ZBar bar code reader . http://zbar.sourceforge.net/ ZBar is licensed under the GNU LGPL 2.1 to enable development of both open source and commercial projects.
Dependents: GR-PEACH_Camera_in_barcode levkov_ov7670
qrdec.h
00001 /*Copyright (C) 2008-2009 Timothy B. Terriberry (tterribe@xiph.org) 00002 You can redistribute this library and/or modify it under the terms of the 00003 GNU Lesser General Public License as published by the Free Software 00004 Foundation; either version 2.1 of the License, or (at your option) any later 00005 version.*/ 00006 #if !defined(_qrdec_H) 00007 # define _qrdec_H (1) 00008 00009 #include <zbar.h> 00010 00011 typedef struct qr_code_data_entry qr_code_data_entry; 00012 typedef struct qr_code_data qr_code_data; 00013 typedef struct qr_code_data_list qr_code_data_list; 00014 00015 typedef enum qr_mode{ 00016 /*Numeric digits ('0'...'9').*/ 00017 QR_MODE_NUM=1, 00018 /*Alphanumeric characters ('0'...'9', 'A'...'Z', plus the punctuation 00019 ' ', '$', '%', '*', '+', '-', '.', '/', ':').*/ 00020 QR_MODE_ALNUM, 00021 /*Structured-append header.*/ 00022 QR_MODE_STRUCT, 00023 /*Raw 8-bit bytes.*/ 00024 QR_MODE_BYTE, 00025 /*FNC1 marker (for more info, see http://www.mecsw.com/specs/uccean128.html). 00026 In the "first position" data is formatted in accordance with GS1 General 00027 Specifications.*/ 00028 QR_MODE_FNC1_1ST, 00029 /*Mode 6 reserved?*/ 00030 /*Extended Channel Interpretation code.*/ 00031 QR_MODE_ECI=7, 00032 /*SJIS kanji characters.*/ 00033 QR_MODE_KANJI, 00034 /*FNC1 marker (for more info, see http://www.mecsw.com/specs/uccean128.html). 00035 In the "second position" data is formatted in accordance with an industry 00036 application as specified by AIM Inc.*/ 00037 QR_MODE_FNC1_2ND 00038 }qr_mode; 00039 00040 /*Check if a mode has a data buffer associated with it. 00041 Currently this is only modes with exactly one bit set.*/ 00042 #define QR_MODE_HAS_DATA(_mode) (!((_mode)&(_mode)-1)) 00043 00044 /*ECI may be used to signal a character encoding for the data.*/ 00045 typedef enum qr_eci_encoding{ 00046 /*GLI0 is like CP437, but the encoding is reset at the beginning of each 00047 structured append symbol.*/ 00048 QR_ECI_GLI0, 00049 /*GLI1 is like ISO8859_1, but the encoding is reset at the beginning of each 00050 structured append symbol.*/ 00051 QR_ECI_GLI1, 00052 /*The remaining encodings do not reset at the start of the next structured 00053 append symbol.*/ 00054 QR_ECI_CP437, 00055 /*Western European.*/ 00056 QR_ECI_ISO8859_1, 00057 /*Central European.*/ 00058 QR_ECI_ISO8859_2, 00059 /*South European.*/ 00060 QR_ECI_ISO8859_3, 00061 /*North European.*/ 00062 QR_ECI_ISO8859_4, 00063 /*Cyrillic.*/ 00064 QR_ECI_ISO8859_5, 00065 /*Arabic.*/ 00066 QR_ECI_ISO8859_6, 00067 /*Greek.*/ 00068 QR_ECI_ISO8859_7, 00069 /*Hebrew.*/ 00070 QR_ECI_ISO8859_8, 00071 /*Turkish.*/ 00072 QR_ECI_ISO8859_9, 00073 /*Nordic.*/ 00074 QR_ECI_ISO8859_10, 00075 /*Thai.*/ 00076 QR_ECI_ISO8859_11, 00077 /*There is no ISO/IEC 8859-12.*/ 00078 /*Baltic rim.*/ 00079 QR_ECI_ISO8859_13=QR_ECI_ISO8859_11+2, 00080 /*Celtic.*/ 00081 QR_ECI_ISO8859_14, 00082 /*Western European with euro.*/ 00083 QR_ECI_ISO8859_15, 00084 /*South-Eastern European (with euro).*/ 00085 QR_ECI_ISO8859_16, 00086 /*ECI 000019 is reserved?*/ 00087 /*Shift-JIS.*/ 00088 QR_ECI_SJIS=20 00089 }qr_eci_encoding; 00090 00091 00092 /*A single unit of parsed QR code data.*/ 00093 struct qr_code_data_entry{ 00094 /*The mode of this data block.*/ 00095 qr_mode mode; 00096 union{ 00097 /*Data buffer for modes that have one.*/ 00098 struct{ 00099 unsigned char *buf; 00100 int len; 00101 }data; 00102 /*Decoded "Extended Channel Interpretation" data.*/ 00103 unsigned eci; 00104 /*Structured-append header data.*/ 00105 struct{ 00106 unsigned char sa_index; 00107 unsigned char sa_size; 00108 unsigned char sa_parity; 00109 }sa; 00110 }payload; 00111 }; 00112 00113 00114 00115 /*Low-level QR code data.*/ 00116 struct qr_code_data{ 00117 /*The decoded data entries.*/ 00118 qr_code_data_entry *entries; 00119 int nentries; 00120 /*The code version (1...40).*/ 00121 unsigned char version; 00122 /*The ECC level (0...3, corresponding to 'L', 'M', 'Q', and 'H').*/ 00123 unsigned char ecc_level; 00124 /*Structured-append information.*/ 00125 /*The index of this code in the structured-append group. 00126 If sa_size is zero, this is undefined.*/ 00127 unsigned char sa_index; 00128 /*The size of the structured-append group, or 0 if there was no S-A header.*/ 00129 unsigned char sa_size; 00130 /*The parity of the entire structured-append group. 00131 If sa_size is zero, this is undefined.*/ 00132 unsigned char sa_parity; 00133 /*The parity of this code. 00134 If sa_size is zero, this is undefined.*/ 00135 unsigned char self_parity; 00136 /*An approximate bounding box for the code. 00137 Points appear in the order up-left, up-right, down-left, down-right, 00138 relative to the orientation of the QR code.*/ 00139 qr_point bbox[4]; 00140 }; 00141 00142 00143 struct qr_code_data_list{ 00144 qr_code_data *qrdata; 00145 int nqrdata; 00146 int cqrdata; 00147 }; 00148 00149 00150 /*Extract symbol data from a list of QR codes and attach to the image. 00151 All text is converted to UTF-8. 00152 Any structured-append group that does not have all of its members is decoded 00153 as ZBAR_PARTIAL with ZBAR_PARTIAL components for the discontinuities. 00154 Note that isolated members of a structured-append group may be decoded with 00155 the wrong character set, since the correct setting cannot be propagated 00156 between codes. 00157 Return: The number of symbols which were successfully extracted from the 00158 codes; this will be at most the number of codes.*/ 00159 int qr_code_data_list_extract_text(const qr_code_data_list *_qrlist, 00160 zbar_image_scanner_t *iscn, 00161 zbar_image_t *img); 00162 00163 00164 /*TODO: Parse DoCoMo standard barcode data formats. 00165 See http://www.nttdocomo.co.jp/english/service/imode/make/content/barcode/function/application/ 00166 for details.*/ 00167 00168 #endif 00169
Generated on Tue Jul 12 2022 18:54:12 by
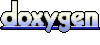