struct _ADMW_CORE_Channel_Alert_Status_t { 00523 union { 00524 struct { 00525 uint16_t Alert_Ch0 : 1; /**< Indicates Channel 0 Alert Active */ 00526 uint16_t Alert_Ch1 : 1; /**< Indicates Channel 1 Alert Active */ 00527 uint16_t Alert_Ch2 : 1; /**< Indicates Channel 2 Alert Active */ 00528 uint16_t Alert_Ch3 : 1; /**< Indicates Channel 3 Alert Active */ 00529 uint16_t Alert_Ch4 : 1; /**< Indicates Channel 4 Alert Active */ 00530 uint16_t Alert_Ch5 : 1; /**< Indicates Channel 5Alert Active */ 00531 uint16_t Alert_Ch6 : 1; /**< Indicates Channel 6 Alert Active */ 00532 uint16_t Alert_Ch7 : 1; /**< Indicates Channel 7 Alert Active */ 00533 uint16_t Alert_Ch8 : 1; /**< Indicates Channel 8 Alert Active */ 00534 uint16_t Alert_Ch9 : 1; /**< Indicates Channel 9 Alert Active */ 00535 uint16_t Alert_Ch10 : 1; /**< Indicates Channel 10 Alert Active */ 00536 uint16_t Alert_Ch11 : 1; /**< Indicates Channel 11 Alert Active */ 00537 uint16_t Alert_Ch12 : 1; /**< Indicates Channel 12 Alert Active */ 00538 uint16_t reserved13 : 3; 00539 }; 00540 uint16_t VALUE16; 00541 }; 00542 } ADMW_CORE_Channel_Alert_Status_t; 00543 00544 /*@}*/ 00545 00546 /** @defgroup Alert_Detail_Ch Detailed Channel Error Information (Alert_Detail_Ch) Register 00547 * Detailed Channel Error Information (Alert_Detail_Ch) Register. 00548 * @{ 00549 */ 00550 00551 /* ========================================================================== 00552 *! \struct ADMW_CORE_Alert_Detail_Ch_Struct 00553 *! \brief Detailed Channel Error Information Register bit field structure 00554 * ========================================================================== */ 00555 typedef struct _ADMW_CORE_Alert_Detail_Ch_t { 00556 union { 00557 struct { 00558 uint16_t Result_Valid : 1; /**< Set If a Result is Valid */ 00559 uint16_t ADC_Near_Overrange : 1; /**< Indicates If the ADC is Near Overrange */ 00560 uint16_t Sensor_UnderRange : 1; /**< Indicates If the Sensor is Underrange */ 00561 uint16_t Sensor_OverRange : 1; /**< Indicates If the Sensor is Overrange */ 00562 uint16_t CJ_Soft_Fault : 1; /**< Cold Junction Soft Fault */ 00563 uint16_t CJ_Hard_Fault : 1; /**< Cold Junction Hard Fault */ 00564 uint16_t ADC_Input_OverRange : 1; /**< Indicates the ADC Input is Overrange */ 00565 uint16_t Sensor_HardFault : 1; /**< Indicates Sensor Hard Fault */ 00566 uint16_t Threshold_Exceeded : 1; /**< Channel Threshold Limits Exceeded */ 00567 uint16_t reserved9 : 7; 00568 }; 00569 uint16_t VALUE16; 00570 }; 00571 } ADMW_CORE_Alert_Detail_Ch_t; 00572 00573 /*@}*/ 00574 00575 /** @defgroup Error_Code Code Indicating Source of Error (Error_Code) Register 00576 * Code Indicating Source of Error (Error_Code) Register. 00577 * @{ 00578 */ 00579 00580 /* ========================================================================== 00581 *! \struct ADMW_CORE_Error_Code_Struct 00582 *! \brief Code Indicating Source of Error Register bit field structure 00583 * ========================================================================== */ 00584 typedef struct _ADMW_CORE_Error_Code_t { 00585 union { 00586 struct { 00587 uint16_t Error_Code : 16; /**< Code Indicating Type of Error */ 00588 }; 00589 uint16_t VALUE16; 00590 }; 00591 } ADMW_CORE_Error_Code_t; 00592 00593 /*@}*/ 00594 00595 /** @defgroup External_Reference_Resistor External Reference Resistor Value (External_Reference_Resistor) Register 00596 * External Reference Resistor Value (External_Reference_Resistor) Register. 00597 * @{ 00598 */ 00599 00600 /* ========================================================================== 00601 *! \struct ADMW_CORE_External_Reference_Resistor_Struct 00602 *! \brief External Reference Resistor Value Register bit field structure 00603 * ========================================================================== */ 00604 typedef struct _ADMW_CORE_External_Reference_Resistor_t { 00605 union { 00606 struct { 00607 float Ext_Refin1_Value; /**< External Reference Resistor Value */ 00608 }; 00609 float VALUE32; 00610 }; 00611 } ADMW_CORE_External_Reference_Resistor_t; 00612 00613 /*@}*/ 00614 00615 /** @defgroup External_Voltage_Reference External Reference Information (External_Voltage_Reference) Register 00616 * External Reference Information (External_Voltage_Reference) Register. 00617 * @{ 00618 */ 00619 00620 /* ========================================================================== 00621 *! \struct ADMW_CORE_External_Voltage_Reference_Struct 00622 *! \brief External Reference Information Register bit field structure 00623 * ========================================================================== */ 00624 typedef struct _ADMW_CORE_External_Voltage_Reference_t { 00625 union { 00626 struct { 00627 float Ext_Refin2_Value; /**< Reference Input Value */ 00628 }; 00629 float VALUE32; 00630 }; 00631 } ADMW_CORE_External_Voltage_Reference_t; 00632 00633 /*@}*/ 00634 00635 /** @defgroup AVDD_Voltage AVDD Voltage (AVDD_Voltage) Register 00636 * AVDD Voltage (AVDD_Voltage) Register. 00637 * @{ 00638 */ 00639 00640 /* ========================================================================== 00641 *! \struct ADMW_CORE_AVDD_Voltage_Struct 00642 *! \brief AVDD Voltage Register bit field structure 00643 * ========================================================================== */ 00644 typedef struct _ADMW_CORE_AVDD_Voltage_t { 00645 union { 00646 struct { 00647 float Avdd_Voltage; /**< AVDD Voltage */ 00648 }; 00649 float VALUE32; 00650 }; 00651 } ADMW_CORE_AVDD_Voltage_t; 00652 00653 /*@}*/ 00654 00655 /** @defgroup Diagnostics_Control Diagnostic Control (Diagnostics_Control) Register 00656 * Diagnostic Control (Diagnostics_Control) Register. 