16-channel, 12-bit PWM Fm I2C-bus LED controller
Embed:
(wiki syntax)
Show/hide line numbers
PCA9685.h
00001 /** 00002 * @brief PCA9685.h 00003 * @details 16-channel, 12-bit PWM Fm+ I2C-bus LED controller. 00004 * Header file. 00005 * 00006 * 00007 * @return NA 00008 * 00009 * @author Manuel Caballero 00010 * @date 31/October/2017 00011 * @version 31/October/2017 The ORIGIN 00012 * @pre NaN. 00013 * @warning NaN 00014 * @pre This code belongs to AqueronteBlog ( http://unbarquero.blogspot.com ). 00015 */ 00016 #ifndef PCA9685_H 00017 #define PCA9685_H 00018 00019 #include "mbed.h" 00020 00021 00022 /** 00023 Example: 00024 00025 #include "mbed.h" 00026 #include "PCA9685.h" 00027 00028 PCA9685 myPWMSensor ( I2C_SDA, I2C_SCL, PCA9685::PCA9685_ADDRESS_0, 400000 ); 00029 00030 00031 Ticker newPWMOutput; 00032 DigitalOut myled(LED1); 00033 00034 PCA9685::PCA9685_status_t aux; 00035 uint8_t myState = 0; 00036 00037 00038 void changeDATA ( void ) 00039 { 00040 myState++; 00041 } 00042 00043 00044 int main() 00045 { 00046 // Reset the device 00047 aux = myPWMSensor.PCA9685_SoftReset (); 00048 wait_us ( 5 ); 00049 00050 // Configure the PWM frequency and wake up the device 00051 aux = myPWMSensor.PCA9685_SetPWM_Freq ( 1000 ); // PWM frequency: 1kHz 00052 aux = myPWMSensor.PCA9685_SetMode ( PCA9685::MODE1_SLEEP_DISABLED ); 00053 00054 00055 newPWMOutput.attach( &changeDATA, 1 ); // the address of the function to be attached ( changeDATA ) and the interval ( 1s ) 00056 00057 // Let the callbacks take care of everything 00058 while(1) { 00059 sleep(); 00060 00061 myled = 1; 00062 00063 switch ( myState ) { 00064 default: 00065 case 0: 00066 // All LEDs: Delay Time = 1% | PWM duty cycle = 99% 00067 aux = myPWMSensor.PCA9685_SetPWM_DutyCycle_AllLEDs ( 0, 99 ); 00068 00069 myState = 1; 00070 break; 00071 00072 case 1: 00073 // All LEDs: Delay Time = 100% | PWM duty cycle = 1% 00074 aux = myPWMSensor.PCA9685_SetPWM_DutyCycle_AllLEDs ( 100, 1 ); 00075 00076 myState = 2; 00077 break; 00078 00079 case 2: 00080 // LED1: Delay Time = 10% | PWM duty cycle = 30% 00081 aux = myPWMSensor.PCA9685_SetPWM_DutyCycle ( PCA9685::PCA9685_LED1, 10, 30 ); 00082 00083 myState = 0; 00084 break; 00085 } 00086 00087 myled = 0; 00088 } 00089 } 00090 */ 00091 00092 00093 /*! 00094 Library for the PCA9685 16-channel, 12-bit PWM Fm+ I2C-bus LED controller. 00095 */ 00096 class PCA9685 00097 { 00098 public: 00099 /** 00100 * @brief DEFAULT ADDRESSES. NOTE: There are a maximum of 64 possible programmable addresses using the 6 hardware 00101 * address pins. Two of these addresses, Software Reset and LED All Call, cannot be used 00102 * because their default power-up state is ON, leaving a maximum of 62 addresses. Using 00103 * other reserved addresses, as well as any other subcall address, will reduce the total 00104 * number of possible addresses even further. 