Library for I2C 4 channel relay module
Dependents: K9_Head_Controller
MultiChannelRelay.cpp
00001 /* 00002 MultiChannelRelay.h 00003 Seeed multi channel relay library 00004 00005 Copyright (c) 2018 Seeed Technology Co., Ltd. 00006 Author : lambor 00007 Create Time : June 2018 00008 Change Log : 00009 00010 This library is free software; you can redistribute it and/or 00011 modify it under the terms of the GNU Lesser General Public 00012 License as published by the Free Software Foundation; either 00013 version 2.1 of the License, or (at your option) any later version. 00014 00015 This library is distributed in the hope that it will be useful, 00016 but WITHOUT ANY WARRANTY; without even the implied warranty of 00017 MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU 00018 Lesser General Public License for more details. 00019 00020 You should have received a copy of the GNU Lesser General Public 00021 License along with this library; if not, write to the Free Software 00022 Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA 00023 */ 00024 00025 #include "MultiChannelRelay.h" 00026 00027 MultiChannelRelay::MultiChannelRelay(I2C *i2c, uint8_t slave_adr) 00028 : _i2c(i2c) 00029 //, _i2cAddr(slave_adr) 00030 { 00031 channel_state = 0; 00032 _i2cAddr = slave_adr << 1; 00033 } 00034 00035 00036 uint8_t MultiChannelRelay::getFirmwareVersion(void) 00037 { 00038 char cmd[2]; 00039 cmd[0] = CMD_READ_FIRMWARE_VER; 00040 00041 sendToDevice( cmd, 1, 1, 10 ); 00042 00043 return cmd[0]; 00044 } 00045 00046 void MultiChannelRelay::changeI2CAddress(uint8_t old_addr, uint8_t new_addr) 00047 { 00048 char cmd[2]; 00049 cmd[0] = CMD_SAVE_I2C_ADDR; 00050 cmd[1] = new_addr; 00051 00052 sendToDevice( cmd, 2, 0, 10 ); 00053 _i2cAddr = new_addr; 00054 } 00055 00056 uint8_t MultiChannelRelay::getChannelState(void) 00057 { 00058 return channel_state; 00059 } 00060 00061 void MultiChannelRelay::channelCtrl(uint8_t state) 00062 { 00063 channel_state = state; 00064 char cmd[2]; 00065 cmd[0] = CMD_CHANNEL_CTRL; 00066 cmd[1] = channel_state; 00067 00068 sendToDevice( cmd, 2, 0, 10 ); 00069 } 00070 00071 void MultiChannelRelay::turn_on_channel(uint8_t channel) 00072 { 00073 channel_state |= (1 << (channel - 1)); 00074 char cmd[2]; 00075 cmd[0] = CMD_CHANNEL_CTRL; 00076 cmd[1] = channel_state; 00077 00078 sendToDevice( cmd, 2, 0, 10 ); 00079 } 00080 00081 void MultiChannelRelay::turn_off_channel(uint8_t channel) 00082 { 00083 channel_state &= ~(1 << (channel - 1)); 00084 char cmd[2]; 00085 cmd[0] = CMD_CHANNEL_CTRL; 00086 cmd[1] = channel_state; 00087 00088 sendToDevice( cmd, 2, 0, 10 ); 00089 } 00090 00091 00092 /** Send a request to the chip, perform a wait and get response 00093 * @param cmd Pointer to character array containing request, no line endings needed 00094 * @param reqSize Size of the request in characters 00095 * @param respSize Size of the response in bytes, must be at least 1 character 00096 * @param msWait Delay between sending request and reading response in mS 00097 * @return true/false to indicate command was processed sucessfully or not, true success, false failure 00098 */ 00099 bool MultiChannelRelay::sendToDevice( char *cmd, int reqSize, int respSize, long msWait ) 00100 { 00101 bool retstat = false; 00102 int result = -10; 00103 _i2c->frequency(100000); 00104 _i2c->lock(); 00105 result = _i2c->write(_i2cAddr, cmd, reqSize); 00106 if ( result ) { 00107 _i2c->unlock(); 00108 return retstat; 00109 } 00110 00111 wait_us( msWait * 100 ); 00112 if( respSize == 0 ) { 00113 _i2c->unlock(); 00114 return true; 00115 } 00116 00117 result = _i2c->read(_i2cAddr, cmd, respSize); 00118 _i2c->unlock(); 00119 if ( result ) { 00120 } else 00121 retstat = true; 00122 00123 return retstat; 00124 } 00125 00126
Generated on Sun Aug 28 2022 09:22:22 by
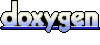