00657 * @{ 00658 */ 00659 00660 /* ========================================================================== 00661 *! \struct ADMW_CORE_Diagnostics_Control_Struct 00662 *! \brief Diagnostic Control Register bit field structure 00663 * ========================================================================== */ 00664 typedef struct _ADMW_CORE_Diagnostics_Control_t { 00665 union { 00666 struct { 00667 uint8_t Diag_Meas_En : 1; /**< Diagnostics Measure Enable */ 00668 uint8_t Diag_OSD_Freq : 7; /**< Diagnostics Open Sensor Detect Frequency */ 00669 }; 00670 uint8_t VALUE8; 00671 }; 00672 } ADMW_CORE_Diagnostics_Control_t; 00673 00674 /*@}*/ 00675 00676 /** @defgroup EXT_VBUFF External Reference Buffer (EXT_VBUFF) Register 00677 * External Reference Buffer (EXT_VBUFF) Register. 00678 * @{ 00679 */ 00680 00681 /* ========================================================================= 00682 *! \enum ADMW_CORE_EXT_VBUFF_EXT_VBUFF 00683 *! \brief This field is used to configure the reference buffers (EXT_VBUFF) Enumerations 00684 * ========================================================================= */ 00685 typedef enum 00686 { 00687 CORE_EXT_VBUFF_BOTH_INACTIVE_MODE = 0, /**< Both Reference Buffers Disabled */ 00688 CORE_EXT_VBUFF_BOTH_ACTIVE_MODE = 1, /**< Both Reference Buffers Enabled */ 00689 CORE_EXT_VBUFF_ONLY_VPOS_MODE = 2 /**< VREF+ Enabled Only */ 00690 } ADMW_CORE_EXT_VBUFF_EXT_VBUFF ; 00691 00692 00693 /* ========================================================================== 00694 *! \struct ADMW_CORE_EXT_VBUFF_Struct 00695 *! \brief External Reference Buffer Register bit field structure 00696 * ========================================================================== */ 00697 typedef struct _ADMW_CORE_EXT_VBUFF_t { 00698 union { 00699 struct { 00700 uint8_t EXT_VBUFF : 2; /**< This field is used to configure the reference buffers */ 00701 uint8_t reserved2 : 6; 00702 }; 00703 uint8_t VALUE8; 00704 }; 00705 } ADMW_CORE_EXT_VBUFF_t; 00706 00707 /*@}*/ 00708 00709 /** @defgroup Data_FIFO FIFO Buffer of Sensor Results (Data_FIFO) Register 00710 * FIFO Buffer of Sensor Results (Data_FIFO) Register. 00711 * @{ 00712 */ 00713 00714 /* ========================================================================== 00715 *! \struct ADMW_CORE_Data_FIFO_Struct 00716 *! \brief FIFO Buffer of Sensor Results Register bit field structure 00717 * ========================================================================== */ 00718 typedef struct _ADMW_CORE_Data_FIFO_t { 00719 union { 00720 struct { 00721 uint8_t Data_Fifo : 8; /**< FIFO Buffer of Sensor Results */ 00722 }; 00723 uint8_t VALUE8; 00724 }; 00725 } ADMW_CORE_Data_FIFO_t; 00726 00727 /*@}*/ 00728 00729 /** @defgroup Debug_Code Additional Information on Source of Alert or Errors (Debug_Code) Register 00730 * Additional Information on Source of Alert or Errors (Debug_Code) Register. 00731 * @{ 00732 */ 00733 00734 /* ========================================================================== 00735 *! \struct ADMW_CORE_Debug_Code_Struct 00736 *! \brief Additional Information on Source of Alert or Errors Register bit field structure 00737 * ========================================================================== */ 00738 typedef struct _ADMW_CORE_Debug_Code_t { 00739 union { 00740 struct { 00741 uint32_t Debug_Code : 32; /**< Additional Information on Source of Alert or Errors */ 00742 }; 00743 uint32_t VALUE32; 00744 }; 00745 } ADMW_CORE_Debug_Code_t; 00746 00747 /*@}*/ 00748 00749 /** @defgroup Test_Reg_Access Allows Access to Test (Hidden) Registers and Features (Test_Reg_Access) Register 00750 * Allows Access to Test (Hidden) Registers and Features (Test_Reg_Access) Register. 00751 * @{ 00752 */ 00753 00754 /* ========================================================================== 00755 *! \struct ADMW_CORE_Test_Reg_Access_Struct 00756 *! \brief Allows Access to Test (Hidden) Registers and Features Register bit field structure 00757 * ========================================================================== */ 00758 typedef struct _ADMW_CORE_Test_Reg_Access_t { 00759 union { 00760 struct { 00761 uint16_t Test_Access : 16; /**< Test Register Access. Specific Write Sequence Required */ 00762 }; 00763 uint16_t VALUE16; 00764 }; 00765 } ADMW_CORE_Test_Reg_Access_t; 00766 00767 /*@}*/ 00768 00769 /** @defgroup LUT_Select LUT Read/Write Strobe (LUT_Select) Register 00770 * LUT Read/Write Strobe (LUT_Select) Register. 00771 * @{ 00772 */ 00773 00774 /* ========================================================================= 00775 *! \enum ADMW_CORE_LUT_Select_LUT_RW 00776 *! \brief Read or Write LUT Data (LUT_RW) Enumerations 00777 * ========================================================================= */ 00778 typedef enum 00779 { 00780 CORE_LUT_SELECT_LUT_READ = 0, /**< Read addressed LUT data */ 00781 CORE_LUT_SELECT_LUT_WRITE = 1 /**< Write addressed LUT data */ 00782 } ADMW_CORE_LUT_Select_LUT_RW ; 00783 00784 00785 /* ========================================================================== 00786 *! \struct ADMW_CORE_LUT_Select_Struct 00787 *! \brief LUT Read/Write Strobe Register bit field structure 00788 * ========================================================================== */ 00789 typedef struct _ADMW_CORE_LUT_Select_t { 00790 union { 00791 struct { 00792 uint8_t reserved0 : 7; 00793 uint8_t LUT_RW : 1; /**< Read or Write LUT Data */ 00794 }; 00795 uint8_t VALUE8; 00796 }; 00797 } ADMW_CORE_LUT_Select_t; 00798 00799 /*@}*/ 00800 00801 /** @defgroup LUT_Offset Offset into Selected LUT (LUT_Offset) Register 00802 * Offset into Selected LUT (LUT_Offset) Register. 