00105 * 00106 * To access to a certain address just use the following method: PCA9685_ADDRESS_0 + Counter_Address 00107 * Ex: 00108 * PCA9685_ADDRESS_0 + 1 = 0x40 + 1 = 0x41 ( 0b1000001 ) 00109 * PCA9685_ADDRESS_0 + 10 = 0x40 + 10 = 0x4A ( 0b1001010 ) 00110 * and so on... 00111 */ 00112 typedef enum { 00113 PCA9685_ADDRESS_0 = ( 0x40 << 1 ) /*!< A5 A4 A3 A2 A1 A0: 000 000 */ 00114 } PCA9685_address_t; 00115 00116 00117 // REGISTERS 00118 /** 00119 * @brief REGISTER DEFINITIONS 00120 */ 00121 typedef enum { 00122 MODE1 = 0x00, /*!< Mode register 1 */ 00123 MODE2 = 0x01, /*!< Mode register 2 */ 00124 SUBADR1 = 0x02, /*!< I2C-bus subaddress 1 */ 00125 SUBADR2 = 0x03, /*!< I2C-bus subaddress 2 */ 00126 SUBADR3 = 0x04, /*!< I2C-bus subaddress 3 */ 00127 ALLCALLADR = 0x05, /*!< LED All Call I2C-bus address */ 00128 LED0_ON_L = 0x06, /*!< LED0 output and brightness control byte 0 */ 00129 LED0_ON_H = 0x07, /*!< LED0 output and brightness control byte 1 */ 00130 LED0_OFF_L = 0x08, /*!< LED0 output and brightness control byte 2 */ 00131 LED0_OFF_H = 0x09, /*!< LED0 output and brightness control byte 3 */ 00132 LED1_ON_L = 0x0A, /*!< LED1 output and brightness control byte 0 */ 00133 LED1_ON_H = 0x0B, /*!< LED1 output and brightness control byte 1 */ 00134 LED1_OFF_L = 0x0C, /*!< LED1 output and brightness control byte 2 */ 00135 LED1_OFF_H = 0x0D, /*!< LED1 output and brightness control byte 3 */ 00136 LED2_ON_L = 0x0E, /*!< LED2 output and brightness control byte 0 */ 00137 LED2_ON_H = 0x0F, /*!< LED2 output and brightness control byte 1 */ 00138 LED2_OFF_L = 0x10, /*!< LED2 output and brightness control byte 2 */ 00139 LED2_OFF_H = 0x11, /*!< LED2 output and brightness control byte 3 */ 00140 LED3_ON_L = 0x12, /*!< LED3 output and brightness control byte 0 */ 00141 LED3_ON_H = 0x13, /*!< LED3 output and brightness control byte 1 */ 00142 LED3_OFF_L = 0x14, /*!< LED3 output and brightness control byte 2 */ 00143 LED3_OFF_H = 0x15, /*!< LED3 output and brightness control byte 3 */ 00144 LED4_ON_L = 0x16, /*!< LED4 output and brightness control byte 0 */ 00145 LED4_ON_H = 0x17, /*!< LED4 output and brightness control byte 1 */ 00146 LED4_OFF_L = 0x18, /*!< LED4 output and brightness control byte 2 */ 00147 LED4_OFF_H = 0x19, /*!< LED4 output and brightness control byte 3 */ 00148 LED5_ON_L = 0x1A, /*!< LED5 output and brightness control byte 0 */ 00149 LED5_ON_H = 0x1B, /*!< LED5 output and brightness control byte 1 */ 00150 LED5_OFF_L = 0x1C, /*!< LED5 output and brightness control byte 2 */ 00151 LED5_OFF_H = 0x1D, /*!