Dual CANbus monitor and instrumentation cluster
Embed:
(wiki syntax)
Show/hide line numbers
displayModes.cpp
00001 //displayModes.cpp 00002 #include "displayModes.h" 00003 00004 char sTemp1[40]; 00005 char sTemp2[16]; 00006 00007 void printLast (bool force, bool showButtons){ 00008 CANMessage msg; 00009 tt.locate(0,6); 00010 tt.foreground(Red); 00011 tt.background(Yellow); 00012 if(force) tt.cls(); // Just clear screen if forced - always update display 00013 tt.set_font((unsigned char*) Arial12x12_prop); // select the font 00014 for(int i=0; i<19; i++){ 00015 msg = lastMsg[i+indexOffset]; 00016 printf("%03x : %02x %02x %02x %02x %02x %02x %02x %02x \n",msg.id,msg.data[0],msg.data[1],msg.data[2],msg.data[3],msg.data[4],msg.data[5],msg.data[6],msg.data[7]); 00017 } 00018 if((sMode==1)&&showButtons){ 00019 tt.foreground(Yellow); 00020 tt.background(DarkCyan); 00021 tt.set_font((unsigned char*) Arial12x12); 00022 00023 showButton(0,0," <up>","",4,4); 00024 showButton(2,0,"<down>","",4,4); 00025 } 00026 } 00027 00028 void printChanged (bool force, bool showButtons){ 00029 CANMessage msg; 00030 unsigned char i,j; 00031 tt.locate(0,6); 00032 tt.foreground(Red); 00033 tt.background(Yellow); 00034 if(force) tt.cls(); // Just clear screen if forced - always update display 00035 tt.set_font((unsigned char*) Arial12x12_prop); // select the font 00036 i=0; 00037 j=indexOffset; 00038 do{ 00039 j=j<99?j+1:j; 00040 if(msgChanged[j]>0){ 00041 msg = lastMsg[j]; 00042 printf("%03x : %02x %02x %02x %02x %02x %02x %02x %02x \n",msg.id,msg.data[0],msg.data[1],msg.data[2],msg.data[3],msg.data[4],msg.data[5],msg.data[6],msg.data[7]); 00043 i++; 00044 }// if changed 00045 }while(i<19&&j<99); 00046 if((sMode==1)&&showButtons){ 00047 tt.foreground(Yellow); 00048 tt.background(DarkCyan); 00049 tt.set_font((unsigned char*) Arial12x12); 00050 00051 showButton(0,0," <up>","",4,4); 00052 showButton(2,0," <down>","",4,4); 00053 showButton(1,0," Reset","Baseline",4,4); 00054 } 00055 } 00056 00057 void printLog (bool force, bool showButtons){ 00058 static unsigned char lastDisplayLoc = 0; 00059 if(force||displayLoc!=lastDisplayLoc){ //only update if changed 00060 tt.foreground(Amber); 00061 tt.background(Black); 00062 tt.cls(); 00063 tt.locate(0,6); 00064 tt.set_font((unsigned char*) Arial12x12); 00065 for(int i=0; i<19; i++){ 00066 printf("%s",displayLog[displayLoc]); 00067 displayLoc=displayLoc>17?0:displayLoc+1; 00068 } 00069 } 00070 lastDisplayLoc=displayLoc; 00071 } 00072 00073 void mainDisplay (bool force, bool showButtons){ 00074 unsigned short gids, SOC, packV; 00075 static unsigned short lgids=0, lSOC=0, lpackV=0, maxPS=0; 00076 static unsigned char lbattTemp_x4=0; 00077 static float lkW=0, laccV=0, lmpkWh=0; 00078 CANMessage msg; 00079 00080 msg = lastMsg[indexLastMsg[0x5bc]]; //Get gids 00081 gids = (msg.data[0]<<2)+(msg.data[1]>>6); 00082 msg = lastMsg[indexLastMsg[0x55b]]; //Get SOC 00083 SOC = (msg.data[0]<<2)+(msg.data[1]>>6); 00084 msg = lastMsg[indexLastMsg[0x1db]]; //Get pack volts 00085 packV = (msg.data[2]<<2)+(msg.data[3]>>6); 00086 00087 tt.background(Navy); 00088 tt.foreground(Yellow); 00089 tt.set_font((unsigned char*) Arial28x28); 00090 if(force) tt.cls(); 00091 if(skin==ttSkin){ 00092 if(force||gids!=lgids||mpkWh[dtePeriod]!=lmpkWh){ 00093 tt.locate(10,10); 00094 printf("%4d gids \n",gids); 00095 if(debugMode){ 00096 if(pointerSep>maxPS){maxPS=pointerSep;} 00097 tt.locate(10,70); 00098 printf("%3d sep %3d max\n",pointerSep,maxPS); 00099 } 00100 tt.locate(10,40); 00101 printf("%4.1f kWh \n",(float)(gids-5)*0.075); 00102 tt.set_font((unsigned char*) SCProSB31x55); 00103 tt.foreground(Green); 00104 tt.locate(60,96); 00105 printf("%4.1f mi \n",mpkWh[dtePeriod]*((float)(gids-5)*.075)); 00106 lgids=gids; 00107 lmpkWh=mpkWh[dtePeriod]; 00108 tt.foreground(Yellow); 00109 tt.set_font((unsigned char*) Arial28x28); 00110 } 00111 if(force||SOC!=lSOC){ 00112 tt.locate(200,10); 00113 printf("%4.1f%s\n",(float)SOC/10,"% "); 00114 lSOC=SOC; 00115 } 00116 if(force||packV!=lpackV){ 00117 tt.locate(200,200); 00118 printf("%4.1fV \n",(float)packV/2); 00119 lpackV=packV; 00120 } 00121 if(force||battTemp_x4!=lbattTemp_x4){ 00122 tt.locate(200,170); 00123 printf("%4.1fF\n",(float)battTemp_x4*9/20+32); 00124 lbattTemp_x4=battTemp_x4; 00125 } 00126 if(force||accV!=laccV){ 00127 tt.locate(20,200); 00128 printf("%3.1fV \n",accV); 00129 laccV=accV; 00130 } 00131 if(force||kW[0]!=lkW){ 00132 tt.locate(180,40); 00133 printf("%3.2fkW \n",kW[0]); 00134 //printf("%3.1f mpkWh \n",mpkWh[0]); 00135 lkW=kW[0]; 00136 } 00137 }else {//if(skin==ggSkin){ 00138 if(force||gids!