libuav original
Dependents: UAVCAN UAVCAN_Subscriber
NodeInfoRetriever Class Reference
This class automatically retrieves a response to GetNodeInfo once a node appears online or restarts. More...
#include <node_info_retriever.hpp>
Inherits uavcan::NodeStatusMonitor, and uavcan::TimerBase.
Public Member Functions | |
int | start (const TransferPriority priority=TransferPriority::OneHigherThanLowest) |
Starts the retriever. | |
void | invalidateAll () |
This method forces the class to re-request uavcan.protocol.GetNodeInfo from all nodes as if they have just appeared in the network. | |
int | addListener (INodeInfoListener *listener) |
Adds one listener. | |
void | removeListener (INodeInfoListener *listener) |
Removes the listener. | |
uint8_t | getNumRequestAttempts () const |
Number of attempts to retrieve GetNodeInfo response before giving up on the assumption that the service is not implemented. | |
MonotonicDuration | getRequestInterval () const |
Request interval also implicitly defines the maximum number of concurrent requests. | |
bool | isRetrievingInProgress () const |
These methods are needed mostly for testing. | |
int | start () |
Starts the monitor. | |
void | forgetNode (NodeID node_id) |
Make the node unknown. | |
void | forgetAllNodes () |
Make all nodes unknown. | |
NodeStatus | getNodeStatus (NodeID node_id) const |
Returns status of a given node. | |
bool | isNodeKnown (NodeID node_id) const |
Whether the class has observed this node at least once since initialization. | |
NodeID | findNodeWithWorstHealth () const |
This helper method allows to quickly estimate the overall network health. | |
template<typename Operator > | |
void | forEachNode (Operator op) const |
Calls the operator for every known node. | |
Private Member Functions | |
void | startOneShotWithDeadline (MonotonicTime deadline) |
Various ways to start the timer - periodically or once. | |
MonotonicDuration | getPeriod () const |
Returns period if the timer is in periodic mode. |
Detailed Description
This class automatically retrieves a response to GetNodeInfo once a node appears online or restarts.
It does a number of attempts in case if there's a communication failure before assuming that the node does not implement the GetNodeInfo service. All parameters are pre-configured with sensible default values that should fit virtually any use case, but they can be overriden if needed - refer to the setter methods below for details.
Defaults are pre-configured so that the class is able to query 123 nodes (node ID 1..125, where 1 is our local node and 1 is one node that implements GetNodeInfo service, hence 123) of which none implements GetNodeInfo service in under 5 seconds. The 5 second limitation is imposed by UAVCAN-compatible bootloaders, which are unlikely to wait for more than that before continuing to boot. In case if this default value is not appropriate for the end application, the request interval can be overriden via setRequestInterval().
Following the above explained requirements, the default request interval is defined as follows: request interval [ms] = floor(5000 [ms] bootloader timeout / 123 nodes) Which yields 40 ms.
Given default service timeout 1000 ms and the defined above request frequency 40 ms, the maximum number of concurrent requests will be: max concurrent requests = ceil(1000 [ms] timeout / 40 [ms] request interval) Which yields 25 requests.
Keep the above equations in mind when changing the default request interval.
Obviously, if all calls are completing in under (request interval), the number of concurrent requests will never exceed one. This is actually the most likely scenario.
Note that all nodes are queried in a round-robin fashion, regardless of their uptime, number of requests made, etc.
Events from this class can be routed to many listeners, INodeInfoListener.
Definition at line 91 of file node_info_retriever.hpp.
Member Function Documentation
int addListener | ( | INodeInfoListener * | listener ) |
Adds one listener.
Does nothing if such listener already exists. May return -ErrMemory if there's no space to add the listener.
Definition at line 386 of file node_info_retriever.hpp.
NodeID findNodeWithWorstHealth | ( | ) | const [inherited] |
This helper method allows to quickly estimate the overall network health.
Health of the local node is not considered. Returns an invalid Node ID value if there's no known nodes in the network.
Definition at line 274 of file node_status_monitor.hpp.
void forEachNode | ( | Operator | op ) | const [inherited] |
Calls the operator for every known node.
Operator signature: void (NodeID, NodeStatus)
Definition at line 303 of file node_status_monitor.hpp.
void forgetAllNodes | ( | ) | [inherited] |
Make all nodes unknown.
Definition at line 223 of file node_status_monitor.hpp.
void forgetNode | ( | NodeID | node_id ) | [inherited] |
Make the node unknown.
Definition at line 207 of file node_status_monitor.hpp.
NodeStatus getNodeStatus | ( | NodeID | node_id ) | const [inherited] |
Returns status of a given node.
Unknown nodes are considered offline. Complexity O(1).
Definition at line 236 of file node_status_monitor.hpp.
uint8_t getNumRequestAttempts | ( | ) | const |
Number of attempts to retrieve GetNodeInfo response before giving up on the assumption that the service is not implemented.
Zero is a special value that can be used to set unlimited number of attempts, UnlimitedRequestAttempts.
Definition at line 422 of file node_info_retriever.hpp.
MonotonicDuration getRequestInterval | ( | ) | const |
Request interval also implicitly defines the maximum number of concurrent requests.
Read the class documentation for details.
Definition at line 432 of file node_info_retriever.hpp.
void invalidateAll | ( | ) |
This method forces the class to re-request uavcan.protocol.GetNodeInfo from all nodes as if they have just appeared in the network.
Definition at line 370 of file node_info_retriever.hpp.
bool isNodeKnown | ( | NodeID | node_id ) | const [inherited] |
Whether the class has observed this node at least once since initialization.
Complexity O(1).
Definition at line 259 of file node_status_monitor.hpp.
bool isRetrievingInProgress | ( | ) | const |
These methods are needed mostly for testing.
Definition at line 452 of file node_info_retriever.hpp.
void removeListener | ( | INodeInfoListener * | listener ) |
Removes the listener.
If the listener was not registered, nothing will be done.
Definition at line 403 of file node_info_retriever.hpp.
int start | ( | ) | [inherited] |
Starts the monitor.
Destroy the object to stop it. Returns negative error code.
Definition at line 193 of file node_status_monitor.hpp.
int start | ( | const TransferPriority | priority = TransferPriority::OneHigherThanLowest ) |
Starts the retriever.
Destroy the object to stop it. Returns negative error code.
Definition at line 347 of file node_info_retriever.hpp.
Generated on Tue Jul 12 2022 17:17:37 by
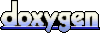