< LED5 output and brightness control byte 3 */ 00152 LED6_ON_L = 0x1E, /*!< LED6 output and brightness control byte 0 */ 00153 LED6_ON_H = 0x1F, /*!< LED6 output and brightness control byte 1 */ 00154 LED6_OFF_L = 0x20, /*!< LED6 output and brightness control byte 2 */ 00155 LED6_OFF_H = 0x21, /*!< LED6 output and brightness control byte 3 */ 00156 LED7_ON_L = 0x22, /*!< LED7 output and brightness control byte 0 */ 00157 LED7_ON_H = 0x23, /*!< LED7 output and brightness control byte 1 */ 00158 LED7_OFF_L = 0x24, /*!< LED7 output and brightness control byte 2 */ 00159 LED7_OFF_H = 0x25, /*!< LED7 output and brightness control byte 3 */ 00160 LED8_ON_L = 0x26, /*!< LED8 output and brightness control byte 0 */ 00161 LED8_ON_H = 0x27, /*!< LED8 output and brightness control byte 1 */ 00162 LED8_OFF_L = 0x28, /*!< LED8 output and brightness control byte 2 */ 00163 LED8_OFF_H = 0x29, /*!< LED8 output and brightness control byte 3 */ 00164 LED9_ON_L = 0x2A, /*!< LED9 output and brightness control byte 0 */ 00165 LED9_ON_H = 0x2B, /*!< LED9 output and brightness control byte 1 */ 00166 LED9_OFF_L = 0x2C, /*!< LED9 output and brightness control byte 2 */ 00167 LED9_OFF_H = 0x2D, /*!< LED9 output and brightness control byte 3 */ 00168 LED10_ON_L = 0x2E, /*!< LED10 output and brightness control byte 0 */ 00169 LED10_ON_H = 0x2F, /*!< LED10 output and brightness control byte 1 */ 00170 LED10_OFF_L = 0x30, /*!< LED10 output and brightness control byte 2 */ 00171 LED10_OFF_H = 0x31, /*!< LED10 output and brightness control byte 3 */ 00172 LED11_ON_L = 0x32, /*!< LED11 output and brightness control byte 0 */ 00173 LED11_ON_H = 0x33, /*!< LED11 output and brightness control byte 1 */ 00174 LED11_OFF_L = 0x34, /*!< LED11 output and brightness control byte 2 */ 00175 LED11_OFF_H = 0x35, /*!< LED11 output and brightness control byte 3 */ 00176 LED12_ON_L = 0x36, /*!< LED12 output and brightness control byte 0 */ 00177 LED12_ON_H = 0x37, /*!< LED12 output and brightness control byte 1 */ 00178 LED12_OFF_L = 0x38, /*!< LED12 output and brightness control byte 2 */ 00179 LED12_OFF_H = 0x39, /*!< LED12 output and brightness control byte 3 */ 00180 LED13_ON_L = 0x3A, /*!< LED13 output and brightness control byte 0 */ 00181 LED13_ON_H = 0x3B, /*!< LED13 output and brightness control byte 1 */ 00182 LED13_OFF_L = 0x3C, /*!< LED13 output and brightness control byte 2 */ 00183 LED13_OFF_H = 0x3D, /*!< LED13 output and brightness control byte 3 */ 00184 LED14_ON_L = 0x3E, /*!< LED14 output and brightness control byte 0 */ 00185 LED14_ON_H = 0x3F, /*!< LED14 output and brightness control byte 1 */ 00186 LED14_OFF_L = 0x40, /*!