=lgids){ 00139 tt.locate(10,10); 00140 printf("%4d GIDs \n",gids); 00141 00142 tt.locate(40,40); // gg - add GIDs Percent of 281 00143 printf("%4.1f%s \n", (float)gids*0.355872, "% ") ; 00144 tt.locate(20,70); 00145 //printf("%4.1f kWh \n",(float)gids*0.08); // is input, not usable 00146 printf("%4.1f kwh \n",(float)gids*0.075); // gg - closer to usable 00147 00148 tt.set_font((unsigned char*) SCProSB31x55); 00149 tt.foreground(Green); 00150 //tt.locate(60,96); 00151 tt.locate(60,116); // gg - move down a little 00152 printf("%4.1f mi \n",(float)(gids-5)*0.31); // Approx for now 00153 lgids=gids; 00154 tt.foreground(Yellow); 00155 tt.set_font((unsigned char*) Arial28x28); 00156 } 00157 00158 if(force||SOC!=lSOC){ 00159 tt.locate(200,10); 00160 printf("%4.1f%s\n",(float)SOC/10,"% "); 00161 lSOC=SOC; 00162 } 00163 if(force||packV!=lpackV){ 00164 tt.locate(200,200); 00165 printf("%4.1fV \n",(float)packV/2); 00166 lpackV=packV; 00167 } 00168 if(force||accV!=laccV){ 00169 tt.locate(20,200); 00170 printf("%3.1fV \n",accV); 00171 laccV=accV; 00172 } 00173 if(force||kW[0]!=lkW){ 00174 tt.locate(160,40); // gg - move left to keep from wrap 00175 printf("%3.2fkw \n",kW[0]); // use small w to save space 00176 lkW=kW[0]; 00177 } 00178 } 00179 } 00180 00181 void braking (bool force, bool showButtons, bool prdata=false){ 00182 unsigned long targetBraking, regenBraking; 00183 static unsigned long maxTarget = 1000, maxRegen = 1000, tardivreg_x1000 = 1000; 00184 unsigned long temp; 00185 static unsigned char lastPressure[4] = {200,200,200,200}; 00186 unsigned char i,r,t; 00187 static unsigned char lr=0, lt=0; 00188 signed short steering; 00189 unsigned short s; 00190 static unsigned short ls; 00191 unsigned char throttle; 00192 static unsigned char lthrottle; 00193 short steerOutBounds = 0 ; 00194 CANMessage msg; 00195 00196 //--------------- 00197 msg = lastMsg[indexLastMsg[0x180]]; //Get Throttle position 00198 throttle = msg.data[5]; 00199 00200 // ---- steering ---- 00201 msg = lastMsg[indexLastMsg[0x002]]; //Get Steering angle 00202 steering = (msg.data[1]<<8)+msg.data[0]; 00203 00204 if(skin==ttSkin){ 00205 s= (unsigned short) ((steering/10)+155)%310; // this modulo wraps display 00206 }else{// if(skin==ggSkin){ 00207 // do not go off screen left or right. gg - steering 00208 short ss = (short) ((steering/15)+160); // less gain 10 -> 15 00209 if(ss<0) { ss=0; steerOutBounds = 1; } 00210 if(ss>310) { ss=310; steerOutBounds = 1; } 00211 s = (unsigned short) ss; 00212 } 00213 00214 //-------------- 00215 msg = lastMsg[indexLastMsg[0x1ca]]; //Get brake pressure 00216 tt.background(Navy); 00217 if (force) { 00218 tt.cls(); 00219 tt.rect(0,111,170,239,White); 00220 tt.line(0,207,170,207,White); 00221 tt.line(0,175,170,175,White); 00222 tt.line(0,143,170,143,White); 00223 lastPressure[0] = 200; 00224 lastPressure[1] = 200; 00225 lastPressure[2] = 200; 00226 lastPressure[3] = 200; 00227 } 00228 00229 // display the steering position small square 00230 if (s!=ls){ 00231 // steering position has moved 00232 //tt.fillrect(ls,5,ls+9,14, Navy); // blank old position 00233 00234 //---- gg - steering red 00235 // box is blanked by top of Braking bar, so move up 5 00236 tt.fillrect(ls,0,ls+9,9, Navy); // blank old position 00237 if( steerOutBounds != 0 ) // draw out-of-bounds as a red box 00238 tt.fillrect(s,0,s+9,9, Red); // draw out-of-bounds position 00239 else 00240 tt.fillrect(s,0,s+9,9, White); // draw new in-bounds position 00241 00242 //---- 00243 //tt.foreground(Yellow); 00244 //tt.set_font((unsigned char*) Arial28x28); 00245 //tt.locate(10,40); 00246 //printf("%d %d \n",s,ls); 00247 ls=s; 00248 } 00249 00250 if (throttle!=lthrottle){ 00251 if (throttle>239) throttle=239; 00252 if(throttle<lthrottle){ 00253 tt.fillrect(280,239-lthrottle,310,239-throttle,Navy); 00254 }else{ 00255 tt.fillrect(280,239-throttle,310,239,Yellow); 00256 } 00257 lthrottle=throttle; 00258 } 00259 00260 // plot bar graph for each wheel pressure 00261 for (i=0; i<4; i++){ 00262 if (msg.data[i]<239) { 00263 if (msg.data[i]>lastPressure[i]){ 00264 tt.fillrect(10+40*i,239-msg.data[i],40+40*i,239,Red); 00265 } else if (msg.data[i]<lastPressure[i]) { 00266 tt.fillrect(10+40*i,238-lastPressure[i],40+40*i,238-msg.data[i],Navy); 00267 } 00268 lastPressure[i]=msg.data[i]; 00269 } 00270 } 00271 00272 msg = lastMsg[indexLastMsg[0x1cb]]; //Get Target and Regen 00273 regenBraking = (msg.data[0]<<3)+(msg.data[1]>>5); 00274 targetBraking = (msg.data[2]<<3)+(msg.data[3]>>5); 00275 00276 if (targetBraking<2045){ 00277 if ((targetBraking>50)&&(regenBraking>50)){ 00278 temp = targetBraking; 00279 temp *= 1000; 00280 temp /= regenBraking; 00281 if (temp<tardivreg_x1000) tardivreg_x1000=temp; 00282 } 00283 if (targetBraking>maxTarget) maxTarget=targetBraking; 00284 if (regenBraking>maxRegen) maxRegen=regenBraking; 00285 temp = targetBraking; 00286 temp *=200; 00287 temp /= maxTarget; 00288 t = (char) temp; 00289 if (t>200) t=200; 00290 temp = regenBraking; 00291 temp *= tardivreg_x1000; 00292 temp /= maxTarget; 00293 temp /= 5; // 1000/200=5 00294 r = (char) temp; 00295 if (r>200) r=200; 00296 if(lr!