STMPE610 touch sensor driver library
Dependents: TS_Eyes Tokei testUniGraphic_150217 AfficheurTFTAdafruit ... more
SPI_STMPE610.h
00001 /* mbed SPI_STMPE610.h to test adafruit 2.8" TFT LCD shield w Touchscreen 00002 * Copyright (c) 2014 Motoo Tanaka @ Design Methodology Lab 00003 * 00004 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00005 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00006 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00007 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00008 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00009 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00010 * THE SOFTWARE. 00011 */ 00012 /* 00013 * Note: Since the interrupt siganl of the shield was not connected 00014 * to an interrupt pin on my frdm-kl25z, I just used polling mode. 00015 */ 00016 /* 00017 * Note: To make this work with FRDM-K64F 00018 * PTA0 must be disconnected from the swd clk by cutting J11. 00019 * But to re-active SWD you need to put jumper header to J11 00020 * so that it can be re-connected by a jumper. 00021 */ 00022 #ifndef SPI_STMPE610_H 00023 #define SPI_STMPE610_H 00024 00025 #include "mbed.h" 00026 00027 00028 /** SPI_STMPE610 Touch Sensor 00029 * Example: 00030 * @code 00031 * #include "mbed.h" 00032 * #include "SPI_STMPE610.h" 00033 * 00034 * #define PIN_MOSI PTD2 00035 * #define PIN_MISO PTD3 00036 * #define PIN_SCLK PTD1 00037 * #define PIN_CS_TSC PTA13 00038 * #define PIN_TSC_INTR PTC9 00039 * 00040 * SPI_STMPE610 TSC(PIN_MOSI, PIN_MISO, PIN_SCLK, PIN_CS_TSC) ; 00041 * 00042 * int main() 00043 * { 00044 * uint16_t touched, x, y, z ; 00045 * printf("Test SPI STMPE610\n\r") ; 00046 * while (true) { 00047 * touched = TSC.getRAWPoint(&x, &y, &z) ; 00048 * if (touched) { 00049 * printf("x = %d, y = %d, z = %d\n\r", x, y, z) ; 00050 * } 00051 * wait(0.1) ; 00052 * } 00053 * } 00054 * @endcode 00055 */ 00056 00057 class SPI_STMPE610 00058 { 00059 public: 00060 /** 00061 * STMPE610 constructor 00062 * 00063 * @param mosi SPI_MOSI pin 00064 * @param miso SPI_MISO pin 00065 * @param sclk SPI_CLK pin 00066 * @param cs SPI_CS pin 00067 */ 00068 00069 SPI_STMPE610(PinName mosi, PinName miso, PinName sclk, PinName cs) ; 00070 00071 /** 00072 * Destructor 00073 */ 00074 ~SPI_STMPE610() ; 00075 00076 /* 00077 * some member functions here (yet to be written) 00078 */ 00079 00080 SPI m_spi; 00081 DigitalOut m_cs ; 00082 int _mode ; 00083 00084 void readRegs(int addr, uint8_t *data, int len) ; 00085 void writeRegs(uint8_t *data, int len) ; 00086 uint8_t read8(int addr) ; 00087 void write8(int addr, uint8_t data) ; 00088 uint16_t read16(int addr) ; 00089 void write16(int addr, uint16_t data) ; 00090 00091 /** 00092 * specify spi frequency from this class 00093 * @param freq frequency in Hz 00094 */ 00095 void spi_frequency(unsigned long freq) ; 00096 00097 /** 00098 * specify spi format from this class 00099 * @param bits number of bits per spi frame 00100 * @param mode clock polarity and phase mode 00101 * @note please refer to the spi class for more details 00102 */ 00103 void spi_format(int bits, int mode) ; 00104 00105 /** 00106 * get RAW value of x, y, z 00107 * @param *x raw value of x 00108 * @param *y raw value of y 00109 * @param *z raw value of z 00110 * @return if touched 00111 * @note For my device usually the value seems to be between 300 ~ 3000 00112 * @note when it fails to acquire value the value of 0 seems to be returned 00113 */ 00114 int getRAWPoint(uint16_t *x, uint16_t *y, uint16_t *z) ; 00115 00116 /** 00117 * get value of x, y, z 00118 * @param *x x value relative to TFT 00119 * @param *y y value relative to TFT 00120 * @param *z z RAW value if non-null pointer is passed 00121 * @note default calibration was for my particular device 00122 * @note for more accuracy measure your device and modify 00123 * @note those values in SPI_STMPE610.c 00124 */ 00125 int getPoint(uint16_t *x, uint16_t *y, uint16_t *z = 0) ; 00126 00127 void calibrate(int x_at_10, int y_at_10, int x_at_230, int y_at_310) ; 00128 00129 private: 00130 float hramp ; 00131 float hoffset ; 00132 float vramp ; 00133 float voffset ; 00134 } ; 00135 00136 #endif /* SPI_STMPE610_H */
Generated on Tue Jul 12 2022 19:25:25 by
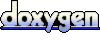