< LED14 output and brightness control byte 2 */ 00187 LED14_OFF_H = 0x41, /*!< LED14 output and brightness control byte 3 */ 00188 LED15_ON_L = 0x42, /*!< LED15 output and brightness control byte 0 */ 00189 LED15_ON_H = 0x43, /*!< LED15 output and brightness control byte 1 */ 00190 LED15_OFF_L = 0x44, /*!< LED15 output and brightness control byte 2 */ 00191 LED15_OFF_H = 0x45, /*!< LED15 output and brightness control byte 3 */ 00192 ALL_LED_ON_L = 0xFA, /*!< load all the LEDn_ON registers, byte 0 */ 00193 ALL_LED_ON_H = 0xFB, /*!< load all the LEDn_ON registers, byte 1 */ 00194 ALL_LED_OFF_L = 0xFC, /*!< load all the LEDn_OFF registers, byte 0 */ 00195 ALL_LED_OFF_H = 0xFD, /*!< load all the LEDn_OFF registers, byte 1 */ 00196 PRE_SCALE = 0xFE, /*!< prescaler for PWM output frequency */ 00197 TESTMODE = 0xFF /*!< defines the test mode to be entered */ 00198 } PCA9685_registers_t; 00199 00200 00201 00202 // LED Sub Call I2C-bus addresses 00203 /** 00204 * @brief SUBADDRESS. NOTE: At power-up, Sub Call I2C-bus addresses are disabled. PCA9685 does not send an 00205 * ACK when E2h (R/W = 0) or E3h (R/W = 1), E4h (R/W = 0) or E5h (R/W = 1), or 00206 * E8h (R/W = 0) or E9h (R/W = 1) is sent by the master. 00207 */ 00208 typedef enum { 00209 SUBADR1_REG = ( 0xE2 << 1 ), /*!< Subaddress 1 */ 00210 SUBADR2_REG = ( 0xE4 << 1 ), /*!< Subaddress 2 */ 00211 SUBADR3_REG = ( 0xE8 << 1 ) /*!< Subaddress 3 */ 00212 } PCA9685_subaddresses_t; 00213 00214 00215 00216 00217 // Software Reset I2C-bus address 00218 /** 00219 * @brief SWRST. NOTE: The Software Reset address (SWRST Call) must be used with 00220 * R/#W = logic 0. If R/#W = logic 1, the PCA9685 does not acknowledge the SWRST. 00221 */ 00222 typedef enum { 00223 GENERAL_CALL_ADDRESS = ( 0x00 << 1 ), /*!< Software reset */ 00224 SWRST = 0x06 /*!< Software reset */ 00225 } PCA9685_software_reset_t; 00226 00227 00228 00229 00230 // MODE REGISTER 1, MODE1 00231 /** 00232 * @brief RESTART 00233 */ 00234 typedef enum { 00235 MODE1_RESTART_MASK = ( 1 << 7 ), /*!< RESTART bit mask */ 00236 MODE1_RESTART_ENABLED = ( 1 << 7 ), /*!< Restart enabled */ 00237 MODE1_RESTART_DISABLED = ( 0 << 7 ) /*!< Restart disabled ( default ) */ 00238 } PCA9685_mode1_restart_t; 00239 00240 00241 /** 00242 * @brief EXTCLK 00243 */ 00244 typedef enum { 00245 MODE1_EXTCLK_MASK = ( 1 << 6 ), /*!< EXTCLK bit mask */ 00246 MODE1_EXTCLK_ENABLED = ( 1 << 6 ), /*!< Use EXTERNAL clock */ 00247 MODE1_EXTCLK_DISABLED = ( 0 << 6 ) /*!< Use INTERNAL clock ( default ) */ 00248 } PCA9685_mode1_extclk_t; 00249 00250 00251 /** 00252 * @brief AI 00253 */ 00254 typedef enum { 00255 MODE1_AI_MASK = ( 1 << 5 ), /*!< AI bit mask */ 00256 MODE1_AI_ENABLED = ( 1 << 5 ), /*!