
Sensor ultrasónico con GAP
Dependencies: BLE_API HC_SR04_Ultrasonic_Library mbed
Fork of LPC1768_HCSR04_HelloWorld by
main.cpp
00001 #include "mbed.h" 00002 #include "ultrasonic.h" 00003 #include "ble/BLE.h" 00004 Serial pc(USBTX, USBRX); 00005 00006 const static char DEVICE_NAME[] = "EVA BRA TEMP"; 00007 00008 void disconnectionCallback(const Gap::DisconnectionCallbackParams_t *params) 00009 { 00010 BLE::Instance().gap().startAdvertising(); 00011 } 00012 00013 void onBleInitError(BLE &ble, ble_error_t error) 00014 { 00015 /* Avoid compiler warnings */ 00016 (void) ble; 00017 (void) error; 00018 00019 /* Initialization error handling should go here */ 00020 } 00021 00022 void bleInitComplete(BLE::InitializationCompleteCallbackContext *params) 00023 { 00024 BLE& ble = params->ble; 00025 ble_error_t error = params->error; 00026 00027 if (error != BLE_ERROR_NONE) { 00028 /* In case of error, forward the error handling to onBleInitError */ 00029 onBleInitError(ble, error); 00030 return; 00031 } 00032 00033 /* Ensure that it is the default instance of BLE */ 00034 if(ble.getInstanceID() != BLE::DEFAULT_INSTANCE) { 00035 return; 00036 } 00037 /* Set device name characteristic data */ 00038 ble.gap().setDeviceName((const uint8_t *) DEVICE_NAME); 00039 00040 /* Optional: add callback for disconnection */ 00041 ble.gap().onDisconnection(disconnectionCallback); 00042 00043 ble.gap().accumulateAdvertisingPayload(GapAdvertisingData::SERVICE_DATA, distance); 00044 00045 /* Sacrifice 3B of 31B to Advertising Flags */ 00046 ble.gap().accumulateAdvertisingPayload(GapAdvertisingData::BREDR_NOT_SUPPORTED | GapAdvertisingData::LE_GENERAL_DISCOVERABLE ); 00047 ble.gap().setAdvertisingType(GapAdvertisingParams::ADV_CONNECTABLE_UNDIRECTED); 00048 00049 00050 /* Set advertising interval. Longer interval == longer battery life */ 00051 ble.gap().setAdvertisingInterval(100); /* 100ms */ 00052 00053 /* Start advertising */ 00054 ble.gap().startAdvertising(); 00055 00056 } 00057 00058 void dist(uint8_t distance) 00059 { 00060 //put code here to execute when the distance has changed 00061 pc.printf("Distance %d mm\r\n", distance); 00062 } 00063 00064 ultrasonic mu(D6, D7, .1, 1, &dist); //Set the trigger pin to D8 and the echo pin to D9 00065 //have updates every .1 seconds and a timeout after 1 00066 //second, and call dist when the distance changes 00067 00068 int main() 00069 { 00070 BLE& ble = BLE::Instance(BLE::DEFAULT_INSTANCE); 00071 ble.init(bleInitComplete); 00072 00073 mu.startUpdates();//start measuring the distance 00074 while(1) 00075 { 00076 ble.waitForEvent(); 00077 //Do something else here 00078 mu.checkDistance(); //call checkDistance() as much as possible, as this is where 00079 //the class checks if dist needs to be called. 00080 } 00081 }
Generated on Fri Jul 15 2022 23:52:54 by
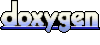