
Little domotic project: Controlling RGB color's intensity of the JUMA card with an Android App. Here's the corresponding App's source code --> https://github.com/NordineKhelfi/Android_Bluetooth_Low_Energy
Dependencies: BLE_API mbed nRF51822
Fork of BLE_GATT_Example by
main.cpp
00001 #include "mbed.h" 00002 #include "ble/BLE.h" 00003 00004 DigitalOut led(LED1, 1); 00005 Serial pc(P0_0, P0_1); 00006 00007 DigitalOut dSwitch(P0_4); 00008 00009 PwmOut ledB(P0_5); 00010 PwmOut ledG(P0_6); 00011 PwmOut ledR(P0_7); 00012 00013 uint16_t customServiceUUID = 0x1812; 00014 00015 uint16_t readCharUUID = 0xA001; 00016 uint16_t writeCharUUID = 0xA002; 00017 uint16_t writeRedCharUUID = 0xA003; 00018 uint16_t writeGreenCharUUID = 0xA004; 00019 00020 00021 const static char DEVICE_NAME[] = "GATT Example"; // change this 00022 static const uint16_t uuid16_list[] = {0xFFFF}; //Custom UUID, FFFF is reserved for development 00023 00024 00025 00026 /* Set Up custom Characteristics -----------------------------------------------------------------------------------------------------*/ 00027 static uint8_t readValue[10] = {0}; 00028 ReadOnlyArrayGattCharacteristic<uint8_t, sizeof(readValue)> readChar(readCharUUID, readValue); 00029 00030 static uint8_t writeValue[10] = {0}; 00031 WriteOnlyArrayGattCharacteristic<uint8_t, sizeof(writeValue)> writeChar(writeCharUUID, writeValue); 00032 00033 //On construit un "WriteOnlyArrayGattCharacteristic" --> writeRedChar 00034 static uint8_t writeRedValue[10] = {0}; 00035 WriteOnlyArrayGattCharacteristic<uint8_t, sizeof(writeRedValue)> writeRedChar(writeRedCharUUID, writeRedValue); 00036 00037 static uint8_t writeGreenValue[10] = {0}; 00038 WriteOnlyArrayGattCharacteristic<uint8_t, sizeof(writeGreenValue)> writeGreenChar(writeGreenCharUUID, writeGreenValue); 00039 00040 00041 /* ---------------------------------------------------------------------------------------------------------------------*/ 00042 00043 00044 /* Set up custom service */ 00045 GattCharacteristic *characteristics[] = {&readChar, &writeChar, &writeRedChar, &writeGreenChar}; 00046 GattService customService(customServiceUUID, characteristics, sizeof(characteristics) / sizeof(GattCharacteristic *)); 00047 00048 00049 /* 00050 * Restart advertising when phone app disconnects 00051 */ 00052 void disconnectionCallback(const Gap::DisconnectionCallbackParams_t *) 00053 { 00054 BLE::Instance(BLE::DEFAULT_INSTANCE).gap().startAdvertising(); 00055 } 00056 00057 /* 00058 * Handle writes to writeCharacteristic 00059 */ 00060 void writeCharCallback(const GattWriteCallbackParams *params) 00061 { 00062 /* Check to see what characteristic was written, by handle */ 00063 if(params->handle == writeChar.getValueHandle()) { 00064 00065 float fPwm = (float) ( 100 - params->data[0] ) / 100.0; 00066 pc.printf("fPwm --> %f\n", fPwm); 00067 ledB.write(fPwm); 00068 00069 /* Update the readChar with the value of writeChar */ 00070 BLE::Instance(BLE::DEFAULT_INSTANCE).gattServer().write(readChar.getValueHandle(), params->data, params->len); 00071 } 00072 /* Check to see what characteristic was written, by handle */ 00073 else if(params->handle == writeRedChar.getValueHandle()) { 00074 00075 float fPwm = (float) ( 100 - params->data[0] ) / 100.0; 00076 pc.printf("fPwm --> %f\n", fPwm); 00077 ledR.write(fPwm); 00078 00079 if(fPwm >= 0.50) 00080 dSwitch = true; 00081 else 00082 dSwitch = false; 00083 } 00084 /* Check to see what characteristic was written, by handle */ 00085 else if(params->handle == writeGreenChar.getValueHandle()) { 00086 00087 float fPwm = (float) ( 100 - params->data[0] ) / 100.0; 00088 pc.printf("fPwm --> %f\n", fPwm); 00089 ledG.write(fPwm); 00090 } 00091 } 00092 /* 00093 * Initialization callback 00094 */ 00095 void bleInitComplete(BLE::InitializationCompleteCallbackContext *params) 00096 { 00097 BLE &ble = params->ble; 00098 ble_error_t error = params->error; 00099 00100 if (error != BLE_ERROR_NONE) { 00101 return; 00102 } 00103 00104 ble.gap().onDisconnection(disconnectionCallback); 00105 ble.gattServer().onDataWritten(writeCharCallback); 00106 00107 /* Setup advertising */ 00108 ble.gap().accumulateAdvertisingPayload(GapAdvertisingData::BREDR_NOT_SUPPORTED | GapAdvertisingData::LE_GENERAL_DISCOVERABLE); // BLE only, no classic BT 00109 ble.gap().setAdvertisingType(GapAdvertisingParams::ADV_CONNECTABLE_UNDIRECTED); // advertising type 00110 ble.gap().accumulateAdvertisingPayload(GapAdvertisingData::COMPLETE_LOCAL_NAME, (uint8_t *)DEVICE_NAME, sizeof(DEVICE_NAME)); // add name 00111 ble.gap().accumulateAdvertisingPayload(GapAdvertisingData::COMPLETE_LIST_16BIT_SERVICE_IDS, (uint8_t *)uuid16_list, sizeof(uuid16_list)); // UUID's broadcast in advertising packet 00112 ble.gap().setAdvertisingInterval(100); // 100ms. 00113 00114 /* Add our custom service */ 00115 ble.addService(customService); 00116 00117 /* Start advertising */ 00118 ble.gap().startAdvertising(); 00119 } 00120 00121 /* 00122 * Main loop 00123 */ 00124 int main(void) 00125 { 00126 /* initialize stuff */ 00127 pc.printf("\n\r********* Starting Main Loop *********\n\r"); 00128 00129 ledB = 1; 00130 ledG = 1; 00131 ledR = 1; 00132 00133 dSwitch = false; 00134 00135 BLE& ble = BLE::Instance(BLE::DEFAULT_INSTANCE); 00136 ble.init(bleInitComplete); 00137 00138 /* SpinWait for initialization to complete. This is necessary because the 00139 * BLE object is used in the main loop below. */ 00140 while (ble.hasInitialized() == false) { 00141 /* spin loop */ 00142 } 00143 00144 /* Infinite loop waiting for BLE interrupt events */ 00145 while (true) { 00146 ble.waitForEvent(); /* Save power */ 00147 } 00148 }
Generated on Mon Jul 18 2022 21:54:58 by
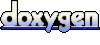