< Auto-Increment enabled */ 00257 MODE1_AI_DISABLED = ( 0 << 5 ) /*!< Auto-Increment disabled ( default ) */ 00258 } PCA9685_mode1_ai_t; 00259 00260 00261 /** 00262 * @brief SLEEP 00263 */ 00264 typedef enum { 00265 MODE1_SLEEP_MASK = ( 1 << 4 ), /*!< SLEEP bit mask */ 00266 MODE1_SLEEP_ENABLED = ( 1 << 4 ), /*!< Low power mode. Oscillator off ( default ) */ 00267 MODE1_SLEEP_DISABLED = ( 0 << 4 ) /*!< Normal mode */ 00268 } PCA9685_mode1_sleep_t; 00269 00270 00271 /** 00272 * @brief SUB1 00273 */ 00274 typedef enum { 00275 MODE1_SUB1_MASK = ( 1 << 3 ), /*!< SUB1 bit mask */ 00276 MODE1_SUB1_ENABLED = ( 1 << 3 ), /*!< PCA9685 responds to I2C-bus subaddress 1 */ 00277 MODE1_SUB1_DISABLED = ( 0 << 3 ) /*!< PCA9685 does not respond to I2C-bus subaddress 1 ( default ) */ 00278 } PCA9685_mode1_sub1_t; 00279 00280 00281 /** 00282 * @brief SUB2 00283 */ 00284 typedef enum { 00285 MODE1_SUB2_MASK = ( 1 << 2 ), /*!< SUB2 bit mask */ 00286 MODE1_SUB2_ENABLED = ( 1 << 2 ), /*!< PCA9685 responds to I2C-bus subaddress 2 */ 00287 MODE1_SUB2_DISABLED = ( 0 << 2 ) /*!< PCA9685 does not respond to I2C-bus subaddress 2 ( default ) */ 00288 } PCA9685_mode1_sub2_t; 00289 00290 00291 /** 00292 * @brief SUB3 00293 */ 00294 typedef enum { 00295 MODE1_SUB3_MASK = ( 1 << 1 ), /*!< SUB1 bit mask */ 00296 MODE1_SUB3_ENABLED = ( 1 << 1 ), /*!< PCA9685 responds to I2C-bus subaddress 3 */ 00297 MODE1_SUB3_DISABLED = ( 0 << 1 ) /*!< PCA9685 does not respond to I2C-bus subaddress 3 ( default ) */ 00298 } PCA9685_mode1_sub3_t; 00299 00300 00301 /** 00302 * @brief ALLCALL 00303 */ 00304 typedef enum { 00305 MODE1_ALLCALL_MASK = ( 1 << 0 ), /*!< ALLCALL bit mask */ 00306 MODE1_ALLCALL_ENABLED = ( 1 << 0 ), /*!< PCA9685 responds to LED All Call I2C-bus address ( default ) */ 00307 MODE1_ALLCALL_DISABLED = ( 0 << 0 ) /*!< PCA9685 does not respond to LED All Call I2C-bus address */ 00308 } PCA9685_mode1_allcall_t; 00309 00310 00311 00312 // MODE REGISTER 2, MODE2 00313 /** 00314 * @brief INVRT 00315 */ 00316 typedef enum { 00317 MODE2_INVRT_MASK = ( 1 << 4 ), /*!< INVRT bit mask */ 00318 MODE2_INVRT_ENABLED = ( 1 << 4 ), /*!< Output logic state inverted. Value to use when no external driver used. Applicable when OE = 0 */ 00319 MODE2_INVRT_DISABLED = ( 0 << 4 ) /*!< Output logic state not inverted. Value to use when external driver used. Applicable when OE = 0. ( default ) */ 00320 } PCA9685_mode2_invrt_t; 00321 00322 00323 /** 00324 * @brief OCH 00325 */ 00326 typedef enum { 00327 MODE2_OCH_MASK = ( 1 << 3 ), /*!< ALLCALL bit mask */ 00328 MODE2_OCH_OUTPUT_CHANGE_STOP_CMD = ( 0 << 3 ), /*!< Outputs change on STOP command ( default ) */ 00329 MODE2_OCH_OUTPUT_CHANGE_ACK_CMD = ( 1 << 3 ) /*!