Library for the AMS CC811 digitial gas sensor
Dependencies: AMS_ENS210_temp_humid_sensor
AMS_CCS811 Class Reference
The AMS CCS811 class. More...
#include <AMS_CCS811.h>
Data Structures | |
struct | ccs811_errors |
Holds error information. More... | |
Public Types | |
enum | OP_MODES { IDLE, SECOND, TEN_SECOND, SIXTY_SECOND, CONSTANT, INVALID } |
Sensor operation modes. More... | |
Public Member Functions | |
AMS_CCS811 (I2C *i2c, PinName n_wake_pin) | |
Create an AMS_CCS811 instance. | |
AMS_CCS811 (I2C *i2c, PinName n_wake_pin, I2C *ens210_i2c) | |
Create an AMS_CCS811 instance. | |
~AMS_CCS811 () | |
Destroy the AMS_CCS811 instance. | |
bool | init () |
Initalise the sensor. | |
void | i2c_interface (I2C *i2c) |
Set the I2C interface. | |
bool | ens210_i2c_interface (I2C *i2c) |
Set the ENS210 I2C interface. | |
bool | enable_ens210 (bool enable) |
Set whether the attached AMS_ENS210 is enabled. | |
bool | ens210_is_enabled () |
Get whether the attached AMS_ENS210 is enabled. | |
void | ens210_poll_interval (int poll_ms) |
Set the AMS_ENS210 poll interval. | |
int | ens210_poll_interval () |
Get the AMS_ENS210 poll interval. | |
int | firmware_mode () |
Get the current firmware mode. | |
bool | mode (OP_MODES mode) |
Set the operation mode Notes: 1. When a sensor operating mode is changed to a new mode with a lower sample rate (e.g. from SECOND to SIXTY_SECOND), it should be placed in IDLE for at least 10 minutes before enabling the new mode. When a sensor operating mode is changed to a new mode with a higher sample rate (e.g. from SIXTY_SECOND to SECOND), there is no requirement to wait before enabling the new mode. 2. If this method fails, the state of the config register cannot be guaranteed. Check errors and ensure all config settings are as expected. | |
AMS_CCS811::OP_MODES | mode () |
Get the current power mode. | |
bool | addr_mode (bool high) |
Set the ADDR mode . | |
bool | addr_mode () |
Get the the ADDR mode. | |
bool | addr_pin (PinName pin) |
Set the ADDR pin. | |
PinName | addr_pin () |
Get the the ADDR pin. | |
bool | n_wake_pin (PinName pin) |
Set the nWAKE pin. | |
PinName | n_wake_pin () |
Get the the nWAKE pin. | |
bool | env_data (float humid, float temp) |
Set the relative humidity (%) and temperature (C). Use when AMS ENS210 is not linked. Humidity values are clipped between 0 and CCS811_MAX_HUMID. Temperature values are clipped between -25 and CCS811_MAX_TEMP. | |
int | has_new_data () |
Get the sensor collection state Use when interrupts are disabled. | |
uint16_t | co2_read () |
Get the most recent CO2 measurement. Must call has_new_data() first when when interupts are disabled otherwise the same data will be returned. | |
uint16_t | tvoc_read () |
Get the most recent TVOC measurement. Must call has_new_data() first when when interupts are disabled otherwise the same data will be returned. | |
uint16_t | raw_read () |
Get the most recent RAW data. Must call has_new_data() first when NOT in CONSTANT mode and interupts are disabled otherwise the same data will be returned. When in CONSTANT mode only this read method will return anything other than 0 or NULL. If 0 is returned, check for errors. | |
float | temp_read () |
Get the most recent Tempurature(C) measurement from the ENS210 (if attached and enabled). Will be at most as old as the ENS210 poll time. | |
float | humid_read () |
Get the most recent Relative Humidity(%) measurement from the ENS210 (if attached and enabled). Will be at most as old as the ENS210 poll time. | |
bool | error_status () |
Get current error status. | |
ccs811_errors | errors () |
Get the latest errors. | |
const char * | error_string (int err_code) |
Get the error string. | |
bool | attach (void(*func_ptr)(void), PinName pin) |
Attach a function to be called when data is ready. | |
template<typename T > | |
bool | attach (T *type_ptr, void(T::*mem_ptr)(void), PinName pin) |
Attach a member function to be called when data is ready. | |
bool | enable_interupt (bool enable) |
Set whether the data ready interupt is enabled. Note: If this method fails, the state of the config register cannot be guaranteed. Check errors and ensure all config settings are as expected. | |
int | interupt_enabled () |
Get whether the data ready interupt is enabled. | |
bool | interrupt_pin (PinName pin) |
Set the nINT pin. | |
PinName | interrupt_pin () |
Get the the nINT pin. |
Detailed Description
The AMS CCS811 class.
