A Library for the AMS ENS210 temperature and humidity sensor.
Dependents: AMS_CCS811_gas_sensor AMS_CCS811_gas_sensor
AMS_ENS210 Class Reference
The AMS ENS210 class. More...
#include <AMS_ENS210.h>
Public Member Functions | |
AMS_ENS210 (PinName sda, PinName scl) | |
Create an AMS_ENS210 instance. | |
AMS_ENS210 (PinName sda, PinName scl, bool temp_continuous, bool humid_continuous) | |
Create an AMS_ENS210 instance. | |
AMS_ENS210 (PinName sda, PinName scl, bool temp_continuous, bool humid_continuous, bool low_power) | |
Create an AMS_ENS210 instance. | |
~AMS_ENS210 () | |
Destroy the AMS_ENS210 instance. | |
bool | init () |
Initalise the sensor. | |
bool | reset () |
Software reset the sensor. | |
bool | low_power_mode (bool low_power) |
Set the power mode. | |
bool | low_power_mode () |
Get the current power mode. | |
bool | is_active () |
Get whether the sensor is in the active state or not. | |
bool | temp_continuous_mode (bool continuous) |
Set the tempurature operation mode. | |
bool | temp_continuous_mode () |
Get the current tempurature operation mode. | |
bool | humid_continuous_mode (bool continuous) |
Set the humidity operation mode. | |
bool | humid_continuous_mode () |
Get the current humidity operation mode. | |
void | i2c_interface (I2C *i2c) |
Set the I2C interface. | |
I2C * | i2c_interface () |
Get the I2C interface. | |
bool | start (bool temp=true, bool humid=true) |
Start measurement collection. | |
bool | stop (bool temp=true, bool humid=true) |
Stop measurement collection. | |
bool | temp_is_measuring () |
Get whether the sensor is measuring tempurature data. | |
bool | humid_is_measuring () |
Get whether the sensor is measuring humidity data. | |
bool | temp_has_data () |
Get whether the sensor has collected tempurature data. | |
bool | humid_has_data () |
Get whether the sensor has collected humidity data. | |
uint16_t | temp_read () |
Get the most recent tempurature measurement, must call temp_has_data() first when in single shot mode otherwise the same data will be returned. | |
uint16_t | humid_read () |
Get the most recent humidty measurement, must call humid_has_data() first when in single shot mode otherwise the same data will be returned. |
Detailed Description
The AMS ENS210 class.
Definition at line 39 of file AMS_ENS210.h.
Constructor & Destructor Documentation
AMS_ENS210 | ( | PinName | sda, |
PinName | scl | ||
) |
Create an AMS_ENS210 instance.
- Parameters:
-
sda I2C SDA pin scl I2C SCL pin
Definition at line 4 of file AMS_ENS210.cpp.
AMS_ENS210 | ( | PinName | sda, |
PinName | scl, | ||
bool | temp_continuous, | ||
bool | humid_continuous | ||
) |
Create an AMS_ENS210 instance.
- Parameters:
-
sda I2C SDA pin scl I2C SCL pin temp_continuous Set tempurature operation mode, true for continuous and false for single shot humid_continuous Set humidity operation mode, true for continuous and false for single shot
Definition at line 14 of file AMS_ENS210.cpp.
AMS_ENS210 | ( | PinName | sda, |
PinName | scl, | ||
bool | temp_continuous, | ||
bool | humid_continuous, | ||
bool | low_power | ||
) |
Create an AMS_ENS210 instance.
- Parameters:
-
sda I2C SDA pin scl I2C SCL pin temp_continuous Set tempurature operation mode, true for continuous and false for single shot humid_continuous Set humidity operation mode, true for continuous and false for single shot low_power Set power mode, true for low power/standby and false for active
Definition at line 24 of file AMS_ENS210.cpp.
~AMS_ENS210 | ( | ) |
Destroy the AMS_ENS210 instance.