< Outputs change on ACK */ 00330 } PCA9685_mode2_och_t; 00331 00332 00333 /** 00334 * @brief OUTDRV 00335 */ 00336 typedef enum { 00337 MODE2_OUTDRV_MASK = ( 1 << 2 ), /*!< OUTDRV bit mask */ 00338 MODE2_OUTDRV_TOTEM_POLE_STRUCTURE = ( 1 << 2 ), /*!< The 16 LEDn outputs are configured with a totem pole structure ( default ) */ 00339 MODE2_OUTDRV_OPEN_DRAIN_STRUCTURE = ( 0 << 2 ) /*!< The 16 LEDn outputs are configured with an open-drain structure */ 00340 } PCA9685_mode2_outdrv_t; 00341 00342 00343 /** 00344 * @brief OUTNE 00345 */ 00346 typedef enum { 00347 MODE2_OUTNE_MASK = ( 3 << 0 ), /*!< OUTNE bit mask */ 00348 MODE2_OUTNE_LEDn_LOW = ( 0 << 0 ), /*!< When #OE = 1 (output drivers not enabled), LEDn = 0 ( default ) */ 00349 MODE2_OUTNE_LEDn_HIGH = ( 1 << 0 ), /*!< When #OE = 1 (output drivers not enabled): LEDn = 1 when OUTDRV = 1 00350 LEDn = high-impedance when OUTDRV = 0 (same as OUTNE[1:0] = 10) */ 00351 MODE2_OUTNE_LEDn_HIGH_IMPEDANCE = ( 2 << 0 ) /*!< When #OE = 1 (output drivers not enabled), LEDn = high-impedance */ 00352 } PCA9685_mode2_outne_t; 00353 00354 00355 00356 00357 /** 00358 * @brief INTERNAL CONSTANTS 00359 */ 00360 typedef enum { 00361 PCA9685_INTERNAL_CLOCK = 25000000, /*!< Internal clock frequency */ 00362 PCA9685_ADC_STEPS = 4096 /*!< ADC 12-bits */ 00363 } PCA9685_internal_parameters_t; 00364 00365 00366 typedef enum { 00367 PCA9685_LED0 = 0x00, /*!< LED0 channel */ 00368 PCA9685_LED1 = 0x01, /*!< LED1 channel */ 00369 PCA9685_LED2 = 0x02, /*!< LED2 channel */ 00370 PCA9685_LED3 = 0x03, /*!< LED3 channel */ 00371 PCA9685_LED4 = 0x04, /*!< LED4 channel */ 00372 PCA9685_LED5 = 0x05, /*!< LED5 channel */ 00373 PCA9685_LED6 = 0x06, /*!< LED6 channel */ 00374 PCA9685_LED7 = 0x07, /*!< LED7 channel */ 00375 PCA9685_LED8 = 0x08, /*!< LED8 channel */ 00376 PCA9685_LED9 = 0x09, /*!< LED9 channel */ 00377 PCA9685_LED10 = 0x0A, /*!< LED10 channel */ 00378 PCA9685_LED11 = 0x0B, /*!< LED11 channel */ 00379 PCA9685_LED12 = 0x0C, /*!< LED12 channel */ 00380 PCA9685_LED13 = 0x0D, /*!< LED13 channel */ 00381 PCA9685_LED14 = 0x0E, /*!< LED14 channel */ 00382 PCA9685_LED15 = 0x0F /*!< LED15 channel */ 00383 } PCA9685_led_channel_t ; 00384 00385 00386 00387 00388 00389 /** 00390 * @brief INTERNAL CONSTANTS 00391 */ 00392 typedef enum { 00393 PCA9685_SUCCESS = 0, 00394 PCA9685_FAILURE = 1, 00395 I2C_SUCCESS = 0 /*!