00803 * @{ 00804 */ 00805 00806 /* ========================================================================== 00807 *! \struct ADMW_CORE_LUT_Offset_Struct 00808 *! \brief Offset into Selected LUT Register bit field structure 00809 * ========================================================================== */ 00810 typedef struct _ADMW_CORE_LUT_Offset_t { 00811 union { 00812 struct { 00813 uint16_t LUT_Offset : 11; /**< Offset into the Lookup Table */ 00814 uint16_t reserved11 : 5; 00815 }; 00816 uint16_t VALUE16; 00817 }; 00818 } ADMW_CORE_LUT_Offset_t; 00819 00820 /*@}*/ 00821 00822 /** @defgroup LUT_Data Data to Read/Write from Addressed LUT Entry (LUT_Data) Register 00823 * Data to Read/Write from Addressed LUT Entry (LUT_Data) Register. 00824 * @{ 00825 */ 00826 00827 /* ========================================================================== 00828 *! \struct ADMW_CORE_LUT_Data_Struct 00829 *! \brief Data to Read/Write from Addressed LUT Entry Register bit field structure 00830 * ========================================================================== */ 00831 typedef struct _ADMW_CORE_LUT_Data_t { 00832 union { 00833 struct { 00834 uint8_t LUT_Data : 8; /**< Data Byte to Write to and Read from the Lookup Table */ 00835 }; 00836 uint8_t VALUE8; 00837 }; 00838 } ADMW_CORE_LUT_Data_t; 00839 00840 /*@}*/ 00841 00842 /** @defgroup Revision Hardware, Firmware Revision (Revision) Register 00843 * Hardware, Firmware Revision (Revision) Register. 00844 * @{ 00845 */ 00846 00847 /* ========================================================================== 00848 *! \struct ADMW_CORE_Revision_Struct 00849 *! \brief Hardware, Firmware Revision Register bit field structure 00850 * ========================================================================== */ 00851 typedef struct _ADMW_CORE_Revision_t { 00852 union { 00853 struct { 00854 uint32_t Rev_Patch : 16; /**< Patch Revision Information */ 00855 uint32_t Rev_Minor : 8; /**< Minor Revision Information */ 00856 uint32_t Rev_Major : 8; /**< Major Revision Information */ 00857 }; 00858 uint32_t VALUE32; 00859 }; 00860 } ADMW_CORE_Revision_t; 00861 00862 /*@}*/ 00863 00864 /** @defgroup Channel_Count Number of Channel Occurrences per Measurement Cycle (Channel_Count) Register 00865 * Number of Channel Occurrences per Measurement Cycle (Channel_Count) Register. 00866 * @{ 00867 */ 00868 00869 /* ========================================================================== 00870 *! \struct ADMW_CORE_Channel_Count_Struct 00871 *! \brief Number of Channel Occurrences per Measurement Cycle Register bit field structure 00872 * ========================================================================== */ 00873 typedef struct _ADMW_CORE_Channel_Count_t { 00874 union { 00875 struct { 00876 uint8_t Channel_Count : 7; /**< How Many Times Channel Appears in One Cycle */ 00877 uint8_t Channel_Enable : 1; /**< Enable Channel in Measurement Cycle */ 00878 }; 00879 uint8_t VALUE8; 00880 }; 00881 } ADMW_CORE_Channel_Count_t; 00882 00883 /*@}*/ 00884 00885 /** @defgroup Channel_Options Position of Channel Within Sequence (Channel_Options) Register 00886 * Position of Channel Within Sequence (Channel_Options) Register. 00887 * @{ 00888 */ 00889 00890 /* ========================================================================== 00891 *! \struct ADMW_CORE_Channel_Options_Struct 00892 *! \brief Position of Channel Within Sequence Register bit field structure 00893 * ========================================================================== */ 00894 typedef struct _ADMW_CORE_Channel_Options_t { 00895 union { 00896 struct { 00897 uint8_t Channel_Priority : 4; /**< Indicates Priority or Position of This Channel in Sequence */ 00898 uint8_t reserved4 : 4; 00899 }; 00900 uint8_t VALUE8; 00901 }; 00902 } ADMW_CORE_Channel_Options_t; 00903 00904 /*@}*/ 00905 00906 /** @defgroup Sensor_Type Sensor Select (Sensor_Type) Register 00907 * Sensor Select (Sensor_Type) Register. 00908 * @{ 00909 */ 00910 00911 /* ========================================================================= 00912 *! \enum ADMW_CORE_Sensor_Type_Sensor_Type 00913 *! \brief Sensor Type (Sensor_Type) Enumerations 00914 * ========================================================================= */ 00915 typedef enum 00916 { 00917 CORE_SENSOR_TYPE_THERMOCOUPLE_T = 0, /**< Thermocouple T-Type sensor */ 00918 CORE_SENSOR_TYPE_THERMOCOUPLE_J = 1, /**< Thermocouple J-Type Sensor */ 00919 CORE_SENSOR_TYPE_THERMOCOUPLE_K = 2, /**< Thermocouple K-Type Sensor */ 00920 CORE_SENSOR_TYPE_THERMOCOUPLE_E = 3, /**< Thermocouple E-Type Sensor */ 00921 CORE_SENSOR_TYPE_THERMOCOUPLE_N = 4, /**< Thermocouple N-Type Sensor */ 00922 CORE_SENSOR_TYPE_THERMOCOUPLE_R = 5, /**< Thermocouple R-Type Sensor */ 00923 CORE_SENSOR_TYPE_THERMOCOUPLE_S = 6, /**< Thermocouple S-Type Sensor */ 00924 CORE_SENSOR_TYPE_THERMOCOUPLE_B = 7, /**< Thermocouple B-Type Sensor */ 00925 CORE_SENSOR_TYPE_THERMOCOUPLE_CUSTOM = 8, /**< Thermocouple CUSTOM-Type Sensor */ 00926 CORE_SENSOR_TYPE_RTD_2W_PT100 = 32, /**< RTD 2 wire PT100 sensor */ 00927 CORE_SENSOR_TYPE_RTD_2W_PT1000 = 33, /**< RTD 2 wire PT1000 sensor */ 00928 CORE_SENSOR_TYPE_RTD_2W_PT10 = 34, /**< RTD 2 wire PT10 sensor */ 00929 CORE_SENSOR_TYPE_RTD_2W_PT50 = 35, /**< RTD 2 wire PT50 sensor */ 00930 CORE_SENSOR_TYPE_RTD_2W_PT200 = 36, /**< RTD 2 wire PT200 sensor */ 00931 CORE_SENSOR_TYPE_RTD_2W_PT500 = 37, /**< RTD 2 wire PT500 sensor */ 00932 CORE_SENSOR_TYPE_RTD_2W_PT1000_0P00375 = 38, /**< RTD 2 wire PT1000 sensor */ 00933 CORE_SENSOR_TYPE_RTD_2W_NI120 = 39, /**< RTD 2 wire PT1000 sensor */ 00934 CORE_SENSOR_TYPE_RTD_2W_CUSTOM = 40, /**< RTD 2 wire Custom sensor */ 00935 CORE_SENSOR_TYPE_RTD_3W_PT100 = 64, /**< RTD 3 wire PT100 sensor */ 00936 CORE_SENSOR_TYPE_RTD_3W_PT1000 = 65, /**< RTD 3 wire PT1000 sensor */ 00937 CORE_SENSOR_TYPE_RTD_3W_PT10 = 66, /**< RTD 3 wire PT10 sensor */ 00938 CORE_SENSOR_TYPE_RTD_3W_PT50 = 67, /**< RTD 3 wire PT50 sensor */ 00939 CORE_SENSOR_TYPE_RTD_3W_PT200 = 68, /**< RTD 3 wire PT200 sensor */ 00940 CORE_SENSOR_TYPE_RTD_3W_PT500 = 69, /**< RTD 3 wire PT500 sensor */ 00941 CORE_SENSOR_TYPE_RTD_3W_PT1000_0P00375 = 70, /**< RTD 3 wire PT1000 sensor */ 00942 CORE_SENSOR_TYPE_RTD_3W_NI120 = 71, /**< RTD 3 wire NI120 sensor */ 00943 CORE_SENSOR_TYPE_RTD_3W_CUSTOM = 72, /**< RTD 3 wire Custom sensor */ 00944 CORE_SENSOR_TYPE_RTD_4W_PT100 = 96, /**< RTD 4 wire PT100 sensor */ 00945 CORE_SENSOR_TYPE_RTD_4W_PT1000 = 97, /**< RTD 4 wire PT1000 sensor */ 00946 CORE_SENSOR_TYPE_RTD_4W_PT10 = 98, /**< RTD 4 wire PT10 sensor */ 00947 CORE_SENSOR_TYPE_RTD_4W_PT50 = 99, /**< RTD 4 wire PT50 sensor */ 00948 CORE_SENSOR_TYPE_RTD_4W_PT200 = 100, /**< RTD 4 wire PT200 sensor */ 00949 CORE_SENSOR_TYPE_RTD_4W_PT500 = 101, /**< RTD 4 wire PT500 sensor */ 00950 CORE_SENSOR_TYPE_RTD_4W_PT1000_0P00375 = 102, /**< RTD 4 wire PT1000 0.00375 sensor */ 00951 CORE_SENSOR_TYPE_RTD_4W_NI120 = 103, /**< RTD 4 wire NI120 */ 00952 CORE_SENSOR_TYPE_RTD_4W_CUSTOM = 104, /**< RTD 4 wire Custom sensor */ 00953 CORE_SENSOR_TYPE_THERMISTOR_44004_44033_2P252K_AT_25C = 128, /**< THERMISTOR_44004_44033_2P252K_AT_25C */ 00954 CORE_SENSOR_TYPE_THERMISTOR_44005_44030_3K_AT_25C = 129, /**< THERMISTOR_44005_44030_3K_AT_25C */ 00955 CORE_SENSOR_TYPE_THERMISTOR_44007_44034_5K_AT_25C = 130, /**< THERMISTOR_44007_44034_5K_AT_25C */ 00956 CORE_SENSOR_TYPE_THERMISTOR_44006_44031_10K_AT_25C = 131, /**< THERMISTOR_44006_44031_10K_AT_25C */ 00957 CORE_SENSOR_TYPE_THERMISTOR_44008_44032_30K_AT_25C = 132, /**< THERMISTOR_44008_44032_30K_AT_25C */ 00958 CORE_SENSOR_TYPE_THERMISTOR_YSI_400 = 133, /**< THERMISTOR_YSI_400 */ 00959 CORE_SENSOR_TYPE_THERMISTOR_SPECTRUM_1003K_1K = 134, /**< THERMISTOR_SPECTRUM_1003K_1K */ 00960 CORE_SENSOR_TYPE_THERMISTOR_CUSTOM_STEINHART_HART = 135, /**< THERMISTOR_CUSTOM_STEINHART_HART */ 00961 CORE_SENSOR_TYPE_THERMISTOR_CUSTOM_TABLE = 136, /**< THERMISTOR_CUSTOM_TABLE */ 00962 CORE_SENSOR_TYPE_BRIDGE_4WIRE = 168, /**< Bridge 4 wire sensor */ 00963 CORE_SENSOR_TYPE_BRIDGE_6WIRE = 200, /**< Bridge 6 wire sensor */ 00964 CORE_SENSOR_TYPE_DIODE = 224, /**< DIODE Temperature Sensor */ 00965 CORE_SENSOR_TYPE_SINGLE_ENDED_ABSOLUTE = 576, /**< Voltage Input Single Ended on V+ */ 00966 CORE_SENSOR_TYPE_DIFFERENTIAL_ABSOLUTE = 640, /**< Voltage Input Differential Ended on V+ and V- */ 00967 CORE_SENSOR_TYPE_SINGLE_ENDED_RATIO = 656, /**< Ratiometeric Output, Voltage_IN/Voltage_Reference */ 00968 CORE_SENSOR_TYPE_DIFFERENTIAL_RATIO = 672, /**< Ratiometeric Output, Voltage_IN/Voltage_Reference */ 00969 CORE_SENSOR_TYPE_I2C_HUMIDITY = 2112, /**< I2C humidity sensor B */ 00970 CORE_SENSOR_TYPE_I2C_TEMPERATURE_ADT742X = 2218 /**< ADI Precision I2C Digital Temperature Sensor */ 00971 } ADMW_CORE_Sensor_Type_Sensor_Type ; 00972 00973 00974 /* ========================================================================== 00975 *! \struct ADMW_CORE_Sensor_Type_Struct 00976 *! \brief Sensor Select Register bit field structure 00977 * ========================================================================== */ 00978 typedef struct _ADMW_CORE_Sensor_Type_t { 00979 union { 00980 struct { 00981 uint16_t Sensor_Type : 12; /**< Sensor Type */ 00982 uint16_t reserved12 : 4; 00983 }; 00984 uint16_t VALUE16; 00985 }; 00986 } ADMW_CORE_Sensor_Type_t; 00987 00988 /*@}*/ 00989 00990 /** @defgroup Sensor_Details Sensor Details (Sensor_Details) Register 00991 * Sensor Details (Sensor_Details) Register. 00992 * @{ 00993 */ 00994 00995 /* ========================================================================= 00996 *! \enum ADMW_CORE_Sensor_Details_Measurement_Units 00997 *! \brief Units of Sensor Measurement (Measurement_Units) Enumerations 00998 * ========================================================================= */ 00999 typedef enum 01000 { 01001 CORE_SENSOR_DETAILS_UNITS_UNSPECIFIED = 0, /**< Not Specified */ 01002 CORE_SENSOR_DETAILS_UNITS_RESERVED = 1, /**< Reserved */ 01003 CORE_SENSOR_DETAILS_UNITS_DEGC = 2, /**< Degrees C */ 01004 CORE_SENSOR_DETAILS_UNITS_DEGF = 3 /**< Degrees F */ 01005 } ADMW_CORE_Sensor_Details_Measurement_Units ; 01006 01007 01008 /* ========================================================================= 01009 *! \enum ADMW_CORE_Sensor_Details_LUT_Select 01010 *! \brief Lookup Table Select (LUT_Select) Enumerations 01011 * ========================================================================= */ 01012 typedef enum 01013 { 01014 CORE_SENSOR_DETAILS_LUT_DEFAULT = 0, /**< Default lookup table for selected sensor type */ 01015 CORE_SENSOR_DETAILS_LUT_CUSTOM = 1, /**< User defined custom lookup table. */ 01016 CORE_SENSOR_DETAILS_LUT_RESERVED = 2 /**< Reserved */ 01017 } ADMW_CORE_Sensor_Details_LUT_Select ; 01018 01019 01020 /* ========================================================================= 01021 *! \enum ADMW_CORE_Sensor_Details_Reference_Select 01022 *! \brief Reference Selection (Reference_Select) Enumerations 01023 * ========================================================================= */ 01024 typedef enum 01025 { 01026 CORE_SENSOR_DETAILS_REF_VINT = 0, /**< Internal voltage reference (1.2V) */ 01027 CORE_SENSOR_DETAILS_REF_VEXT1 = 1, /**< External voltage reference applied to VERF+ and VREF- */ 01028 CORE_SENSOR_DETAILS_REF_AVDD = 3 /**< AVDD supply internally used as reference */ 01029 } ADMW_CORE_Sensor_Details_Reference_Select ; 01030 01031 01032 /* ========================================================================= 01033 *! \enum ADMW_CORE_Sensor_Details_PGA_Gain 01034 *! \brief PGA Gain (PGA_Gain) Enumerations 01035 * ========================================================================= */ 01036 typedef enum 01037 { 01038 CORE_SENSOR_DETAILS_PGA_GAIN_1 = 0, /**< Gain of 1 */ 01039 CORE_SENSOR_DETAILS_PGA_GAIN_2 = 1, /**< Gain of 2 */ 01040 CORE_SENSOR_DETAILS_PGA_GAIN_4 = 2, /**< Gain of 4 */ 01041 CORE_SENSOR_DETAILS_PGA_GAIN_8 = 3, /**< Gain of 8 */ 01042 CORE_SENSOR_DETAILS_PGA_GAIN_16 = 4, /**< Gain of 16 */ 01043 CORE_SENSOR_DETAILS_PGA_GAIN_32 = 5, /**< Gain of 32 */ 01044 CORE_SENSOR_DETAILS_PGA_GAIN_64 = 6, /**< Gain of 64 */ 01045 CORE_SENSOR_DETAILS_PGA_GAIN_128 = 7 /**< Gain of 128 */ 01046 } ADMW_CORE_Sensor_Details_PGA_Gain ; 01047 01048 01049 /* ========================================================================= 01050 *! \enum ADMW_CORE_Sensor_Details_RTD_Curve 01051 *! \brief Select RTD Curve for Linearization (RTD_Curve) Enumerations 01052 * ========================================================================= */ 01053 typedef enum 01054 { 01055 CORE_SENSOR_DETAILS_EUROPEAN_CURVE = 0, /**< European curve */ 01056 CORE_SENSOR_DETAILS_AMERICAN_CURVE = 1, /**< American curve */ 01057 CORE_SENSOR_DETAILS_JAPANESE_CURVE = 2, /**< Japanese curve */ 01058 CORE_SENSOR_DETAILS_ITS90_CURVE = 3 /**< ITS-90 curve */ 01059 } ADMW_CORE_Sensor_Details_RTD_Curve ; 01060 01061 01062 /* ========================================================================== 01063 *! \struct ADMW_CORE_Sensor_Details_Struct 01064 *! \brief Sensor Details Register bit field structure 01065 * ========================================================================== */ 01066 typedef struct _ADMW_CORE_Sensor_Details_t { 01067 union { 01068 struct { 01069 uint32_t Measurement_Units : 4; /**< Units of Sensor Measurement */ 01070 uint32_t Compensation_Channel : 4; /**< Indicates Which Channel Used to Compensate the Sensor Result */ 01071 uint32_t reserved8 : 7; 01072 uint32_t LUT_Select : 2; /**< Lookup Table Select */ 01073 uint32_t Do_Not_Publish : 1; /**< Do Not Publish Channel Result */ 01074 uint32_t reserved18 : 2; 01075 uint32_t Reference_Select : 4; /**< Reference Selection */ 01076 uint32_t PGA_Gain : 3; /**< PGA Gain */ 01077 uint32_t RTD_Curve : 2; /**< Select RTD Curve for Linearization */ 01078 uint32_t reserved29 : 2; 01079 uint32_t Compensation_Disable : 1; /**< This Bit Indicates Compensation Data Must Not Be Used */ 01080 }; 01081 uint32_t VALUE32; 01082 }; 01083 } ADMW_CORE_Sensor_Details_t; 01084 01085 /*@}*/ 01086 01087 /** @defgroup Channel_Excitation Excitation Current (Channel_Excitation) Register 01088 * Excitation Current (Channel_Excitation) Register. 01089 * @{ 01090 */ 01091 01092 /* ========================================================================= 01093 *! \enum ADMW_CORE_Channel_Excitation_IOUT_Excitation_Current 01094 *! \brief Current Source Value (IOUT_Excitation_Current) Enumerations 01095 * ========================================================================= */ 01096 typedef enum 01097 { 01098 CORE_CHANNEL_EXCITATION_NONE = 0, /**< Excitation Current Disabled */ 01099 CORE_CHANNEL_EXCITATION_RESERVED = 1, /**< Reserved */ 01100 CORE_CHANNEL_EXCITATION_IEXC_10UA = 2, /**< 10 \mu;A */ 01101 CORE_CHANNEL_EXCITATION_RESERVED2 = 3, /**< Reserved */ 01102 CORE_CHANNEL_EXCITATION_IEXC_50UA = 4, /**< 50 \mu;A */ 01103 CORE_CHANNEL_EXCITATION_IEXC_100UA = 5, /**< 100 \mu;A */ 01104 CORE_CHANNEL_EXCITATION_IEXC_250UA = 6, /**< 250 \mu;A */ 01105 CORE_CHANNEL_EXCITATION_IEXC_500UA = 7, /**< 500 \mu;A */ 01106 CORE_CHANNEL_EXCITATION_IEXC_1000UA = 8, /**< 1000 \mu;A */ 01107 CORE_CHANNEL_EXCITATION_EXTERNAL = 15 /**< External current sourced */ 01108 } ADMW_CORE_Channel_Excitation_IOUT_Excitation_Current ; 01109 01110 01111 /* ========================================================================= 01112 *! \enum ADMW_CORE_Channel_Excitation_IOUT_Diode_Ratio 01113 *! \brief Modify Current Ratios Used for Diode Sensor (IOUT_Diode_Ratio) Enumerations 01114 * ========================================================================= */ 01115 typedef enum 01116 { 01117 CORE_CHANNEL_EXCITATION_DIODE_2PT_10UA_100UA = 0, /**< 2 Current measurement 10uA 100uA */ 01118 CORE_CHANNEL_EXCITATION_DIODE_2PT_20UA_160UA = 1, /**< 2 Current measurement 20uA 160uA */ 01119 CORE_CHANNEL_EXCITATION_DIODE_2PT_50UA_300UA = 2, /**< 2 Current measurement 50uA 300uA */ 01120 CORE_CHANNEL_EXCITATION_DIODE_2PT_100UA_600UA = 3, /**< 2 Current measurement 100uA 600uA */ 01121 CORE_CHANNEL_EXCITATION_DIODE_3PT_10UA_50UA_100UA = 4, /**< 3 current measuremet 10uA 50uA 100uA */ 01122 CORE_CHANNEL_EXCITATION_DIODE_3PT_20UA_100UA_160UA = 5, /**< 3 current measuremet 20uA 100uA 160uA */ 01123 CORE_CHANNEL_EXCITATION_DIODE_3PT_50UA_150UA_300UA = 6, /**< 3 current measuremet 50uA 150uA 300uA */ 01124 CORE_CHANNEL_EXCITATION_DIODE_3PT_100UA_300UA_600UA = 7 /**< 3 current measuremet 100uA 300uA 600uA */ 01125 } ADMW_CORE_Channel_Excitation_IOUT_Diode_Ratio ; 01126 01127 01128 /* ========================================================================== 01129 *! \struct ADMW_CORE_Channel_Excitation_Struct 01130 *! \brief Excitation Current Register bit field structure 01131 * ========================================================================== */ 01132 typedef struct _ADMW_CORE_Channel_Excitation_t { 01133 union { 01134 struct { 01135 uint16_t IOUT_Excitation_Current : 4; /**< Current Source Value */ 01136 uint16_t reserved4 : 2; 01137 uint16_t IOUT_Diode_Ratio : 3; /**< Modify Current Ratios Used for Diode Sensor */ 01138 uint16_t reserved9 : 7; 01139 }; 01140 uint16_t VALUE16; 01141 }; 01142 } ADMW_CORE_Channel_Excitation_t; 01143 01144 /*@}*/ 01145 01146 /** @defgroup Settling_Time Settling Time (Settling_Time) Register 01147 * Settling Time (Settling_Time) Register. 01148 * @{ 01149 */ 01150 01151 /* ========================================================================== 01152 *! \struct ADMW_CORE_Settling_Time_Struct 01153 *! \brief Settling Time Register bit field structure 01154 * ========================================================================== */ 01155 typedef struct _ADMW_CORE_Settling_Time_t { 01156 union { 01157 struct { 01158 uint16_t Settling_Time : 8; /**< Additional Settling Time in Milliseconds. Max 255ms */ 01159 uint16_t reserved8 : 8; 01160 }; 01161 uint16_t VALUE16; 01162 }; 01163 } ADMW_CORE_Settling_Time_t; 01164 01165 /*@}*/ 01166 01167 /** @defgroup Measurement_Setup ADC Measurement Setup (Measurement_Setup) Register 01168 * ADC Measurement Setup (Measurement_Setup) Register. 01169 * @{ 01170 */ 01171 01172 /* ========================================================================= 01173 *! \enum ADMW_CORE_Measurement_Setup_NOTCH_EN_2 01174 *! \brief Enable Notch 2 Filter Mode (NOTCH_EN_2) Enumerations 01175 * ========================================================================= */ 01176 typedef enum 01177 { 01178 CORE_MEASUREMENT_SETUP_NOTCH_DIS = 0, /**< Disable notch filter */ 01179 CORE_MEASUREMENT_SETUP_NOTCH_EN = 1 /**< Enable notch 2 filter option. */ 01180 } ADMW_CORE_Measurement_Setup_NOTCH_EN_2 ; 01181 01182 01183 /* ========================================================================= 01184 *! \enum ADMW_CORE_Measurement_Setup_Chop_Mode 01185 *! \brief Enabled and Disable Chop Mode (Chop_Mode) Enumerations 01186 * ========================================================================= */ 01187 typedef enum 01188 { 01189 CORE_MEASUREMENT_SETUP_DISABLE_CHOP = 0, /**< ADC front end chopping disabled */ 01190 CORE_MEASUREMENT_SETUP_ENABLE_CHOP = 1 /**< ADC front end chopping enabled */ 01191 } ADMW_CORE_Measurement_Setup_Chop_Mode ; 01192 01193 01194 /* ========================================================================= 01195 *! \enum ADMW_CORE_Measurement_Setup_ADC_Filter_Type 01196 *! \brief ADC Digital Filter Type (ADC_Filter_Type) Enumerations 01197 * ========================================================================= */ 01198 typedef enum 01199 { 01200 CORE_MEASUREMENT_SETUP_ENABLE_SINC4 = 0, /**< Enabled SINC4 filter */ 01201 CORE_MEASUREMENT_SETUP_ENABLE_SINC3 = 1 /**< Enabled SINC3 filter */ 01202 } ADMW_CORE_Measurement_Setup_ADC_Filter_Type ; 01203 01204 01205 /* ========================================================================= 01206 *! \enum ADMW_CORE_Measurement_Setup_Buffer_Bypass 01207 *! \brief Disable Buffers (Buffer_Bypass) Enumerations 01208 * ========================================================================= */ 01209 typedef enum 01210 { 01211 CORE_MEASUREMENT_SETUP_BUFFERS_ENABLED = 0, /**< Input buffers enabled */ 01212 CORE_MEASUREMENT_SETUP_BUFFERS_DISABLED = 1 /**< Input buffers disabled */ 01213 } ADMW_CORE_Measurement_Setup_Buffer_Bypass ; 01214 01215 01216 /* ========================================================================== 01217 *! \struct ADMW_CORE_Measurement_Setup_Struct 01218 *! \brief ADC Measurement Setup Register bit field structure 01219 * ========================================================================== */ 01220 typedef struct _ADMW_CORE_Measurement_Setup_t { 01221 union { 01222 struct { 01223 uint32_t ADC_SF : 7; /**< ADC Digital Filter Speed */ 01224 uint32_t reserved7 : 1; 01225 uint32_t NOTCH_EN_2 : 1; /**< Enable Notch 2 Filter Mode */ 01226 uint32_t reserved9 : 1; 01227 uint32_t Chop_Mode : 1; /**< Enabled and Disable Chop Mode */ 01228 uint32_t reserved11 : 1; 01229 uint32_t ADC_Filter_Type : 1; /**< ADC Digital Filter Type */ 01230 uint32_t reserved13 : 2; 01231 uint32_t Buffer_Bypass : 1; /**< Disable Buffers */ 01232 uint32_t reserved16 : 16; 01233 }; 01234 uint32_t VALUE32; 01235 }; 01236 } ADMW_CORE_Measurement_Setup_t; 01237 01238 /*@}*/ 01239 01240 /** @defgroup Ideality_Factor Diode Ideality Factor Register (Ideality_Factor) Register 01241 * Diode Ideality Factor Register (Ideality_Factor) Register. 01242 * @{ 01243 */ 01244 01245 /* ========================================================================== 01246 *! \struct ADMW_CORE_Ideality_Factor_Struct 01247 *! \brief Diode Ideality Factor Register bit field structure 01248 * ========================================================================== */ 01249 typedef struct _ADMW_CORE_Ideality_Factor_t { 01250 union { 01251 struct { 01252 float32_t Ideality_Factor; /**< Diode Ideality Factor, Default 1.003. */ 01253 }; 01254 float32_t VALUE32; 01255 }; 01256 } ADMW_CORE_Ideality_Factor_t; 01257 01258 /*@}*/ 01259 01260 /** @defgroup High_Threshold_Limit High Threshold (High_Threshold_Limit) Register 01261 * High Threshold (High_Threshold_Limit) Register. 