
Bouncing ball demo for the Gameduino
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "GD.h" 00003 #include "shield.h" 00004 #include "ball.h" 00005 00006 GDClass GD(ARD_MOSI, ARD_MISO, ARD_SCK, ARD_D9, USBTX, USBRX) ; 00007 00008 void setup() { 00009 GD.begin(); 00010 00011 // Background image 00012 GD.copy(RAM_PIC, bg_pic, sizeof(bg_pic)); 00013 GD.copy(RAM_CHR, bg_chr, sizeof(bg_chr)); 00014 GD.copy(RAM_PAL, bg_pal, sizeof(bg_pal)); 00015 00016 // Sprite graphics 00017 GD.uncompress(RAM_SPRIMG, ball); 00018 00019 // Palettes 0 and 1 are for the ball itself, 00020 // and palette 2 is the shadow. Set it to 00021 // all gray. 00022 int i; 00023 for (i = 0; i < 256; i++) 00024 GD.wr16(RAM_SPRPAL + (2 * (512 + i)), RGB(64, 64, 64)); 00025 00026 // Set color 255 to transparent in all three palettes 00027 GD.wr16(RAM_SPRPAL + 2 * 0xff, TRANSPARENT); 00028 GD.wr16(RAM_SPRPAL + 2 * 0x1ff, TRANSPARENT); 00029 GD.wr16(RAM_SPRPAL + 2 * 0x2ff, TRANSPARENT); 00030 } 00031 00032 #define RADIUS (112 / 2) // radius of the ball, in pixels 00033 00034 #define YBASE (300 - RADIUS) 00035 00036 int main() { 00037 setup(); 00038 while (1) { 00039 int x = 200, y = RADIUS; // ball position 00040 int xv = 2, yv = 0; // ball velocity 00041 00042 int r; // frame counter 00043 for (r = 0; ; r++) { 00044 GD.__wstartspr((r & 1) ? 256 : 0); // write sprites to other frame 00045 draw_ball(x + 15, y + 15, 2); // draw shadow using palette 2 00046 draw_ball(x, y, r & 1); // draw ball using palette 0 or 1 00047 GD.__end(); 00048 00049 // paint the new palette 00050 uint16_t palette = RAM_SPRPAL + 512 * (r & 1); 00051 byte li; 00052 for (li = 0; li < 7; li++) { 00053 byte liv = 0x90 + 0x10 * li; // brightness goes 0x90, 0xa0, etc 00054 uint16_t red = RGB(liv, 0, 0); 00055 uint16_t white = RGB(liv, liv, liv); 00056 byte i; 00057 for (i = 0; i < 32; i++) { // palette cycling using 'r' 00058 GD.wr16(palette, ((i + r) & 16) ? red : white); 00059 palette += 2; 00060 } 00061 } 00062 00063 // bounce the ball around 00064 x += xv; 00065 if ((x < RADIUS) || (x > (400 - RADIUS))) 00066 xv = -xv; 00067 y += yv; 00068 if ((yv > 0) && (y > YBASE)) { 00069 y = YBASE - (y - YBASE); // reflect in YBASE 00070 yv = -yv; // reverse Y velocity 00071 } 00072 if (0 == (r & 3)) 00073 yv++; // gravity 00074 00075 // swap frames 00076 GD.waitvblank(); 00077 GD.wr(SPR_PAGE, (r & 1)); 00078 } 00079 } 00080 }
Generated on Fri Jul 15 2022 00:15:27 by
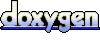