=r&&prdata){ 00297 tt.foreground(Yellow); 00298 tt.set_font((unsigned char*) Arial28x28); 00299 tt.locate(100,40); 00300 printf("%d %d \n",regenBraking,maxRegen); 00301 tt.locate(100,70); 00302 printf("%3.1f (%3.1f%s) \n",(float)tardivreg_x1000/10,(float)regenBraking*tardivreg_x1000/targetBraking/10,"%"); 00303 } 00304 if(lt!=t&&prdata){ 00305 tt.foreground(Yellow); 00306 tt.set_font((unsigned char*) Arial28x28); 00307 tt.locate(100,10); 00308 printf("%d %d \n",targetBraking,maxTarget); 00309 } 00310 if (r>t) t=r; //Should never happen 00311 if((lr!=r||lt!=t)&&!prdata){ 00312 tt.fillrect(190,10,260,239-t,Navy); 00313 tt.fillrect(190,239-t,260,239-r,Red); 00314 tt.fillrect(190,239-r,260,239,Green); 00315 } 00316 lt=t; 00317 lr=r; 00318 } 00319 } 00320 00321 void cpData(bool force, bool showButtons){ 00322 short unsigned max, min, jv, i, bd; 00323 unsigned avg; 00324 if(force){ 00325 tt.foreground(White); 00326 tt.background(Navy); 00327 tt.set_font((unsigned char*) Arial12x12_prop); // select the font 00328 max=0; 00329 min=9999; 00330 avg=0; 00331 for(i=0; i<96; i++){ 00332 bd=(battData[i*2+3]<<8)+battData[i*2+4]; 00333 avg+=bd; 00334 if(bd>max) max=bd; 00335 if(bd<min) min=bd; 00336 } 00337 avg /= 96; 00338 if(min<3713) { 00339 jv=avg-(max-avg)*1.5; 00340 } else { // Only compute judgement value if min cellpair meets <= 3712mV requirement 00341 jv=0; 00342 } 00343 tt.cls(); 00344 tt.locate(0,6); 00345 // BatDataBaseG4 * 7 = 224 00346 printf(" MAX MIN AVG CVLI T1 T2 T3 T4\n %04d %04d %04d %04d %02dC %02dC %02dC %02dC\n\n", 00347 max,min,avg,jv, battData[224+5],battData[224+8],battData[224+11],battData[224+14]); 00348 tt.locate(0,36); 00349 for(i=0; i<16; i++){ 00350 printf("%02d-%02d : %04d %04d %04d %04d %04d %04d\n", 00351 i*6+1,i*6+6, 00352 (battData[i*12+3]<<8)+battData[i*12+4],(battData[i*12+5]<<8)+battData[i*12+6], 00353 (battData[i*12+7]<<8)+battData[i*12+8],(battData[i*12+9]<<8)+battData[i*12+10], 00354 (battData[i*12+11]<<8)+battData[i*12+12],(battData[i*12+13]<<8)+battData[i*12+14]); 00355 } 00356 tt.rect(8+0*41,16,40+0*41,28,Green); 00357 tt.rect(8+1*41,16,40+1*41,28,Yellow); 00358 //tt.rect(8+2*41,16,40+2*41,28,White); 00359 tt.rect(8+3*41,16,40+3*41,28,Red); 00360 for(i=0; i<96; i++){ 00361 bd=(battData[i*2+3]<<8)+battData[i*2+4]; 00362 if(bd>0){ 00363 if(bd==max) tt.rect(58+(i%6)*41,34+(int)(i/6)*12,90+(i%6)*41,46+(int)(i/6)*12,Green); 00364 //if(bd==avg) tt.rect(58+(i%6)*41,34+(int)(i/6)*12,90+(i%6)*41,46+(int)(i/6)*12,White); 00365 if(bd==min) tt.rect(58+(i%6)*41,34+(int)(i/6)*12,90+(i%6)*41,46+(int)(i/6)*12,Yellow); 00366 if(bd<jv) tt.rect(58+(i%6)*41,34+(int)(i/6)*12,90+(i%6)*41,46+(int)(i/6)*12,Red); 00367 } 00368 } 00369 showCP=false; 00370 } 00371 if((sMode==1)&&showButtons){ 00372 tt.foreground(Yellow); 00373 tt.background(DarkCyan); 00374 tt.set_font((unsigned char*) Arial12x12); 00375 00376 showButton(1,0,"Request","CP Data",4,4); 00377 } 00378 } 00379 00380 //---------------- 00381 // gg - index 00382 void showIndex(bool force, bool showButtons){ 00383 00384 if(force){ 00385 tt.foreground(White); 00386 tt.background(Navy); 00387 //tt.set_font((unsigned char*) Arial12x12_prop); // select the font 00388 00389 tt.cls(); 00390 00391 // add the buttons to GoTo to other screens 00392 00393 tt.foreground(Yellow); 00394 tt.background(DarkCyan); 00395 tt.set_font((unsigned char*) Arial12x12); 00396 00397 // top row 00398 showButton(0,0," GoTo"," Main",4,4); 00399 showButton(1,0," GoTo"," Brake",4,4); 00400 showButton(2,0," GoTo"," EFF",4,4); 00401 showButton(3,0," GoTo"," DTE",4,4); 00402 // middle row 00403 showButton(0,1," GoTo","CP Data",4,4); 00404 showButton(1,1," GoTo","CP Hist",4,4); 00405 showButton(2,1," GoTo","CP Bars",4,4); 00406 // bottom (not Nav) row 00407 showButton(0,2," GoTo"," Config",4,4); 00408 showButton(1,2," GoTo","Playback",4,4); 00409 showButton(2,2," GoTo","Set Time",4,4); 00410 showButton(3,2," GoTo"," Log",4,4); 00411 00412 showCP=false; 00413 } 00414 00415 if(sMode==1&&showButtons){ 00416 tt.foreground(Yellow); 00417 tt.background(DarkCyan); 00418 tt.set_font((unsigned char*) Arial12x12); 00419 00420 // do nothing here? 00421 } 00422 } 00423 00424 //---------------- 00425 // gg - cpbars 00426 void cpBarPlot(bool force, bool showButtons){ 00427 short unsigned max, min, jv, i, bd; 00428 unsigned avg; 00429 short unsigned nBar[96] ; // bar height over min 00430 00431 if(force){ 00432 tt.foreground(White); 00433 tt.background(Navy); 00434 tt.set_font((unsigned char*) Arial12x12_prop); // select