ZBar bar code reader . http://zbar.sourceforge.net/ ZBar is licensed under the GNU LGPL 2.1 to enable development of both open source and commercial projects.
Dependents: GR-PEACH_Camera_in_barcode levkov_ov7670
symbol.h
00001 /*------------------------------------------------------------------------ 00002 * Copyright 2007-2009 (c) Jeff Brown <spadix@users.sourceforge.net> 00003 * 00004 * This file is part of the ZBar Bar Code Reader. 00005 * 00006 * The ZBar Bar Code Reader is free software; you can redistribute it 00007 * and/or modify it under the terms of the GNU Lesser Public License as 00008 * published by the Free Software Foundation; either version 2.1 of 00009 * the License, or (at your option) any later version. 00010 * 00011 * The ZBar Bar Code Reader is distributed in the hope that it will be 00012 * useful, but WITHOUT ANY WARRANTY; without even the implied warranty 00013 * of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00014 * GNU Lesser Public License for more details. 00015 * 00016 * You should have received a copy of the GNU Lesser Public License 00017 * along with the ZBar Bar Code Reader; if not, write to the Free 00018 * Software Foundation, Inc., 51 Franklin St, Fifth Floor, 00019 * Boston, MA 02110-1301 USA 00020 * 00021 * http://sourceforge.net/projects/zbar 00022 *------------------------------------------------------------------------*/ 00023 #ifndef _SYMBOL_H_ 00024 #define _SYMBOL_H_ 00025 00026 #include <stdlib.h> 00027 #include <zbar.h> 00028 #include "refcnt.h" 00029 00030 typedef struct point_s { 00031 int x, y; 00032 } point_t; 00033 00034 struct zbar_symbol_set_s { 00035 refcnt_t refcnt; 00036 int nsyms; /* number of filtered symbols */ 00037 zbar_symbol_t *head; /* first of decoded symbol results */ 00038 zbar_symbol_t *tail; /* last of unfiltered symbol results */ 00039 }; 00040 00041 struct zbar_symbol_s { 00042 zbar_symbol_type_t type; /* symbol type */ 00043 unsigned int data_alloc; /* allocation size of data */ 00044 unsigned int datalen; /* length of binary symbol data */ 00045 char *data; /* symbol data */ 00046 00047 unsigned pts_alloc; /* allocation size of pts */ 00048 unsigned npts; /* number of points in location polygon */ 00049 point_t *pts; /* list of points in location polygon */ 00050 00051 refcnt_t refcnt; /* reference count */ 00052 zbar_symbol_t *next; /* linked list of results (or siblings) */ 00053 zbar_symbol_set_t *syms; /* components of composite result */ 00054 unsigned long time; /* relative symbol capture time */ 00055 int cache_count; /* cache state */ 00056 int quality; /* relative symbol reliability metric */ 00057 }; 00058 00059 extern void _zbar_symbol_free(zbar_symbol_t*); 00060 00061 extern zbar_symbol_set_t *_zbar_symbol_set_create(void); 00062 extern void _zbar_symbol_set_free(zbar_symbol_set_t*); 00063 00064 static inline void sym_add_point (zbar_symbol_t *sym, 00065 int x, 00066 int y) 00067 { 00068 int i = sym->npts; 00069 if(++sym->npts >= sym->pts_alloc) 00070 sym->pts = realloc(sym->pts, ++sym->pts_alloc * sizeof(point_t)); 00071 sym->pts[i].x = x; 00072 sym->pts[i].y = y; 00073 } 00074 00075 static inline void _zbar_symbol_refcnt (zbar_symbol_t *sym, 00076 int delta) 00077 { 00078 if(!_zbar_refcnt(&sym->refcnt, delta) && delta <= 0) 00079 _zbar_symbol_free(sym); 00080 } 00081 00082 static inline void _zbar_symbol_set_add (zbar_symbol_set_t *syms, 00083 zbar_symbol_t *sym) 00084 { 00085 sym->next = syms->head; 00086 syms->head = sym; 00087 syms->nsyms++; 00088 00089 _zbar_symbol_refcnt(sym, 1); 00090 } 00091 00092 #endif 00093
Generated on Tue Jul 12 2022 18:54:12 by
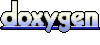