Definition at line 81 of file AMS_CCS811.h.
Member Enumeration Documentation
enum OP_MODES |
Sensor operation modes.
- Enumerator:
Definition at line 87 of file AMS_CCS811.h.
Constructor & Destructor Documentation
AMS_CCS811 | ( | I2C * | i2c, |
PinName | n_wake_pin | ||
) |
Create an AMS_CCS811 instance.
- Parameters:
-
i2c The I2C interface to use for communication n_wake_pin Pin nWAKE is attached to
Definition at line 4 of file AMS_CCS811.cpp.
AMS_CCS811 | ( | I2C * | i2c, |
PinName | n_wake_pin, | ||
I2C * | ens210_i2c | ||
) |
Create an AMS_CCS811 instance.
- Parameters:
-
i2c The I2C interface to use for communication n_wake_pin Pin nWAKE is attached to ens210_i2c The I2C interface for an attached AMS_ENS210
Definition at line 9 of file AMS_CCS811.cpp.
~AMS_CCS811 | ( | ) |
Destroy the AMS_CCS811 instance.
Definition at line 15 of file AMS_CCS811.cpp.
Member Function Documentation
bool addr_mode | ( | bool | high ) |
Set the ADDR mode
.
- Parameters:
-
high True sets to high, false to low
- Returns:
- Write success
Definition at line 140 of file AMS_CCS811.cpp.
bool addr_mode | ( | ) |
Get the the ADDR mode.
- Returns:
- The current ADDR mode, true for high, false for low
Definition at line 149 of file AMS_CCS811.cpp.
bool addr_pin | ( | PinName | pin ) |
Set the ADDR pin.
- Parameters:
-
pin Pin ADDR is attached to
- Returns:
- Write success
Definition at line 158 of file AMS_CCS811.cpp.
PinName addr_pin | ( | ) |
Get the the ADDR pin.
- Returns:
- The addr pin
bool attach | ( | void(*)(void) | func_ptr, |
PinName | pin | ||
) |
Attach a function to be called when data is ready.
Calling this method enables interupts
- Parameters:
-
func_ptr A pointer to the function to be called pin Pin attached to nINT
- Returns:
- Attach success
Definition at line 325 of file AMS_CCS811.h.
bool attach | ( | T * | type_ptr, |
void(T::*)(void) | mem_ptr, | ||
PinName | pin | ||
) |
Attach a member function to be called when data is ready.
Calling this method enables interupts
- Parameters:
-
type_ptr A pointer to the instance of the class mem_ptr A pointer to the member function pin Pin attached to nINT
- Returns:
- Attach success
Definition at line 341 of file AMS_CCS811.h.
uint16_t co2_read | ( | ) |
Get the most recent CO2 measurement.
Must call has_new_data() first when when interupts are disabled otherwise the same data will be returned.
- Returns:
- Most recent eCO2 measurement in ppm
Definition at line 216 of file AMS_CCS811.cpp.
bool enable_ens210 | ( | bool | enable ) |
Set whether the attached AMS_ENS210 is enabled.
If an I2C interface is not set for the ENS210, calling this method will have no effect.
- Parameters:
-
enabled True for enabled, false for disabled
- Returns:
- enabled True for enabled, false for disabled
Definition at line 76 of file AMS_CCS811.cpp.
bool enable_interupt | ( | bool | enable ) |
Set whether the data ready interupt is enabled.
Note: If this method fails, the state of the config register cannot be guaranteed.
Check errors and ensure all config settings are as expected.
- Parameters:
-
enabled True for enabled, false for disabled
- Returns:
- Write success
Definition at line 281 of file AMS_CCS811.cpp.
bool ens210_i2c_interface | ( | I2C * | i2c ) |
Set the ENS210 I2C interface.
- Parameters:
-
i2c The I2C interface for an attached AMS_ENS210
- Returns:
- Success
Definition at line 55 of file AMS_CCS811.cpp.
bool ens210_is_enabled | ( | ) |
Get whether the attached AMS_ENS210 is enabled.
- Returns:
- enabled True for enabled, false for disabled
Definition at line 86 of file AMS_CCS811.cpp.
void ens210_poll_interval | ( | int | poll_ms ) |
Set the AMS_ENS210 poll interval.
- Parameters:
-
poll_ms Poll interval in ms
Definition at line 91 of file AMS_CCS811.cpp.
int ens210_poll_interval | ( | ) |
Get the AMS_ENS210 poll interval.
