
An example program that shows how to read a Maxim DS1631 temperature sensor via the I2C bus and print the results to an LCD.
main.cpp
00001 /********************************* 00002 **Read DS1631 Temperature Sensor** 00003 ******Made by Stijn Sontrop******* 00004 **********22 Dec 2010************* 00005 **********************************/ 00006 00007 #include "mbed.h" 00008 #include <TextLCD.h> //Include the TextLCD lib (add this one to your project) 00009 00010 ///DS1631 Address: 00011 //Formation: 1001 A2 A1 A0 R/W' 00012 //Write: Make R/W' 0 00013 #define DS1631WRITEADDRESS 0x90 00014 //Read: Make R/W' 1 00015 #define DS1631READADDRESS 0x91 00016 00017 TextLCD lcd(p15, p16, p17, p18, p19, p20); //LCD config rs, e, d4-d7 00018 00019 I2C i2c(p9, p10); //SDA & SCL from I2C 00020 typedef struct { //Struct to contain read values 00021 char msb; 00022 char lsb; 00023 } temperature; 00024 00025 temperature readds1631(void) { 00026 temperature readval; 00027 i2c.start(); 00028 i2c.write(DS1631WRITEADDRESS); 00029 i2c.write(0xAA); //Read the temperature 00030 i2c.stop(); 00031 wait_ms(1); 00032 i2c.start(); 00033 i2c.write(DS1631READADDRESS); 00034 readval.msb = i2c.read(1); //Read the MSB (and do an ACK) 00035 readval.lsb = i2c.read(0); //Read the LSB (and don't do an ACK) 00036 i2c.stop(); 00037 readval.msb = readval.msb - 2; //Apparently you need to do -2 to get the right value 00038 return readval; //Return the value 00039 } 00040 00041 int main() { 00042 temperature readval; 00043 i2c.start(); 00044 i2c.write(DS1631WRITEADDRESS); //address 00045 i2c.write(0xAC); //config reg 00046 i2c.write(0x02); //config 00047 i2c.stop(); 00048 00049 //Start conversion: 00050 i2c.start(); 00051 i2c.write(DS1631WRITEADDRESS); 00052 i2c.write(0x51); //start conversion 00053 i2c.stop(); 00054 while(1) { 00055 readval = readds1631(); //Call the readds1631 function 00056 lcd.cls(); //Clear the LCD 00057 lcd.printf("Temp: %d\nLSB:%d", readval.msb, readval.lsb); 00058 wait(0.3); 00059 } 00060 }
Generated on Tue Jul 19 2022 06:41:17 by
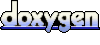