< I2C communication was fine */ 00396 } PCA9685_status_t; 00397 00398 00399 // MACRO: round function 00400 #define _MYROUND( x ) ({ \ 00401 uint32_t aux_pre; \ 00402 float aux_x; \ 00403 \ 00404 aux_x = (x); \ 00405 aux_pre = (x); \ 00406 aux_x -= aux_pre; \ 00407 aux_x *= 10; \ 00408 \ 00409 if ( aux_x >= 5 ) \ 00410 aux_pre++; \ 00411 \ 00412 aux_pre; \ 00413 }) 00414 00415 00416 00417 00418 00419 /** Create an PCA9685 object connected to the specified I2C pins. 00420 * 00421 * @param sda I2C data pin 00422 * @param scl I2C clock pin 00423 * @param addr I2C slave address 00424 * @param freq I2C frequency in Hz. 00425 */ 00426 PCA9685 ( PinName sda, PinName scl, uint32_t addr, uint32_t freq ); 00427 00428 /** Delete PCA9685 object. 00429 */ 00430 ~PCA9685(); 00431 00432 /** It resets the device by software. 00433 */ 00434 PCA9685_status_t PCA9685_SoftReset ( void ); 00435 00436 /** It configures the mode of the device: Sleep or Normal operation mode. 00437 */ 00438 PCA9685_status_t PCA9685_SetMode ( PCA9685_mode1_sleep_t myMode ); 00439 00440 /** It configures a new PWM frequency. 00441 */ 00442 PCA9685_status_t PCA9685_SetPWM_Freq ( float myNewFrequency ); 00443 00444 /** It configures a new PWM duty cycle on a given LED. 00445 */ 00446 PCA9685_status_t PCA9685_SetPWM_DutyCycle ( PCA9685_led_channel_t myLEDchannel, uint8_t myDelay, uint8_t myPWM_DutyCycle ); 00447 00448 /** It configures a new PWM duty cycle on all LEDs. 00449 */ 00450 PCA9685_status_t PCA9685_SetPWM_DutyCycle_AllLEDs ( uint8_t myDelay, uint8_t myPWM_DutyCycle ); 00451 00452 /** It sets the LEDn ON. 00453 */ 00454 PCA9685_status_t PCA9685_SetLED_ON ( PCA9685_led_channel_t myLEDchannel ); 00455 00456 /** It sets the LEDn OFF. 00457 */ 00458 PCA9685_status_t PCA9685_SetLED_OFF ( PCA9685_led_channel_t myLEDchannel ); 00459 00460 /** It sets All LEDs ON. 00461 */ 00462 PCA9685_status_t PCA9685_SetAllLED_ON ( void ); 00463 00464 /** It sets All LEDs OFF. 00465 */ 00466 PCA9685_status_t PCA9685_SetAllLED_OFF ( void ); 00467 00468 /** It sets SUB1 mode. 00469 */ 00470 PCA9685_status_t PCA9685_SetSUB1 ( PCA9685_mode1_sub1_t mySUB1_mode ); 00471 00472 /** It sets SUB2 mode. 00473 */ 00474 PCA9685_status_t PCA9685_SetSUB2 ( PCA9685_mode1_sub2_t mySUB2_mode ); 00475 00476 /** It sets SUB3 mode. 00477 */ 00478 PCA9685_status_t PCA9685_SetSUB3 ( PCA9685_mode1_sub3_t mySUB3_mode ); 00479 00480 /** It sets ALLCALL mode. 00481 */ 00482 PCA9685_status_t PCA9685_SetALLCALL ( PCA9685_mode1_allcall_t myALLCALL_mode ); 00483 00484 /** It sets INVERT mode. 00485 */ 00486 PCA9685_status_t PCA9685_SetINVERT ( PCA9685_mode2_invrt_t myINVERT_mode ); 00487 00488 /** It sets OCH mode. 00489 */ 00490 PCA9685_status_t PCA9685_SetOCH ( PCA9685_mode2_och_t myOCH_mode ); 00491 00492 /** It sets OUTDRV mode. 00493 */ 00494 PCA9685_status_t PCA9685_SetOUTDRV ( PCA9685_mode2_outdrv_t myOUTDRV_mode ); 00495 00496 00497 00498 private: 00499 I2C i2c; 00500 uint32_t PCA9685_Addr; 00501 }; 00502 00503 #endif
Generated on Sun Jul 17 2022 17:06:29 by
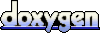