SRF02 sensor
Fork of SRF02 by
Embed:
(wiki syntax)
Show/hide line numbers
SRF02.h
Go to the documentation of this file.
00001 /** 00002 @file SRF02.h 00003 00004 @brief Header file containing member functions and variables 00005 00006 */ 00007 00008 #ifndef SRF02_H 00009 #define SRF02_H 00010 00011 // addresses 00012 #define SRF02_R_ADD 0xE1 00013 #define SRF02_W_ADD 0xE0 00014 00015 // registers 00016 #define CMD_REG 0x00 00017 #define RANGE_H_REG 0x02 00018 #define RANGE_L_REG 0x03 00019 00020 // commands 00021 #define INCH_CMD 0x50 00022 #define CM_CMD 0x51 00023 #define US_CMD 0x52 00024 00025 #include "mbed.h" 00026 00027 /** 00028 @brief Library for interfacing with SRF02 Ultrasonic Sensor in I2C 00029 @see http://www.robot-electronics.co.uk/htm/srf02tech.htm 00030 00031 @brief Revision 1.0 00032 00033 @author Craig A. Evans 00034 @date March 2014 00035 * 00036 * Example: 00037 * @code 00038 00039 #include "mbed.h" 00040 #include "SRF02.h" 00041 00042 int main() { 00043 00044 while(1) { 00045 00046 // read sensor distance in cm and print over serial port 00047 int distance = sensor.getDistanceCm(); 00048 serial.printf("Distance = %d cm\n",distance); 00049 // short delay before next measurement 00050 wait(0.5); 00051 00052 } 00053 } 00054 * @endcode 00055 */ 00056 00057 class SRF02 00058 { 00059 public: 00060 00061 /** Create a SRF02 object connected to the specified I2C pins 00062 * 00063 * @param sdaPin - mbed SDA pin 00064 * @param sclPin - mbed SCL pin 00065 * 00066 */ 00067 SRF02(PinName sdaPin, PinName sclPin); 00068 /** Read distance in centimetres 00069 * 00070 * @returns distance in centimetres (int) 00071 * 00072 */ 00073 int getDistanceCm(); 00074 int averageDistance(); 00075 00076 private: 00077 /** Hangs in infinite loop flashing 'blue lights of death' 00078 * 00079 */ 00080 void error(); 00081 00082 00083 private: // private variables 00084 I2C* i2c; 00085 BusOut* leds; 00086 }; 00087 00088 #endif
Generated on Thu Jul 14 2022 09:11:56 by
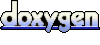