the font 00435 max=0; 00436 min=9999; 00437 avg=0; 00438 00439 // calc each cell-pair voltage, find max and min 00440 for(i=0; i<96; i++){ 00441 bd=(battData[i*2+3]<<8)+battData[i*2+4]; 00442 nBar[i] = bd; // init to bar height 00443 avg+=bd; 00444 if(bd>max) max=bd; 00445 if(bd<min) min=bd; 00446 } 00447 avg /= 96; 00448 00449 if(min<3713) { 00450 jv=avg-(max-avg)*1.5; 00451 } else { // Only compute judgement value if min cellpair meets <= 3712mV requirement 00452 jv=0; 00453 } 00454 00455 //------------------ 00456 tt.cls(); 00457 00458 // show as vertical bar plot 00459 int xWinMin = 26; 00460 int xWinMax = 316; 00461 int yWinMin = 50; 00462 int yWinMax = 150; 00463 // draw the Bar Graph Frame, 2 pixels wide 00464 tt.rect( xWinMin-1,yWinMin-1, xWinMax+1,yWinMax+1,Red); 00465 tt.rect( xWinMin-2,yWinMin-2, xWinMax+2,yWinMax+2,Green); 00466 00467 // bar heights 00468 int height = yWinMax - yWinMin ; 00469 int iBarValMax = max - min ; // zero to N 00470 00471 //---------------- 00472 if( iBarValMax == 0 ) { 00473 // for testing 00474 min = 3501 ; 00475 //max = min + 95*2 ; // for tall values 00476 max = min + 95/4 ; // for small values 00477 avg = ( max + min ) / 2; 00478 iBarValMax = max - min ; // zero to N 00479 for(int i=0; i<96; i++) { 00480 //nBar[i] = i*2 + min ; // test tall values 00481 nBar[i] = i/4 + min ; // test small values 00482 } 00483 } 00484 //--------------- 00485 float nBarScale = float(height) / iBarValMax ; 00486 if( nBarScale < 0.1 ) nBarScale = 0.1 ; 00487 00488 // do the Bar-height scaling 00489 for(int i=0; i<96; i++){ 00490 nBar[i] -= min ; // now, 0 to N = iBinValMax 00491 nBar[i] *= nBarScale ; // scale, as needed 00492 } 00493 00494 // values, for now 00495 // BatDataBaseG4 * 7 = 224 00496 tt.locate( 0, yWinMax+40 ); 00497 printf(" MAX MIN AVG CVLI T1 T2 T3 T4\n %04d %04d %04d %04d %02dC %02dC %02dC %02dC\n\n", 00498 max,min,avg,jv, battData[224+5],battData[224+8], battData[224+11],battData[224+14]); 00499 00500 // label the X axis (approximate) 00501 tt.locate( 2, yWinMax+5); printf("%04d", min ); 00502 //tt.locate( 2, yWinMin-14 ); printf("%04d = %04d from %1.4f", max, int( height / nBarScale ) + min, nBarScale ); 00503 tt.locate( 2, yWinMin-14 ); printf("%04d = (%d) mv range.", max , max - min ); 00504 00505 //--------------- 00506 // show the bars 00507 int nBarWidth = 2 ; 00508 int nBarSpace = 1 ; // 1 for testing 00509 00510 int xPos = xWinMin + 2 ; // start one from the left 00511 00512 for( int i=0; i<96; i++) { 00513 height = nBar[i] ; 00514 if( height > 100 ) height = 100 ; // clip tops 00515 00516 // draw the bar, is always inside x-window 00517 tt.fillrect( xPos,yWinMax-height, xPos+nBarWidth-1,yWinMax, Green); 00518 00519 // tic mark the y axis each 5 00520 if(i%5 == 4){ 00521 tt.line( xPos,yWinMax+2, xPos,yWinMax+5, White); // a white tick mark 00522 tt.line( xPos+1,yWinMax+2, xPos+1,yWinMax+5, White); // a white tick mark, to widen 00523 //tt.rect( xPos,yWinMax+2, xPos+1,yWinMax+5, White); // a white 2-wide tick mark is SLOW 00524 } 00525 // label the y axis each 10 00526 if(i%10 == 9){ 00527 tt.locate( xPos-6, yWinMax+8 ); 00528 printf("%02d\n", i+1 ); 00529 } 00530 00531 // step to the next bar position 00532 xPos += nBarWidth + nBarSpace ; 00533 } 00534 00535 showCP=false; 00536 } 00537 00538 // handle the button 00539 if(sMode==1&&showButtons){ 00540 tt.foreground(Yellow); 00541 tt.background(DarkCyan); 00542 tt.set_font((unsigned char*) Arial12x12); 00543 00544 showButton(1,0,"Request","CP Data",4,4); 00545 } 00546 } 00547 00548 //---------------- 00549 // gg - hist 00550 void cpHistogram(bool force, bool showButtons){ 00551 short unsigned max, min, jv, i, bd; 00552 unsigned avg; 00553 if(force){ 00554 tt.foreground(White); 00555 tt.background(Navy); 00556 tt.set_font((unsigned char*) Arial12x12_prop); // select the font 00557 max=0; 00558 min=9999; 00559 avg=0; 00560 for(i=0; i<96; i++){ 00561 bd=(battData[i*2+3]<<8)+battData[i*2+4]; 00562 avg+=bd; 00563 if(bd>max) max=bd; 00564 if(bd<min) min=bd; 00565 } 00566 avg /= 96; 00567 if(min<3713) { 00568 jv=avg-(max-avg)*1.5; 00569 } else { // Only compute judgement value if min cellpair meets <= 3712mV requirement 00570 jv=0; 00571 } 00572 00573 //------------------ 00574 tt.cls(); 00575 00576 // show as histogram 00577 int xWinMin = 20; 00578 int xWinMax = 300; 00579 int yWinMin = 50; 00580 int yWinMax = 150; 00581 // draw the Histogram Frame, 2 pixels wide 00582 tt.rect( xWinMin-1,yWinMin-1, xWinMax+1,yWinMax+1,Red); 00583 tt.rect( xWinMin-2,yWinMin-2, xWinMax+2,yWinMax+2,Green); 00584 00585 // binning 00586 short nBin[301] ; // bins to count Min values in nBin[0], etc. 00587 int height ; 00588 int iBinIndxMax = 300 ; 00589 int iBinValMax = max - min ; // zero to N 