Maxbotix ultrasonic distance sensor library
Fork of MaxbotixDriver by
sonar.h
00001 #include "mbed.h" 00002 /*** @file Driver for Maxbotix sonar modules 00003 * Uses pulse width input to read range results. 00004 * @author Daniel Casner <http://www.danielcasner.org> 00005 * 00006 * Known Issues: 00007 * If a timer rollover occures during a sample, the driver will drop that sample. 00008 */ 00009 00010 /** Object representation of a sonar instance 00011 */ 00012 class Sonar { 00013 public: 00014 /** Sets up the driver and starts taking measurements 00015 * @param input The pin to read pulses from 00016 * @param t A running timer instance used to measure pulse width. 00017 * Note that the timer must have been started or results will be 00018 * invalid. 00019 */ 00020 Sonar(PinName input, Timer& t); 00021 00022 /// Returns range in cm as int 00023 int readCm(); 00024 00025 /// Returns range in uS as int 00026 int read(); 00027 00028 /// Returns the range in CM as an int 00029 operator int(); 00030 00031 bool getRange( int *outRawDistance, int *outDistance, float temperature ); 00032 00033 private: 00034 /// Inturrupt at start of pulse 00035 void pulseStart(); 00036 /// Interrupt at end of pulse 00037 void pulseStop(); 00038 00039 /// Interrupt driver for the input pin 00040 InterruptIn interrupt; 00041 /// Reference to global timer instance 00042 Timer& time; 00043 /// Time of the start of the current pulse 00044 int pulseStartTime; 00045 /// The most recent sample 00046 int range; 00047 };
Generated on Fri Jul 29 2022 10:14:03 by
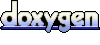