Software serial, for when you are out of serial pins
Dependents: BufferedSoftSerial neurGAI_Seeed_BLUETOOTH LPC-SD-35 ESP-WROOM-02_test ... more
SoftSerial.h
00001 #ifndef SOFTSERIAL_H 00002 #define SOFTSERIAL_H 00003 00004 #include "mbed.h" 00005 #include "SoftSerial_Ticker.h" 00006 /** A software serial implementation 00007 * 00008 */ 00009 class SoftSerial: public Stream { 00010 00011 public: 00012 /** 00013 * Constructor 00014 * 00015 * @param TX Name of the TX pin, NC for not connected 00016 * @param RX Name of the RX pin, NC for not connected, must be capable of being InterruptIn 00017 * @param name Name of the connection 00018 */ 00019 SoftSerial(PinName TX, PinName RX, const char* name = NULL); 00020 virtual ~SoftSerial(); 00021 00022 /** Set the baud rate of the serial port 00023 * 00024 * @param baudrate The baudrate of the serial port (default = 9600). 00025 */ 00026 void baud(int baudrate); 00027 00028 enum Parity { 00029 None = 0, 00030 Odd, 00031 Even, 00032 Forced1, 00033 Forced0 00034 }; 00035 00036 enum IrqType { 00037 RxIrq = 0, 00038 TxIrq 00039 }; 00040 00041 /** Set the transmission format used by the serial port 00042 * 00043 * @param bits The number of bits in a word (default = 8) 00044 * @param parity The parity used (SerialBase::None, SerialBase::Odd, SerialBase::Even, SerialBase::Forced1, SerialBase::Forced0; default = SerialBase::None) 00045 * @param stop The number of stop bits (default = 1) 00046 */ 00047 void format(int bits=8, Parity parity=SoftSerial::None, int stop_bits=1); 00048 00049 /** Determine if there is a character available to read 00050 * 00051 * @returns 00052 * 1 if there is a character available to read, 00053 * 0 otherwise 00054 */ 00055 int readable(); 00056 00057 /** Determine if there is space available to write a character 00058 * 00059 * @returns 00060 * 1 if there is space to write a character, 00061 * 0 otherwise 00062 */ 00063 int writeable(); 00064 00065 /** Attach a function to call whenever a serial interrupt is generated 00066 * 00067 * @param fptr A pointer to a void function, or 0 to set as none 00068 * @param type Which serial interrupt to attach the member function to (Seriall::RxIrq for receive, TxIrq for transmit buffer empty) 00069 */ 00070 void attach(void (*fptr)(void), IrqType type=RxIrq) { 00071 fpointer[type].attach(fptr); 00072 } 00073 00074 /** Attach a member function to call whenever a serial interrupt is generated 00075 * 00076 * @param tptr pointer to the object to call the member function on 00077 * @param mptr pointer to the member function to be called 00078 * @param type Which serial interrupt to attach the member function to (Seriall::RxIrq for receive, TxIrq for transmit buffer empty) 00079 */ 00080 template<typename T> 00081 void attach(T* tptr, void (T::*mptr)(void), IrqType type=RxIrq) { 00082 fpointer[type].attach(tptr, mptr); 00083 } 00084 00085 /** Generate a break condition on the serial line 00086 */ 00087 void send_break(); 00088 00089 protected: 00090 DigitalOut *tx; 00091 InterruptIn *rx; 00092 00093 bool tx_en, rx_en; 00094 int bit_period; 00095 int _bits, _stop_bits, _total_bits; 00096 Parity _parity; 00097 00098 FunctionPointer fpointer[2]; 00099 00100 //rx 00101 void rx_gpio_irq_handler(void); 00102 void rx_handler(void); 00103 int read_buffer, rx_bit; 00104 volatile int out_buffer; 00105 volatile bool out_valid; 00106 bool rx_error; 00107 FlexTicker rxticker; 00108 00109 //tx 00110 void tx_handler(void); 00111 void prepare_tx(int c); 00112 FlexTicker txticker; 00113 int _char; 00114 volatile int tx_bit; 00115 00116 00117 00118 virtual int _getc(); 00119 virtual int _putc(int c); 00120 }; 00121 00122 00123 #endif 00124
Generated on Wed Jul 13 2022 05:29:29 by
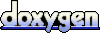