00590 if( iBinValMax > iBinIndxMax ) iBinValMax = iBinIndxMax ; 00591 00592 // clean the bins 00593 for(int i=0; i<=iBinIndxMax; i++) { 00594 nBin[i] = 0; 00595 } 00596 00597 // do the bin counting 00598 for(int i=0; i<96; i++){ 00599 bd=(battData[i*2+3]<<8)+battData[i*2+4] - min ; 00600 if( bd > iBinValMax ) bd = iBinValMax ; 00601 nBin[bd] ++ ; 00602 } 00603 00604 //---------------- 00605 if( iBinValMax == 0 ) { 00606 // for testing 00607 min = 10 ; 00608 max = 50 ; 00609 avg = ( max + min ) / 2; 00610 iBinValMax = max - min ; 00611 for(int i=0; i<=(iBinValMax/2); i++) { 00612 nBin[i] = i ; 00613 nBin[iBinValMax-i] = i ; 00614 } 00615 } 00616 00617 // the values, for now 00618 // BatDataBaseG4 * 7 = 224 00619 tt.locate( 0, yWinMax+40 ); 00620 printf(" MAX MIN AVG CVLI T1 T2 T3 T4\n %04d %04d %04d %04d %02dC %02dC %02dC %02dC\n\n", 00621 max,min,avg,jv, battData[224+5],battData[224+8], battData[224+11],battData[224+14]); 00622 00623 //--------------- 00624 // show the bars 00625 int nBarWidth = 3 ; 00626 int nBarSpace = 1 ; // 1 for testing 00627 00628 int xPos = (xWinMin + xWinMax) / 2 ; 00629 xPos -= (avg-min) * (nBarWidth + nBarSpace) ; 00630 00631 for( int i=0; i<=iBinValMax; i++) { 00632 height = 4 * nBin[i] ; 00633 if( height > 100 ) height = 100 ; // clip tops 00634 00635 // if inside the window, draw the bar 00636 if( ( xPos + nBarWidth < xWinMax ) && ( xPos > xWinMin ) ) 00637 tt.fillrect( xPos,yWinMax-height, xPos+nBarWidth-1,yWinMax, Green); 00638 00639 // step to the next bar position 00640 xPos += nBarWidth + nBarSpace ; 00641 } 00642 00643 showCP=false; 00644 } 00645 00646 // handle the button 00647 if(sMode==1&&showButtons){ 00648 tt.foreground(Yellow); 00649 tt.background(DarkCyan); 00650 tt.set_font((unsigned char*) Arial12x12); 00651 00652 showButton(1,0,"Request","CP Data",4,4); 00653 } 00654 } 00655 00656 //--------------- 00657 void config1(bool force, bool showButtons){ 00658 if (force) { 00659 tt.background(Black); 00660 tt.cls(); 00661 } 00662 tt.foreground(Yellow); 00663 tt.background(DarkCyan); 00664 tt.set_font((unsigned char*) Arial12x12); 00665 00666 //-------- top row -------- 00667 showButton(0,0,"Calibrate"," Touch",4,4); // gg - 4x4 00668 showButton(1,0," Reset","",4,4); 00669 showButton(2,0," Save"," Config",4,4); 00670 00671 // a button to step to the next skin 00672 unsigned int nextSkin = skin + 1 ; 00673 if( nextSkin > maxSkin ) nextSkin = 0 ; 00674 00675 if( nextSkin == ttSkin ) sprintf(sTemp1,"Skin TT"); 00676 else if( nextSkin == ggSkin ) sprintf(sTemp1,"Skin GG"); 00677 else sprintf(sTemp1,"Skin %d",nextSkin); 00678 00679 showButton(3,0," Use",sTemp1,4,4); 00680 00681 //------- second row ----- 00682 if (logEn) { 00683 sprintf(sTemp1,"Disable"); 00684 } else { 00685 sprintf(sTemp1,"Enable"); 00686 } 00687 showButton(0,1,sTemp1,"Logging",4,4); 00688 00689 if (repeatPoll) { 00690 sprintf(sTemp1,"Disable"); 00691 } else { 00692 sprintf(sTemp1,"Enable"); 00693 } 00694 showButton(1,1,sTemp1,"Auto CP",4,4); 00695 00696 // add Enable/Disable Batt Log gg - yesBattLog 00697 if (yesBattLog) { 00698 sprintf(sTemp1,"Disable"); 00699 } else { 00700 sprintf(sTemp1,"Enable"); 00701 } 00702 showButton(2,1,sTemp1,"Batt Log",4,4); 00703 00704 // add Enable/Disable Debug - debugMode 00705 if (debugMode) { 00706 sprintf(sTemp1,"Disable"); 00707 } else { 00708 sprintf(sTemp1,"Enable"); 00709 } 00710 showButton(3,1,sTemp1," Debug",4,4); 00711 } 00712 00713 void pbScreen(bool force, bool showButtons){ 00714 if (force) { 00715 tt.background(Black); 00716 tt.cls(); 00717 } 00718 tt.foreground(Yellow); 00719 tt.background(DarkCyan); 00720 tt.set_font((unsigned char*) Arial12x12); 00721 if(playbackOpen){ 00722 showButton(0,0,"Slower"," <--",4,4); 00723 00724 if(playbackEn){ 00725 sprintf(sTemp1,"Pause"); 00726 }else{ 00727 sprintf(sTemp1," Run"); 00728 } 00729 sprintf(sTemp2,"%4.3f ",playbackInt); 00730 showButton(1,0,sTemp1,sTemp2,4,4); 00731 00732 showButton(2,0,"Faster"," -->",4,4); 00733 } 00734 if(playbackOpen){ 00735 sprintf(sTemp1," Stop"); 00736 }else{ 00737 sprintf(sTemp1,"Start"); 00738 } 00739 showButton(1,1,sTemp1,"Playback",4,4); 00740 } 00741 00742 void showDateTime(bool force, bool showButtons){ 00743 struct tm t; // pointer to a static tm structure 00744 time_t seconds ; 00745 tt.foreground(Yellow); 00746 tt.background(Black); 00747 if (force) { 00748 tt.cls(); 00749 seconds = time(NULL); 00750 t = *localtime(&seconds) ; 00751 00752 tt.locate(10,10); 00753 tt.set_font((unsigned char*) Arial12x12); 00754 strftime(sTemp1, 32, "%a %m/%d/%Y %X \n", &t); 00755 printf("%s",sTemp1); 00756 if((sMode==1)&&showButtons){ 00757 switch(dtMode){ 00758 case 0: 00759 sprintf(sTemp1,"Year"); 00760 break; 00761 case 1: 00762 sprintf(sTemp1,"Month"); 