01262 * @{ 01263 */ 01264 01265 /* ========================================================================== 01266 *! \struct ADMW_CORE_High_Threshold_Limit_Struct 01267 *! \brief High Threshold Register bit field structure 01268 * ========================================================================== */ 01269 typedef struct _ADMW_CORE_High_Threshold_Limit_t { 01270 union { 01271 struct { 01272 float High_Threshold; /**< Upper Limit for Sensor Alert Comparison */ 01273 }; 01274 float VALUE32; 01275 }; 01276 } ADMW_CORE_High_Threshold_Limit_t; 01277 01278 /*@}*/ 01279 01280 /** @defgroup Low_Threshold_Limit Low Threshold (Low_Threshold_Limit) Register 01281 * Low Threshold (Low_Threshold_Limit) Register. 01282 * @{ 01283 */ 01284 01285 /* ========================================================================== 01286 *! \struct ADMW_CORE_Low_Threshold_Limit_Struct 01287 *! \brief Low Threshold Register bit field structure 01288 * ========================================================================== */ 01289 typedef struct _ADMW_CORE_Low_Threshold_Limit_t { 01290 union { 01291 struct { 01292 float Low_Threshold; /**< Lower Limit for Sensor Alert Comparison */ 01293 }; 01294 float VALUE32; 01295 }; 01296 } ADMW_CORE_Low_Threshold_Limit_t; 01297 01298 /*@}*/ 01299 01300 /** @defgroup Sensor_Offset Sensor Offset Adjustment (Sensor_Offset) Register 01301 * Sensor Offset Adjustment (Sensor_Offset) Register. 01302 * @{ 01303 */ 01304 01305 /* ========================================================================== 01306 *! \struct ADMW_CORE_Sensor_Offset_Struct 01307 *! \brief Sensor Offset Adjustment Register bit field structure 01308 * ========================================================================== */ 01309 typedef struct _ADMW_CORE_Sensor_Offset_t { 01310 union { 01311 struct { 01312 float Sensor_Offset; /**< Sensor Offset Adjustment */ 01313 }; 01314 float VALUE32; 01315 }; 01316 } ADMW_CORE_Sensor_Offset_t; 01317 01318 /*@}*/ 01319 01320 /** @defgroup Sensor_Gain Sensor Gain Adjustment (Sensor_Gain) Register 01321 * Sensor Gain Adjustment (Sensor_Gain) Register. 01322 * @{ 01323 */ 01324 01325 /* ========================================================================== 01326 *! \struct ADMW_CORE_Sensor_Gain_Struct 01327 *! \brief Sensor Gain Adjustment Register bit field structure 01328 * ========================================================================== */ 01329 typedef struct _ADMW_CORE_Sensor_Gain_t { 01330 union { 01331 struct { 01332 float Sensor_Gain; /**< Sensor Gain Adjustment */ 01333 }; 01334 float VALUE32; 01335 }; 01336 } ADMW_CORE_Sensor_Gain_t; 01337 01338 /*@}*/ 01339 01340 /** @defgroup Channel_Skip Indicates If Channel Will Skip Some Measurement Cycles (Channel_Skip) Register 01341 * Indicates If Channel Will Skip Some Measurement Cycles (Channel_Skip) Register. 01342 * @{ 01343 */ 01344 01345 /* ========================================================================== 01346 *! \struct ADMW_CORE_Channel_Skip_Struct 01347 *! \brief Indicates If Channel Will Skip Some Measurement Cycles Register bit field structure 01348 * ========================================================================== */ 01349 typedef struct _ADMW_CORE_Channel_Skip_t { 01350 union { 01351 struct { 01352 uint16_t Channel_Skip : 8; /**< Indicates If Channel Will Skip Some Measurement Cycles */ 01353 uint16_t reserved8 : 8; 01354 }; 01355 uint16_t VALUE16; 01356 }; 01357 } ADMW_CORE_Channel_Skip_t; 01358 01359 /*@}*/ 01360 01361 /** @defgroup Sensor_Parameter Sensor Parameter Adjustment (Sensor_Parameter) Register 01362 * Sensor Parameter Adjustment (Sensor_Parameter) Register. 01363 * @{ 01364 */ 01365 01366 /* ========================================================================== 01367 *! \struct ADMW_CORE_Sensor_Parameter_Struct 01368 *! \brief Sensor Parameter Adjustment Register bit field structure 01369 * ========================================================================== */ 01370 typedef struct _ADMW_CORE_Sensor_Parameter_t { 01371 union { 01372 struct { 01373 float Sensor_Parameter; /**< Sensor Parameter Adjustment */ 01374 }; 01375 float VALUE32; 01376 }; 01377 } ADMW_CORE_Sensor_Parameter_t; 01378 01379 /*@}*/ 01380 01381 /** @defgroup Digital_Sensor_Config Digital Sensor Data Coding (Digital_Sensor_Config) Register 01382 * Digital Sensor Data Coding (Digital_Sensor_Config) Register. 01383 * @{ 01384 */ 01385 01386 /* ========================================================================= 01387 *! \enum ADMW_CORE_Digital_Sensor_Config_Digital_Sensor_Coding 01388 *! \brief Data Encoding of Sensor Result (Digital_Sensor_Coding) Enumerations 01389 * ========================================================================= */ 01390 typedef enum 01391 { 01392 CORE_DIGITAL_SENSOR_CONFIG_CODING_NONE = 0, /**< None/Invalid */ 01393 CORE_DIGITAL_SENSOR_CONFIG_CODING_UNIPOLAR = 1, /**< Unipolar */ 01394 CORE_DIGITAL_SENSOR_CONFIG_CODING_TWOS_COMPL = 2, /**< Twos complement */ 01395 CORE_DIGITAL_SENSOR_CONFIG_CODING_OFFSET_BINARY = 3 /**< Offset binary */ 01396 } ADMW_CORE_Digital_Sensor_Config_Digital_Sensor_Coding ; 01397 01398 01399 /* ========================================================================== 01400 *! \struct ADMW_CORE_Digital_Sensor_Config_Struct 01401 *! \brief Digital Sensor Data Coding Register bit field structure 01402 * ========================================================================== */ 