SRF02 sensor
Fork of SRF02 by
Embed:
(wiki syntax)
Show/hide line numbers
SRF02.cpp
Go to the documentation of this file.
00001 /** 00002 @file SRF02.cpp 00003 00004 @brief Member functions implementations 00005 00006 */ 00007 #include "mbed.h" 00008 #include "SRF02.h" 00009 00010 SRF02::SRF02(PinName sdaPin, PinName sclPin) 00011 { 00012 i2c = new I2C(sdaPin,sclPin); // create new I2C instance and initialise 00013 i2c->frequency(400000); // I2C Fast Mode - 400kHz 00014 leds = new BusOut(LED4,LED3,LED2,LED1); 00015 00016 } 00017 00018 int SRF02::getDistanceCm() 00019 { 00020 char data[2]; 00021 00022 // need to send CM command to command register 00023 data[0] = CMD_REG; 00024 data[1] = CM_CMD; 00025 int ack = i2c->write(SRF02_W_ADD,data,2); 00026 if (ack) 00027 error(); // if we don't receive acknowledgement, flash error message 00028 00029 // this will start the sensor ranging, the datasheet suggests a delay of at least 65 ms before reading the result 00030 wait_ms(70); 00031 00032 // we can now read the result - tell the sensor we want the high byte 00033 char reg = RANGE_H_REG; 00034 ack = i2c->write(SRF02_W_ADD,®,1); 00035 if (ack) 00036 error(); // if we don't receive acknowledgement, flash error message 00037 00038 // if we read two bytes, the register is automatically incremented (H and L) 00039 ack = i2c->read(SRF02_R_ADD,data,2); 00040 if (ack) 00041 error(); // if we don't receive acknowledgement, flash error message 00042 00043 // high byte is first, then low byte, so combine into 16-bit value 00044 return (data[0] << 8) | data[1]; 00045 } 00046 00047 void SRF02::error() 00048 { 00049 while(1) { 00050 leds->write(15); 00051 wait(0.1); 00052 leds->write(0); 00053 wait(0.1); 00054 } 00055 }
Generated on Thu Jul 14 2022 09:11:56 by
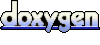