00763 break; 00764 case 2: 00765 sprintf(sTemp1,"Day"); 00766 break; 00767 case 3: 00768 sprintf(sTemp1,"Hour"); 00769 break; 00770 case 4: 00771 sprintf(sTemp1,"Minute"); 00772 break; 00773 case 5: 00774 sprintf(sTemp1,"Second"); 00775 break; 00776 case 6: 00777 sprintf(sTemp1,"Select"); 00778 break; 00779 default: 00780 break; 00781 } 00782 tt.background(DarkCyan); 00783 showButton(0,1,sTemp1,"",4,4); 00784 showButton(1,1," UP","",4,4); 00785 showButton(2,1," DOWN","",4,4); 00786 } 00787 } 00788 } 00789 00790 void dteDisplay(bool force, bool showButtons, bool showMiles){ 00791 unsigned short i,x,y,lx,ly,gids,radius,color,r,t; 00792 unsigned char toVal; 00793 static unsigned short lgids=0; 00794 static unsigned char leff[39]={0}; 00795 CANMessage msg; 00796 unsigned long targetBraking, regenBraking, temp; 00797 static unsigned long maxTarget = 1000, maxRegen = 1000, tardivreg_x1000 = 1000; 00798 static unsigned char lr=0, lt=0; 00799 00800 msg = lastMsg[indexLastMsg[0x5bc]]; //Get gids 00801 gids = (msg.data[0]<<2)+(msg.data[1]>>6); 00802 if(gids==0){ 00803 gids=281; // Display new, fully charged capacity until real data obtained 00804 } 00805 00806 tt.background(Navy); 00807 tt.foreground(Yellow); 00808 if(force){ 00809 tt.cls(); 00810 toVal=33; 00811 00812 x=50+0*6; 00813 tt.locate(x-10,226); 00814 printf("sec\n"); 00815 tt.line(x,10,x,220,DarkGrey); 00816 x=50+9*6; 00817 tt.locate(x-10,226); 00818 printf("min\n"); 00819 tt.line(x,10,x,220,DarkGrey); 00820 x=50+18*6; 00821 tt.locate(x-10,226); 00822 printf("hour\n"); 00823 tt.line(x,10,x,220,DarkGrey); 00824 x=50+25*6; 00825 tt.locate(x-10,226); 00826 printf("day\n"); 00827 tt.line(x,10,x,220,DarkGrey); 00828 x=50+32*6; 00829 tt.locate(x-10,226); 00830 printf("mon\n"); 00831 tt.line(x,10,x,220,DarkGrey); 00832 x=50+38*6; 00833 //tt.locate(x-10,226); 00834 //printf("year\n"); 00835 //tt.line(x,10,x,220,DarkGrey); 00836 } else { 00837 toVal=18;// no need to constantly update the long tc values 00838 } 00839 if(force||lgids!=gids){ // update Y axis when kWh changes 00840 tt.set_font((unsigned char*) Arial12x12); 00841 for(i=0;i<10;i++){ 00842 y=200-i*20; 00843 tt.locate(10,y-8); 00844 if (showMiles){ 00845 printf("%3.0f\n",i*((float)(gids-5)*.075)); 00846 }else{ 00847 printf("%d.0\n",i); 00848 } 00849 tt.line(40,y,toVal*6+56,y,DarkGrey); 00850 } 00851 lgids=gids; 00852 } 00853 if(updateDTE||force){ 00854 for(i=0;i<10;i++){ 00855 y=200-i*20; 00856 tt.line(40,y,158,y,DarkGrey); 00857 } 00858 00859 x=50+0*6; 00860 tt.line(x,10,x,220,DarkGrey); 00861 x=50+9*6; 00862 tt.line(x,10,x,220,DarkGrey); 00863 x=50+18*6; 00864 tt.line(x,10,x,220,DarkGrey); 00865 //x=50+25*6; 00866 //tt.line(x,60,x,220,DarkGrey); 00867 //x=50+32*6; 00868 //tt.line(x,60,x,220,DarkGrey); 00869 //x=50+38*6; 00870 //tt.line(x,60,x,220,DarkGrey); 00871 tt.set_font((unsigned char*) SCProSB31x55); 00872 tt.foreground(Green); 00873 if (showMiles){ 00874 float miles = mpkWh[dtePeriod]*((float)(gids-5)*.075); 00875 // Right justify 00876 if (miles>99.9){ //space=18; num=31; . = 23 00877 tt.locate(161,8); 00878 printf("%4.1f\n",miles); 00879 } else if (miles>9.9){ 00880 tt.locate(156,8); 00881 printf(" %3.1f\n",miles); 00882 } else { 00883 tt.locate(151,8); 00884 printf(" %2.1f\n",miles); 00885 } 00886 } else { 00887 tt.locate(180,10); 00888 printf("%3.1f \n",mpkWh[dtePeriod]); 00889 } 00890 lx=50; 00891 ly=mpkWh[0]*20; 00892 if(ly<200) { 00893 ly=200-ly; 00894 }else{ 00895 ly=0; 00896 } 00897 if(dtePeriod==0){ 00898 radius=6; 00899 color=Yellow; 00900 }else{ 00901 radius=2; 00902 color=Green; 00903 } 00904 tt.fillcircle(lx,leff[0],radius,Navy); 00905 tt.fillcircle(lx,ly,radius,color); 00906 00907 for(i=1;i<toVal;i++){ 00908 x=50+i*6; 00909 y=mpkWh[i]*20; 00910 if(y<200) { 00911 y=200-y; 00912 }else{ 00913 y=0; 00914 } 00915 if(i==dtePeriod){ 00916 radius=6; 00917 color=Yellow; 00918 }else{ 00919 radius=2; 00920 color=Green; 00921 } 00922 tt.fillcircle(x,leff[i],radius,Navy); 00923 tt.line(x-6,leff[i-1],x,leff[i],Navy); 00924 leff[i-1]=ly; 00925 if(y>0){ 00926 tt.fillcircle(x,y,radius,color); 00927 } 00928 tt.line(lx,ly,x,y,White); 00929 lx=x; 00930 ly=y; 00931 } 00932 leff[i-1]=y; 00933 updateDTE=false; 00934 } 00935 00936 msg = lastMsg[indexLastMsg[0x1cb]]; //Get Target and Regen 00937 regenBraking = (msg.data[0]<<3)+(msg.data[1]>>5); 00938 targetBraking = (msg.data[2]<<3)+(msg.data[3]>>5); 00939 00940 if (targetBraking<2045){ 00941 if ((targetBraking>50)&&(regenBraking>50)){ 00942 temp = targetBraking; 00943 temp *= 1000; 00944 temp /= regenBraking; 00945 if (temp<tardivreg_x1000) tardivreg_x1000=temp; 00946 } 00947 if (targetBraking>maxTarget) maxTarget=targetBraking; 00948 if (regenBraking>maxRegen) maxRegen=regenBraking; 00949 00950 temp = targetBraking; 00951 temp *=200; 00952 temp /= maxTarget; 00953 t = (char) temp; 00954 if (t>175) t=175; 00955 temp = regenBraking; 00956 temp *= tardivreg_x1000; 00957 temp /= maxTarget; 00958 temp /= 5; // 1000/200=5 00959 r = (char) temp; 00960 if (r>175) r=175; 00961 if (r>t) t=r; //Should never happen 00962 if(lr!