Software serial, for when you are out of serial pins
Dependents: BufferedSoftSerial neurGAI_Seeed_BLUETOOTH LPC-SD-35 ESP-WROOM-02_test ... more
SoftSerial_rx.cpp
00001 #include "SoftSerial.h" 00002 00003 uint32_t overhead_us = 200 * 1000000 / SystemCoreClock; //Random estimation of the overhead of mbed libs, makes slow devices like LPC812 @ 12MHz perform better 00004 00005 int SoftSerial::_getc( void ) { 00006 while(!readable()); 00007 out_valid = false; 00008 return out_buffer; 00009 } 00010 00011 int SoftSerial::readable(void) { 00012 return out_valid; 00013 } 00014 00015 //Start receiving byte 00016 void SoftSerial::rx_gpio_irq_handler(void) { 00017 rxticker.prime(); 00018 rxticker.setNext(bit_period + (bit_period >> 1) - overhead_us); 00019 rx->fall(NULL); 00020 rx_bit = 0; 00021 rx_error = false; 00022 }; 00023 00024 void SoftSerial::rx_handler(void) { 00025 //Receive data 00026 int val = rx->read(); 00027 00028 rxticker.setNext(bit_period); 00029 rx_bit++; 00030 00031 00032 if (rx_bit <= _bits) { 00033 read_buffer |= val << (rx_bit - 1); 00034 return; 00035 } 00036 00037 //Receive parity 00038 bool parity_count; 00039 if (rx_bit == _bits + 1) { 00040 switch (_parity) { 00041 case Forced1: 00042 if (val == 0) 00043 rx_error = true; 00044 return; 00045 case Forced0: 00046 if (val == 1) 00047 rx_error = true; 00048 return; 00049 case Even: 00050 case Odd: 00051 parity_count = val; 00052 for (int i = 0; i<_bits; i++) { 00053 if (((read_buffer >> i) & 0x01) == 1) 00054 parity_count = !parity_count; 00055 } 00056 if ((parity_count) && (_parity == Even)) 00057 rx_error = true; 00058 if ((!parity_count) && (_parity == Odd)) 00059 rx_error = true; 00060 return; 00061 } 00062 } 00063 00064 //Receive stop 00065 if (rx_bit < _bits + (bool)_parity + _stop_bits) { 00066 if (!val) 00067 rx_error = true; 00068 return; 00069 } 00070 00071 //The last stop bit 00072 if (!val) 00073 rx_error = true; 00074 00075 if (!rx_error) { 00076 out_valid = true; 00077 out_buffer = read_buffer; 00078 fpointer[RxIrq].call(); 00079 } 00080 read_buffer = 0; 00081 rxticker.detach(); 00082 rx->fall(this, &SoftSerial::rx_gpio_irq_handler); 00083 } 00084
Generated on Wed Jul 13 2022 05:29:29 by
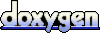