
Bluetooth Low Energy for Smart Plug
Dependencies: BLE_API mbed nRF51822
Fork of SmartPlugBLE by
SmartPlugService.cpp
00001 #include "ble/BLE.h" 00002 #include "mbed.h" 00003 #include "SmartPlugService.h" 00004 #include "SmartPlugBLE.h" 00005 00006 00007 00008 void convert(uint8_t* res,uint32_t &num) 00009 { 00010 res[3] = (num&0xFF000000)>>24; 00011 res[2] = (num&0x00FF0000)>>16; 00012 res[1] = (num&0x0000FF00)>>8; 00013 res[0] = num&0x000000FF; 00014 } 00015 00016 SmartPlugService::SmartPlugService(BLE &_ble, SmartPlugBLE &sys): 00017 ble(_ble), led(LED3,1),system(sys), 00018 voltageChar(SPS_UUID_VOLTAGE_CHAR,&voltage,GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_NOTIFY), 00019 currentChar(SPS_UUID_CURERNT_CHAR,¤t,GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_NOTIFY), 00020 powerChar(SPS_UUID_POWER_CHAR,&power,GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_NOTIFY), 00021 powerFactorChar(SPS_UUID_POWER_FACTOR_CHAR,&powerFactor,GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_NOTIFY), 00022 energyChar(SPS_UUID_ENERGY_CHAR,&energy,GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_NOTIFY), 00023 relayChar(SPS_UUID_RELAY_CHAR, relayValue.getDataPointer(), 00024 relayValue.getLenBytes(), RelayValueBytes::MAX_SIZE_BYTES, 00025 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_WRITE | GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_NOTIFY | 00026 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_READ), 00027 updateChar(SPS_UUID_UPDATE_CHAR,NULL) 00028 { 00029 system.addObserver(this); 00030 } 00031 00032 void SmartPlugService::onDataWritten(const GattWriteCallbackParams *params) 00033 { 00034 if(params->handle == updateChar.getValueHandle()) 00035 { 00036 system.updateData(); 00037 } 00038 else if(params->handle == relayChar.getValueHandle()) 00039 { 00040 system.onRelayWrite(params->data); 00041 } 00042 } 00043 00044 void SmartPlugService::updateObserver(void* data) 00045 { 00046 SmartPlug* sp = (SmartPlug*)data; 00047 updateVoltage(sp->getVoltage()); 00048 updateCurrent(sp->getCurrent()); 00049 updatePower(sp->getPower()); 00050 updatePowerFactor(sp->getPowerFactor()); 00051 updateEnergy(sp->getEnergy()); 00052 updateRelay(sp->getRelay()); 00053 } 00054 00055 void SmartPlugService::updateVoltage(uint32_t v) 00056 { 00057 if (ble.getGapState().connected) 00058 { 00059 convert(voltage,v); 00060 ble.updateCharacteristicValue(voltageChar.getValueHandle(),voltage,sizeof(voltage)); 00061 } 00062 } 00063 00064 void SmartPlugService::updateCurrent(uint32_t c) 00065 { 00066 if (ble.getGapState().connected) 00067 { 00068 convert(current,c); 00069 ble.updateCharacteristicValue(currentChar.getValueHandle(),(current),sizeof(current)); 00070 } 00071 } 00072 00073 void SmartPlugService::updatePower(uint32_t p) 00074 { 00075 if (ble.getGapState().connected) 00076 { 00077 convert(power,p); 00078 ble.updateCharacteristicValue(powerChar.getValueHandle(),(power),sizeof(power)); 00079 } 00080 } 00081 00082 void SmartPlugService::updatePowerFactor(uint32_t pf) 00083 { 00084 if (ble.getGapState().connected) 00085 { 00086 convert(powerFactor,pf); 00087 ble.updateCharacteristicValue(powerFactorChar.getValueHandle(),(powerFactor),sizeof(powerFactor)); 00088 } 00089 } 00090 00091 void SmartPlugService::updateEnergy(uint32_t e) 00092 { 00093 if(ble.getGapState().connected) 00094 { 00095 convert(energy,e); 00096 ble.updateCharacteristicValue(energyChar.getValueHandle(),energy,sizeof(energy)); 00097 } 00098 } 00099 00100 void SmartPlugService::updateRelay(Relay* relay) 00101 { 00102 if (ble.getGapState().connected) 00103 { 00104 relayValue.updateData(relay); 00105 ble.updateCharacteristicValue(relayChar.getValueHandle(),relayValue.getDataPointer(), 00106 relayValue.getLenBytes()); 00107 } 00108 } 00109 00110 void SmartPlugService::setupService(void) 00111 { 00112 00113 static bool serviceAdded = false; /* We should only ever need to add the service once. */ 00114 if (serviceAdded) 00115 { 00116 return; 00117 } 00118 00119 00120 GattCharacteristic *charTable[] = {&voltageChar,¤tChar,&powerChar, 00121 &powerFactorChar,&energyChar,&relayChar, 00122 &updateChar 00123 }; 00124 GattService smartPlugService = GattService(SPS_UUID_SERVICE, charTable, sizeof(charTable) / sizeof(GattCharacteristic *)); 00125 ble.addService(smartPlugService); 00126 00127 }
Generated on Fri Jul 22 2022 14:22:22 by
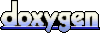