=r||lt!=t){ 00963 tt.fillrect(264,64,310,239-t,Navy); 00964 tt.fillrect(264,239-t,310,239-r,Red); 00965 tt.fillrect(264,239-r,310,239,Green); 00966 } 00967 lt=t; 00968 lr=r; 00969 } 00970 } 00971 00972 void updateDisplay(char display){ 00973 bool changed; 00974 changed = dMode[display]!=lastDMode[display]; 00975 tt.set_display(display); 00976 switch (dMode[display]) { 00977 case logScreen: 00978 printLog(changed,(display==whichTouched)); 00979 break; 00980 case mainScreen: 00981 mainDisplay(changed,(display==whichTouched)); 00982 break; 00983 case brakeScreen: 00984 braking(changed,(display==whichTouched)); 00985 break; 00986 case dteScreen: 00987 dteDisplay(changed,(display==whichTouched),true); 00988 break; 00989 case effScreen: 00990 dteDisplay(changed,(display==whichTouched),false); 00991 break; 00992 case monitorScreen: 00993 printLast(changed,(display==whichTouched)); 00994 break; 00995 case changedScreen: 00996 printChanged(changed,(display==whichTouched)); 00997 break; 00998 case cpScreen: 00999 cpData(changed||showCP,(display==whichTouched)); 01000 break; 01001 case config1Screen: 01002 config1(changed,(display==whichTouched)); 01003 break; 01004 case playbackScreen: 01005 pbScreen(changed,(display==whichTouched)); 01006 break; 01007 case dateScreen: 01008 showDateTime(changed,(display==whichTouched)); 01009 break; 01010 case cpHistScreen: // gg - hist 01011 cpHistogram(changed||showCP,(display==whichTouched)); 01012 break; 01013 case cpBarScreen: // gg - cpbars 01014 cpBarPlot(changed||showCP,(display==whichTouched)); 01015 break; 01016 case indexScreen: 01017 showIndex(changed,(display==whichTouched)); 01018 break; 01019 default: 01020 if (changed){ 01021 tt.background(Black); 01022 tt.cls(); 01023 } 01024 break; 01025 } 01026 lastDMode[display]=dMode[display]; 01027 01028 if(display==whichTouched){ 01029 switch (sMode) { 01030 case 1: // Select screens 01031 tt.foreground(Yellow); 01032 tt.background(DarkCyan); 01033 tt.set_font((unsigned char*) Arial12x12); 01034 01035 showButton(0,tNavRow," <-Prev","",4,4); // gg - 4x4 01036 // col 1 see below 01037 showButton(2,tNavRow," Go To"," Index",4,4); // gg - index 01038 showButton(3,tNavRow," Next->","",4,4); // gg - move next 01039 01040 // col 1 in Nav row 01041 switch (dMode[display]) { 01042 case offScreen: 01043 sprintf(sTemp2," Off"); 01044 break; 01045 case logScreen: 01046 sprintf(sTemp2," Log"); 01047 break; 01048 case mainScreen: 01049 sprintf(sTemp2," Main"); 01050 break; 01051 case brakeScreen: 01052 sprintf(sTemp2,"Braking"); 01053 break; 01054 case dteScreen: 01055 sprintf(sTemp2," DTE"); 01056 break; 01057 case effScreen: 01058 sprintf(sTemp2," Eff"); 01059 break; 01060 case monitorScreen: 01061 sprintf(sTemp2," Monitor"); 01062 break; 01063 case changedScreen: 01064 sprintf(sTemp2,"DeltaMon"); 01065 break; 01066 case cpScreen: 01067 sprintf(sTemp2,"CP Data"); 01068 break; 01069 case config1Screen: 01070 sprintf(sTemp2," Config"); 01071 break; 01072 case playbackScreen: 01073 sprintf(sTemp2,"Playback"); 01074 break; 01075 case dateScreen: 01076 sprintf(sTemp2,"Set Time"); 01077 break; 01078 case cpHistScreen: // gg - hist 01079 sprintf(sTemp2,"CP Hist"); 01080 break; 01081 case cpBarScreen: // gg - cpbars 01082 sprintf(sTemp2,"CP Bars"); 01083 break; 01084 case indexScreen: // gg - index 01085 sprintf(sTemp2," Index"); 01086 break; 01087 } 01088 showButton(1,tNavRow," Select",sTemp2,4,4); 01089 01090 wait_ms(100); // pause a moment to reduce flicker 01091 break; 01092 01093 case 2: // numpad 01094 tt.foreground(Yellow); 01095 tt.background(DarkCyan); 01096 tt.set_font((unsigned char*) Arial24x23); 01097 01098 sprintf(sTemp2,""); 01099 showButton(0,0," 1",sTemp2,4,4); 01100 showButton(1,0," 2",sTemp2,4,4); 01101 showButton(2,0," 3",sTemp2,4,4); 01102 showButton(0,1," 4",sTemp2,4,4); 01103 showButton(1,1," 5",sTemp2,4,4); 01104 showButton(2,1," 6",sTemp2,4,4); 01105 showButton(0,2," 7",sTemp2,4,4); 01106 showButton(1,2," 8",sTemp2,4,4); 01107 showButton(2,2," 9",sTemp2,4,4); 01108 showButton(1,3," 0",sTemp2,4,4); 01109 01110 showButton(0,3,"<--",sTemp2,4,4); 01111 showButton(2,3,"-->",sTemp2,4,4); 01112 showButton(3,3,"return",sTemp2,4,4); 01113 case 3: 01114 break; 01115 default: 01116 break; 01117 } 01118 } 01119 } 01120 01121 //--------------------- 01122 // gg - highlight 01123 void highlightButton(unsigned char column, unsigned char row, unsigned char tScn, unsigned char columns, unsigned