- Returns:
- The poll interval in ms
Definition at line 96 of file AMS_CCS811.cpp.
bool env_data | ( | float | humid, |
float | temp | ||
) |
Set the relative humidity (%) and temperature (C).
Use when AMS ENS210 is not linked.
Humidity values are clipped between 0 and CCS811_MAX_HUMID.
Temperature values are clipped between -25 and CCS811_MAX_TEMP.
- Returns:
- Write success
Definition at line 170 of file AMS_CCS811.cpp.
bool error_status | ( | ) |
Get current error status.
- Returns:
- True when error has occured, false when no error has occured
Definition at line 236 of file AMS_CCS811.cpp.
const char * error_string | ( | int | err_code ) |
Get the error string.
- Parameters:
-
err_code Error code to be translated
- Returns:
- Error String.
Definition at line 270 of file AMS_CCS811.cpp.
AMS_CCS811::ccs811_errors errors | ( | ) |
int firmware_mode | ( | ) |
Get the current firmware mode.
- Returns:
- 1 application mode, 0 for boot mode, -1 for error
Definition at line 100 of file AMS_CCS811.cpp.
int has_new_data | ( | ) |
Get the sensor collection state Use when interrupts are disabled.
- Returns:
- Current collection state, 1 for new data ready, 0 for data not ready and -1 for error
Definition at line 186 of file AMS_CCS811.cpp.
float humid_read | ( | ) |
Get the most recent Relative Humidity(%) measurement from the ENS210 (if attached and enabled).
Will be at most as old as the ENS210 poll time.
- Returns:
- Most recent Relative Humidity(%) measurement from the ENS210
Definition at line 232 of file AMS_CCS811.cpp.
void i2c_interface | ( | I2C * | i2c ) |
Set the I2C interface.
- Parameters:
-
i2c The I2C interface to use for communication
Definition at line 51 of file AMS_CCS811.cpp.
bool init | ( | ) |
PinName interrupt_pin | ( | ) |
Get the the nINT pin.
- Returns:
- The nINT pin
bool interrupt_pin | ( | PinName | pin ) |
Set the nINT pin.
- Parameters:
-
pin Pin nINT is attached to
- Returns:
- Write success
Definition at line 304 of file AMS_CCS811.cpp.
int interupt_enabled | ( | ) |
Get whether the data ready interupt is enabled.
- Returns:
- 1 for enabled, 0 for disabled, -1 for error
Definition at line 293 of file AMS_CCS811.cpp.
bool mode | ( | OP_MODES | mode ) |
Set the operation mode
Notes:
1. When a sensor operating mode is changed to a new mode with
a lower sample rate (e.g. from SECOND to SIXTY_SECOND), it should be
placed in IDLE for at least 10 minutes before enabling the new mode.
When a sensor operating mode is changed to a new mode with a higher
sample rate (e.g. from SIXTY_SECOND to SECOND), there is no requirement
to wait before enabling the new mode.
2. If this method fails, the state of the config register cannot be guaranteed.
Check errors and ensure all config settings are as expected.
- Parameters:
-
mode OP_MODES mode to set
- Returns:
- Write success
Definition at line 113 of file AMS_CCS811.cpp.
AMS_CCS811::OP_MODES mode | ( | ) |
Get the current power mode.
- Returns:
- The current OP_MODES mode
Definition at line 126 of file AMS_CCS811.cpp.
bool n_wake_pin | ( | PinName | pin ) |
Set the nWAKE pin.
- Parameters:
-
pin Pin nWAKE is attached to
- Returns:
- Write success
Definition at line 165 of file AMS_CCS811.cpp.
PinName n_wake_pin | ( | ) |
Get the the nWAKE pin.
- Returns:
- The nWAKE pin
uint16_t raw_read | ( | ) |
Get the most recent RAW data.
Must call has_new_data() first when NOT in CONSTANT mode and interupts are disabled otherwise the same data will be returned.
When in CONSTANT mode only this read method will return anything other than 0 or NULL. If 0 is returned, check for errors.
- Returns:
- Most recent RAW data
Definition at line 224 of file AMS_CCS811.cpp.
float temp_read | ( | ) |
Get the most recent Tempurature(C) measurement from the ENS210 (if attached and enabled).
Will be at most as old as the ENS210 poll time.
- Returns:
- Most recent Tempurature(C) measurement from the ENS210
Definition at line 228 of file AMS_CCS811.cpp.
uint16_t tvoc_read | ( | ) |
Get the most recent TVOC measurement.
Must call has_new_data() first when when interupts are disabled otherwise the same data will be returned.
- Returns:
- Most recent TVOC measurement in ppb
Definition at line 220 of file AMS_CCS811.cpp.
Generated on Wed Jul 13 2022 07:08:22 by
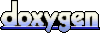