Definition at line 34 of file AMS_ENS210.cpp.
Member Function Documentation
bool humid_continuous_mode | ( | bool | continuous ) |
Set the humidity operation mode.
- Parameters:
-
single_shot True for continuous and false for single shot
- Returns:
- Write success
Definition at line 83 of file AMS_ENS210.cpp.
bool humid_continuous_mode | ( | ) |
Get the current humidity operation mode.
- Returns:
- Write success
Definition at line 89 of file AMS_ENS210.cpp.
bool humid_has_data | ( | ) |
Get whether the sensor has collected humidity data.
- Returns:
- Has data
Definition at line 143 of file AMS_ENS210.cpp.
bool humid_is_measuring | ( | ) |
Get whether the sensor is measuring humidity data.
- Returns:
- Sensor activity, true for measureing, false for idle
Definition at line 113 of file AMS_ENS210.cpp.
uint16_t humid_read | ( | ) |
Get the most recent humidty measurement, must call humid_has_data() first when in single shot mode otherwise the same data will be returned.
- Returns:
- Most recent humidity measurement as a 16 bits unsigned value in 1/64 Kelvin
Definition at line 184 of file AMS_ENS210.cpp.
void i2c_interface | ( | I2C * | i2c ) |
Set the I2C interface.
- Parameters:
-
i2c The I2C interface
I2C* i2c_interface | ( | ) |
Get the I2C interface.
- Returns:
- The I2C interface
bool init | ( | ) |
bool is_active | ( | ) |
Get whether the sensor is in the active state or not.
- Returns:
- The active state, true for active, false for inactive
Definition at line 65 of file AMS_ENS210.cpp.
bool low_power_mode | ( | ) |
Get the current power mode.
- Returns:
- The power mode, true for low power, false for active
Definition at line 60 of file AMS_ENS210.cpp.
bool low_power_mode | ( | bool | low_power ) |
Set the power mode.
- Parameters:
-
low_power True for low power/standby and false for active
- Returns:
- Write success
Definition at line 54 of file AMS_ENS210.cpp.
bool reset | ( | ) |
bool start | ( | bool | temp = true , |
bool | humid = true |
||
) |
Start measurement collection.
- Parameters:
-
temp Start the tempurature sensor humid Start the humidity sensor
- Returns:
- Write success
Definition at line 94 of file AMS_ENS210.cpp.
bool stop | ( | bool | temp = true , |
bool | humid = true |
||
) |
Stop measurement collection.
- Parameters:
-
temp Stop the tempurature sensor humid Stop the humidity sensor
- Returns:
- Write success
Definition at line 100 of file AMS_ENS210.cpp.
bool temp_continuous_mode | ( | bool | continuous ) |
Set the tempurature operation mode.
- Parameters:
-
single_shot True for continuous and false for single shot
- Returns:
- Write success
Definition at line 72 of file AMS_ENS210.cpp.
bool temp_continuous_mode | ( | ) |
Get the current tempurature operation mode.
- Returns:
- Write success
Definition at line 78 of file AMS_ENS210.cpp.
bool temp_has_data | ( | ) |
Get whether the sensor has collected tempurature data.
- Returns:
- Has data
Definition at line 120 of file AMS_ENS210.cpp.
bool temp_is_measuring | ( | ) |
Get whether the sensor is measuring tempurature data.
- Returns:
- Sensor activity, true for measureing, false for idle
Definition at line 106 of file AMS_ENS210.cpp.
uint16_t temp_read | ( | ) |
Get the most recent tempurature measurement, must call temp_has_data() first when in single shot mode otherwise the same data will be returned.
- Returns:
- Most recent tempurature measurement as a 16 bits unsigned value in 1/64 Kelvin
Definition at line 165 of file AMS_ENS210.cpp.
Generated on Thu Jul 14 2022 01:41:22 by
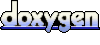