char rows){ 01124 01125 unsigned short x1,x2,y1,y2; 01126 01127 x1=column*(320/columns)+btnGap/2; 01128 x2=(column+1)*(320/columns)-btnGap/2; 01129 y1=row*(240/rows)+btnGap/2; 01130 y2=(row+1)*(240/rows)-btnGap/2; 01131 01132 tt.set_display(tScn); 01133 01134 if( skin == ggSkin ){ 01135 // paint the whole button box, for a better visual effect 01136 // especially on a screen with a yellow background 01137 if( tScn == 0 ) 01138 tt.fillrect(x1,y1,x2,y2,White); // DarkCyan); 01139 else 01140 tt.fillrect(x1,y1,x2,y2,Green); // DarkCyan); 01141 } else { 01142 tt.fillrect(x1,y1,x2,y2,Green); // DarkCyan); 01143 } 01144 01145 // paint the outer pixel as a yellow frame 01146 tt.rect(x1,y1,x2,y2,Yellow) ; // DarkCyan); 01147 } 01148 01149 //--------------------- 01150 void showButton(unsigned char column, unsigned char row, char * text1, char * text2, unsigned char columns, unsigned char rows){ 01151 unsigned short x1,x2,y1,y2; 01152 01153 x1=column*(320/columns)+btnGap/2; 01154 x2=(column+1)*(320/columns)-btnGap/2; 01155 y1=row*(240/rows)+btnGap/2; 01156 y2=(row+1)*(240/rows)-btnGap/2; 01157 tt.fillrect(x1,y1,x2,y2,DarkCyan); 01158 01159 // adapt formatting of text to the smaller 4x4 box 01160 tt.locate(x1+btnGap/2,y1+btnGap); // gg - 4x4 01161 printf("%s\n",text1); 01162 01163 tt.locate(x1+btnGap/2,y1+btnGap+20); 01164 printf("%s\n",text2); 01165 } 01166 01167 //------------- 01168 // below is braking screen normalized to power rather than force 01169 // changed to force since power had too large a dynamic range 01170 /*void braking (bool force, bool showButtons, bool prdata=false){ 01171 unsigned long targetBraking, regenBraking, speed; 01172 static unsigned long maxTarget = 20000, maxRegen = 20000, tardivreg_x1000 = 1000; 01173 short rpm; 01174 unsigned long temp; 01175 static unsigned char lastPressure[4] = {200,200,200,200}; 01176 unsigned char i,r,t; 01177 static unsigned char lr, lt; 01178 CANMessage msg; 01179 01180 msg = lastMsg[indexLastMsg[0x1cb]]; //Get Target and Regen 01181 regenBraking = (msg.data[0]<<3)+(msg.data[1]>>5); 01182 targetBraking = (msg.data[2]<<3)+(msg.data[3]>>5); 01183 msg = lastMsg[indexLastMsg[0x176]]; //Get rpms - not sure what this is but scales to mph with .0725 01184 rpm = ((short)msg.data[0]<<8)+msg.data[1]; 01185 speed =rpm>0?rpm>>3:-rpm>>3; //Take absolute to get speed; div8 01186 if ((targetBraking>2039)||(speed>200)) { //Filter weird messages 01187 targetBraking = 0; 01188 regenBraking = 0; 01189 } else { 01190 if ((targetBraking>50)&&(regenBraking>50)){ 01191 temp = targetBraking; 01192 temp *= 1000; 01193 temp /= regenBraking; 01194 if (temp<tardivreg_x1000) tardivreg_x1000=temp; 01195 } 01196 targetBraking *= speed; 01197 regenBraking *= speed; 01198 if (targetBraking>maxTarget) maxTarget=targetBraking; 01199 if (regenBraking>maxRegen) maxRegen=regenBraking; 01200 } 01201 01202 msg = lastMsg[indexLastMsg[0x1ca]]; //Get brake pressure 01203 tt.background(Navy); 01204 if (force) { 01205 tt.cls(); 01206 tt.rect(0,111,170,239,White); 01207 tt.line(0,207,170,207,White); 01208 tt.line(0,175,170,175,White); 01209 tt.line(0,143,170,143,White); 01210 lastPressure[0] = 200; 01211 lastPressure[1] = 200; 01212 lastPressure[2] = 200; 01213 lastPressure[3] = 200; 01214 } 01215 // plot bar graph for each wheel pressure 01216 for (i=0; i<4; i++){ 01217 if (msg.data[i]<239) { 01218 if (msg.data[i]>lastPressure[i]){ 01219 tt.fillrect(10+40*i,239-msg.data[i],40+40*i,239,Red); 01220 } else if (msg.data[i]<lastPressure[i]) { 01221 tt.fillrect(10+40*i,238-lastPressure[i],40+40*i,238-msg.data[i],Navy); 01222 } 01223 lastPressure[i]=msg.data[i]; 01224 } 01225 } 01226 01227 temp = targetBraking; 01228 temp *=200; 01229 temp /= maxTarget; 01230 t = (char) temp; 01231 if (t>200) t=200; 01232 temp = regenBraking; 01233 temp *= tardivreg_x1000; 01234 temp /= maxTarget; 01235 temp /= 5; 01236 r = (char) temp; 01237 if (r>200) r=200; 01238 if(lr!=r&&prdata){ 01239 tt.foreground(Yellow); 01240 tt.set_font((unsigned char*) Arial28x28); 01241 tt.locate(100,40); 01242 printf("%d %d \n",regenBraking,maxRegen); 01243 tt.locate(100,70); 01244 printf("%3.1f (%3.1f%s) \n",(float)tardivreg_x1000/10,(float)regenBraking*tardivreg_x1000/targetBraking/10,"%"); 01245 } 01246 if(lt!=t&&prdata){ 01247 tt.foreground(Yellow); 01248 tt.set_font((unsigned char*) Arial28x28); 01249 tt.locate(100,10); 01250 printf("%d %d \n",targetBraking,maxTarget); 01251 } 01252 if (r>t) t=r; //Should never happen 01253 if((lr!=r||lt!=t)&&!prdata){ 01254 tt.fillrect(200,10,300,239-t,Navy); 01255 tt.fillrect(200,239-t,300,239-r,Red); 01256 tt.fillrect(200,239-r,300,239,Green); 01257 } 01258 lt=t; 01259 lr=r; 01260 }*/
Generated on Wed Jul 13 2022 09:43:57 by
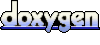