01403 typedef struct _ADMW_CORE_Digital_Sensor_Config_t { 01404 union { 01405 struct { 01406 uint16_t Digital_Sensor_Coding : 2; /**< Data Encoding of Sensor Result */ 01407 uint16_t Digital_Sensor_Little_Endian : 1; /**< Data Endianness of Sensor Result */ 01408 uint16_t Digital_Sensor_Left_Aligned : 1; /**< Data Alignment Within the Data Frame */ 01409 uint16_t Digital_Sensor_Bit_Offset : 4; /**< Data Bit Offset, Relative to Alignment */ 01410 uint16_t Digital_Sensor_Read_Bytes : 3; /**< Number of Bytes to Read from the Sensor */ 01411 uint16_t Digital_Sensor_Data_Bits : 5; /**< Number of Relevant Data Bits */ 01412 }; 01413 uint16_t VALUE16; 01414 }; 01415 } ADMW_CORE_Digital_Sensor_Config_t; 01416 01417 /*@}*/ 01418 01419 /** @defgroup Digital_Sensor_Address Sensor Address (Digital_Sensor_Address) Register 01420 * Sensor Address (Digital_Sensor_Address) Register. 01421 * @{ 01422 */ 01423 01424 /* ========================================================================== 01425 *! \struct ADMW_CORE_Digital_Sensor_Address_Struct 01426 *! \brief Sensor Address Register bit field structure 01427 * ========================================================================== */ 01428 typedef struct _ADMW_CORE_Digital_Sensor_Address_t { 01429 union { 01430 struct { 01431 uint8_t Digital_Sensor_Address : 8; /**< I2C Address or Write Address Command for SPI Sensor */ 01432 }; 01433 uint8_t VALUE8; 01434 }; 01435 } ADMW_CORE_Digital_Sensor_Address_t; 01436 01437 /*@}*/ 01438 01439 /** @defgroup Digital_Sensor_Comms Digital Sensor Communication Clock Configuration (Digital_Sensor_Comms) Register 01440 * Digital Sensor Communication Clock Configuration (Digital_Sensor_Comms) Register. 01441 * @{ 01442 */ 01443 01444 /* ========================================================================= 01445 *! \enum ADMW_CORE_Digital_Sensor_Comms_SPI_Clock 01446 *! \brief Controls Clock Frequency for SPI Sensors (SPI_Clock) Enumerations 01447 * ========================================================================= */ 01448 typedef enum 01449 { 01450 CORE_DIGITAL_SENSOR_COMMS_SPI_8MHZ = 0, /**< 8MHz */ 01451 CORE_DIGITAL_SENSOR_COMMS_SPI_4MHZ = 1, /**< 4MHz */ 01452 CORE_DIGITAL_SENSOR_COMMS_SPI_2MHZ = 2, /**< 2MHz */ 01453 CORE_DIGITAL_SENSOR_COMMS_SPI_1MHZ = 3, /**< 1MHz */ 01454 CORE_DIGITAL_SENSOR_COMMS_SPI_500KHZ = 4, /**< 500kHz */ 01455 CORE_DIGITAL_SENSOR_COMMS_SPI_250KHZ = 5, /**< 250kHz */ 01456 CORE_DIGITAL_SENSOR_COMMS_SPI_125KHZ = 6, /**< 125kHz */ 01457 CORE_DIGITAL_SENSOR_COMMS_SPI_62P5KHZ = 7, /**< 62.5kHz */ 01458 CORE_DIGITAL_SENSOR_COMMS_SPI_31P3KHZ = 8, /**< 31.25kHz */ 01459 CORE_DIGITAL_SENSOR_COMMS_SPI_15P6KHZ = 9, /**< 15.625kHz */ 01460 CORE_DIGITAL_SENSOR_COMMS_SPI_7P8KHZ = 10, /**< 7.8kHz */ 01461 CORE_DIGITAL_SENSOR_COMMS_SPI_3P9KHZ = 11, /**< 3.9kHz */ 01462 CORE_DIGITAL_SENSOR_COMMS_SPI_1P9KHZ = 12, /**< 1.95kHz */ 01463 CORE_DIGITAL_SENSOR_COMMS_SPI_977HZ = 13, /**< 977Hz */ 01464 CORE_DIGITAL_SENSOR_COMMS_SPI_488HZ = 14, /**< 488Hz */ 01465 CORE_DIGITAL_SENSOR_COMMS_SPI_244HZ = 15 /**< 244Hz */ 01466 } ADMW_CORE_Digital_Sensor_Comms_SPI_Clock ; 01467 01468 01469 /* ========================================================================= 01470 *! \enum ADMW_CORE_Digital_Sensor_Comms_I2C_Clock 01471 *! \brief Controls SCLK Frequency for I2C Sensors (I2C_Clock) Enumerations 01472 * ========================================================================= */ 01473 typedef enum 01474 { 01475 CORE_DIGITAL_SENSOR_COMMS_I2C_100K = 0, /**< 100kHz SCL */ 01476 CORE_DIGITAL_SENSOR_COMMS_I2C_400K = 1, /**< 400kHz SCL */ 01477 CORE_DIGITAL_SENSOR_COMMS_I2C_RESERVED1 = 2, /**< Reserved */ 01478 CORE_DIGITAL_SENSOR_COMMS_I2C_RESERVED2 = 3 /**< Reserved */ 01479 } ADMW_CORE_Digital_Sensor_Comms_I2C_Clock ; 01480 01481 01482 /* ========================================================================= 01483 *! \enum ADMW_CORE_Digital_Sensor_Comms_SPI_Mode 01484 *! \brief Configuration for Sensor SPI Protocol (SPI_Mode) Enumerations 01485 * ========================================================================= */ 01486 typedef enum 01487 { 01488 CORE_DIGITAL_SENSOR_COMMS_SPI_MODE_0 = 0, /**< Clock polarity = 0 Clock phase = 0 */ 01489 CORE_DIGITAL_SENSOR_COMMS_SPI_MODE_1 = 1, /**< Clock polarity = 0 Clock phase = 1 */ 01490 CORE_DIGITAL_SENSOR_COMMS_SPI_MODE_2 = 2, /**< Clock polarity = 1 Clock phase = 0 */ 01491 CORE_DIGITAL_SENSOR_COMMS_SPI_MODE_3 = 3 /**< Clock polarity = 1 Clock phase = 1 */ 01492 } ADMW_CORE_Digital_Sensor_Comms_SPI_Mode ; 01493 01494 01495 /* ========================================================================== 01496 *! \struct ADMW_CORE_Digital_Sensor_Comms_Struct 01497 *! \brief Digital Sensor Communication Clock Configuration Register bit field structure 01498 * ========================================================================== */ 01499 typedef struct _ADMW_CORE_Digital_Sensor_Comms_t { 01500 union { 01501 struct { 01502 uint16_t reserved0 : 1; 01503 uint16_t SPI_Clock : 4; /**< Controls Clock Frequency for SPI Sensors */ 01504 uint16_t I2C_Clock : 2; /**< Controls SCLK Frequency for I2C Sensors */ 01505 uint16_t reserved7 : 3; 01506 uint16_t SPI_Mode : 2; /**< Configuration for Sensor SPI Protocol */ 01507 uint16_t reserved12 : 4; 01508 }; 01509 uint16_t VALUE16; 01510 }; 01511 } ADMW_CORE_Digital_Sensor_Comms_t; 01512 01513 /*@}*/ 01514 01515 01516 #if defined (__CC_ARM) 01517 #pragma pop 01518 #endif 01519 01520 #endif
Generated on Thu Jul 14 2022 10:33:00 by
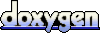